Tuesday, 05 April 2011 09:07
PDF Font in C#, VB.NET
using System; using System.Drawing; using Spire.Pdf; using Spire.Pdf.Graphics; namespace Font { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); // Create one page PdfPageBase page = doc.Pages.Add(); //Draw the text float l = page.Canvas.ClientSize.Width / 2; PointF center = new PointF(l, l); float r = (float)Math.Sqrt(2 * l * l); PdfRadialGradientBrush brush = new PdfRadialGradientBrush(center, 0f, center, r, Color.Blue, Color.Red); PdfFontFamily[] fontFamilies = (PdfFontFamily[])Enum.GetValues(typeof(PdfFontFamily)); float y = 10; for (int i = 0; i < fontFamilies.Length; i++) { String text = String.Format("Font Family: {0}", fontFamilies[i]); float x1 = 0; y = 10 + i * 16; PdfFont font1 = new PdfFont(PdfFontFamily.Courier, 14f); PdfFont font2 = new PdfFont(fontFamilies[i], 14f); float x2 = x1 + 10 + font1.MeasureString(text).Width; page.Canvas.DrawString(text, font1, brush, x1, y); page.Canvas.DrawString(text, font2, brush, x2, y); } //true type font - embedded. System.Drawing.Font font = new System.Drawing.Font("Arial", 14f, FontStyle.Bold); PdfTrueTypeFont trueTypeFont = new PdfTrueTypeFont(font); page.Canvas.DrawString("Font Family: Arial - Embedded", trueTypeFont, brush, 0, (y = y + 16f)); //right to left String arabicText = "\u0627\u0644\u0630\u0647\u0627\u0628\u0021\u0020" + "\u0628\u062F\u0648\u0631\u0647\u0020\u062D\u0648\u0644\u0647\u0627\u0021\u0020" + "\u0627\u0644\u0630\u0647\u0627\u0628\u0021\u0020" + "\u0627\u0644\u0630\u0647\u0627\u0628\u0021\u0020" + "\u0627\u0644\u0630\u0647\u0627\u0628\u0021"; trueTypeFont = new PdfTrueTypeFont(font, true); RectangleF rctg = new RectangleF(new PointF(0, (y = y + 16f)), page.Canvas.ClientSize); PdfStringFormat format = new PdfStringFormat(PdfTextAlignment.Right); format.RightToLeft = true; page.Canvas.DrawString(arabicText, trueTypeFont, brush, rctg, format); //true type font - not embedded font = new System.Drawing.Font("Batang", 14f, FontStyle.Italic); trueTypeFont = new PdfTrueTypeFont(font); page.Canvas.DrawString("Font Family: Batang - Not Embedded", trueTypeFont, brush, 0, (y = y + 16f)); //font file String fontFileName = "Hawaii_Killer.ttf"; trueTypeFont = new PdfTrueTypeFont(fontFileName, 20f); page.Canvas.DrawString("Hawaii Killer Font", trueTypeFont, brush, 0, (y = y + 16f)); page.Canvas.DrawString("Hawaii Killer Font, from http://www.1001freefonts.com", new PdfFont(PdfFontFamily.Helvetica, 8f), brush, 10, (y = y + 20f)); //cjk font PdfCjkStandardFont cjkFont = new PdfCjkStandardFont(PdfCjkFontFamily.MonotypeHeiMedium, 14f); page.Canvas.DrawString("How to say 'Font' in Chinese? \u5B57\u4F53", cjkFont, brush, 0, (y = y + 16f)); cjkFont = new PdfCjkStandardFont(PdfCjkFontFamily.HanyangSystemsGothicMedium, 14f); page.Canvas.DrawString("How to say 'Font' in Japanese? \u30D5\u30A9\u30F3\u30C8", cjkFont, brush, 0, (y = y + 16f)); cjkFont = new PdfCjkStandardFont(PdfCjkFontFamily.HanyangSystemsShinMyeongJoMedium, 14f); page.Canvas.DrawString("How to say 'Font' in Korean? \uAE00\uAF34", cjkFont, brush, 0, (y = y + 16f)); //Save pdf file. doc.SaveToFile("Font.pdf"); doc.Close(); //Launching the Pdf file. System.Diagnostics.Process.Start("Font.pdf"); } } }
Imports System.Drawing Imports Spire.Pdf Imports Spire.Pdf.Graphics Namespace FontFormat Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() ' Create one page Dim page As PdfPageBase = doc.Pages.Add() 'Draw the text Dim l As Single = page.Canvas.ClientSize.Width / 2 Dim center As New PointF(l, l) Dim r As Single = CSng(Math.Sqrt(2 * l * l)) Dim brush As New PdfRadialGradientBrush(center, 0.0F, center, r, Color.Blue, Color.Red) Dim fontFamilies() As PdfFontFamily _ = CType(System.Enum.GetValues(GetType(PdfFontFamily)), PdfFontFamily()) Dim y As Single = 10 For i As Integer = 0 To fontFamilies.Length - 1 Dim text As String = String.Format("Font Family: {0}", fontFamilies(i)) Dim x1 As Single = 0 y = 10 + i * 16 Dim font1 As New PdfFont(PdfFontFamily.Courier, 14.0F) Dim font2 As New PdfFont(fontFamilies(i), 14.0F) Dim x2 As Single = x1 + 10 + font1.MeasureString(text).Width page.Canvas.DrawString(text, font1, brush, x1, y) page.Canvas.DrawString(text, font2, brush, x2, y) Next i 'true type font - embedded. Dim font As New System.Drawing.Font("Arial", 14.0F, FontStyle.Bold) Dim trueTypeFont As New PdfTrueTypeFont(font) y = y + 16.0F page.Canvas.DrawString("Font Family: Arial - Embedded", trueTypeFont, brush, 0, y) 'right to left Dim arabicText As String _ = ChrW(&H627).ToString() & ChrW(&H644).ToString() & ChrW(&H630).ToString() _ & ChrW(&H647).ToString() & ChrW(&H627).ToString() & ChrW(&H628).ToString() _ & ChrW(&H21).ToString() & ChrW(&H20).ToString() & ChrW(&H628).ToString() _ & ChrW(&H62F).ToString() & ChrW(&H648).ToString() & ChrW(&H631).ToString() _ & ChrW(&H647).ToString() & ChrW(&H20).ToString() & ChrW(&H62D).ToString() _ & ChrW(&H648).ToString() & ChrW(&H644).ToString() & ChrW(&H647).ToString() _ & ChrW(&H627).ToString() & ChrW(&H21).ToString() & ChrW(&H20).ToString() _ & ChrW(&H627).ToString() & ChrW(&H644).ToString() & ChrW(&H630).ToString() _ & ChrW(&H647).ToString() & ChrW(&H627).ToString() & ChrW(&H628).ToString() _ & ChrW(&H21).ToString() & ChrW(&H20).ToString() & ChrW(&H627).ToString() _ & ChrW(&H644).ToString() & ChrW(&H630).ToString() & ChrW(&H647).ToString() _ & ChrW(&H627).ToString() & ChrW(&H628).ToString() & ChrW(&H21).ToString() _ & ChrW(&H20).ToString() & ChrW(&H627).ToString() & ChrW(&H644).ToString() _ & ChrW(&H630).ToString() & ChrW(&H647).ToString() & ChrW(&H627).ToString() _ & ChrW(&H628).ToString() & ChrW(&H21).ToString() trueTypeFont = New PdfTrueTypeFont(font, True) y = y + 16.0F Dim rctg As New RectangleF(New PointF(0, y), page.Canvas.ClientSize) Dim format As New PdfStringFormat(PdfTextAlignment.Right) format.RightToLeft = True page.Canvas.DrawString(arabicText, trueTypeFont, brush, rctg, format) 'true type font - not embedded font = New System.Drawing.Font("Batang", 14.0F, FontStyle.Italic) trueTypeFont = New PdfTrueTypeFont(font) y = y + 16.0F page.Canvas.DrawString("Font Family: Batang - Not Embedded", trueTypeFont, brush, 0, y) 'font file Dim fontFileName As String = "Hawaii_Killer.ttf" trueTypeFont = New PdfTrueTypeFont(fontFileName, 20.0F) y = y + 16.0F page.Canvas.DrawString("Hawaii Killer Font", trueTypeFont, brush, 0, y) y = y + 20.0F page.Canvas.DrawString("Hawaii Killer Font, from http://www.1001freefonts.com", _ New PdfFont(PdfFontFamily.Helvetica, 8.0F), brush, 10, y) 'cjk font Dim cjkFont As New PdfCjkStandardFont(PdfCjkFontFamily.MonotypeHeiMedium, 14.0F) y = y + 16.0F page.Canvas.DrawString("How to say 'Font' in Chinese? " _ & ChrW(&H5B57).ToString() & ChrW(&H4F53).ToString(), cjkFont, brush, 0, y) cjkFont = New PdfCjkStandardFont(PdfCjkFontFamily.HanyangSystemsGothicMedium, 14.0F) y = y + 16.0F page.Canvas.DrawString("How to say 'Font' in Japanese? " _ & ChrW(&H30D5).ToString() & ChrW(&H30A9).ToString() _ & ChrW(&H30F3).ToString() & ChrW(&H30C8).ToString(), cjkFont, brush, 0, y) cjkFont = New PdfCjkStandardFont(PdfCjkFontFamily.HanyangSystemsShinMyeongJoMedium, 14.0F) y = y + 16.0F page.Canvas.DrawString("How to say 'Font' in Korean? " _ & ChrW(&HAE00).ToString() & ChrW(&HAF34).ToString(), cjkFont, brush, 0, y) 'Save pdf file. doc.SaveToFile("Font.pdf") doc.Close() 'Launching the Pdf file. Process.Start("Font.pdf") End Sub End Class End Namespace
Published in
Formating
Friday, 02 July 2010 23:37
EXCEL Font Styles in C#, VB.NET
The sample demonstrates how to set font formatting in an excel workbook.
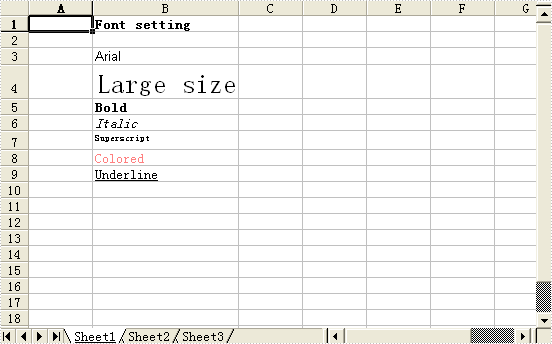
private void ExcelDocViewer( string fileName ) { try { System.Diagnostics.Process.Start(fileName); } catch{} } private void btnRun_Click(object sender, System.EventArgs e) { Workbook workbook = new Workbook(); Worksheet sheet = workbook.Worksheets[0]; sheet.Range["B1"].Text = "Font setting"; sheet.Range["B1"].Style.Font.IsBold = true; sheet.Range["B3"].Text = "Arial"; sheet.Range["B3"].Style.Font.FontName = "Arial"; sheet.Range["B4"].Text = "Large size"; sheet.Range["B4"].Style.Font.Size = 20; sheet.Range["B5"].Text = "Bold"; sheet.Range["B5"].Style.Font.IsBold = true; sheet.Range["B6"].Text = "Italic"; sheet.Range["B6"].Style.Font.IsItalic = true; sheet.Range["B7"].Text = "Superscript"; sheet.Range["B7"].Style.Font.IsSuperscript = true; sheet.Range["B8"].Text = "Colored"; sheet.Range["B8"].Style.Font.Color = Color.FromArgb(255,125,125); sheet.Range["B9"].Text = "Underline"; sheet.Range["B9"].Style.Font.Underline = FontUnderlineType.Single; sheet.AutoFitColumn(2); workbook.SaveToFile("Sample.xls"); ExcelDocViewer(workbook.FileName); }
Private Sub ExcelDocViewer(ByVal fileName As String) Try System.Diagnostics.Process.Start(fileName) Catch End Try End Sub Private Sub btnRun_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnRun.Click Dim workbook As Workbook = New Workbook() Dim sheet As Worksheet = workbook.Worksheets(0) sheet.Range("B1").Text = "Font setting" sheet.Range("B1").Style.Font.IsBold = True sheet.Range("B3").Text = "Arial" sheet.Range("B3").Style.Font.FontName = "Arial" sheet.Range("B4").Text = "Large size" sheet.Range("B4").Style.Font.Size = 20 sheet.Range("B5").Text = "Bold" sheet.Range("B5").Style.Font.IsBold = True sheet.Range("B6").Text = "Italic" sheet.Range("B6").Style.Font.IsItalic = True sheet.Range("B7").Text = "Superscript" sheet.Range("B7").Style.Font.IsSuperscript = True sheet.Range("B8").Text = "Colored" sheet.Range("B8").Style.Font.Color = Color.FromArgb(255,125,125) sheet.Range("B9").Text = "Underline" sheet.Range("B9").Style.Font.Underline = FontUnderlineType.Single sheet.AutoFitColumn(2) workbook.SaveToFile("Sample.xls") ExcelDocViewer(workbook.FileName) End Sub
Published in
Styles