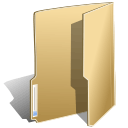
.NET (1273)
Children categories
Editing a Word document is necessary when you want to improve readability, correct errors, refine formatting, maintain consistency, adapt content, facilitate collaboration, and optimize the document for any other purposes. Programmatically editing a Word document using C# can be a powerful approach to automate document processing and manipulation tasks.
In this article, you will learn how to edit a Word document using C# and the Spire.Doc for .NET library.
- Modify Text in a Word Document
- Change Formatting of Text in a Word Document
- Add New Elements to a Word Document
- Remove Paragraphs from a Word Document
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Modify Text in a Word Document in C#
Spire.Doc allows you to programmatically access specific sections and paragraphs in Word documents. To retrieve a particular section, use the Document.Sections[index] property. Then, to get a particular paragraph within that section, leverage the Section.Paragraphs[index] property. Finally, you can update the text content of the paragraph using the Paragraph.Text property.
The steps to modify text in a Word document using C# are as follows:
- Create a Document object.
- Load a Word file from the given file path.
- Get a specific section through Document.Sections[index] property.
- Get a specific paragraph through Section.Paragraphs[index] property.
- Reset the text of the paragraph through Paragraph.Text property.
- Save the updated document to a different Word file.
- C#
using Spire.Doc; using Spire.Doc.Documents; namespace ModifyText { class Program { static void Main(string[] args) { // Create a new document object Document document = new Document(); // Load an existing Word file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Get a specific section Section section = document.Sections[0]; // Get a specific paragraph Paragraph paragraph = section.Paragraphs[0]; // Modify the text of the paragraph paragraph.Text = "Updated Title"; // Save the document to a different Word file document.SaveToFile("ModifyText.docx", FileFormat.Docx); // Dispose resource document.Dispose(); } } }
Change Formatting of Text in a Word Document in C#
To change the text formatting within a paragraph, first obtain the paragraph object, then iterate through its child objects to locate the individual text ranges. For each text range, you can reset the formatting using the CharacterFormat property of the TextRange.
The steps to change text formatting in a Word document are as follows:
- Create a Document object.
- Load a Word file from the given file path.
- Get a specific section through Document.Sections[index] property.
- Get a specific paragraph through Section.Paragraphs[index] property.
- Iterate through the child objects in the paragraph.
- Determine if a child object is a text range.
- Get a specific text range.
- Reset the text formatting through TextRange.CharacterFormat property.
- Save the updated document to a different Word file.
- C#
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; using System.Drawing; namespace ChangeTextFont { class Program { static void Main(string[] args) { // Create a new document object Document document = new Document(); // Load an existing Word file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Get a specific section Section section = document.Sections[0]; // Get a specific paragraph Paragraph paragraph = section.Paragraphs[2]; // Iterate through the child objects in the paragraph for (int i = 0; i < paragraph.ChildObjects.Count; i++) { // Determine if a child object is text range if (paragraph.ChildObjects[i] is TextRange) { // Get a specific text range TextRange textRange = (TextRange)paragraph.ChildObjects[i]; // Reset font name for it textRange.CharacterFormat.FontName = "Corbel Light"; // Reset font size for it textRange.CharacterFormat.FontSize = 11; // Reset text color for it textRange.CharacterFormat.TextColor = Color.Blue; // Apply italic to the text range textRange.CharacterFormat.Italic = true; } } // Save the document to a different Word file document.SaveToFile("ChangeFont.docx", FileFormat.Docx); // Dispose resource document.Dispose(); } } }
Add New Elements to a Word Document in C#
In addition to modifying the existing content in a Word document, you can also insert various types of new elements, such as text, images, tables, lists, and charts. As most elements are paragraph-based, you have the flexibility to add a new paragraph at the end of the document or insert it mid-document. You can then populate this new paragraph with the desired content, whether that's plain text, images, or other elements.
Below are the steps to add new elements (text and images) to a Word document using C#:
- Create a Document object.
- Load a Word file from the given file path.
- Get a specific section through Document.Sections[index] property.
- Add a paragraph to the section using Section.AddParagraph() method.
- Add text to the paragraph using Paragraph.AppendText() method.
- Add an image to the paragraph using Paragraph.AppendPicture() method.
- Save the updated document to a different Word file.
- C#
using Spire.Doc; using Spire.Doc.Documents; namespace AddNewElementsToWord { class Program { static void Main(string[] args) { // Create a new document object Document document = new Document(); // Load an existing Word file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Get the last section Section lastSection = document.LastSection; // Add a paragraph to the section Paragraph paragraph = lastSection.AddParagraph(); // Add text to the paragraph paragraph.AppendText("This text and the image shown below are added programmatically using C# and Spire.Doc for .NET."); // Add an image to the paragraph paragraph.AppendPicture("C:\\Users\\Administrator\\Desktop\\logo.png"); // Create a paragraph style ParagraphStyle style = new ParagraphStyle(document); style.Name = "FontStyle"; style.CharacterFormat.FontName = "Times New Roman"; style.CharacterFormat.FontSize = 12; document.Styles.Add(style); // Apply the style to the paragraph paragraph.ApplyStyle(style.Name); // Save the document to a different Word file document.SaveToFile("AddNewElements.docx", FileFormat.Docx); // Dispose resource document.Dispose(); } } }
Remove Paragraphs from a Word Document in C#
With the Spire.Doc library, you can perform a variety of document operations, including updating existing content, adding new elements, as well as removing elements from a Word document. For example, to remove a paragraph from the document, you can use the Section.Paragraphs.RemoveAt() method.
The following are the steps to remove paragraphs from a Word document using C#:
- Create a Document object.
- Load a Word file from the given file path.
- Get a specific section through Document.Sections[index] property.
- Remove a specific paragraph from the section using Section.Paragraphs.RemoveAt() method.
- Save the updated document to a different Word file.
- C#
using Spire.Doc; namespace RemoveParagraphs { class Program { static void Main(string[] args) { // Create a new document object Document document = new Document(); // Load an existing Word file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx"); // Get a specific section Section section = document.Sections[0]; // Remove a specific paragraph section.Paragraphs.RemoveAt(0); // Save the document to a different Word file document.SaveToFile("RemoveParagraph.docx", FileFormat.Docx); // Dispose resource document.Dispose(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
There's no doubt that Word document is one of the most popular document file types today. Because Word document is an ideal file format for generating letters, memos, reports, term papers, novels and magazines, etc. In this article, you will learn how to create a simple Word document from scratch in C# and VB.NET by using Spire.Doc for .NET.
Spire.Doc for .NET provides the Document class to represent a Word document model, allowing users to read and edit existing documents or create new ones. A Word document must contain at least one section (represented by Section class) and each section is a container for basic Word elements like paragraphs, tables, headers, footers and so on. The table below lists the important classes and methods involved in this tutorial.
Member | Description |
Document class | Represents a Word document model. |
Section class | Represents a section in a Word document. |
Paragraph class | Represents a paragraph in a section. |
ParagraphStyle class | Defines the font formatting information that can be applied to a paragraph. |
Section.AddParagraph() method | Adds a paragraph to a section. |
Paragraph.AppendText() method | Appends text to a paragraph at the end. |
Paragraph.ApplyStyle() method | Applies a style to a paragraph. |
Document.SaveToFile() method | Saves the document to a Word file with an extension of .doc or .docx. This method also supports saving the document to PDF, XPS, HTML, PLC, etc. |
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Create a Simple Word Document
The following are the steps to create a simple Word document that contains several paragraphs by using Spire.Doc for .NET.
- Create a Document object.
- Add a section using Document.AddSection() method.
- Set the page margins through Section.PageSetUp.Margins property.
- Add several paragraphs to the section using Section.AddParagraph() method.
- Add text to the paragraphs using Paragraph.AppendText() method.
- Create a ParagraphStyle object, and apply it to a specific paragraph using Paragraph.ApplyStyle() method.
- Save the document to a Word file using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; using System.Drawing; namespace CreateWordDocument { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Add a section Section section = doc.AddSection(); //Set the page margins section.PageSetup.Margins.All = 40f; //Add a paragraph as title Paragraph titleParagraph = section.AddParagraph(); titleParagraph.AppendText("Introduction of Spire.Doc for .NET"); //Add two paragraphs as body Paragraph bodyParagraph_1 = section.AddParagraph(); bodyParagraph_1.AppendText("Spire.Doc for .NET is a professional Word.NET library specifically designed " + "for developers to create, read, write, convert, compare and print Word documents on any.NET platform " + "(.NET Framework, .NET Core, .NET Standard, Xamarin & Mono Android) with fast and high-quality performance."); Paragraph bodyParagraph_2 = section.AddParagraph(); bodyParagraph_2.AppendText("As an independent Word .NET API, Spire.Doc for .NET doesn't need Microsoft Word to " + "be installed on neither the development nor target systems. However, it can incorporate Microsoft Word " + "document creation capabilities into any developers' .NET applications."); //Create a style for title paragraph ParagraphStyle style1 = new ParagraphStyle(doc); style1.Name = "titleStyle"; style1.CharacterFormat.Bold = true; style1.CharacterFormat.TextColor = Color.Purple; style1.CharacterFormat.FontName = "Times New Roman"; style1.CharacterFormat.FontSize = 12; doc.Styles.Add(style1); titleParagraph.ApplyStyle("titleStyle"); //Create a style for body paragraphs ParagraphStyle style2 = new ParagraphStyle(doc); style2.Name = "paraStyle"; style2.CharacterFormat.FontName = "Times New Roman"; style2.CharacterFormat.FontSize = 12; doc.Styles.Add(style2); bodyParagraph_1.ApplyStyle("paraStyle"); bodyParagraph_2.ApplyStyle("paraStyle"); //Set the horizontal alignment of paragraphs titleParagraph.Format.HorizontalAlignment = HorizontalAlignment.Center; bodyParagraph_1.Format.HorizontalAlignment = HorizontalAlignment.Justify; bodyParagraph_2.Format.HorizontalAlignment = HorizontalAlignment.Justify; //Set the first line indent bodyParagraph_1.Format.FirstLineIndent = 30; bodyParagraph_2.Format.FirstLineIndent = 30; //Set the after spacing titleParagraph.Format.AfterSpacing = 10; bodyParagraph_1.Format.AfterSpacing = 10; //Save to file doc.SaveToFile("WordDocument.docx", FileFormat.Docx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Spire.Doc for .NET is a professional Word .NET library specifically designed for developers to create, read, write, convert, compare and print Word documents on any .NET platform (Target .NET Framework, .NET Core, .NET Standard, .NET 5.0, .NET 6.0, Xamarin & Mono Android) with fast and high quality performance.
As an independent Word .NET API, Spire.Doc for .NET doesn't need Microsoft Word to be installed on neither the development nor target systems. However, it can incorporate Microsoft Word document creation capabilities into any developers' .NET applications.
No matter what users want to do on Word document, they should open it. This guide demonstrates several solutions to open Word in C# and VB.NET via Spire.Doc for .NET.
Open Existing Word
Spire.Doc for .NET provides a Document(String) constructor to enable users to initialize a new instance of Document class from the specified existing document.
Document document = new Document(@"E:\Work\Documents\Spire.Doc for .NET.docx");
Dim document As New Document("E:\Work\Documents\Spire.Doc for .NET.docx")
Spire.Doc for .NET also provides Document.LoadFromFile(String) method of Document class to open a Word document. The Word document can be .doc(Word 97-2003), .docx(Word 2007 and 2010) and .docm(Word with macro).
Document document = new Document(); document.LoadFromFile(@"E:\Work\Documents\Spire.Doc for .NET.docx");
Dim document As New Document() document.LoadFromFile("E:\Work\Documents\Spire.Doc for .NET.docx")
Open Word in Read Mode
Spire.Doc for .NET provides Document.LoadFromFileInReadMode(String, FileFormat) method of Document class to load Word in Read-Only mode.
Document document = new Document(); document.LoadFromFileInReadMode(@"E:\Work\Documents\Spire.Doc for .NET.docx",FileFormat.Docx);
Dim document As New Document() document.LoadFromFileInReadMode("E:\Work\Documents\Spire.Doc for .NET.docx", FileFormat.Docx)
Load Word from Stream
Spire.Doc for .NET provides the constructor Document(Stream) to initialize a new instance of Document class from specified data stream and the method Document.LoadFromStream(Stream, FileFormat) to open document from Stream in XML or Microsoft Word document.
Stream stream = File.OpenRead(@"E:\Work\Documents\Spire.Doc for .NET.docx"); Document document = new Document(stream);
Stream stream = File.OpenRead(@"E:\Work\Documents\Spire.Doc for .NET.docx"); Document document = new Document(); document.LoadFromStream(stream, FileFormat.Docx);
Dim stream As Stream = File.OpenRead("E:\Work\Documents\Spire.Doc for .NET.docx") Dim document As New Document(stream)
Dim stream As Stream = File.OpenRead("E:\Work\Documents\Spire.Doc for .NET.docx") Dim document As New Document() document.LoadFromStream(stream, FileFormat.Docx)
Spire.Doc, an easy-to-use component to operate Word document, allows developers to fast generate, write, edit and save Word (Word 97-2003, Word 2007, Word 2010) in C# and VB.NET for .NET, Silverlight and WPF.
The sample demonstrates how to work with Word page setup.
The sample demonstrates how to set document properties.
The sample demonstrates how to handle merge event.
The sample demonstrates how to export doc document to PDF file.
The sample demonstrates how to export doc document to XML file.
The sample demonstrates how to export doc document to TIFF image.
