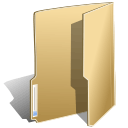
.NET (1273)
Children categories
How to Expand/Collapse the rows in an existing Pivot Table in C#
2014-07-25 02:52:19 Written by support icebluePivot table displays the data in sort, count total or give the average of the data stored in one table or spreadsheet. So it gives readers clear information of the data's trends and patterns rather than a large amount of similar data. Sometimes, there are so many rows in one pivot table and we may need to expand or collapse them to make the pivot table more clearly.
By using Spire.XLS for .NET, developers can create pivot table. This article will show you how to expand and collapse the rows in an existing Pivot table in C#.
Firstly, make sure that Spire.XLS for .NET (version7.5.5 or above) has been installed on your machine. And then, adds Spire.XLS.dll as reference in the downloaded Bin folder thought the below path: "..\Spire.XLS\Bin\NET4.0\ Spire.XLS.dll".
//Create a new excel document Workbook book = new Workbook(); //load an excel document with Pivot table from the file book.LoadFromFile("test.xlsx"); //Find the Pivot Table sheet Worksheet sheet = book.Worksheets["Pivot Table"]; //Get the data in Pivot Table Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotTable pivotTable = sheet.PivotTables[0] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotTable; //Calculate Data pivotTable.CalculateData(); //Collapse the rows (pivotTable.PivotFields["Vendor No"] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotField).HideItemDetail("1501", true); //Expand the rows (pivotTable.PivotFields["Vendor No"] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotField).HideItemDetail("1502", false); //Save the document to file book.SaveToFile("result.xlsx", ExcelVersion.Version2007);
Effective screenshots:
Collapse the rows in Pivot table in C#
Expand the rows in Pivot table in C#
Full codes:
using Spire.Xls; namespace HighlightValues { class Program { static void Main(string[] args) { Workbook book = new Workbook(); book.LoadFromFile("test.xlsx"); Worksheet sheet = book.Worksheets["Pivot Table"]; Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotTable pivotTable = sheet.PivotTables[0] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotTable; pivotTable.CalculateData(); (pivotTable.PivotFields["Vendor No"] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotField).HideItemDetail("1501", true); (pivotTable.PivotFields["Vendor No"] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotField).HideItemDetail("1502", true); book.SaveToFile("result_1.xlsx", ExcelVersion.Version2007); (pivotTable.PivotFields["Vendor No"] as Spire.Xls.Core.Spreadsheet.PivotTables.XlsPivotField).HideItemDetail("1502", false); book.SaveToFile("result_2.xlsx", ExcelVersion.Version2007); } } }
Spire.PDF for .NET is a PDF component which contains an incredible wealth of features to create, read, edit and manipulate PDF documents on .NET, Silverlight and WPF Platform. As a professional .NET PDF component, it also includes many useful features, for example, functionalities of adding header and footer, drawing table, saving PDF document as tiff and Splitting tiff image and drawing to pdf document without installing Adobe Acrobat or any other third party libraries.
This article would introduce a detail method to split the tiff image and draw to pdf document. The below picture show the effect of splitting tiff image and drawing to pdf document:
The main steps of the method are:
One: Split multi-page TIFF file to multiple frames
- Load a multipage Tiff.
- Use Image.GetFrameCount method to get the number of frames of tiff Image.
- Initialize a new instance of Guid to save the dimension from tiff image.
- Using Guid to initialize a new instance of the System.Drawing.Imaging.FrameDimension class.
- Iterate over the Tiff Frame Collection and save them to Image array.
Two: Draw image to PDF
- Initialize a new instance of Spire.Pdf.PdfDocument.
- Iterate over the image array.
- Use Spire.Pdf.PdfPageBase.Canvas.DrawImage method to draw image into page with the specified coordinates and image size.
Download and install Spire.Pdf for .NET and use below code to experience this method to split tiff and convert it to pdf document.
The full code of method:
- C#
- VB.NET
using System; using System.Drawing; using System.Drawing.Imaging; using System.IO; using Spire.Pdf; using Spire.Pdf.Graphics; namespace SplitTiff { class Program { static void Main(string[] args) { using (PdfDocument pdfDocument = new PdfDocument()) { Image tiffImage = Image.FromFile(@"..\..\demo.tiff"); Image[] images = SplitTIFFImage(tiffImage); for (int i = 0; i < images.Length; i++) { PdfImage pdfImg = PdfImage.FromImage(images[i]); PdfPageBase page = pdfDocument.Pages.Add(); float width = pdfImg.Width * 0.5f; float height = pdfImg.Height * 0.5f; float x = (page.Canvas.ClientSize.Width - width) / 2; //set the image of the page page.Canvas.DrawImage(pdfImg, x, 0, width, height); } pdfDocument.SaveToFile(@"..\..\result.pdf"); System.Diagnostics.Process.Start(@"..\..\result.pdf"); } } public static Image[] SplitTIFFImage(Image tiffImage) { int frameCount = tiffImage.GetFrameCount(FrameDimension.Page); Image[] images = new Image[frameCount]; Guid objGuid = tiffImage.FrameDimensionsList[0]; FrameDimension objDimension = new FrameDimension(objGuid); for (int i = 0; i < frameCount; i++) { tiffImage.SelectActiveFrame(objDimension, i); using (MemoryStream ms = new MemoryStream()) { tiffImage.Save(ms, ImageFormat.Tiff); images[i] = Image.FromStream(ms); } } return images; } } }
If you are interested in the method of converting pdf to tiff image you can refer the Save PDF Document as tiff image article in our website.
How to reset page number for each section start at 1 in a word document in C#
2014-07-17 09:27:54 Written by support iceblueSections are widely used by developers to set different formatting or layout options to each different section, such as use the different header and footer information for different sections and reset page number for each section dynamically. With the help of Spire.Doc for .NET, we can easily insert word section and remove word section in C# and VB.NET. We will show you how to reset page numbering that starts at 1 for each section easily by using Spire.Doc.
Firstly make sure Spire.Doc for .NET has been installed correctly and then add Spire.Doc.dll as reference in the downloaded Bin folder though the below path: "..\Spire.Doc\Bin\NET4.0\ Spire.Doc.dll". Here comes to the codes of how to reset page numbering for each section.
Step 1: Load three different word documents
Document document1 = new Document(); document1.LoadFromFile("..\\..\\1.docx"); Document document2 = new Document(); document2.LoadFromFile("..\\..\\2.docx"); Document document3 = new Document(); document3.LoadFromFile("..\\..\\3.docx");
Step 2: Use section method to combine all documents into one word document
foreach (Section sec in document2.Sections) { document1.Sections.Add(sec.Clone()); } foreach (Section sec in document3.Sections) { document1.Sections.Add(sec.Clone()); }
Step 3: Traverse the document
//Traverse every section of document1 foreach (Section sec in document1.Sections) { //Traverse every object of the footer foreach (DocumentObject obj in sec.HeadersFooters.Footer.ChildObjects) { if (obj.DocumentObjectType == DocumentObjectType.StructureDocumentTag) { DocumentObject para = obj.ChildObjects[0]; foreach (DocumentObject item in para.ChildObjects) { if (item.DocumentObjectType == DocumentObjectType.Field)
Step 4: Find the field type FieldNumPages and change it to FieldSectionPages
//Find the item and its field type is FieldNumPages if ((item as Field).Type == FieldType.FieldNumPages) { //Change field type to FieldSectionPages (item as Field).Type = FieldType.FieldSectionPages;
Step 5: Restart page number of section and set the starting page number to 1
document1.Sections[1].PageSetup.RestartPageNumbering = true; document1.Sections[1].PageSetup.PageStartingNumber = 1; document1.Sections[2].PageSetup.RestartPageNumbering = true; document1.Sections[2].PageSetup.PageStartingNumber = 1;
Step 6: Save the document to file and launch it.
document1.SaveToFile("sample.docx",FileFormat.Docx); System.Diagnostics.Process.Start("sample.docx");
Full codes:
namespace ResetPageNumber { class Program { static void Main(string[] args) { Document document1 = new Document(); document1.LoadFromFile("..\\..\\1.docx"); Document document2 = new Document(); document2.LoadFromFile("..\\..\\2.docx"); Document document3 = new Document(); document3.LoadFromFile("..\\..\\3.docx"); foreach (Section sec in document2.Sections) { document1.Sections.Add(sec.Clone()); } foreach (Section sec in document3.Sections) { document1.Sections.Add(sec.Clone()); } foreach (Section sec in document1.Sections) { foreach (DocumentObject obj in sec.HeadersFooters.Footer.ChildObjects) { if (obj.DocumentObjectType == DocumentObjectType.StructureDocumentTag) { DocumentObject para = obj.ChildObjects[0]; foreach (DocumentObject item in para.ChildObjects) { if (item.DocumentObjectType == DocumentObjectType.Field) { if ((item as Field).Type == FieldType.FieldNumPages) { (item as Field).Type = FieldType.FieldSectionPages; } } } } } } document1.Sections[1].PageSetup.RestartPageNumbering = true; document1.Sections[1].PageSetup.PageStartingNumber = 1; document1.Sections[2].PageSetup.RestartPageNumbering = true; document1.Sections[2].PageSetup.PageStartingNumber = 1; document1.SaveToFile("sample.docx",FileFormat.Docx); System.Diagnostics.Process.Start("sample.docx"); } } }
In Microsoft PowerPoint, a comment is a note that you can attach to a phrase or paragraph on a slide. Viewing comments added by the author, readers can learn more information about the content. Likewise, readers can also add comments to provide reviews or feedbacks to the author. In this article, you will learn how to programmatically add or remove comments in a PowerPoint slide using Spire.Presentation for .NET.
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Add Comments to a Presentation Slide
The detailed steps are as follows:
- Create a Presentation instance.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Get CommentAuthor List using Presentation.CommentAuthors property.
- Add the author of the comment using CommentAuthorList.AddAuthor() method.
- Get a specified slide using Presentation.Slides[] property, and then add a comment to the slide using ISlide.AddComment(ICommentAuthor, String, PointF, DateTime) method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; using System; namespace AddComment { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation presentation = new Presentation(); //Load a PowerPoint document presentation.LoadFromFile(@"D:\Files\Test.pptx"); //Add the author of the comment ICommentAuthor author = presentation.CommentAuthors.AddAuthor("E-iceblue", "comment:"); //Add a comment to the specified slide presentation.Slides[0].AddComment(author, "Summary of Spire.Presentation functions", new System.Drawing.PointF(25, 22), DateTime.Now); //Save the document presentation.SaveToFile("comment.pptx", FileFormat.Pptx2010); } } }
Remove Comments from a Presentation Slide
The detailed steps are as follows:
- Create a Presentation instance.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Get a specified slide using Presentation.Slides[] property.
- Remove comment from the specified slide using ISlide.DeleteComment(Comment) method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace RemoveComment { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation presentation = new Presentation(); //Load a PowerPoint document presentation.LoadFromFile("comment.pptx"); //Get the first slide ISlide slide = presentation.Slides[0]; //Remove comment from the specified slide slide.DeleteComment(slide.Comments[0]); //Save the document presentation.SaveToFile("RemoveComment.pptx", FileFormat.Pptx2010); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Spire.PDF has a function of adding, removing the blank pages in C#. We have already shown you how to remove the blank page in a PDF file. This article will show you how to insert an empty page in a PDF file in C#. By using the Spire.PDF, we can add the blank page to any place in the PDF file you want, such as at the first, the middle of the PDF file or at the end of the PDF file. It is very easy and you only need three lines of code to accomplish this task.
Make sure Spire.PDF for .NET has been installed correctly and then add Spire.Pdf.dll as reference in the downloaded Bin folder though the below path: "..\Spire.Pdf\Bin\NET4.0\Spire.Pdf.dll".
The following code snippet shows you how to insert an empty page in a PDF file. We will show you how to add the empty page at the end of the file and as the second page of the file.
//create a PDF document and load file PdfDocument doc = new PdfDocument(); doc.LoadFromFile("sample.pdf"); //insert blank page at the end of the PDF file doc.Pages.Add(); //insert blank page as the second page doc.Pages.Insert(1); //Save the document to file doc.SaveToFile("result.pdf");
Check the effective screenshots as below:
Add the blank page at the end of the PDF file:
Add the blank page as the second page of the PDF file:
Full codes:
using Spire.Pdf; using System; namespace InsertPage { class Program { static void Main(string[] args) { //create PdfDocument instance and load file PdfDocument doc = new PdfDocument(); doc.LoadFromFile("sample.pdf"); //insert blank page as last page doc.Pages.Add(); doc.SaveToFile("result.pdf"); doc.Close(); System.Diagnostics.Process.Start("result.pdf"); //create PdfDocument instance and load file PdfDocument doc2 = new PdfDocument(); doc2.LoadFromFile("sample.pdf"); //insert blank page as second page doc2.Pages.Insert(1); doc2.SaveToFile("result2.pdf"); doc2.Close(); System.Diagnostics.Process.Start("result2.pdf"); } } }
PDF files are widely used for sharing and viewing documents across different platforms, while TIFF files are preferred for storing high-quality images with detailed graphics or photographs. Converting a PDF file to TIFF can maintain the quality of images within the file. Similarly, converting a TIFF image to PDF ensures that the image can be easily viewed, shared, and printed without compatibility issues. In this article, you will learn how to programmatically convert PDF to TIFF or TIFF to PDF in C# using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.PDF
Convert PDF to TIFF in C#
The TIFF format allows multiple images to be stored in a single file. With Spire.PDF for .NET, you can convert each page of a PDF file into a separate image, and then call the custom method JoinTiffImages() to combine these images and save them as a single TIFF image.
The following are the steps to convert a PDF into a multi-page TIFF file using C#.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.LoadFromFile() method.
- Call custom method SaveAsImage() to convert each page of the PDF to a separate image.
- Call custom method JoinTiffImages() to merge the converted images into a multi-page TIFF image.
- C#
using System; using System.Drawing; using System.Drawing.Imaging; using Spire.Pdf; namespace SavePdfAsTiff { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.LoadFromFile("Report.pdf"); //Convert PDF pages to images Image[] images = SaveAsImage(pdf); //Combine the images and save them as a multi-page TIFF file JoinTiffImages(images, "result.tiff", EncoderValue.CompressionLZW); } private static Image[] SaveAsImage(PdfDocument document) { //Create a new image array Image[] images = new Image[document.Pages.Count]; //Iterate through all pages in the document for (int i = 0; i < document.Pages.Count; i++) { //Convert a specific page to an image images[i] = document.SaveAsImage(i); } return images; } private static ImageCodecInfo GetEncoderInfo(string mimeType) { //Get the image encoders ImageCodecInfo[] encoders = ImageCodecInfo.GetImageEncoders(); for (int j = 0; j < encoders.Length; j++) { //Find the encoder that matches the specified MIME type if (encoders[j].MimeType == mimeType) return encoders[j]; } throw new Exception(mimeType + " mime type not found in ImageCodecInfo"); } public static void JoinTiffImages(Image[] images, string outFile, EncoderValue compressEncoder) { //Set the encoder parameters Encoder enc = Encoder.SaveFlag; EncoderParameters ep = new EncoderParameters(2); ep.Param[0] = new EncoderParameter(enc, (long)EncoderValue.MultiFrame); ep.Param[1] = new EncoderParameter(Encoder.Compression, (long)compressEncoder); //Get the first image Image pages = images[0]; //Initialize a frame int frame = 0; //Get an ImageCodecInfo object for processing TIFF image codec information ImageCodecInfo info = GetEncoderInfo("image/tiff"); //Iterate through each Image foreach (Image img in images) { //If it's the first frame, save it to the output file with specified encoder parameters if (frame == 0) { pages = img; pages.Save(outFile, info, ep); } else { //Save the intermediate frames ep.Param[0] = new EncoderParameter(enc, (long)EncoderValue.FrameDimensionPage); pages.SaveAdd(img, ep); } //If it's the last frame, flush the encoder parameters and close the file if (frame == images.Length - 1) { ep.Param[0] = new EncoderParameter(enc, (long)EncoderValue.Flush); pages.SaveAdd(ep); } frame++; } } } }
Convert TIFF to PDF in C#
To convert a multi-page TIFF image to a PDF file, you need to convert each frame of the TIFF image to a separate PDF image. Then draw each image at a specified location on a PDF page through the PdfPageBase.Canvas.DrawImage() method.
The following are the steps to convert a TIFF image to a PDF file using C#.
- Create a PdfDocument object.
- Load a TIFF image using Image.FromFile() method.
- Call custom method SplitTiffImage() to split the TIFF image into separate images.
- Iterate through the split images, and then convert each into a PDF image.
- Add a page to the PDF document using PdfDocument.Pages.Add() method.
- Draw the PDF image at a specified location on the page using PdfPageBase.Canvas.DrawImage() method.
- Save the result PDF file using PdfDocument.SaveToFile() method.
- C#
using System; using System.Drawing; using System.Drawing.Imaging; using System.IO; using Spire.Pdf; using Spire.Pdf.Graphics; namespace TiffToPdf { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument pdf = new PdfDocument(); //Load a TIFF image Image tiffImage = Image.FromFile("result.tiff"); //Split the Tiff image into separate images Image[] images = SplitTiffImage(tiffImage); //Iterate through the images for (int i = 0; i < images.Length; i++) { //Convert a specified image into a PDF image PdfImage pdfImg = PdfImage.FromImage(images[i]); //Get image width and height float width = pdfImg.Width; float height = pdfImg.Height; //Add a page with the same size as the image SizeF size = new SizeF(width, height); PdfPageBase page = pdf.Pages.Add(size); //Draw the image at a specified location on the page page.Canvas.DrawImage(pdfImg, 0, 0, width, height); } //Save the result file pdf.SaveToFile("TiffToPdf.pdf"); } public static Image[] SplitTiffImage(Image tiffImage) { //Get the number of frames in the Tiff image int frameCount = tiffImage.GetFrameCount(FrameDimension.Page); //Create an image array to store the split tiff images Image[] images = new Image[frameCount]; //Gets the GUID of the first frame dimension Guid objGuid = tiffImage.FrameDimensionsList[0]; //Create a FrameDimension object FrameDimension objDimension = new FrameDimension(objGuid); //Iterate through each frame for (int i = 0; i < frameCount; i++) { //Select a specified frame tiffImage.SelectActiveFrame(objDimension, i); //Save the frame in TIFF format to a memory stream MemoryStream ms = new MemoryStream(); tiffImage.Save(ms, ImageFormat.Tiff); //Load an image from memory stream images[i] = Image.FromStream(ms); } return images; } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Excel documents are easy to print, but it would be a bit tricky if you have some special printing requirements. For example, printing only selected range of a sheet, repeating the header row on each page, or fitting a worksheet on one page. This article covers how to set Excel print options via page setup and how to send an Excel document to printer in C# and VB.NET by using Spire.XLS for .NET.
- Set Excel Print Options via Page Setup in C# and VB.NET
- Print Excel Documents Using Print Dialog in C# and VB.NET
- Silently Print Excel Documents in C# and VB.NET
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.XLS
Set Excel Print Options via Page Setup in C# and VB.NET
Excel Page Setup provides options to control how a worksheet will be printed, such as whether to print comments, whether to print gridlines and specify the cell range to print. Spire.XLS offers the PageSetup object to deal with these things. The following are the steps to set Excel print options through PageSetup using Spire.XLS for .NET.
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet through Workbook.Worksheets[index] property.
- Get PageSetup object through Worksheet.PageSetup property.
- Set page margins, print area, pint title row, print quality, etc. through the properties under PageSetup object.
- Save the workbook to another Excel file using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; namespace PrintOptions { class Program { static void Main(string[] args) { //Create a workbook Workbook workbook = new Workbook(); //Load an Excel document workbook.LoadFromFile(@"C:\Users\Administrator\Desktop\sample.xlsx"); //Get the first worksheet Worksheet worksheet = workbook.Worksheets[0]; //Get the PageSetup object of the first worksheet PageSetup pageSetup = worksheet.PageSetup; //Set page margins pageSetup.TopMargin = 0.3; pageSetup.BottomMargin = 0.3; pageSetup.LeftMargin = 0.3; pageSetup.RightMargin = 0.3; //Specify print area pageSetup.PrintArea = "A1:D10"; //Specify title row pageSetup.PrintTitleRows = "$1:$2"; //Allow to print with row/column headings pageSetup.IsPrintHeadings = true; //Allow to print with gridlines pageSetup.IsPrintGridlines = true; //Allow to print comments as displayed on worksheet pageSetup.PrintComments = PrintCommentType.InPlace; //Set printing quality (dpi) pageSetup.PrintQuality = 300; //Allow to print worksheet in black & white mode pageSetup.BlackAndWhite = true; //Set the printing order pageSetup.Order = OrderType.OverThenDown; //Fit worksheet on one page pageSetup.IsFitToPage = true; //Save the workbook workbook.SaveToFile("PagePrintOptions.xlsx", ExcelVersion.Version2016); } } }
Print Excel Documents Using Print Dialog in C# and VB.NET
A Print Dialog box lets users to select options for a particular print job. For example, the user can specify the printer to use. The following are the steps to send an Excel document to a print dialog using Spire.XLS for .NET.
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Create a PrintDialog object.
- Specify printer settings through the properties under PrintDialog object.
- Apply the print dialog to workbook.
- Get PrintDocument object from the workbook through Workbook.PrintDocument property.
- Invoke the print dialog and start printing using PrintDocument.Print() method.
- C#
- VB.NET
using System; using Spire.Xls; using System.Drawing.Printing; using System.Windows.Forms; namespace PrintExcelUsingPrintDialog { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { //Create a Workbook object Workbook workbook = new Workbook(); //Load an Excel file workbook.LoadFromFile(@"C:\Users\Administrator\Desktop\sample.xlsx"); //Fit worksheet on one page PageSetup pageSetup = workbook.Worksheets[0].PageSetup; pageSetup.IsFitToPage = true; //Create a PrintDialog object PrintDialog dialog = new PrintDialog(); //Specify printer settings dialog.AllowCurrentPage = true; dialog.AllowSomePages = true; dialog.AllowSelection = true; dialog.UseEXDialog = true; dialog.PrinterSettings.Duplex = Duplex.Simplex; //Apply the dialog to workbook workbook.PrintDialog = dialog; //Create a PrintDocument object based on the workbook PrintDocument printDocument = workbook.PrintDocument; //Invoke the print dialog if (dialog.ShowDialog() == DialogResult.OK) { printDocument.Print(); } } } }
Silently Print Excel Documents in C# and VB.NET
If you do not want to see the print dialog or the print process, you can silently print Excel documents to a specified printer. The following are the steps.
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Set the print controller to StandardPrintController, which will prevent print process from showing.
- Get PrinterSettings object from the workbook through Workbook.PrintDocument.PrinterSettings property.
- Specify printer name, duplex mode and print pages through the properties under PrinerSettings object.
- Print the workbook using Workbook.PrintDocument.Print() method.
- C#
- VB.NET
using Spire.Xls; using System.Drawing.Printing; namespace SilentlyPrint { class Program { static void Main(string[] args) { //Create a Workbook object Workbook workbook = new Workbook(); //Load an Excel file workbook.LoadFromFile(@"C:\Users\Administrator\Desktop\sample.xlsx"); //Fit worksheet on one page PageSetup pageSetup = workbook.Worksheets[0].PageSetup; pageSetup.IsFitToPage = true; //Set the print controller to StandardPrintController, which will prevent print process from showing workbook.PrintDocument.PrintController = new StandardPrintController(); //Get PrinterSettings from the workbook PrinterSettings settings = workbook.PrintDocument.PrinterSettings; //Specify printer name, duplex mode and print pages settings.PrinterName = "HP LaserJet P1007"; settings.Duplex = Duplex.Simplex; settings.FromPage = 1; settings.ToPage = 3; //Print the workbook workbook.PrintDocument.Print(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Spire.XLS for .NET is a professional Excel component which enables developers/programmers to fast generate, read, write and modify Excel document for .NET. Spire.XLS for .NET doesn't need Microsoft Office Excel Automation. It allows user to operate Excel document directly such as save to stream, save as web response, copy, lock/unlock worksheet, set up workbook properties, etc. As a professional .NET Excel component, it also includes many useful features, for example, functionalities of importing data from Excel to dataTable and exporting dataTable to Excel from Database.
In this article introduces a method of updating excel data by dataTable via using sheet.ExportDataTable() method and sheet.InsertDataTable() method to import data from excel to dataTable and export dataTable to excel from Database.
The main steps of method are:
Step 1: Load the excel document and use sheet.ExportDataTable() method extract data to dataTable and show by dataGridView control.
private void Form1_Load(object sender, EventArgs e) { //load excel document to workbook workbook.LoadFromFile(@"DatatableSample.xls"); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Original table"; //extract data to dataTable from sheet DataTable dataTable = sheet.ExportDataTable(); //show the data to dataGridView this.dataGridView.DataSource = dataTable; }
The effect screenshot:
Step 2: Create a new sheet to save the updata data and use sheet.InsertDataTable() method to insert dataTable to the sheet.
//create a new sheet to save Updata data. Worksheet sheet = workbook.CreateEmptySheet("Updata Table"); //extract data from dataGridView DataTable dataTable = this.dataGridView.DataSource as DataTable; // insert dataTable to sheet sheet.InsertDataTable(dataTable, true, 1, 1);
Step 3: Save the result excel document.
workbook.SaveToFile("result.xlsx", ExcelVersion.Version2007);
The effect screenshot:
Download and install Spire.XLS for .NET and use below code to experience this method to update excel data by dataTable.
The full code:
using System; using System.Data; using System.Windows.Forms; using Spire.Xls; namespace UpdataExcelDataByDataTable { public partial class UpdataExcelData : Form { private Workbook workbook = new Workbook(); private void Form1_Load(object sender, EventArgs e) { workbook.LoadFromFile(@"DatatableSample.xls"); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Original table"; DataTable dataTable = sheet.ExportDataTable(); this.dataGridView.DataSource = dataTable; } private void Updata_Click(object sender, EventArgs e) { Worksheet sheet = workbook.CreateEmptySheet("Updata Table"); DataTable dataTable = this.dataGridView.DataSource as DataTable; sheet.InsertDataTable(dataTable, true, 1, 1); workbook.SaveToFile("result.xlsx", ExcelVersion.Version2007); System.Diagnostics.Process.Start("result.xlsx"); } } }
Imports System.Data Imports System.Windows.Forms Imports Spire.Xls Public Class Form1 Private workbook As New Workbook() Private Sub Form1_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load 'load excel document to workbook workbook.LoadFromFile("DatatableSample.xls") Dim sheet As Worksheet = workbook.Worksheets(0) sheet.Name = "Original table" 'extract data to dataTable from sheet Dim dataTable As DataTable = sheet.ExportDataTable() 'show the data to dataGridView Me.DataGridView.DataSource = dataTable End Sub
If you couldn't successfully use the Spire.Xls, please refer Spire.XLS Quick Start which can guide you quickly use the Spire.Xls.
This sample demo has demonstrated how to draw nested grid in PDF document and set grid row&cell format. In the following section, we are going to create a simple PDF grid and show you how to insert an image to a specific PDF grid cell in C#. Before we can follow the code snippet below to accomplish the task, we have to prepare the environment first.
Download Spire.PDF and install it on system, create or open a .NET class application in Visual Studio 2005 or above versions, add Spire.PDF.dll to your .NET project assemblies.Then let's code step by step to make a better understanding about the whole procedure.
Step 1: Create a PDF document and add a new page.
PdfDocument doc = new PdfDocument(); PdfPageBase page = doc.Pages.Add();
Step 2: Create a 2×2 grid to PDF.
PdfGrid grid = new PdfGrid(); PdfGridRow row = grid.Rows.Add(); row = grid.Rows.Add(); grid.Columns.Add(2);
Step 3: Set the cell padding of the PDF grid.
grid.Style.CellPadding = new PdfPaddings(1, 1, 1, 1);
Step 4: Set the width of the columns.
float width = page.Canvas.ClientSize.Width - (grid.Columns.Count + 1); grid.Columns[0].Width = width * 0.25f; grid.Columns[1].Width = width * 0.25f;
Step 5: Load an image from disk.
PdfGridCellContentList lst = new PdfGridCellContentList(); PdfGridCellContent textAndStyle = new PdfGridCellContent(); textAndStyle.Image = PdfImage.FromFile("..\\..\\image1.jpg");
Step 6: Set the size of image and insert it to the first cell.
textAndStyle.ImageSize = new SizeF(50, 50); lst.List.Add(textAndStyle); grid.Rows[0].Cells[0].Value = lst; grid.Rows[1].Height = grid.Rows[0].Height;
Step 7: Draw PDF grid into page at the specific location.
PdfLayoutResult result = grid.Draw(page, new PointF(10, 30));
Step 8: Save to a PDF file and launch the file.
doc.SaveToFile(outputFile, FileFormat.PDF); System.Diagnostics.Process.Start(outputFile);
Result:
Full C# Code:
using Spire.Pdf; using Spire.Pdf.Graphics; using Spire.Pdf.Grid; using System.Drawing; namespace InsertImage { class Program { static void Main(string[] args) { string outputFile = @"..\..\output.pdf"; //Create a pdf document PdfDocument doc = new PdfDocument(); //Add a page for the pdf document PdfPageBase page = doc.Pages.Add(); //Create a pdf grid PdfGrid grid = new PdfGrid(); //Set the cell padding of pdf grid grid.Style.CellPadding = new PdfPaddings(1, 1, 1, 1); //Add a row for pdf grid PdfGridRow row = grid.Rows.Add(); //Add two columns for pdf grid grid.Columns.Add(2); float width = page.Canvas.ClientSize.Width - (grid.Columns.Count + 1); //Set the width of the first column grid.Columns[0].Width = width * 0.25f; grid.Columns[1].Width = width * 0.25f; //Add a image PdfGridCellContentList lst = new PdfGridCellContentList(); PdfGridCellContent textAndStyle = new PdfGridCellContent(); textAndStyle.Image = PdfImage.FromFile("..\\..\\image1.jpg"); //Set the size of image textAndStyle.ImageSize = new SizeF(50, 50); lst.List.Add(textAndStyle); //Add a image into the first cell. row.Cells[0].Value = lst; //Draw pdf grid into page at the specific location PdfLayoutResult result = grid.Draw(page, new PointF(10, 30)); //Save to a pdf file doc.SaveToFile(outputFile, FileFormat.PDF); System.Diagnostics.Process.Start(outputFile); } } }
Counting the number of pages in a PDF file is essential for various purposes, such as determining document length, organizing content, and evaluating printing requirements. Apart from knowing page count information using PDF viewers, you can also automate the task through programming. In this article, you will learn how to use C# to get the number of pages in a PDF file using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.PDF
Get the Number of Pages in a PDF File in C#
Spire.PDF for .NET offers the PdfDocument.Pages.Count property to quickly count the number of pages in a PDF file without opening it. The following are the detailed steps.
- Create a PdfDocument object.
- Load a sample PDF file using PdfDocument.LoadFromFile() method.
- Count the number of pages in the PDF file using PdfDocument.Pages.Count property.
- Output the result and close the PDF.
- C#
using Spire.Pdf; namespace GetNumberOfPages { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument pdf = new PdfDocument(); //Load a sample PDF file pdf.LoadFromFile("Contract.pdf"); //Count the number of pages in the PDF int PageNumber = pdf.Pages.Count; Console.WriteLine("The PDF file has {0} pages", PageNumber); //Close the PDF pdf.Close(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
