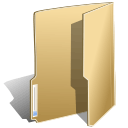
.NET (1270)
Children categories
In MS Word, the tables can organize and present data in rows and columns, which makes the information easier to understand and analyze. In this article, you will learn how to programmatically create a table with data in a Word document using Spire.Doc for .NET.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Create a Simple Table in Word
Below are some of the core classes and methods provided by Spire.Doc for .NET for creating and formatting tables in Word.
Name | Description |
Table Class | Represents a table in a Word document. |
TableRow Class | Represents a row in a table. |
TableCell Class | Represents a specific cell in a table. |
Section.AddTbale() Method | Adds a new table to the specified section. |
Table.ResetCells() Method | Resets row number and column number. |
Table.Rows Property | Gets the table rows. |
TableRow.Height Property | Sets the height of the specified row. |
TableRow.Cells Property | Returns the cells collection. |
TableRow.RowFormat Property | Gets the format of the specified row. |
The detailed steps are as follows
- Create a Document object and add a section to it.
- Prepare the data for the header row and other rows, storing them in a one-dimensional string array and a two-dimensional string array respectively.
- Add a table to the section using Section.AddTable() method.
- Insert data to the header row, and set the row formatting, including row height, background color, and text alignment.
- Insert data to the rest of the rows and apply formatting to these rows.
- Save the document to another file using Document.SaveToFile() method.
- C#
- VB.NET
using System; using System.Drawing; using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; namespace WordTable { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Add a section Section s = doc.AddSection(); //Define the data for the table String[] Header = { "Date", "Description", "Country", "On Hands", "On Order" }; String[][] data = { new String[]{ "08/07/2021","Dive kayak","United States","24","16"}, new String[]{ "08/07/2021","Underwater Diver Vehicle","United States","5","3"}, new String[]{ "08/07/2021","Regulator System","Czech Republic","165","216"}, new String[]{ "08/08/2021","Second Stage Regulator","United States","98","88"}, new String[]{ "08/08/2021","Personal Dive Sonar","United States","46","45"}, new String[]{ "08/09/2021","Compass Console Mount","United States","211","300"}, new String[]{ "08/09/2021","Regulator System","United Kingdom","166","100"}, new String[]{ "08/10/2021","Alternate Inflation Regulator","United Kingdom","47","43"}, }; //Add a table Table table = s.AddTable(true); table.ResetCells(data.Length + 1, Header.Length); //Set the first row as table header TableRow FRow = table.Rows[0]; FRow.IsHeader = true; //Set the height and color of the first row FRow.Height = 23; FRow.RowFormat.BackColor = Color.LightSeaGreen; for (int i = 0; i < Header.Length; i++) { //Set alignment for cells Paragraph p = FRow.Cells[i].AddParagraph(); FRow.Cells[i].CellFormat.VerticalAlignment = VerticalAlignment.Middle; p.Format.HorizontalAlignment = HorizontalAlignment.Center; //Set data format TextRange TR = p.AppendText(Header[i]); TR.CharacterFormat.FontName = "Calibri"; TR.CharacterFormat.FontSize = 12; TR.CharacterFormat.Bold = true; } //Add data to the rest of rows and set cell format for (int r = 0; r < data.Length; r++) { TableRow DataRow = table.Rows[r + 1]; DataRow.Height = 20; for (int c = 0; c < data[r].Length; c++) { DataRow.Cells[c].CellFormat.VerticalAlignment = VerticalAlignment.Middle; Paragraph p2 = DataRow.Cells[c].AddParagraph(); TextRange TR2 = p2.AppendText(data[r][c]); p2.Format.HorizontalAlignment = HorizontalAlignment.Center; //Set data format TR2.CharacterFormat.FontName = "Calibri"; TR2.CharacterFormat.FontSize = 11; } } //Save the document doc.SaveToFile("WordTable.docx", FileFormat.Docx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Grouping rows and columns in an Excel worksheet can separate data into groups, and each group presents information about one item. You can then expand or collapse certain groups to display only the most important information. In this article, you'll learn how to group or ungroup Excel rows and columns using Spire.XLS for .NET in C# and VB.NET.
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.XLS
Group Rows and Columns
The following are the steps to group rows and columns using Spire.XLS for .NET.
- Create a Workbook object.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get the specific sheet through Workbook.Worksheets[index] property.
- Group rows using Worksheet.GroupByRows() method.
- Group columns using Worksheet.GroupByColumns() method.
- Save the result to another Excel file using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; namespace GroupRowsAndColumns { class Program { static void Main(string[] args) { //Create a Workbook object Workbook workbook = new Workbook(); //Load a sample Excel file workbook.LoadFromFile(@"C:\Users\Administrator\Desktop\sample.xlsx"); //Get the first worksheet Worksheet sheet = workbook.Worksheets[0]; //Group rows sheet.GroupByRows(2, 5, false); sheet.GroupByRows(7, 10, false); //Group columns sheet.GroupByColumns(5, 6, false); //Save to another Excel file workbook.SaveToFile("GroupRowsAndColumns.xlsx", ExcelVersion.Version2016); } } }
Ungroup Rows and Columns
The following are the steps to ungroup rows and columns using Spire.XLS for .NET.
- Create a Workbook object.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get the specific sheet through Workbook.Worksheets[index] property.
- Ungroup rows using Worksheet.UngroupByRows() method.
- Ungroup columns using Worksheet.UngroupByColumns() method.
- Save the result to another Excel file using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; namespace UngroupRowsAndColumns { class Program { static void Main(string[] args) { //Create a Workbook object Workbook workbook = new Workbook(); //Load a sample Excel file workbook.LoadFromFile(@"C:\Users\Administrator\Desktop\sample.xlsx"); //Get the first worksheet Worksheet sheet = workbook.Worksheets[0]; //Ungroup rows sheet.UngroupByRows(2, 5); sheet.UngroupByRows(7, 10); //Ungroup columns sheet.UngroupByColumns(5, 6); //Save to a different Excel file workbook.SaveToFile("UngroupRowsAndColumns.xlsx", ExcelVersion.Version2016); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
In Word documents, indentation is a paragraph format used to adjust the distance between paragraph body and page margin. It includes left indent, right indent, first line indent and hanging indent. Left indent and right indent can be applied to all lines of a paragraph, while first line indent can only be applied to first line of a paragraph. As for the hanging indent, it can be applied to every line of the paragraph except the first one. This article introduces how to programmatically set paragraph indents in a Word document using Spire.Doc for .NET.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Set Paragraph Indents in Word
The table below lists some of the core classes and properties that are used to set different paragraph indents in a Word document.
Name | Description |
ParagraphFormat Class | Represents the format of a paragraph. |
ParagraphFormat.LeftIndent Property | Returns or sets the value that represents the left indent for paragraph. |
ParagraphFormat.RightIndent Property | Returns or sets the value that represents the right indent for paragraph. |
ParagraphFormat.FirstLineIndent Property | Gets or sets the value for first line or hanging indent. Positive value represents first-line indent, and Negative value represents hanging indent. |
The detailed steps are as follows:
- Create a Document instance.
- Load a sample Word document using Document.LoadFromFile() method.
- Get a specified section using Document.Sections[] property.
- Get a specified paragraph using Section.Paragraphs[] property.
- Get the paragraph format using Paragraph.Format property, and then set the paragraph indent using the above listed properties of ParagraphFormat class.
- Save the document to another file using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; namespace WordIndent { class Program { static void Main(string[] args) { //Create a Document instance Document doc = new Document(); //Load a sample Word document doc.LoadFromFile("sample.docx"); //Get the first paragraph and set left indent Paragraph para1 = doc.Sections[0].Paragraphs[0]; para1.Format.LeftIndent = 30; //Get the second paragraph and set right indent Paragraph para2 = doc.Sections[0].Paragraphs[1]; para2.Format.RightIndent = 30; //Get the third paragraph and set first line indent Paragraph para3 = doc.Sections[0].Paragraphs[2]; para3.Format.FirstLineIndent = 30; //Get the fourth paragraph and set hanging indent Paragraph para4 = doc.Sections[0].Paragraphs[3]; para4.Format.FirstLineIndent = -30; //Save the document to file doc.SaveToFile("Indent.docx", FileFormat.Docx2010); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
A page break is a marker that controls where one page ends and where a new page begins. If you want to move the content after a certain place to the next page in your Word document, you can insert a page break. In this article, you will learn how to insert page break into Word documents in C# and VB.NET using Spire.Doc for .NET library.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Insert Page Break after a Specific Paragraph
The following are the steps to insert page break after a specific paragraph:
- Create a Document instance.
- Load a Word document using Document.LoadFromFile() method.
- Get the desired section using Document.Sections[sectionIndex] property.
- Get the desired paragraph using Section.Paragraphs[paragraphIndex] property.
- Add a page break to the paragraph using Paragraph.AppendBreak(BreakType.PageBreak) method.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; namespace InsertPageBreakAfterParagraph { class Program { static void Main(string[] args) { //Create a Document instance Document document = new Document(); //Load a Word document document.LoadFromFile("Sample.docx"); //Get the first section Section section = document.Sections[0]; //Get the 2nd paragraph in the section Paragraph paragraph = section.Paragraphs[1]; //Append a page break to the paragraph paragraph.AppendBreak(BreakType.PageBreak); //Save the result document document.SaveToFile("InsertPageBreak.docx", FileFormat.Docx2013); } } }
Insert Page Break after a Specific Text
The following are the steps to insert a page break after a specific text:
- Create a Document instance.
- Load a Word document using Document.LoadFromFile() method.
- Find a specific text using Document.FindString() method.
- Access the text range of the searched text using TextSelection.GetAsOneRange() method.
- Get the paragraph where the text range is located using ParagraphBase.OwnerParagraph property.
- Get the position index of the text range in the paragraph using Paragraph.ChildObjects.IndexOf() method.
- Initialize an instance of Break class to create a page break.
- Insert the page break after the searched text using Paragraph.ChildObjects.Insert() method.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; using System; namespace InsertPageBreakAfterText { class Program { static void Main(string[] args) { //Create a Document instance Document document = new Document(); //Load a Word document document.LoadFromFile("Sample.docx"); //Search a specific text TextSelection selection = document.FindString("celebration", true, true); //Get the text range of the seached text TextRange range = selection.GetAsOneRange(); //Get the paragraph where the text range is located Paragraph paragraph = range.OwnerParagraph; //Get the position index of the text range in the paragraph int index = paragraph.ChildObjects.IndexOf(range); //Create a page break Break pageBreak = new Break(document, BreakType.PageBreak); //Insert the page break after the searched text paragraph.ChildObjects.Insert(index + 1, pageBreak); //Save the result document document.SaveToFile("InsertPageBreakAfterText.docx", FileFormat.Docx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
The comment feature in Microsoft Word provides an excellent way for people to add their insights or opinions to a Word document without having to change or interrupt the content of the document. If someone comments on a document, the document author or other users can reply to the comment to have a discussion with him, even if they're not viewing the document at the same time. This article will demonstrate how to add, reply to or delete comments in Word in C# and VB.NET using Spire.Doc for .NET library.
- Add a Comment to Paragraph in Word in C# and VB.NET
- Add a Comment to Text in Word in C# and VB.NET
- Reply to a Comment in Word in C# and VB.NET
- Delete Comments in Word in C# and VB.NET
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Add a Comment to Paragraph in Word in C# and VB.NET
Spire.Doc for .NET provides the Paragraph.AppendComment() method to add a comment to a specific paragraph. The following are the detailed steps:
- Initialize an instance of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Access a specific section in the document by its index through Document.Sections[int] property.
- Access a specific paragraph in the section by its index through Section.Paragraphs[int] property.
- Add a comment to the paragraph using Paragraph.AppendComment() method.
- Set the author of the comment through Comment.Format.Author property.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; namespace AddComments { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document document = new Document(); //Load a Word document document.LoadFromFile(@"Sample.docx"); //Get the first section in the document Section section = document.Sections[0]; //Get the first paragraph in the section Paragraph paragraph = section.Paragraphs[0]; //Add a comment to the paragraph Comment comment = paragraph.AppendComment("This comment is added using Spire.Doc for .NET."); //Set comment author comment.Format.Author = "Eiceblue"; comment.Format.Initial = "CM"; //Save the result document document.SaveToFile("AddCommentToParagraph.docx", FileFormat.Docx2013); document.Close(); } } }
Add a Comment to Text in Word in C# and VB.NET
The Paragraph.AppendComment() method is used to add comments to an entire paragraph. By default, the comment marks will be placed at the end of the paragraph. To add a comment to a specific text, you need to search for the text using Document.FindString() method, then place the comment marks at the beginning and end of the text. The following are the detailed steps:
- Initialize an instance of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Find the specific text in the document using Document.FindString() method.
- Create a comment start mark and a comment end mark, which will be placed at the beginning and end of the found text respectively.
- Initialize an instance of the Comment class to create a new comment. Then set the content and author for the comment.
- Get the owner paragraph of the found text. Then add the comment to the paragraph as a child object.
- Insert the comment start mark before the text range and the comment end mark after the text range.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; namespace AddCommentsToText { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document document = new Document(); //Load a Word document document.LoadFromFile(@"CommentTemplate.docx"); //Find a specific string TextSelection find = document.FindString("Microsoft Office", false, true); //Create the comment start mark and comment end mark CommentMark commentmarkStart = new CommentMark(document); commentmarkStart.Type = CommentMarkType.CommentStart; CommentMark commentmarkEnd = new CommentMark(document); commentmarkEnd.Type = CommentMarkType.CommentEnd; //Create a comment and set its content and author Comment comment = new Comment(document); comment.Body.AddParagraph().Text = "Developed by Microsoft."; comment.Format.Author = "Shaun"; //Get the found text as a single text range TextRange range = find.GetAsOneRange(); //Get the owner paragraph of the text range Paragraph para = range.OwnerParagraph; //Add the comment to the paragraph para.ChildObjects.Add(comment); //Get the index of text range in the paragraph int index = para.ChildObjects.IndexOf(range); //Set comment ID for the comment mark start and comment mark end commentmarkStart.CommentId = comment.Format.CommentId; commentmarkEnd.CommentId = comment.Format.CommentId; //Insert the comment start mark before the text range para.ChildObjects.Insert(index, commentmarkStart); //Insert the comment end mark after the text range para.ChildObjects.Insert(index + 2, commentmarkEnd); //Save the result document document.SaveToFile("AddCommentForText.docx", FileFormat.Docx2013); document.Close(); } } }
Reply to a Comment in Word in C# and VB.NET
To add a reply to an existing comment, you can use the Comment.ReplyToComment() method. The following are the detailed steps:
- Initialize an instance of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Get a specific comment in the document through Document.Comments[int] property.
- Initialize an instance of the Comment class to create a new comment. Then set the content and author for the comment.
- Add the new comment as a reply to the specific comment using Comment.ReplyToComment() method.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Fields; namespace ReplyToComments { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document document = new Document(); //Load a Word document document.LoadFromFile(@"AddCommentToParagraph.docx"); //Get the first comment in the document Comment comment1 = document.Comments[0]; //Create a new comment and specify its author and content Comment replyComment1 = new Comment(document); replyComment1.Format.Author = "Michael"; replyComment1.Body.AddParagraph().AppendText("Spire.Doc is a wonderful Word library."); //Add the comment as a reply to the first comment comment1.ReplyToComment(replyComment1); //Save the result document document.SaveToFile("ReplyToComment.docx", FileFormat.Docx2013); document.Close(); } } }
Delete Comments in Word in C# and VB.NET
Spire.Doc for .NET offers the Document.Comments.RemoveAt(int) method to remove a specific comment from a Word document and the Document.Comments.Clear() method to remove all comments from a Word document. The following are the detailed steps:
- Initialize an instance of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Delete a specific comment or all comments in the document using Document.Comments.RemoveAt(int) method or Document.Comments.Clear() method.
- Save the result document using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; namespace DeleteComments { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document document = new Document(); //Load a Word document document.LoadFromFile(@"AddCommentToParagraph.docx"); //Delete the first comment in the document document.Comments.RemoveAt(0); //Delete all comments in the document //document.Comments.Clear(); //Save the result document document.SaveToFile("DeleteComment.docx", FileFormat.Docx2013); document.Close(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Word Decryption is a process to decode encrypted Word document. It requires a password or secret key. If readers want to open and read a protected Word, they need to decrypt this Word document firstly. This guide demonstrates an easy and convenient solution to decrypt Word in C# and VB.NET via Spire.Doc for .NET.
Spire.Doc for .NET, specially developed for programmers to manipulate Word without Word Automation, provides users a method Document.LoadFromFile(String fileName, FileFormat fileFormat, String password) of Document class to open encrypted Word document. It also provides another method Document.RemoveEncryption() to decrypt Word without any protection. Through these two methods, users can decrypt Word easily with Spire.Doc for .NET. Download and install Spire.Doc for .NET. Then follow the code to decrypt.
using Spire.Doc; namespace DecryptWord { class Decryption { static void Main(string[] args) { //Load Encrypted Word Document document = new Document(); document.LoadFromFile(@"E:\Work\Documents\Student Transcript.docx", FileFormat.Docx,"123456"); //Decrypt document.RemoveEncryption(); //Save and Launch document.SaveToFile("decryption.docx", FileFormat.Docx); System.Diagnostics.Process.Start("decryption.docx"); } } }
Imports Spire.Doc Namespace DecryptWord Friend Class Decryption Shared Sub Main(ByVal args() As String) 'Load Encrypted Word Dim document As New Document() document.LoadFromFile("E:\Work\Documents\Student Transcript.docx", FileFormat.Docx, "123456") 'Decrypt document.RemoveEncryption() 'Save and Launch document.SaveToFile("decryption.docx", FileFormat.Docx) System.Diagnostics.Process.Start("decryption.docx") End Sub End Class End Namespace
Spire.Doc, professional Word component, is specially designed for developers to fast generate, write, modify and save Word documents in .NET, Silverlight and WPF with C# and VB.NET. Also, it supports conversion between Word and other popular formats, such as PDF, HTML, Image, Text and so on, in .NET and WPF platform.
In today's digital era, the skill of converting Word documents to PDF has become indispensable for individuals and organizations alike. The ability to transform Word files into the PDF format has a wide range of applications, including submitting official reports, distributing e-books, and archiving important files. Through this conversion process, documents can be seamlessly shared, accessed, and preserved for the long term, ensuring convenience, compatibility, and enhanced document management.
In this article, you will learn how to convert Word to PDF in C# and how to set conversion options as well using Spire.Doc for .NET.
- Convert Word to PDF in C#
- Convert Word to PDF/A in C#
- Convert Word to Password-Protected PDF in C#
- Convert a Specific Section in Word to PDF in C#
- Change Page Size while Converting Word to PDF in C#
- Set Image Quality while Converting Word to PDF in C#
- Embed Fonts while Converting Word to PDF in C#
- Create Bookmarks while Converting Word to PDF in C#
- Disable Hyperlinks while Converting Word to PDF in C#
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Convert Word to PDF in C#
Converting a Word document to a standard PDF using Spire.Doc is a simple task. To get started, you can utilize the LoadFromFile() or LoadFromStream() method from the Document object to load a Word document from a given file path or stream. Then, you can effortlessly convert it as a PDF file by employing the SaveToFile() method.
To convert Word to PDF in C#, follow these steps.
- Create a Document object.
- Load a sample Word document using Document.LoadFromFile() method.
- Save the document to PDF using Doucment.SaveToFile() method.
- C#
using Spire.Doc; namespace ConvertWordToPdf { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Save the document to PDF doc.SaveToFile("ToPDF.pdf", FileFormat.PDF); // Dispose resources doc.Dispose(); } } }
Convert Word to PDF/A in C#
PDF/A is a specialized format that focuses on preserving electronic documents for long-term use, guaranteeing that the content remains accessible and unaltered as time goes on.
To specify the conformance level of the resulting PDF when converting a document, you can make use of the PdfConformanceLevel property found within the ToPdfParameterList object. By passing this object as an argument to the SaveToFile() method, you can indicate the desired conformance level during the conversion process.
The steps to convert Word to PDF/A in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create a ToPdfParameterList object, which is used to set the conversion options.
- Set the conformance level for the generated PDF using PdfConformanceLevel property of the ToPdfParameterList object.
- Save the Word document to PDF/A using Doucment.SaveToFile(string fileName, ToPdfParameterList paramList) method.
- C#
using Spire.Doc; namespace ConvertWordToPdfa { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Create a ToPdfParameterList object ToPdfParameterList parameters = new ToPdfParameterList(); // Set the conformance level for PDF parameters.PdfConformanceLevel = PdfConformanceLevel.Pdf_A1A; // Save the document to a PDF file doc.SaveToFile("ToPdfA.pdf", parameters); // Dispose resources doc.Dispose(); } } }
Convert Word to Password-Protected PDF in C#
Transforming a Word document into a PDF that is protected by a password is a straightforward and efficient method to safeguard sensitive information and maintain its confidentiality and security.
To accomplish this, you can utilize the PdfSecurity.Encrypt() method, which is available within the ToPdfParameterList object. This method enables you to specify both an open password and a permission password for the resulting PDF file. By passing the ToPdfParameterList object as a parameter to the SaveToFile() method, these encryption settings will be implemented during the saving process.
The steps to convert Word to password-protected PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create a ToPdfParameterList object, which is used to set the conversion options.
- Set the open password and permission password for the generated PDF using ToPdfParameterList.PdfSecurity.Encrypt() method.
- Save the Word document to a password protected PDF using Doucment.SaveToFile(string fileName, ToPdfParameterList paramList) method.
- C#
using Spire.Doc; namespace ConvertWordToPasswordProtectedPdf { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Create a ToPdfParameterList object ToPdfParameterList parameters = new ToPdfParameterList(); // Set open password and permission password for PDF parameters.PdfSecurity.Encrypt("openPsd", "permissionPsd", PdfPermissionsFlags.None, PdfEncryptionKeySize.Key128Bit); // Save the document to PDF doc.SaveToFile("PasswordProtected.pdf", parameters); // Dispose resources doc.Dispose(); } } }
Convert a Specific Section in Word to PDF in C#
Being able to convert a specific section of a Microsoft Word document to a PDF can be highly advantageous when you want to extract a portion of a larger document for sharing or archiving purposes.
With the assistance of Spire.Doc, users can create a new Word document that contains the desired section from the source document by employing the Section.Clone() method and the Sections.Add() method. This new document can be then saved as a PDF file.
The following are the steps to convert a specific section of a Word document to PDF in C#.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create another Document object for holding one section from the source document.
- Create a copy of a desired section of the source document using Section.Clone() method.
- Add the copy to the new document using Sections.Add() method.
- Save the new Word document to PDF using Doucment.SaveToFile() method.
- C#
using Spire.Doc; namespace ConvertSectionToPdf { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Get a specific section of the document Section section = doc.Sections[1]; // Create a new document object Document newDoc = new Document(); // Clone the default style to the new document doc.CloneDefaultStyleTo(newDoc); // Clone the section to the new document newDoc.Sections.Add(section.Clone()); // Save the new document to PDF newDoc.SaveToFile("SectionToPDF.pdf", FileFormat.PDF); // Dispose resources doc.Dispose(); newDoc.Dispose(); } } }
Change Page Size while Converting Word to PDF in C#
When converting a Word document to PDF, it may be necessary to modify the page size to align with standard paper sizes like Letter, Legal, Executive, A4, A5, B5, and others. Alternatively, you might need to customize the page dimensions to meet specific requirements.
By making use of the PageSetup.PageSize property, you can adjust the page size of the Word document to either a standard paper size or a custom paper size. This page configuration will be applied during the conversion process, ensuring that the resulting PDF file reflects the desired page dimensions.
The steps to change the page size while convert Word to PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Iterate through the sections in the document, and change the page size of each section to a standard paper size or a custom size using PageSetup.PageSize property.
- Save the Word document to PDF using Doucment.SaveToFile() method.
- C#
using Spire.Doc; using Spire.Doc.Documents; using System.Drawing; namespace ChangePageSize { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Iterate through the sections in the document foreach (Section section in doc.Sections) { // Change the page size of each section to Letter section.PageSetup.PageSize = PageSize.Letter; // Change the page size of each section to a custom size // section.PageSetup.PageSize = new SizeF(500, 800); } // Save the document to PDF doc.SaveToFile("ChangePageSize.pdf", FileFormat.PDF); // Dispose resources doc.Dispose(); } } }
Set Image Quality while Converting Word to PDF in C#
When converting a Word document to PDF, it's essential to consider the quality of the images within the document. Balancing image integrity and file size is crucial to ensure an optimal viewing experience and efficient document handling.
Using Spire.Doc, you have the option to configure the image quality within the document by utilizing the JPEGQuality property of the Document object. For instance, by setting the value of JPEGQuality to 50, the image quality can be reduced to 50% of its original quality.
The steps to set image quality while converting Word to PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document for a given file path.
- Set the image quality using Document.JPEGQuality property.
- Save the document to PDF using Doucment.SaveToFile() method.
- C#
using Spire.Doc; namespace SetImageQuality { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Set the image quality to 50% of the original quality doc.JPEGQuality = 50; // Preserve original image quality // doc.JPEGQuality = 100; // Save the document to PDF doc.SaveToFile("SetImageQuality.pdf", FileFormat.PDF); // Dispose resources doc.Dispose(); } } }
Embed Fonts while Converting Word to PDF in C#
When fonts are embedded in a PDF, it ensures that viewers will see the exact font styles and types intended by the creator, regardless of whether they have the fonts installed on their system.
To include all the fonts used in a Word document in the resulting PDF, you can enable the embedding feature by setting the ToPdfParameterList.IsEmbeddedAllFonts property to true. Alternatively, if you prefer to specify a specific list of fonts to embed, you can make use of the EmbeddedFontNameList property.
The steps to embed fonts while converting Word to PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create a ToPdfParameterList object, which is used to set the conversion options.
- Embed all fonts in the generated PDF by settings IsEmbeddedAllFonts property to true.
- Save the Word document to PDF with fonts embedded using Doucment.SaveToFile(string fileName, ToPdfParameterList paramList) method.
- C#
using Spire.Doc; namespace EmbedFonts { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Create a ToPdfParameterList object ToPdfParameterList parameters = new ToPdfParameterList(); // Embed all the fonts used in Word in the generated PDF parameters.IsEmbeddedAllFonts = true; // Save the document to PDF doc.SaveToFile("EmbedFonts.pdf", FileFormat.PDF); // Dispose resources doc.Dispose(); } } }
Create Bookmarks while Converting Word to PDF in C#
Including bookmarks in a PDF document while converting from Microsoft Word can greatly enhance the navigation and readability of the resulting PDF, especially for long or complex documents.
When utilizing Spire.Doc to convert a Word document to PDF, you have the option to automatically generate bookmarks based on existing bookmarks or headings. You can accomplish this by enabling either the CreateWordsBookmarks property or the CreateWordBookmarksUsingHeadings property of the ToPdfParameterList object.
The steps to create bookmark while converting Word to PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create a ToPdfParameterList object, which is used to set the conversion options.
- Generate bookmarks in PDF based on the existing bookmarks of the Word document by settings CreateWordsBookmarks property to true.
- Save the Word document to PDF using Doucment.SaveToFile(string fileName, ToPdfParameterList paramList) method.
- C#
using Spire.Doc; namespace CreateBookmarkWhenConverting { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Create a ToPdfParameterList object ToPdfParameterList parameters = new ToPdfParameterList(); // Create bookmarks in PDF from existing bookmarks in Word parameters.CreateWordBookmarks = true; // Create bookmarks from Word headings // parameters.CreateWordBookmarksUsingHeadings= true; // Save the document to PDF doc.SaveToFile("CreateBookmarks.pdf", parameters); // Dispose resources doc.Dispose(); } } }
Disable Hyperlinks while Converting Word to PDF in C#
While converting a Word document to PDF, there are instances where it may be necessary to deactivate hyperlinks. This can be done to prevent accidental clicks or to maintain a static view of the document without any navigation away from its pages.
To disable hyperlinks during the conversion process, simply set the DisableLink property of the ToPdfParameterList object to true. By doing so, the resulting PDF will not contain any active hyperlinks.
The steps to disable hyperlinks while converting Word to PDF in C# are as follows.
- Create a Document object.
- Load a sample Word document from a given file path.
- Create a ToPdfParameterList object, which is used to set the conversion options.
- Disable hyperlinks by settings DisableLink property to true.
- Save the Word document to PDF using Doucment.SaveToFile(string fileName, ToPdfParameterList paramList) method.
- C#
using Spire.Doc; namespace DisableHyperlinks { class Program { static void Main(string[] args) { // Create a Document object Document doc = new Document(); // Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.docx"); // Create a ToPdfParameterList object ToPdfParameterList parameters = new ToPdfParameterList(); // Disable hyperlinks parameters.DisableLink = true; // Save the document to PDF doc.SaveToFile("DisableHyperlinks.pdf", parameters); // Dispose resources doc.Dispose(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Word header and footer presents additional information of Word document, which can be text, image or page number. This guide focuses on introducing how to insert image header and footer for Word document in C# and VB.NET.
Header/Footer plays an important role in Word document, which uses text, image or page number to demonstrate some additional information about this document. The information can be company name, logo, author name, document title etc. This guide will demonstrate detailed process to insert image header/footer in Word with C# and VB.NET via Spire.Doc for .NET. The following screenshot displays Word image header/footer result after programming.
Spire.Doc for .NET provides a HeaderFooter. class to enable developers to generate a new header or footer. Firstly, initialize a header instance of HeaderFooter class and then invoke AddParagraph() method to add a paragraph body for this header/footer instance. Next, invoke Paragraph.AppendPicture(Image image) method to append a picture for header/footer paragraph. If you want to add text for paragraph as well, please invoke Paragraph.AppendText(string text) method. Also, you can set format for header/footer paragraph, appended image and text to have a better layout. Code as following:
using System.Drawing; using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; namespace ImageHeaderFooter { class Program { static void Main(string[] args) { //Load Document Document document = new Document(); document.LoadFromFile(@"E:\Work\Documents\Spire.Doc for .NET.docx"); //Initialize a Header Instance HeaderFooter header = document.Sections[0].HeadersFooters.Header; //Add Header Paragraph and Format Paragraph paragraph = header.AddParagraph(); paragraph.Format.HorizontalAlignment = HorizontalAlignment.Right; //Append Picture for Header Paragraph and Format DocPicture headerimage = paragraph.AppendPicture(Image.FromFile(@"E:\Logo\doclog.png")); headerimage.VerticalAlignment = ShapeVerticalAlignment.Bottom; //Initialize a Footer Instance HeaderFooter footer = document.Sections[0].HeadersFooters.Footer; //Add Footer Paragraph and Format Paragraph paragraph2 = footer.AddParagraph(); paragraph2.Format.HorizontalAlignment = HorizontalAlignment.Left; //Append Picture and Text for Footer Paragraph DocPicture footerimage = paragraph2.AppendPicture(Image.FromFile(@"E:\Logo\logo.jpeg")); TextRange TR = paragraph2.AppendText("Copyright © 2013 e-iceblue. All Rights Reserved."); TR.CharacterFormat.FontName = "Arial"; TR.CharacterFormat.FontSize = 10; TR.CharacterFormat.TextColor = Color.Black; //Save and Launch document.SaveToFile("ImageHeaderFooter.docx", FileFormat.Docx); System.Diagnostics.Process.Start("ImageHeaderFooter.docx"); } } }
Imports System.Drawing Imports Spire.Doc Imports Spire.Doc.Documents Imports Spire.Doc.Fields Namespace ImageHeaderFooter Friend Class Program Shared Sub Main(ByVal args() As String) 'Load Document Dim document As New Document() document.LoadFromFile("E:\Work\Documents\Spire.Doc for .NET.docx") 'Initialize a Header Instance Dim header As HeaderFooter = document.Sections(0).HeadersFooters.Header 'Add Header Paragraph and Format Dim paragraph As Paragraph = header.AddParagraph() paragraph.Format.HorizontalAlignment = HorizontalAlignment.Right 'Append Picture for Header Paragraph and Format Dim headerimage As DocPicture = paragraph.AppendPicture(Image.FromFile("E:\Logo\doclog.png")) headerimage.VerticalAlignment = ShapeVerticalAlignment.Bottom 'Initialize a Footer Instance Dim footer As HeaderFooter = document.Sections(0).HeadersFooters.Footer 'Add Footer Paragraph and Format Dim paragraph2 As Paragraph = footer.AddParagraph() paragraph2.Format.HorizontalAlignment = HorizontalAlignment.Left 'Append Picture and Text for Footer Paragraph Dim footerimage As DocPicture = paragraph2.AppendPicture(Image.FromFile("E:\Logo\logo.jpeg")) Dim TR As TextRange = paragraph2.AppendText("Copyright © 2013 e-iceblue. All Rights Reserved.") TR.CharacterFormat.FontName = "Arial" TR.CharacterFormat.FontSize = 10 TR.CharacterFormat.TextColor = Color.Black 'Save and Launch document.SaveToFile("ImageHeaderFooter.docx", FileFormat.Docx) System.Diagnostics.Process.Start("ImageHeaderFooter.docx") End Sub End Class End Namespace
Spire.Doc, an easy-to-use component to perform Word tasks, allows developers to fast generate, write, edit and save Word (Word 97-2003, Word 2007, Word 2010) in C# and VB.NET for .NET, Silverlight and WPF.
Text alignment is a paragraph formatting attribute that determines the appearance of the text in a whole paragraph. There are four types of text alignments available in Microsoft Word: left-aligned, center-aligned, right-aligned, and justified. In this article, you will learn how to programmatically set different text alignments for paragraphs in a Word document using Spire.Doc for .NET.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Align Text in Word
The detailed steps are as follows:
- Create a Document instance.
- Load a sample Word document using Document.LoadFromFile() method.
- Get a specified section using Document.Sections[] property.
- Get a specified paragraph using Section.Paragraphs[] property.
- Get the paragraph format using Paragraph.Format property
- Set text alignment for the specified paragraph using ParagraphFormat.HorizontalAlignment property.
- Save the document to another file using Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; namespace AlignText { class Program { static void Main(string[] args) { //Create a Document instance Document doc = new Document(); //Load a sample Word document doc.LoadFromFile(@"D:\Files\sample.docx"); //Get the first section Section section = doc.Sections[0]; //Get the first paragraph and make it center-aligned Paragraph p = section.Paragraphs[0]; p.Format.HorizontalAlignment = HorizontalAlignment.Center; //Get the second paragraph and make it left-aligned Paragraph p1 = section.Paragraphs[1]; p1.Format.HorizontalAlignment = HorizontalAlignment.Left; //Get the third paragraph and make it right-aligned Paragraph p2 = section.Paragraphs[2]; p2.Format.HorizontalAlignment = HorizontalAlignment.Right; //Get the fourth paragraph and make it justified Paragraph p3 = section.Paragraphs[3]; p3.Format.HorizontalAlignment = HorizontalAlignment.Justify; //Save the document doc.SaveToFile("WordAlignment.docx", FileFormat.Docx); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Word Font setting allows users to change text font style and size in a document. With wonderful font settings, the document layout and appearance will be more appealed. What’s more, in order to make some special words, phrases or paragraphs more obvious, users can set different font styles or size. For example, the title is often set as different font style with bigger size from body.
Spire.Doc for .NET, a professional .NET Word component, enables users to set font in Word document. This guide will shows how to set by using C#, VB.NET via Spire.Doc for .NET and the following screenshot presents result after setting.
- Load the document and get the paragraph which you want to set font.
- Then, declare a new paragraph style and set FontName and FontSize properties of this style.
- Finally, apply the style for paragraph and save document. Code as following:
using Spire.Doc; using Spire.Doc.Documents; namespace WordImage { class ImageinWord { static void Main(string[] args) { //Load Document Document document = new Document(); document.LoadFromFile(@"E:\Work\Documents\WordDocuments\Humor Them.docx"); //Get Paragraph Section s = document.Sections[0]; Paragraph p = s.Paragraphs[1]; //Set Font Style and Size ParagraphStyle style = new ParagraphStyle(document); style.Name = "FontStyle"; style.CharacterFormat.FontName = "Century Gothic"; style.CharacterFormat.FontSize = 20; document.Styles.Add(style); p.ApplyStyle(style.Name); //Save and Launch document.SaveToFile("font.docx", FileFormat.Docx2010); System.Diagnostics.Process.Start("font.docx"); } } }
Imports Spire.Doc Imports Spire.Doc.Documents Namespace WordImage Friend Class ImageinWord Shared Sub Main(ByVal args() As String) 'Load Document Dim document As New Document() document.LoadFromFile("E:\Work\Documents\WordDocuments\Humor Them.docx") 'Get Paragraph Dim s As Section = document.Sections(0) Dim p As Paragraph = s.Paragraphs(1) 'Set Font Style and Size Dim style As New ParagraphStyle(document) style.Name = "FontStyle" style.CharacterFormat.FontName = "Century Gothic" style.CharacterFormat.FontSize = 20 document.Styles.Add(style) p.ApplyStyle(style.Name) 'Save and Launch document.SaveToFile("font.docx", FileFormat.Docx2010) System.Diagnostics.Process.Start("font.docx") End Sub End Class End Namespace
Spire.Doc, a professional Word component, can be used to generate, load, write, edit and save Word documents for .NET, Silverlight and WPF.
