Wednesday, 06 April 2011 09:47
PDF Extract in C#, VB.NET
The sample demonstrates how to extract images and text from PDF document.
(NO screenshot)
using System; using System.Collections.Generic; using System.Drawing; using System.Drawing.Imaging; using System.IO; using System.Text; using Spire.Pdf; namespace Extraction { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); doc.LoadFromFile(@"Sample2.pdf"); StringBuilder buffer = new StringBuilder(); IList<Image> images = new List<Image>(); foreach (PdfPageBase page in doc.Pages) { buffer.Append(page.ExtractText()); foreach (Image image in page.ExtractImages()) { images.Add(image); } } doc.Close(); //save text String fileName = "TextInPdf.txt"; File.WriteAllText(fileName, buffer.ToString()); //save image int index = 0; foreach (Image image in images) { String imageFileName = String.Format("Image-{0}.png", index++); image.Save(imageFileName, ImageFormat.Png); } //Launching the Text file. System.Diagnostics.Process.Start(fileName); } } }
Imports System.Collections.Generic Imports System.Drawing Imports System.Drawing.Imaging Imports System.IO Imports System.Text Imports Spire.Pdf Namespace Extraction Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() doc.LoadFromFile("Sample2.pdf") Dim buffer As New StringBuilder() Dim images As IList(Of Image) = New List(Of Image)() For Each page As PdfPageBase In doc.Pages buffer.Append(page.ExtractText()) For Each image As Image In page.ExtractImages() images.Add(image) Next image Next page doc.Close() 'save text Dim fileName As String = "TextInPdf.txt" File.WriteAllText(fileName, buffer.ToString()) 'save image Dim index As Integer = 0 For Each image As Image In images Dim imageFileName As String = String.Format("Image-{0}.png", index) index += 1 image.Save(imageFileName, ImageFormat.Png) Next image 'Launching the Text file. Process.Start(fileName) End Sub End Class End Namespace
Published in
Document Operation
Wednesday, 06 April 2011 05:52
PDF TextWaterMark in C#, VB.NET
using System; using System.Drawing; using Spire.Pdf; using Spire.Pdf.Graphics; using Spire.Pdf.Tables; namespace TextWaterMark { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); //margin PdfUnitConvertor unitCvtr = new PdfUnitConvertor(); PdfMargins margin = new PdfMargins(); margin.Top = unitCvtr.ConvertUnits(2.54f, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point); margin.Bottom = margin.Top; margin.Left = unitCvtr.ConvertUnits(3.17f, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point); margin.Right = margin.Left; // Create one page PdfPageBase page = doc.Pages.Add(PdfPageSize.A4, margin); PdfTilingBrush brush = new PdfTilingBrush(new SizeF(page.Canvas.ClientSize.Width / 2, page.Canvas.ClientSize.Height / 3)); brush.Graphics.SetTransparency(0.3f); brush.Graphics.Save(); brush.Graphics.TranslateTransform(brush.Size.Width / 2, brush.Size.Height / 2); brush.Graphics.RotateTransform(-45); brush.Graphics.DrawString("Spire.Pdf Demo", new PdfFont(PdfFontFamily.Helvetica, 24), PdfBrushes.Violet, 0, 0, new PdfStringFormat(PdfTextAlignment.Center)); brush.Graphics.Restore(); brush.Graphics.SetTransparency(1); page.Canvas.DrawRectangle(brush, new RectangleF(new PointF(0, 0), page.Canvas.ClientSize)); //Draw the page DrawPage(page); //Save pdf file. doc.SaveToFile("TextWaterMark.pdf"); doc.Close(); //Launching the Pdf file. System.Diagnostics.Process.Start("TextWaterMark.pdf"); } private static void DrawPage(PdfPageBase page) { float y = 10; //title PdfBrush brush1 = PdfBrushes.Black; PdfTrueTypeFont font1 = new PdfTrueTypeFont(new Font("Arial", 16f, FontStyle.Bold)); PdfStringFormat format1 = new PdfStringFormat(PdfTextAlignment.Center); page.Canvas.DrawString("Category Sales by Year", font1, brush1, page.Canvas.ClientSize.Width / 2, y, format1); y = y + font1.MeasureString("Category Sales by Year", format1).Height; y = y + 5; String[][] data = { new String[]{"Category Name", "1994 Sale Amount", "1995 Sale Amount", "1996 Sale Amount"}, new String[]{"Beverages", "38,487.20", "102,479.46", "126,901.53"}, new String[]{"Condiments", "16,402.95", "51,041.83", "38,602.31"}, new String[]{"Confections", "23,812.90", "79,752.25", "63,792.07"}, new String[]{"Dairy Products", "30,027.79", "116,495.45", "87,984.05"}, new String[]{"Grains/Cereals", "7,313.92", "53,823.48", "34,607.19"}, new String[]{"Meat/Poultry", "19,856.86", "77,164.75", "66,000.75"}, new String[]{"Produce", "10,694.96", "45,973.69", "43,315.93"}, new String[]{"Seafood", "16,247.77", "64,195.51", "50,818.46"} }; PdfTable table = new PdfTable(); table.Style.CellPadding = 2; table.Style.BorderPen = new PdfPen(brush1, 0.75f); table.Style.DefaultStyle.BackgroundBrush = PdfBrushes.SkyBlue; table.Style.DefaultStyle.Font = new PdfTrueTypeFont(new Font("Arial", 10f)); table.Style.HeaderSource = PdfHeaderSource.Rows; table.Style.HeaderRowCount = 1; table.Style.HeaderStyle.BackgroundBrush = PdfBrushes.CadetBlue; table.Style.HeaderStyle.Font = new PdfTrueTypeFont(new Font("Arial", 11f, FontStyle.Bold)); table.Style.HeaderStyle.StringFormat = new PdfStringFormat(PdfTextAlignment.Center); table.Style.ShowHeader = true; table.DataSource = data; PdfLayoutResult result = table.Draw(page, new PointF(0, y)); y = y + result.Bounds.Height + 5; PdfBrush brush2 = PdfBrushes.LightGray; PdfTrueTypeFont font2 = new PdfTrueTypeFont(new Font("Arial", 9f)); page.Canvas.DrawString("* All data from NorthWind", font2, brush2, 5, y); } } }
Imports System.Drawing Imports Spire.Pdf Imports Spire.Pdf.Graphics Imports Spire.Pdf.Tables Namespace TextWaterMark Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() 'margin Dim unitCvtr As New PdfUnitConvertor() Dim margin As New PdfMargins() margin.Top = unitCvtr.ConvertUnits(2.54F, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point) margin.Bottom = margin.Top margin.Left = unitCvtr.ConvertUnits(3.17F, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point) margin.Right = margin.Left ' Create one page Dim page As PdfPageBase = doc.Pages.Add(PdfPageSize.A4, margin) Dim brush As New PdfTilingBrush(New SizeF(page.Canvas.ClientSize.Width \ 2, page.Canvas.ClientSize.Height \ 3)) brush.Graphics.SetTransparency(0.3F) brush.Graphics.Save() brush.Graphics.TranslateTransform(brush.Size.Width \ 2, brush.Size.Height \ 2) brush.Graphics.RotateTransform(-45) brush.Graphics.DrawString("Spire.Pdf Demo", New PdfFont(PdfFontFamily.Helvetica, 24), _ PdfBrushes.Violet, 0, 0, New PdfStringFormat(PdfTextAlignment.Center)) brush.Graphics.Restore() brush.Graphics.SetTransparency(1) page.Canvas.DrawRectangle(brush, New RectangleF(New PointF(0, 0), page.Canvas.ClientSize)) 'Draw the page DrawPage(page) 'Save pdf file. doc.SaveToFile("TextWaterMark.pdf") doc.Close() 'Launching the Pdf file. Process.Start("TextWaterMark.pdf") End Sub Private Shared Sub DrawPage(ByVal page As PdfPageBase) Dim y As Single = 10 'title Dim brush1 As PdfBrush = PdfBrushes.Black Dim font1 As New PdfTrueTypeFont(New Font("Arial", 16.0F, FontStyle.Bold)) Dim format1 As New PdfStringFormat(PdfTextAlignment.Center) page.Canvas.DrawString("Category Sales by Year", font1, brush1, _ page.Canvas.ClientSize.Width \ 2, y, format1) y = y + font1.MeasureString("Category Sales by Year", format1).Height y = y + 5 Dim data()() As String _ = {New String() {"Category Name", "1994 Sale Amount", "1995 Sale Amount", "1996 Sale Amount"}, _ New String() {"Beverages", "38,487.20", "102,479.46", "126,901.53"}, _ New String() {"Condiments", "16,402.95", "51,041.83", "38,602.31"}, _ New String() {"Confections", "23,812.90", "79,752.25", "63,792.07"}, _ New String() {"Dairy Products", "30,027.79", "116,495.45", "87,984.05"}, _ New String() {"Grains/Cereals", "7,313.92", "53,823.48", "34,607.19"}, _ New String() {"Meat/Poultry", "19,856.86", "77,164.75", "66,000.75"}, _ New String() {"Produce", "10,694.96", "45,973.69", "43,315.93"}, _ New String() {"Seafood", "16,247.77", "64,195.51", "50,818.46"}} Dim table As New PdfTable() table.Style.CellPadding = 2 table.Style.BorderPen = New PdfPen(brush1, 0.75F) table.Style.DefaultStyle.BackgroundBrush = PdfBrushes.SkyBlue table.Style.DefaultStyle.Font = New PdfTrueTypeFont(New Font("Arial", 10.0F)) table.Style.HeaderSource = PdfHeaderSource.Rows table.Style.HeaderRowCount = 1 table.Style.HeaderStyle.BackgroundBrush = PdfBrushes.CadetBlue table.Style.HeaderStyle.Font = New PdfTrueTypeFont(New Font("Arial", 11.0F, FontStyle.Bold)) table.Style.HeaderStyle.StringFormat = New PdfStringFormat(PdfTextAlignment.Center) table.Style.ShowHeader = True table.DataSource = data Dim result As PdfLayoutResult = table.Draw(page, New PointF(0, y)) y = y + result.Bounds.Height + 5 Dim brush2 As PdfBrush = PdfBrushes.LightGray Dim font2 As New PdfTrueTypeFont(New Font("Arial", 9.0F)) page.Canvas.DrawString("* All data from NorthWind", font2, brush2, 5, y) End Sub End Class End Namespace
Published in
WaterMark
Tuesday, 05 April 2011 09:25
PDF TextLayout in C#, VB.NET
using System; using System.Drawing; using Spire.Pdf; using Spire.Pdf.Graphics; namespace TextLayout { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); // Create one page PdfPageBase page = doc.Pages.Add(); float pageWidth = page.Canvas.ClientSize.Width; float y = 0; //page header PdfPen pen1 = new PdfPen(Color.LightGray, 1f); PdfBrush brush1 = new PdfSolidBrush(Color.LightGray); PdfTrueTypeFont font1 = new PdfTrueTypeFont(new Font("Arial", 8f, FontStyle.Italic)); PdfStringFormat format1 = new PdfStringFormat(PdfTextAlignment.Right); String text = "Demo of Spire.Pdf"; page.Canvas.DrawString(text, font1, brush1, pageWidth, y, format1); SizeF size = font1.MeasureString(text, format1); y = y + size.Height + 1; page.Canvas.DrawLine(pen1, 0, y, pageWidth, y); //title y = y + 5; PdfBrush brush2 = new PdfSolidBrush(Color.Black); PdfTrueTypeFont font2 = new PdfTrueTypeFont(new Font("Arial", 16f, FontStyle.Bold)); PdfStringFormat format2 = new PdfStringFormat(PdfTextAlignment.Center); format2.CharacterSpacing = 1f; text = "Summary of Science"; page.Canvas.DrawString(text, font2, brush2, pageWidth / 2, y, format2); size = font2.MeasureString(text, format2); y = y + size.Height + 6; //icon PdfImage image = PdfImage.FromFile("Wikipedia_Science.png"); page.Canvas.DrawImage(image, new PointF(pageWidth - image.PhysicalDimension.Width, y)); float imageLeftSpace = pageWidth - image.PhysicalDimension.Width - 2; float imageBottom = image.PhysicalDimension.Height + y; //refenrence content PdfTrueTypeFont font3 = new PdfTrueTypeFont(new Font("Arial", 9f)); PdfStringFormat format3 = new PdfStringFormat(); format3.ParagraphIndent = font3.Size * 2; format3.MeasureTrailingSpaces = true; format3.LineSpacing = font3.Size * 1.5f; String text1 = "(All text and picture from "; String text2 = "Wikipedia"; String text3 = ", the free encyclopedia)"; page.Canvas.DrawString(text1, font3, brush2, 0, y, format3); size = font3.MeasureString(text1, format3); float x1 = size.Width; format3.ParagraphIndent = 0; PdfTrueTypeFont font4 = new PdfTrueTypeFont(new Font("Arial", 9f, FontStyle.Underline)); PdfBrush brush3 = PdfBrushes.Blue; page.Canvas.DrawString(text2, font4, brush3, x1, y, format3); size = font4.MeasureString(text2, format3); x1 = x1 + size.Width; page.Canvas.DrawString(text3, font3, brush2, x1, y, format3); y = y + size.Height; //content PdfStringFormat format4 = new PdfStringFormat(); text = System.IO.File.ReadAllText("Summary_of_Science.txt"); PdfTrueTypeFont font5 = new PdfTrueTypeFont(new Font("Arial", 10f)); format4.LineSpacing = font5.Size * 1.5f; PdfStringLayouter textLayouter = new PdfStringLayouter(); float imageLeftBlockHeight = imageBottom - y; PdfStringLayoutResult result = textLayouter.Layout(text, font5, format4, new SizeF(imageLeftSpace, imageLeftBlockHeight)); if (result.ActualSize.Height < imageBottom - y) { imageLeftBlockHeight = imageLeftBlockHeight + result.LineHeight; result = textLayouter.Layout(text, font5, format4, new SizeF(imageLeftSpace, imageLeftBlockHeight)); } foreach (LineInfo line in result.Lines) { page.Canvas.DrawString(line.Text, font5, brush2, 0, y, format4); y = y + result.LineHeight; } PdfTextWidget textWidget = new PdfTextWidget(result.Remainder, font5, brush2); PdfTextLayout textLayout = new PdfTextLayout(); textLayout.Break = PdfLayoutBreakType.FitPage; textLayout.Layout = PdfLayoutType.Paginate; RectangleF bounds = new RectangleF(new PointF(0, y), page.Canvas.ClientSize); textWidget.StringFormat = format4; textWidget.Draw(page, bounds, textLayout); //Save pdf file. doc.SaveToFile("TextLayout.pdf"); doc.Close(); //Launching the Pdf file. System.Diagnostics.Process.Start("TextLayout.pdf"); } } }
Imports System.Drawing Imports Spire.Pdf Imports Spire.Pdf.Graphics Namespace TextLayout Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() ' Create one page Dim page As PdfPageBase = doc.Pages.Add() Dim pageWidth As Single = page.Canvas.ClientSize.Width Dim y As Single = 0 'page header Dim pen1 As New PdfPen(Color.LightGray, 1.0F) Dim brush1 As PdfBrush = New PdfSolidBrush(Color.LightGray) Dim font1 As New PdfTrueTypeFont(New Font("Arial", 8.0F, FontStyle.Italic)) Dim format1 As New PdfStringFormat(PdfTextAlignment.Right) Dim text As String = "Demo of Spire.Pdf" page.Canvas.DrawString(text, font1, brush1, pageWidth, y, format1) Dim size As SizeF = font1.MeasureString(text, format1) y = y + size.Height + 1 page.Canvas.DrawLine(pen1, 0, y, pageWidth, y) 'title y = y + 5 Dim brush2 As PdfBrush = New PdfSolidBrush(Color.Black) Dim font2 As New PdfTrueTypeFont(New Font("Arial", 16.0F, FontStyle.Bold)) Dim format2 As New PdfStringFormat(PdfTextAlignment.Center) format2.CharacterSpacing = 1.0F text = "Summary of Science" page.Canvas.DrawString(text, font2, brush2, pageWidth / 2, y, format2) size = font2.MeasureString(text, format2) y = y + size.Height + 6 'icon Dim image As PdfImage = PdfImage.FromFile("Wikipedia_Science.png") page.Canvas.DrawImage(image, New PointF(pageWidth - image.PhysicalDimension.Width, y)) Dim imageLeftSpace As Single = pageWidth - image.PhysicalDimension.Width - 2 Dim imageBottom As Single = image.PhysicalDimension.Height + y 'refenrence content Dim font3 As New PdfTrueTypeFont(New Font("Arial", 9.0F)) Dim format3 As New PdfStringFormat() format3.ParagraphIndent = font3.Size * 2 format3.MeasureTrailingSpaces = True format3.LineSpacing = font3.Size * 1.5F Dim text1 As String = "(All text and picture from " Dim text2 As String = "Wikipedia" Dim text3 As String = ", the free encyclopedia)" page.Canvas.DrawString(text1, font3, brush2, 0, y, format3) size = font3.MeasureString(text1, format3) Dim x1 As Single = size.Width format3.ParagraphIndent = 0 Dim font4 As New PdfTrueTypeFont(New Font("Arial", 9.0F, FontStyle.Underline)) Dim brush3 As PdfBrush = PdfBrushes.Blue page.Canvas.DrawString(text2, font4, brush3, x1, y, format3) size = font4.MeasureString(text2, format3) x1 = x1 + size.Width page.Canvas.DrawString(text3, font3, brush2, x1, y, format3) y = y + size.Height 'content Dim format4 As New PdfStringFormat() text = System.IO.File.ReadAllText("Summary_of_Science.txt") Dim font5 As New PdfTrueTypeFont(New Font("Arial", 10.0F)) format4.LineSpacing = font5.Size * 1.5F Dim textLayouter As New PdfStringLayouter() Dim imageLeftBlockHeight As Single = imageBottom - y Dim result As PdfStringLayoutResult _ = textLayouter.Layout(text, font5, format4, New SizeF(imageLeftSpace, imageLeftBlockHeight)) If result.ActualSize.Height < imageBottom - y Then imageLeftBlockHeight = imageLeftBlockHeight + result.LineHeight result = textLayouter.Layout(text, font5, format4, New SizeF(imageLeftSpace, imageLeftBlockHeight)) End If For Each line As LineInfo In result.Lines page.Canvas.DrawString(line.Text, font5, brush2, 0, y, format4) y = y + result.LineHeight Next line Dim textWidget As New PdfTextWidget(result.Remainder, font5, brush2) Dim textLayout As New PdfTextLayout() textLayout.Break = PdfLayoutBreakType.FitPage textLayout.Layout = PdfLayoutType.Paginate Dim bounds As New RectangleF(New PointF(0, y), page.Canvas.ClientSize) textWidget.StringFormat = format4 textWidget.Draw(page, bounds, textLayout) 'Save pdf file. doc.SaveToFile("TextLayout.pdf") doc.Close() 'Launching the Pdf file. Process.Start("TextLayout.pdf") End Sub End Class End Namespace
Published in
Formating
Tuesday, 05 April 2011 07:12
PDF DrawText in C#, VB.NET
using System; using System.Drawing; using Spire.Pdf; using Spire.Pdf.Graphics; namespace DrawText { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); // Create one page PdfPageBase page = doc.Pages.Add(); DrawText(page); AlignText(page); AlignTextInRectangle(page); TransformText(page); RotateText(page); //Save doc file. doc.SaveToFile("DrawText.pdf"); doc.Close(); //Launching the Pdf file. System.Diagnostics.Process.Start("DrawText.pdf"); } private static void RotateText(PdfPageBase page) { //save graphics state PdfGraphicsState state = page.Canvas.Save(); //Draw the text - transform PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 10f); PdfSolidBrush brush = new PdfSolidBrush(Color.Blue); PdfStringFormat centerAlignment = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle); float x = page.Canvas.ClientSize.Width / 2; float y = 380; page.Canvas.TranslateTransform(x, y); for (int i = 0; i < 12; i++) { page.Canvas.RotateTransform(30); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, 20, 0, centerAlignment); } //restor graphics page.Canvas.Restore(state); } private static void TransformText(PdfPageBase page) { //save graphics state PdfGraphicsState state = page.Canvas.Save(); //Draw the text - transform PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 18f); PdfSolidBrush brush1 = new PdfSolidBrush(Color.DeepSkyBlue); PdfSolidBrush brush2 = new PdfSolidBrush(Color.CadetBlue); page.Canvas.TranslateTransform(20, 200); page.Canvas.ScaleTransform(1f, 0.6f); page.Canvas.SkewTransform(-10, 0); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush1, 0, 0); page.Canvas.SkewTransform(10, 0); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush2, 0, 0); page.Canvas.ScaleTransform(1f, -1f); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush2, 0, -2 * 18); //restor graphics page.Canvas.Restore(state); } private static void AlignTextInRectangle(PdfPageBase page) { //Draw the text - align in rectangle PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 10f); PdfSolidBrush brush = new PdfSolidBrush(Color.Blue); RectangleF rctg1 = new RectangleF(0, 70, page.Canvas.ClientSize.Width / 2, 100); RectangleF rctg2 = new RectangleF(page.Canvas.ClientSize.Width / 2, 70, page.Canvas.ClientSize.Width / 2, 100); page.Canvas.DrawRectangle(new PdfSolidBrush(Color.LightBlue), rctg1); page.Canvas.DrawRectangle(new PdfSolidBrush(Color.LightSkyBlue), rctg2); PdfStringFormat leftAlignment = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Top); page.Canvas.DrawString("Left! Left!", font, brush, rctg1, leftAlignment); page.Canvas.DrawString("Left! Left!", font, brush, rctg2, leftAlignment); PdfStringFormat rightAlignment = new PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle); page.Canvas.DrawString("Right! Right!", font, brush, rctg1, rightAlignment); page.Canvas.DrawString("Right! Right!", font, brush, rctg2, rightAlignment); PdfStringFormat centerAlignment = new PdfStringFormat(PdfTextAlignment.Center, PdfVerticalAlignment.Bottom); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, rctg1, centerAlignment); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, rctg2, centerAlignment); } private static void AlignText(PdfPageBase page) { //Draw the text - alignment PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 20f); PdfSolidBrush brush = new PdfSolidBrush(Color.Blue); PdfStringFormat leftAlignment = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle); page.Canvas.DrawString("Left!", font, brush, 0, 20, leftAlignment); page.Canvas.DrawString("Left!", font, brush, 0, 50, leftAlignment); PdfStringFormat rightAlignment = new PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle); page.Canvas.DrawString("Right!", font, brush, page.Canvas.ClientSize.Width, 30, rightAlignment); page.Canvas.DrawString("Right!", font, brush, page.Canvas.ClientSize.Width, 60, rightAlignment); PdfStringFormat centerAlignment = new PdfStringFormat(PdfTextAlignment.Center, PdfVerticalAlignment.Middle); page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, page.Canvas.ClientSize.Width / 2, 40, centerAlignment); } private static void DrawText(PdfPageBase page) { //save graphics state PdfGraphicsState state = page.Canvas.Save(); //Draw text - brush String text = "Go! Turn Around! Go! Go! Go!"; PdfPen pen = PdfPens.DeepSkyBlue; PdfSolidBrush brush = new PdfSolidBrush(Color.White); PdfStringFormat format = new PdfStringFormat(); PdfFont font = new PdfFont(PdfFontFamily.Helvetica, 18f, PdfFontStyle.Italic); SizeF size = font.MeasureString(text, format); RectangleF rctg = new RectangleF(page.Canvas.ClientSize.Width / 2 + 10, 180, size.Width / 3 * 2, size.Height * 2); page.Canvas.DrawString(text, font, pen, brush, rctg, format); //restor graphics page.Canvas.Restore(state); } } }
Imports System.Drawing Imports Spire.Pdf Imports Spire.Pdf.Graphics Namespace DrawText Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() ' Create one page Dim page As PdfPageBase = doc.Pages.Add() DrawText(page) AlignText(page) AlignTextInRectangle(page) TransformText(page) RotateText(page) 'Save doc file. doc.SaveToFile("DrawText.pdf") doc.Close() 'Launching the Pdf file. Process.Start("DrawText.pdf") End Sub Private Shared Sub RotateText(ByVal page As PdfPageBase) 'save graphics state Dim state As PdfGraphicsState = page.Canvas.Save() 'Draw the text - transform Dim font As New PdfFont(PdfFontFamily.Helvetica, 10.0F) Dim brush As New PdfSolidBrush(Color.Blue) Dim centerAlignment As New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle) Dim x As Single = page.Canvas.ClientSize.Width \ 2 Dim y As Single = 380 page.Canvas.TranslateTransform(x, y) For i As Integer = 0 To 11 page.Canvas.RotateTransform(30) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, 20, 0, centerAlignment) Next i 'restor graphics page.Canvas.Restore(state) End Sub Private Shared Sub TransformText(ByVal page As PdfPageBase) 'save graphics state Dim state As PdfGraphicsState = page.Canvas.Save() 'Draw the text - transform Dim font As New PdfFont(PdfFontFamily.Helvetica, 18.0F) Dim brush1 As New PdfSolidBrush(Color.DeepSkyBlue) Dim brush2 As New PdfSolidBrush(Color.CadetBlue) page.Canvas.TranslateTransform(20, 200) page.Canvas.ScaleTransform(1.0F, 0.6F) page.Canvas.SkewTransform(-10, 0) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush1, 0, 0) page.Canvas.SkewTransform(10, 0) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush2, 0, 0) page.Canvas.ScaleTransform(1.0F, -1.0F) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush2, 0, -2 * 18) 'restor graphics page.Canvas.Restore(state) End Sub Private Shared Sub AlignTextInRectangle(ByVal page As PdfPageBase) 'Draw the text - align in rectangle Dim font As New PdfFont(PdfFontFamily.Helvetica, 10.0F) Dim brush As New PdfSolidBrush(Color.Blue) Dim rctg1 As New RectangleF(0, 70, page.Canvas.ClientSize.Width \ 2, 100) Dim rctg2 As New RectangleF(page.Canvas.ClientSize.Width \ 2, 70, page.Canvas.ClientSize.Width \ 2, 100) page.Canvas.DrawRectangle(New PdfSolidBrush(Color.LightBlue), rctg1) page.Canvas.DrawRectangle(New PdfSolidBrush(Color.LightSkyBlue), rctg2) Dim leftAlignment As New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Top) page.Canvas.DrawString("Left! Left!", font, brush, rctg1, leftAlignment) page.Canvas.DrawString("Left! Left!", font, brush, rctg2, leftAlignment) Dim rightAlignment As New PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle) page.Canvas.DrawString("Right! Right!", font, brush, rctg1, rightAlignment) page.Canvas.DrawString("Right! Right!", font, brush, rctg2, rightAlignment) Dim centerAlignment As New PdfStringFormat(PdfTextAlignment.Center, PdfVerticalAlignment.Bottom) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, rctg1, centerAlignment) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", font, brush, rctg2, centerAlignment) End Sub Private Shared Sub AlignText(ByVal page As PdfPageBase) 'Draw the text - alignment Dim font As New PdfFont(PdfFontFamily.Helvetica, 20.0F) Dim brush As New PdfSolidBrush(Color.Blue) Dim leftAlignment As New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle) page.Canvas.DrawString("Left!", font, brush, 0, 20, leftAlignment) page.Canvas.DrawString("Left!", font, brush, 0, 50, leftAlignment) Dim rightAlignment As New PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle) page.Canvas.DrawString("Right!", font, brush, page.Canvas.ClientSize.Width, 30, rightAlignment) page.Canvas.DrawString("Right!", font, brush, page.Canvas.ClientSize.Width, 60, rightAlignment) Dim centerAlignment As New PdfStringFormat(PdfTextAlignment.Center, PdfVerticalAlignment.Middle) page.Canvas.DrawString("Go! Turn Around! Go! Go! Go!", _ font, brush, page.Canvas.ClientSize.Width \ 2, 40, centerAlignment) End Sub Private Shared Sub DrawText(ByVal page As PdfPageBase) 'save graphics state Dim state As PdfGraphicsState = page.Canvas.Save() 'Draw text - brush Dim text As String = "Go! Turn Around! Go! Go! Go!" Dim pen As PdfPen = PdfPens.DeepSkyBlue Dim brush As New PdfSolidBrush(Color.White) Dim format As New PdfStringFormat() Dim font As New PdfFont(PdfFontFamily.Helvetica, 18.0F, PdfFontStyle.Italic) Dim size As SizeF = font.MeasureString(text, format) Dim rctg As New RectangleF(page.Canvas.ClientSize.Width \ 2 + 10, 180, size.Width \ 3 * 2, size.Height * 2) page.Canvas.DrawString(text, font, pen, brush, rctg, format) 'restor graphics page.Canvas.Restore(state) End Sub End Class End Namespace
Published in
Drawing
Sunday, 01 August 2010 18:57
Data Export Text/SYLK/CSV/DIF in C#, VB.NET
This sample demonstrates how to export data table to text/sylk/csv/dif file.
The picture above represents using Excel application to open a SYLK type output file.
private void btnText_Click(object sender, EventArgs e) { System.Data.OleDb.OleDbConnection oleDbConnection1 = new System.Data.OleDb.OleDbConnection(); oleDbConnection1.ConnectionString = @"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=..\..\..\..\Database\demo.mdb"; System.Data.OleDb.OleDbCommand oleDbCommand1 = new System.Data.OleDb.OleDbCommand(); oleDbCommand1.CommandText = "select * from parts"; oleDbCommand1.Connection = oleDbConnection1; Spire.DataExport.TXT.TXTExport txtExport1 = new Spire.DataExport.TXT.TXTExport(); txtExport1.ActionAfterExport = Spire.DataExport.Common.ActionType.OpenView; txtExport1.DataFormats.CultureName = "zh-CN"; txtExport1.DataFormats.Currency = "c"; txtExport1.DataFormats.DateTime = "yyyy-M-d H:mm"; txtExport1.DataFormats.Float = "g"; txtExport1.DataFormats.Integer = "g"; txtExport1.DataFormats.Time = "H:mm"; txtExport1.DataEncoding = Spire.DataExport.Common.EncodingType.ASCII; txtExport1.SQLCommand = oleDbCommand1; oleDbConnection1.Open(); txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.SYLK; txtExport1.FileName = "sample.slk"; txtExport1.SaveToFile(); txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.CSV; txtExport1.FileName = "sample.csv"; txtExport1.SaveToFile(); txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.DIF; txtExport1.FileName = "sample.dif"; txtExport1.SaveToFile(); txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.TXT; txtExport1.FileName = "sample.txt"; txtExport1.SaveToFile(); }
Private Sub btnText_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnText.Click Dim oleDbConnection1 As New System.Data.OleDb.OleDbConnection() oleDbConnection1.ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=..\..\..\..\Database\demo.mdb" Dim oleDbCommand1 As New System.Data.OleDb.OleDbCommand() oleDbCommand1.CommandText = "select * from parts" oleDbCommand1.Connection = oleDbConnection1 Dim txtExport1 As New Spire.DataExport.TXT.TXTExport() txtExport1.ActionAfterExport = Spire.DataExport.Common.ActionType.OpenView txtExport1.DataFormats.CultureName = "zh-CN" txtExport1.DataFormats.Currency = "c" txtExport1.DataFormats.DateTime = "yyyy-M-d H:mm" txtExport1.DataFormats.Float = "g" txtExport1.DataFormats.[Integer] = "g" txtExport1.DataFormats.Time = "H:mm" txtExport1.DataEncoding = Spire.DataExport.Common.EncodingType.ASCII txtExport1.SQLCommand = oleDbCommand1 oleDbConnection1.Open() txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.SYLK txtExport1.FileName = "sample.slk" txtExport1.SaveToFile() txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.CSV txtExport1.FileName = "sample.csv" txtExport1.SaveToFile() txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.DIF txtExport1.FileName = "sample.dif" txtExport1.SaveToFile() txtExport1.ExportType = Spire.DataExport.TXT.TextExportType.TXT txtExport1.FileName = "sample.txt" txtExport1.SaveToFile() End Sub
Published in
Demo
Wednesday, 21 July 2010 08:48
Word Text in C#, VB.NET
The sample demonstrates how to set text style in Word document.
private void button1_Click(object sender, EventArgs e) { //Create word document Document document = new Document(); //Create a new secition Section section = document.AddSection(); //Create a new paragraph Paragraph paragraph = section.AddParagraph(); //Append Text paragraph.AppendText("The various ways to format paragraph text in Microsoft Word:"); paragraph.ApplyStyle(BuiltinStyle.Heading1); //Append alignment text AppendAligmentText(section); //Append indentation text AppendIndentationText(section); AppendBulletedList(section); //Save doc file. document.SaveToFile("Sample.doc",FileFormat.Doc); //Launching the MS Word file. WordDocViewer("Sample.doc"); } private void AppendAligmentText(Section section) { Paragraph paragraph = null; paragraph = section.AddParagraph(); //Append Text paragraph.AppendText("Horizontal Aligenment"); paragraph.ApplyStyle(BuiltinStyle.Heading3); foreach (Spire.Doc.Documents.HorizontalAlignment align in Enum.GetValues(typeof(Spire.Doc.Documents.HorizontalAlignment))) { Paragraph paramgraph = section.AddParagraph(); paramgraph.AppendText("This text is " + align.ToString()); paramgraph.Format.HorizontalAlignment = align; } } private void AppendIndentationText(Section section) { Paragraph paragraph = null; paragraph = section.AddParagraph(); //Append Text paragraph.AppendText("Indentation"); paragraph.ApplyStyle(BuiltinStyle.Heading3); paragraph = section.AddParagraph(); paragraph.AppendText("Indentation is the spacing between text and margins. Word allows you to set left and right margins, as well as indentations for the first line of a paragraph and hanging indents"); paragraph.Format.FirstLineIndent = 15; } private void AppendBulletedList(Section section) { Paragraph paragraph = null; paragraph = section.AddParagraph(); //Append Text paragraph.AppendText("Bulleted List"); paragraph.ApplyStyle(BuiltinStyle.Heading3); paragraph = section.AddParagraph(); for (int i = 0; i < 5; i++) { paragraph = section.AddParagraph(); paragraph.AppendText("Item" + i.ToString()); if (i == 0) { paragraph.ListFormat.ApplyBulletStyle(); } else { paragraph.ListFormat.ContinueListNumbering(); } paragraph.ListFormat.ListLevelNumber = 1; } } private void WordDocViewer(string fileName) { try { System.Diagnostics.Process.Start(fileName); } catch { } }
Private Sub button1_Click(ByVal sender As Object, ByVal e As EventArgs) Handles button1.Click 'Create word document Dim document_Renamed As New Document() 'Create a new secition Dim section_Renamed As Section = document_Renamed.AddSection() 'Create a new paragraph Dim paragraph_Renamed As Paragraph = section_Renamed.AddParagraph() 'Append Text paragraph_Renamed.AppendText("The various ways to format paragraph text in Microsoft Word:") paragraph_Renamed.ApplyStyle(BuiltinStyle.Heading1) 'Append alignment text AppendAligmentText(section_Renamed) 'Append indentation text AppendIndentationText(section_Renamed) AppendBulletedList(section_Renamed) 'Save doc file. document_Renamed.SaveToFile("Sample.doc",FileFormat.Doc) 'Launching the MS Word file. WordDocViewer("Sample.doc") End Sub Private Sub AppendAligmentText(ByVal section_Renamed As Section) Dim paragraph_Renamed As Paragraph = Nothing paragraph_Renamed = section_Renamed.AddParagraph() 'Append Text paragraph_Renamed.AppendText("Horizontal Aligenment") paragraph_Renamed.ApplyStyle(BuiltinStyle.Heading3) For Each align As Spire.Doc.Documents.HorizontalAlignment In System.Enum.GetValues(GetType(Spire.Doc.Documents.HorizontalAlignment)) Dim paramgraph As Paragraph = section_Renamed.AddParagraph() paramgraph.AppendText("This text is " & align.ToString()) paramgraph.Format.HorizontalAlignment = align Next align End Sub Private Sub AppendIndentationText(ByVal section_Renamed As Section) Dim paragraph_Renamed As Paragraph = Nothing paragraph_Renamed = section_Renamed.AddParagraph() 'Append Text paragraph_Renamed.AppendText("Indentation") paragraph_Renamed.ApplyStyle(BuiltinStyle.Heading3) paragraph_Renamed = section_Renamed.AddParagraph() paragraph_Renamed.AppendText("Indentation is the spacing between text and margins. Word allows you to set left and right margins, as well as indentations for the first line of a paragraph and hanging indents") paragraph_Renamed.Format.FirstLineIndent = 15 End Sub Private Sub AppendBulletedList(ByVal section_Renamed As Section) Dim paragraph_Renamed As Paragraph = Nothing paragraph_Renamed = section_Renamed.AddParagraph() 'Append Text paragraph_Renamed.AppendText("Bulleted List") paragraph_Renamed.ApplyStyle(BuiltinStyle.Heading3) paragraph_Renamed = section_Renamed.AddParagraph() For i As Integer = 0 To 4 paragraph_Renamed = section_Renamed.AddParagraph() paragraph_Renamed.AppendText("Item" & i.ToString()) If i = 0 Then paragraph_Renamed.ListFormat.ApplyBulletStyle() Else paragraph_Renamed.ListFormat.ContinueListNumbering() End If paragraph_Renamed.ListFormat.ListLevelNumber = 1 Next i End Sub Private Sub WordDocViewer(ByVal fileName As String) Try Process.Start(fileName) Catch End Try End Sub
Published in
Formating
Saturday, 03 July 2010 00:00
Excel TextAlign in C#, VB.NET
The sample demonstrates how to set text alignment in an excel workbook.
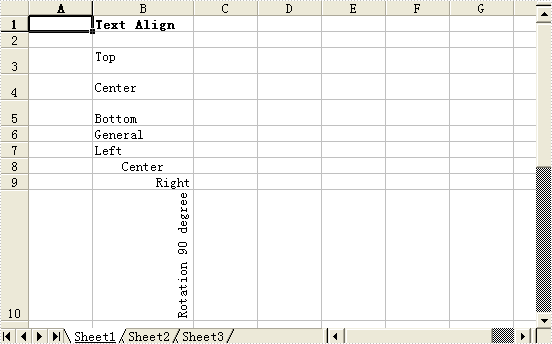
private void ExcelDocViewer( string fileName ) { try { System.Diagnostics.Process.Start(fileName); } catch{} } private void btnRun_Click(object sender, System.EventArgs e) { Workbook workbook = new Workbook(); Worksheet sheet = workbook.Worksheets[0]; sheet.Range["B1"].Text = "Text Align"; sheet.Range["B1"].Style.Font.IsBold = true; sheet.Range["B3"].Text = "Top"; sheet.Range["B3"].Style.VerticalAlignment = VerticalAlignType.Top; sheet.Range["B4"].Text = "Center"; sheet.Range["B4"].Style.VerticalAlignment = VerticalAlignType.Center; sheet.Range["B5"].Text = "Bottom"; sheet.Range["B5"].Style.VerticalAlignment = VerticalAlignType.Bottom; sheet.Range["B6"].Text = "General"; sheet.Range["B6"].Style.HorizontalAlignment = HorizontalAlignType.General; sheet.Range["B7"].Text = "Left"; sheet.Range["B7"].Style.HorizontalAlignment = HorizontalAlignType.Left; sheet.Range["B8"].Text = "Center"; sheet.Range["B8"].Style.HorizontalAlignment = HorizontalAlignType.Center; sheet.Range["B9"].Text = "Right"; sheet.Range["B9"].Style.HorizontalAlignment = HorizontalAlignType.Right; sheet.Range["B10"].Text = "Rotation 90 degree"; sheet.Range["B10"].Style.Rotation = 90; sheet.Range["B11"].Text = "Rotation 45 degree"; sheet.Range["B11"].Style.Rotation = 45; sheet.AllocatedRange.AutoFitColumns(); sheet.Range["B3:B5"].RowHeight = 20; workbook.SaveToFile("Sample.xls"); ExcelDocViewer(workbook.FileName); }
Private Sub ExcelDocViewer(ByVal fileName As String) Try System.Diagnostics.Process.Start(fileName) Catch End Try End Sub Private Sub btnRun_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnRun.Click Dim workbook As Workbook = New Workbook() Dim sheet As Worksheet = workbook.Worksheets(0) sheet.Range("B1").Text = "Text Align" sheet.Range("B1").Style.Font.IsBold = True sheet.Range("B3").Text = "Top" sheet.Range("B3").Style.VerticalAlignment = VerticalAlignType.Top sheet.Range("B4").Text = "Center" sheet.Range("B4").Style.VerticalAlignment = VerticalAlignType.Center sheet.Range("B5").Text = "Bottom" sheet.Range("B5").Style.VerticalAlignment = VerticalAlignType.Bottom sheet.Range("B6").Text = "General" sheet.Range("B6").Style.HorizontalAlignment = HorizontalAlignType.General sheet.Range("B7").Text = "Left" sheet.Range("B7").Style.HorizontalAlignment = HorizontalAlignType.Left sheet.Range("B8").Text = "Center" sheet.Range("B8").Style.HorizontalAlignment = HorizontalAlignType.Center sheet.Range("B9").Text = "Right" sheet.Range("B9").Style.HorizontalAlignment = HorizontalAlignType.Right sheet.Range("B10").Text = "Rotation 90 degree" sheet.Range("B10").Style.Rotation = 90 sheet.Range("B11").Text = "Rotation 45 degree" sheet.Range("B11").Style.Rotation = 45 sheet.AllocatedRange.AutoFitColumns() sheet.Range("B3:B5").RowHeight = 20 workbook.SaveToFile("Sample.xls") ExcelDocViewer(workbook.FileName) End Sub
Published in
Styles