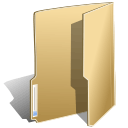
.NET (1270)
Children categories
How to modify hyperlinks in PowerPoint documents in C#
2014-08-20 01:14:28 Written by support iceblueHyperlinks can easily guide the readers to the place you want to point to and it displays large amount of information to readers. Spire.Presentation for .NET enables developers to insert hyperlinks in PowerPoint presentations. Developers can also modify the existing hyperlinks and replace with new link text or target URL. This section will show how to edit hyperlinks from presentation slides in C#. Firstly check the screenshot of the original hyperlink:
Spire.Presentation offers a ClickAction class for users to edit the hyperlinks. You can call the function ClickHyperlink to edit the hyperlinks. Here comes to the steps.
Step 1: Load a PowerPoint documents with hyperlinks.
Presentation pre = new Presentation(); pre.LoadFromFile(@"..\..\sample.pptx");
Step 2: Find the hyperlinks you want to edit.
IAutoShape shape = (IAutoShape)pre.Slides[0].Shapes[2];
Step 3: Edit the link text and the target URL.
shape.TextFrame.TextRange.ClickAction.Address = "http://www.e-iceblue.com"; shape.TextFrame.TextRange.Text = "E-iceblue";
Step 4: Save the document.
pre.SaveToFile(@"..\..\result.pptx",FileFormat.Pptx2010);
Effective screenshot after edit the hyperlink on presentation slide:
Full codes:
namespace EditHyperlink { class Program { static void Main(string[] args) { Presentation pre = new Presentation(); pre.LoadFromFile(@"..\..\sample.pptx"); IAutoShape shape = (IAutoShape)pre.Slides[0].Shapes[2]; shape.TextFrame.TextRange.ClickAction.Address = "http://www.e-iceblue.com"; shape.TextFrame.TextRange.Text = "E-iceblue"; pre.SaveToFile(@"..\..\result.pptx",FileFormat.Pptx2010); System.Diagnostics.Process.Start(@"..\..\result.pptx"); } } }
Embed image in HTML when converting Excel to HTML in C#
2014-08-15 08:02:33 Written by support iceblueIf you created a pretty Excel table and now want to publish it online as a web page, the simplest way is to export it to an old good HTML file. However a problem may occur if you just simply transform image in Excel to HTML code with a relative link (URL). This way, your web page may no longer display properly on client machines since the image can't be reached through that URL on client-side. In this article, we’re going to resolve this issue by embedding image in HTML code when converting Excel to HTML.
Here is an Excel table with some images embedded in.
We're able to convert this Excel file to HTML by following below code snippet:
Step 1: Create a new instance of workbook.
Workbook book = new Workbook(); book.LoadFromFile("Book1.xlsx");
Step 2: Embed images into HTML code using Data URI scheme.
HTMLOptions options = new HTMLOptions(); options.ImageEmbedded = true;
Step 3: Save the worksheet to HTML.
book.Worksheets[0].SaveToHtml("sample.html", options); System.Diagnostics.Process.Start("sample.html");
Output:
HTML Code:
Since the HTML code is too long to be displayed here, we have to present it by a screenshot.
Full C# Code:
using Spire.Xls; using Spire.Xls.Core.Spreadsheet; namespace CreateWorkbook { class Program { static void Main(string[] args) { // create Workbook instance and load file Workbook book = new Workbook(); book.LoadFromFile("Book1.xlsx"); // embed image into html when converting HTMLOptions options = new HTMLOptions(); options.ImageEmbedded = true; // save the sheet to html book.Worksheets[0].SaveToHtml("sample.html", options); System.Diagnostics.Process.Start("sample.html"); } } }
Replace and remove Comment on presentation slides in C#
2014-08-14 08:51:13 Written by support iceblueComments on slides are author reviews and feedbacks about specified contents. Spire.Presentation for .NET enables developers to insert comments in PowerPoint slides with several lines of core code. Developers can also edit and remove the existing comments and replace with new comments for showing different reviews. This section will show how to edit and remove comments from presentation slides in C#.
Firstly check this PowerPoint document with three comments without removing and replacing.
Here comes to the steps of how to edit and remove the comments on presentation slides in C#.
Step 1: Create a new instance of presentation class and load a sample file with comments.
Presentation presentation = new Presentation(); presentation.LoadFromFile(@"..\..\sample.pptx");
Step 2: Replace the content in the first comment.
presentation.Slides[0].Comments[0].Text = "Revised comment";
Step 3: Remove the second comment
presentation.Slides[0].DeleteComment(presentation.Slides[0].Comments[1]);
Step 4: Save the document
presentation.SaveToFile(@"..\..\comment_2.pptx", FileFormat.Pptx2010);
Effective screenshot after edit and remove the comments on presentation slides:
Full codes:
namespace Comment { class Program { static void Main(string[] args) { Presentation presentation = new Presentation(); presentation.LoadFromFile(@"..\..\sample.pptx"); //Edit the first comment presentation.Slides[0].Comments[0].Text = "Revised comment"; //Remove the second comment presentation.Slides[0].DeleteComment(presentation.Slides[0].Comments[1]); //Save the document presentation.SaveToFile(@"..\..\comment_2.pptx", FileFormat.Pptx2010); } } }
Remove borderline of textbox in Excel chart in C#, VB.NET
2014-08-12 07:57:21 Written by support iceblueThere is an article in the tutorials which demonstrates how to insert textbox with contents in Excel. Sometime back, a user of Spire.XLS wanted to know if it is possible to remove the borderline of the textbox that has been inserted in Excel chart. Yes, of course. This article focuses on delivering a solution to this issue.
In the following section, we're going to create two textboxes in the same chart, one textbox is built with borderline, the other one without. Then we can learn how to remove borderline using Spire.XLS by comparison.
Code snippet for remove borderline of textbox:
Step 1: Create a new instance of workbook.
Workbook workbook = new Workbook(); workbook.Version=ExcelVersion.Version2010;
Step 2: Create a new worksheet named "Remove Borderline" and add a chart to the worksheet.
Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Remove Borderline"; Chart chart = sheet.Charts.Add();
Step 3: Create textbox1 in the chart and input text information.
chart.TextBoxes.AddTextBox(50, 50, 100, 500).ShapeType = ExcelShapeType.TextBox; chart.TextBoxes[0].Text = "The original with borderline";
Step 4: Create textbox2 in the chart, input text information and remove borderline.
XlsTextBoxShape textbox = chart.TextBoxes.AddTextBox(500, 50, 100, 500) as XlsTextBoxShape; textbox.Text = "The solution without borderline"; textbox.Line.Weight = 0;
Step 5: Save and launch the file.
workbook.SaveToFile("Sample.xlsx", ExcelVersion.Version2010); Process.Start("Sample.xlsx");
Result:
Full code:
using Spire.Xls; using Spire.Xls.Core.Spreadsheet.Shapes; using System.Diagnostics; namespace RemoveBorderlineofTextbox { class Program { static void Main(string[] args) { Workbook workbook = new Workbook(); workbook.Version = ExcelVersion.Version2010; Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Remove Borderline"; Chart chart = sheet.Charts.Add(); //original chart.TextBoxes.AddTextBox(50, 50, 100, 500).ShapeType = ExcelShapeType.TextBox; chart.TextBoxes[0].Text = "The original with borderline"; //solution XlsTextBoxShape textbox = chart.TextBoxes.AddTextBox(500, 50, 100, 500) as XlsTextBoxShape; textbox.Text = "The solution without borderline"; //set 0 then invisible textbox.Line.Weight = 0; workbook.SaveToFile("Sample.xlsx", ExcelVersion.Version2010); Process.Start("Sample.xlsx"); } } }
Imports Spire.Xls Imports Spire.Xls.Core.Spreadsheet.Shapes Imports System.Diagnostics Namespace RemoveBorderlineofTextbox Class Program Private Shared Sub Main(args As String()) Dim workbook As New Workbook() workbook.Version = ExcelVersion.Version2010 Dim sheet As Worksheet = workbook.Worksheets(0) sheet.Name = "Remove Borderline" Dim chart As Chart = sheet.Charts.Add() 'original chart.TextBoxes.AddTextBox(50, 50, 100, 500).ShapeType = ExcelShapeType.TextBox chart.TextBoxes(0).Text = "The original with borderline" 'solution Dim textbox As XlsTextBoxShape = TryCast(chart.TextBoxes.AddTextBox(500, 50, 100, 500), XlsTextBoxShape) textbox.Text = "The solution without borderline" 'set 0 then invisible textbox.Line.Weight = 0 workbook.SaveToFile("Sample.xlsx", ExcelVersion.Version2010) Process.Start("Sample.xlsx") End Sub End Class End Namespace
Various kinds of shapes like triangle, rectangle, ellipse, star, line and etc, can be created with Spire.Presentation. To make shapes more compatible with the entire slide, not only can we set color and choose fill style of the shape, we can also rotate shapes to a desired degree. This article is aimed to provide a simple example.
To begin with, create or open a .NET class application in Visual Studio 2005 or above versions, add Spire.Presentation.dll to your .NET project assemblies. Then, you are able create and format shapes using the sample C# code we have offered below.
Code snippets for rotate shapes on slide:
Step 1: Create an instance of Presentation class.
Presentation presentation = new Presentation();
Step 2: Add a new shape - Triangle ,to PPT slide.
IAutoShape shape = presentation.Slides[0].Shapes.AppendShape(ShapeType.Triangle, new RectangleF(100, 100, 100, 100));
Step 3: Rotate the shape to 180 degree.
shape.Rotation = 180;
Step 4: Set the color and fill style of shape.
shape.Fill.FillType = FillFormatType.Solid; shape.Fill.SolidColor.Color = Color.BlueViolet; shape.ShapeStyle.LineColor.Color = Color.Black;
Step 5: Save and launch the file.
presentation.SaveToFile("shape.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("shape.pptx");
Effect Screenshot:
Full code:
using Spire.Presentation; using Spire.Presentation.Drawing; using System.Drawing; namespace RotateShape { class Program { static void Main(string[] args) { //create PPT document Presentation presentation = new Presentation(); //append new shape - Triangle IAutoShape shape = presentation.Slides[0].Shapes.AppendShape(ShapeType.Triangle, new RectangleF(100, 100, 100, 100)); //set rotation to 180 shape.Rotation = 180; //set the color and fill style of shape shape.Fill.FillType = FillFormatType.Solid; shape.Fill.SolidColor.Color = Color.BlueViolet; shape.ShapeStyle.LineColor.Color = Color.Black; //save the document presentation.SaveToFile("shape.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("shape.pptx"); } } }
Imports Spire.Presentation Imports Spire.Presentation.Drawing Imports System.Drawing Namespace RotateShape Class Program Private Shared Sub Main(args As String()) 'create PPT document Dim presentation As New Presentation() 'append new shape - Triangle Dim shape As IAutoShape = presentation.Slides(0).Shapes.AppendShape(ShapeType.Triangle, New RectangleF(100, 100, 100, 100)) 'set rotation to 180 shape.Rotation = 180 'set the color and fill style of shape shape.Fill.FillType = FillFormatType.Solid shape.Fill.SolidColor.Color = Color.BlueViolet shape.ShapeStyle.LineColor.Color = Color.Black 'save the document presentation.SaveToFile("shape.pptx", FileFormat.Pptx2010) System.Diagnostics.Process.Start("shape.pptx") End Sub End Class End Namespace
This topic is just another request from one of our users on Spire.Doc Forum. In order to let more people know about this function, we’re going to present the whole procedure through a sample demo in the article. Additionally, we would like to remind you that we offer free customized demo for both pay users and test users.
As a professional .NET Word component, Spire.Doc enables developers to replace specified paragraph with a newly created table or an existing table. In this example, the paragraph 3 in main body of the sample word file will be replaced by a newly-built table.
Test file:
Code snippets for replacing text with table:
Step 1: Create a new word document and load the test file.
Document doc = new Document(); doc.LoadFromFile(@"..\..\test.docx");
Step 2: Return TextSection by finding the key text string "classical antiquity science".
Section section = doc.Sections[0]; TextSelection selection = doc.FindString("classical antiquity science", true, true);
Step 3: Return TextRange from TextSection, then get OwnerParagraph through TextRange.
TextRange range = selection.GetAsOneRange(); Paragraph paragraph = range.OwnerParagraph;
Step 4: Return the zero-based index of the specified paragraph.
Body body = paragraph.OwnerTextBody; int index = body.ChildObjects.IndexOf(paragraph);
Step 5: Create a new table.
Table table = section.AddTable(true); table.ResetCells(3, 3);
Step 6: Remove the paragraph and insert table into the collection at the specified index.
body.ChildObjects.Remove(paragraph); body.ChildObjects.Insert(index, table);
Step 7: Save and launch the file.
doc.SaveToFile("result.doc", FileFormat.Doc); System.Diagnostics.Process.Start("result.doc");
Result:
Full C# code:
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; namespace ReplaceText { class Program { static void Main(string[] args) { Document doc = new Document(); doc.LoadFromFile(@"..\..\test.docx"); Section section = doc.Sections[0]; TextSelection selection = doc.FindString("classical antiquity science", true, true); TextRange range = selection.GetAsOneRange(); Paragraph paragraph = range.OwnerParagraph; Body body = paragraph.OwnerTextBody; int index = body.ChildObjects.IndexOf(paragraph); Table table = section.AddTable(true); table.ResetCells(3, 3); body.ChildObjects.Remove(paragraph); body.ChildObjects.Insert(index, table); doc.SaveToFile("result.doc", FileFormat.Doc); System.Diagnostics.Process.Start("result.doc"); } } }
Word bookmarks are widely used for point out a specified location or give brief information of the paragraph. If you add an image into the bookmark position, the bookmarks will be more obviously and clearly. This article will show you how to insert an image at bookmark position in C# with the help of Spire.Doc.
Spire.Doc offers an instance of BookmarksNavigator to find the bookmarks, and then developers use AppendPicture to add an image. Here comes to the steps:
Step 1: Load a word documents with bookmarks.
Document document = new Document(); document.LoadFromFile("Test.docx");
Step 2: Create an instance of BookmarksNavigator and find the bookmark where you want to insert an image.
//Create an instance of BookmarksNavigator BookmarksNavigator bn = new BookmarksNavigator(document); //Find a bookmark and its name is Spire bn.MoveToBookmark("Spire", true, true);
Step 3: Insert an image at the position of bookmarks you found.
//Add a section and named it section0 Section section0 = document.AddSection(); //Add a paragraph for section0 Paragraph paragraph = section0.AddParagraph(); Image image = Image.FromFile("step.png"); //Add a picture into paragraph DocPicture picture = paragraph.AppendPicture(image); //Add a paragraph with picture at the position of bookmark bn.InsertParagraph(paragraph); document.Sections.Remove(section0);
Step 4: Save the new document and process it.
string output = "sample3.docx"; document.SaveToFile(output, FileFormat.Docx); System.Diagnostics.Process.Start(output);
Spire.Doc also offers the following properties to set the image position based on developers' requirements.
picture.TextWrappingStyle picture.HorizontalAlignment picture.HorizontalOrigin picture.HorizontalPosition picture.VerticalAlignment picture.VerticalOrigin picture.VerticalPosition
Effective screenshot:
Full codes:
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; using System.Drawing; namespace InsertImage { class Program { static void Main(string[] args) { Document document = new Document(); document.LoadFromFile("Test.docx"); BookmarksNavigator bn = new BookmarksNavigator(document); bn.MoveToBookmark("Spire", true, true); Section section0 = document.AddSection(); Paragraph paragraph = section0.AddParagraph(); Image image = Image.FromFile("step.png"); DocPicture picture = paragraph.AppendPicture(image); bn.InsertParagraph(paragraph); document.Sections.Remove(section0); string output = "sample.docx"; document.SaveToFile(output, FileFormat.Docx); System.Diagnostics.Process.Start(output); } } }
Bookmarks give convenience when users want go to specified location and it is clearly to know the contents brief information. Spire.Doc for .NET has a powerful function of operating the word elements of bookmarks. Developers can add bookmarks, Edit/replace bookmarks and remove bookmarks in word documents. Now Spire.Doc starts to support preserve bookmarks in DOCX to PDF conversion. This article will show you how to preserve bookmarks in C# when converting word document into PDF file format.
Download and install Spire.Doc for .NET (Version 5.2.20 or above) and then add Spire.Doc.dll as reference in the downloaded Bin folder though the below path: "..\Spire.Doc\Bin\NET4.0\ Spire.Doc.dll".
Here comes to the details of how to preserve the bookmarks from word to PDF conversion in C#.
Step 1: Load a word documents with bookmarks.
Document doc = new Document(); doc.LoadFromFile("test.docx", FileFormat.Docx);
Step 2: Create an instance of ToPdfParameterList
ToPdfParameterList toPdf = new ToPdfParameterList();
Step 3: Set CreateWordBookmarks to true to use word bookmarks when create the bookmarks.
toPdf.CreateWordBookmarks = true;
Step 4: Save the PDF file.
doc.SaveToFile("test.Pdf",toPdf);
Effective screenshot of preserve the bookmarks in result PDF page:
Full codes:
using Spire.Doc; namespace PreventBookmark { class Program { static void Main(string[] args) { Document doc = new Document(); doc.LoadFromFile("test.docx", FileFormat.Docx); ToPdfParameterList toPdf = new ToPdfParameterList(); toPdf.CreateWordBookmarks = true; doc.SaveToFile("test.Pdf", toPdf); System.Diagnostics.Process.Start("test.Pdf"); } } }
As is shown in the following presentation slide, shapes automatically stack in separate layers as you add them. To change the order of overlapping shapes, we can move the layers forward and backward. But how can we achieve this task programmatically using C# or VB.NET? This article is aimed to present you a solution on how to reorder overlapping shapes using Spire.Presentation.
In the early edition, Spire.Presentation is already capable of adding different kinds of shapes to the slides, setting color and fill style of the shape. In the Version2.0.18, we add reordering overlapping shapes as a new feature to satisfy our customer’s demands. Now, let’s see how to make it happen with sample code snippet.
Step 1: Create a new instance of Spire.Presentation class and load the test file.
Spire.Presentation.Presentation presentation = new Presentation(); presentation.LoadFromFile("..\\..\\test.pptx");
Step 2: Get the first shape from the slide.
IShape shape = presentation.Slides[0].Shapes[0];
Step 3: Change the shape's Zorder by setting its position index.
presentation.Slides[0].Shapes.ZOrder(1, shape);
Step 4: Save to a PPTX file and launch the file.
string output = "output.pptx"; presentation.SaveToFile(output,FileFormat.Pptx2010); System.Diagnostics.Process.Start(output);
Output:
Full code:
using Spire.Presentation; namespace ReorderOverlappingShape { class Program { static void Main(string[] args) { //Create an instance of Spire.Presentation Spire.Presentation.Presentation presentation = new Presentation(); //Load a pptx file presentation.LoadFromFile("..\\..\\test.pptx"); //Get the first shape of the first slide IShape shape = presentation.Slides[0].Shapes[0]; //Change the shape's zorder presentation.Slides[0].Shapes.ZOrder(1, shape); //Save to a pptx2010 file. string output = "output.pptx"; presentation.SaveToFile(output, FileFormat.Pptx2010); //Launch the output file System.Diagnostics.Process.Start(output); } } }
Imports Spire.Presentation Namespace ReorderOverlappingShape Class Program Private Shared Sub Main(args As String()) 'Create an instance of Spire.Presentation Dim presentation As Spire.Presentation.Presentation = New Presentation() 'Load a pptx file presentation.LoadFromFile("..\..\test.pptx") 'Get the first shape of the first slide Dim shape As IShape = presentation.Slides(0).Shapes(0) 'Change the shape's zorder presentation.Slides(0).Shapes.ZOrder(1, shape) 'Save to a pptx2010 file. Dim output As String = "output.pptx" presentation.SaveToFile(output, FileFormat.Pptx2010) 'Launch the output file System.Diagnostics.Process.Start(output) End Sub End Class End Namespace
Remove Encryption on Password-Protected PowerPoint File
2014-07-29 05:58:32 Written by support iceblueEverytime we try to open a password-protected PowerPoint file, we will then be prompted to enter the password. This can be really a bothering thing itself. But things get worse if we forget the password. To avoid this situation from being occurred, we can choose to remove the encryption if the PowerPoint file is not necessarily protected. In this article, I’ll introduce you how to remove encryption on password-protected PowerPoint file using Spire.Presentation.
In the classes of Spire.Presentation, you can invoke Presentation.LoadFromFile(string file, string password) method to load the file that you want to remove protection, then you're entitled to remove encryption by calling Presentation.RemoveEncryption() method. More details:
Step 1: Create Presentation instance and load file.
Presentation presentation = new Presentation(); presentation.LoadFromFile("Presentation1.pptx", "test");
Step 2: Remove encryption.
presentation.RemoveEncryption();
Step 3: Save and launch the file.
presentation.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx");
Effect Screenshot:
Full Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Presentation; namespace RemoveProtect { class Program { static void Main(string[] args) { // create Presentation instance and load file Presentation presentation = new Presentation(); presentation.LoadFromFile("Presentation1.pptx", "test"); //remove encryption presentation.RemoveEncryption(); //save the file presentation.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx"); } } }
Imports System Imports System.Collections.Generic Imports System.Linq Imports System.Text Imports Spire.Presentation Namespace RemoveProtect Class Program Shared Sub Main(ByVal args() As String) ' create Presentation instance and load file Dim presentation As Presentation = New Presentation() presentation.LoadFromFile("Presentation1.pptx", "test") 'remove encryption presentation.RemoveEncryption() 'save the file presentation.SaveToFile("result.pptx", FileFormat.Pptx2010) System.Diagnostics.Process.Start("result.pptx") End Sub End Class End Namespace
