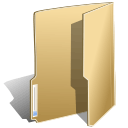
.NET (1273)
Children categories
When creating a PowerPoint presentation, you may want to ensure that some important content in your presentation grabs the audience’s attention. A great way to make the content more prominent and noticeable is to highlight it with a bright color. This article will demonstrate how to highlight text in a PowerPoint presentation in C# and VB.NET using Spire.Presentation for .NET.
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Highlight Text in PowerPoint in C# and VB.NET
The following are the steps to highlight specific text in a PowerPoint document:
- Initialize an instance of Presentation class.
- Load a PowerPoint presentation using Presentation.LoadFromFile() method.
- Loop through the slides in the presentation and the shapes on each slide.
- Check if the current shape is of IAutoShape type.
- If the result is true, typecast it to IAutoShape.
- Initialize an instance of TextHighLightingOptions class, and set the text highlighting options such as whole words only and case sensitive through TextHighLightingOptions.WholeWordsOnly and TextHighLightingOptions.CaseSensitive properties.
- Highlight a specific text in the shape using IAutoShape.TextFrame.HighLightText() method.
- Save the result file using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; using System.Drawing; namespace HighlightTextInPPT { class Program { static void Main(string[] args) { //Create an instance of Presentation class Presentation presentation = new Presentation(); //Load a PowerPoint file presentation.LoadFromFile(@"Sample1.pptx"); //Loop through all slides for (int i = 0; i < presentation.Slides.Count; i++) { //Get the current slide ISlide slide = presentation.Slides[i]; //Loop through the shapes on the slide for (int j = 0; j < slide.Shapes.Count; j++) { //Check if the current shape is of IAutoShape type if (slide.Shapes[j] is IAutoShape) { //Typecast the shape to IAutoShape IAutoShape shape = slide.Shapes[j] as IAutoShape; //Create an instance of TextHighLightingOptions class TextHighLightingOptions options = new TextHighLightingOptions(); //Set text highlighting options options.CaseSensitive = true; options.WholeWordsOnly = true; //Highlight specific text within the shape with color shape.TextFrame.HighLightText("Spire.Presentation", Color.LightYellow, options); } } } //Save the result file presentation.SaveToFile("HighlightText.pptx", FileFormat.Pptx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
How to Mannually Add Spire.PDF as Dependency in a .NET Standard Library Project
2019-10-30 03:43:03 Written by support iceblueStep 1: Download the latest version of Spire.PDF Pack from the link below, unzip it, and you'll get the DLL files for .NET Standarad from the "netstandard2.0" folder. If you already have this folder in your disk, go straight to step two.
Step 2: Create a .NET Standard library project in your Visual Studio.
Step 3: Add all DLL files under the "netstandard2.0" folder as dependencies in your project.
Right-click "Dependencies" – select "Add Reference" – click "Browse" – selcet all DLLs under "netstandard2.0" folder – click "Add".
Step 4: Install the other five packages in your project via the NuGet Package Manager. They are SkiaSharp, System.Buffers, System.Memory, System.Text.Encoding.CodePages and System.Runtime.CompilerServices.Unsafe.
Right-click "Dependencies" – select "Manage NuGet Packages" – click "Browse" –type the package name – select the package from the search results – click "Install".
Step 5: Now that you've added all the dependences successfully, you can start to write your own .NET Standard library that is capable of creating and processing PDF documents.
using Spire.Pdf; using Spire.Pdf.Graphics; using System.Drawing; namespace SpirePdfStandard { public class Class1 { public void CreatePdf() { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Add a page PdfPageBase page = doc.Pages.Add(); //Draw text on the page at the specified position page.Canvas.DrawString("Hello World", new PdfFont(PdfFontFamily.Helvetica, 13f), new PdfSolidBrush(Color.Black), new PointF(50, 50)); //Save the document doc.SaveToFile("Output.pdf"); } } }
Spire.Presentation supports setting alignment for table in a PowerPoint document. This article demonstrates how to align a table to the bottom of a PowerPoint slide using Spire.Presentation.
Below screenshot shows the original table before setting alignment:
using Spire.Presentation; namespace AlignTable { class Program { static void Main(string[] args) { //Load PowerPoint document Presentation ppt = new Presentation(); ppt.LoadFromFile("Table.pptx"); ITable table = null; //Loop through the shapes in the first slide foreach (IShape shape in ppt.Slides[0].Shapes) { //Find the table and align it to the bottom of the slide if (shape is ITable) { table = (ITable)shape; table.SetShapeAlignment(Spire.Presentation.ShapeAlignment.AlignBottom); } } //Save the resultant document ppt.SaveToFile("Result.pptx", FileFormat.Pptx2013); } } }
Output:
With Spire.PDF for .NET, developers can set page size for PDF in C#. This article will demonstrates how to get the PDF page size using Spire.PDF.
Detail steps:
Step 1: Create a PdfDocument instance and load the sample.pdf file.
PdfDocument doc = new PdfDocument(); doc.LoadFromFile("Sample.pdf");
Step 2: Get the width and height of the first page in the PDF file.
PdfPageBase page = doc.Pages[0]; float pointWidth = page.Size.Width; float pointHeight = page.Size.Height;
Step 3: Convert the size with other measurement unit, such as in Inch, Centimeter, Unit or Pixel.
//Create PdfUnitConvertor to convert the unit PdfUnitConvertor unitCvtr = new PdfUnitConvertor(); //Convert the size with "pixel" float pixelWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Pixel); float pixelHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Pixel); //Convert the size with "inch" float inchWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Inch); float inchHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Inch); //Convert the size with "centimeter" float centimeterWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Centimeter); float centimeterHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Centimeter);
Step 4: Save to a .txt file.
//Create StringBuilder to save StringBuilder content = new StringBuilder(); //Add pointSize string to StringBuilder content.AppendLine("The page size of the file is (width: " + pointWidth + "pt, height: " + pointHeight + "pt)."); content.AppendLine("The page size of the file is (width: " + pixelWidth + "pixel, height: " + pixelHeight + "pixel)."); content.AppendLine("The page size of the file is (width: " + inchWidth + "inch, height: " + inchHeight + "inch)."); content.AppendLine("The page size of the file is (width: " + centimeterWidth + "cm, height: " + centimeterHeight + "cm.)"); String output = "GetPageSize_out.txt"; //Save them to a txt file File.WriteAllText(output, content.ToString());
Output:
Full code:
using Spire.Pdf; using Spire.Pdf.Actions; using Spire.Pdf.General; using Spire.Pdf.Graphics; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.IO; using System.Linq; using System.Text; using System.Threading.Tasks; namespace GetPDFPageSize { class Program { static void Main(string[] args) { PdfDocument doc = new PdfDocument(); doc.LoadFromFile("Sample.pdf"); //Get the first page of the loaded PDF file PdfPageBase page = doc.Pages[0]; //Get the width of page based on "point" float pointWidth = page.Size.Width; //Get the height of page float pointHeight = page.Size.Height; //Create PdfUnitConvertor to convert the unit PdfUnitConvertor unitCvtr = new PdfUnitConvertor(); //Convert the size with "pixel" float pixelWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Pixel); float pixelHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Pixel); //Convert the size with "inch" float inchWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Inch); float inchHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Inch); //Convert the size with "centimeter" float centimeterWidth = unitCvtr.ConvertUnits(pointWidth, PdfGraphicsUnit.Point, PdfGraphicsUnit.Centimeter); float centimeterHeight = unitCvtr.ConvertUnits(pointHeight, PdfGraphicsUnit.Point, PdfGraphicsUnit.Centimeter); //Create StringBuilder to save StringBuilder content = new StringBuilder(); //Add pointSize string to StringBuilder content.AppendLine("The page size of the file is (width: " + pointWidth + "pt, height: " + pointHeight + "pt)."); content.AppendLine("The page size of the file is (width: " + pixelWidth + "pixel, height: " + pixelHeight + "pixel)."); content.AppendLine("The page size of the file is (width: " + inchWidth + "inch, height: " + inchHeight + "inch)."); content.AppendLine("The page size of the file is (width: " + centimeterWidth + "cm, height: " + centimeterHeight + "cm.)"); String output = "GetPageSize_out.txt"; //Save them to a txt file File.WriteAllText(output, content.ToString()); } } }
How to Mannually Add Spire.Doc as Dependency in a .NET Standard Library Project
2019-10-22 09:02:07 Written by support iceblueAs System.Drawing only supported on Windows in NET6.0, you need to use SkiaSharp instead under NET5.0 or NET6.0 in Linux, or inside docker container. Please follow the next steps to use the NetStandard dlls to get SkiaSharp to your project manually. We will use Spire.Doc as example.
Step 1: Download the latest version of Spire.Doc Pack from this link, unzip it, and you'll get the DLL files for .NET Standarad from the "netstandard2.0" folder or install via NuGet.
PM> Install-Package Spire.Officefor.NETStandard
If you already have this folder in your disk, go straight to step two.
Step 2: Create a .NET Standard library project in your Visual Studio.
Step 3: Add all DLL files under the "netstandard2.0" folder as dependencies in your project.
Right-click "Dependencies" – select "Add Reference" – click "Browse" – selcet all DLLs under "netstandard2.0" folder – click "Add".
Step 4: Install the other three packages in your project via the NuGet Package Manager. They are SkiaSharp, System.Text.Encoding.CodePages and System.Security.Cryptography.Xml.
Right-click "Dependencies" – select "Manage NuGet Packages" – click "Browse" – type the package name – select the package from the search results – click "Install".
Step 5: Now that you've added all the dependences successfully, you can start to write your own .NET Standard library that is capable of creating and processing Word documents.
using Spire.Doc; using Spire.Doc.Documents; namespace SpireDocStandard { public class Class1 { public void CreateWord() { //Create a document object Document doc = new Document(); //Add a section Section section = doc.AddSection(); //Add a paragrah Paragraph paragraph = section.AddParagraph(); //Append text to the paragraph paragraph.AppendText("Hello World"); //Save to file doc.SaveToFile("Output.docx", FileFormat.Docx2013); } } }
How to Mannually Add Spire.PDF as Dependency in a .NET Core Application
2019-10-17 07:44:00 Written by support iceblueStep 1: Download the latest version of Spire.PDF Pack from the link below, unzip it, and you'll get the DLL files for .NET Core in the "netcoreapp2.0" folder. If you already have this folder in your disk, go straight to step two.
Step 2: Create a .NET Core application in your Visual Studio.
Step 3: Add all DLL files under the "netcoreapp2.0" folder as dependencies in your project.
Right-click "Dependencies" – select "Add Reference" – click "Browse" – selcet all DLLs under "netcoreapp2.0" folder – click "Add".
Step 4: Install the other two packages in your project via the NuGet Package Manager. They are System.Drawing.Common and System.Text.Encoding.CodePages.
Right-click "Dependencies" – select "Manage NuGet Packages" – click "Browse" –type the package name – select the package from the search results – click "Install".
Step 5: Now that you’ve added all the dependences successfully, you can start to code. The following code snippet gives you an exmaple of how to create a simple PDF document using Spire.PDF.
using Spire.Pdf; using Spire.Pdf.Graphics; using System.Drawing; namespace SpirePdfCore { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Add a page PdfPageBase page = doc.Pages.Add(); //Draw text on the page at the specified position page.Canvas.DrawString("Hello World", new PdfFont(PdfFontFamily.Helvetica, 13f), new PdfSolidBrush(Color.Black), new PointF(50, 50)); //Save the document doc.SaveToFile("Output.pdf"); } } }
How to Mannually Add Spire.Doc as Dependency in a .NET Core Application
2019-10-16 02:56:57 Written by support iceblueStep 1: Download the latest version of Spire.Doc Pack from the link below, unzip it, and you'll get the DLL files for .NET Core in the “netcoreapp2.0” folder. If you already have this folder in your disk, go straight to step two.
Step 2: Create a .Net Core application in your Visual Studio.
Step 3: Add all DLL files under the "netcoreapp2.0" folder as dependencies in your project.
Right-click "Dependencies" – select "Add Reference" – click "Browse" – selcet all DLLs under "netcoreapp2.0" folder – click "Add".
Step 4: Install the other three packages in your project via the NuGet Package Manager. They are System.Drawing.Common, System.Text.Encoding.CodePages and System.Security.Cryptography.Xml.
Right-click "Dependencies" – select "Manage NuGet Packages" – click "Browse" –type the package name – select the package from the search results – click "Install".
Step 5: Now that you've added all the dependences successfully, you can start to code. The following code snippet gives you an exmaple of how to create a simple Word document using Spire.Doc.
using Spire.Doc; using Spire.Doc.Documents; namespace SpireDocNetCore { class Program { static void Main(string[] args) { //Create a document object Document doc = new Document(); //Add a section Section section = doc.AddSection(); //Add a paragrah Paragraph paragraph = section.AddParagraph(); //Append text to the paragraph paragraph.AppendText("This article shows you how to mannually add Spire.Doc as dependency in a .NET Core application."); //Save to file doc.SaveToFile("Output.docx", FileFormat.Docx2013); } } }
When generating a QR code, you might want to add a custom image such as your company’s logo or your personal profile image to it. In this article, you will learn how to achieve this task programmatically in C# and VB.NET using Spire.Barcode for .NET library.
Install Spire.Barcode for .NET
To begin with, you need to add the DLL files included in the Spire.Barcode for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Barcode
Note: This feature relies on a commercial license. If you want to test it, please go to the end of this article to request a temporary license.
Generate QR Code with a Logo Image in C# and VB.NET
The following are the steps to generate a QR code with a logo image:
- Create a BarcodeSettings object.
- Set barcode type, error correction level and data etc. using BarcodeSettings.Type, BarcodeSettings.QRCodeECL and BarcodeSetting.Data properties.
- Set logo image using BarcodeSettings.QRCodeLogoImage property.
- Create a BarCodeGenerator object based on the settings.
- Generate QR code image using BarCodeGenerator.GenerateImage() method.
- Save the image using Image.Save() method.
- C#
- VB.NET
using Spire.Barcode; using Spire.License; using System.Drawing; namespace AddLogoToQR { class Program { static void Main(string[] args) { //Load license Spire.License.LicenseProvider.SetLicenseFileFullPath("license.elic.xml"); //Create a BarcodeSettings object BarcodeSettings settings = new BarcodeSettings(); //Set barcode type, error correction level, data, etc. settings.Type = BarCodeType.QRCode; settings.QRCodeECL = QRCodeECL.M; settings.ShowText = false; settings.X = 2.5f; string data = "www.e-iceblue.com"; settings.Data = data; settings.Data2D = data; //Set logo image settings.QRCodeLogoImage = Image.FromFile(@"C:\Users\Administrator\Desktop\logo.png"); //Generate QR image based on the settings BarCodeGenerator generator = new BarCodeGenerator(settings); Image image = generator.GenerateImage(); image.Save("QR.png", System.Drawing.Imaging.ImageFormat.Png); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
People often need to replace text in a PDF document for a variety of reasons. It could be to correct errors or typos, update outdated information, customize the content for a specific audience or purpose, or comply with legal or regulatory requirements. By replacing text in a PDF, individuals can ensure accuracy, maintain document integrity, and enhance the overall quality and relevance of the information presented.
In this article, you will learn how to replace text in a PDF document in C# by using the Spire.PDF for .NET library.
- Replace Text in a Specific PDF Page in C#
- Replace Text in an Entire PDF Document in C#
- Replace the First Occurrence of the Target Text in C#
- Replace Text Based on a Regular Expression in C#
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.PDF
Replace Text in a Specific PDF Page in C#
Spire.PDF for .NET offers the PdfTextReplacer.ReplaceAllText() method, allowing users to replace all occurrences of target text in a page with new text. The following are the steps to replace text in a specific page using C#.
- Create a PdfDocument object.
- Load a PDF file for a specified path.
- Get a specific page from the document.
- Create a PdfTextReplaceOptions object, and specify the replace options using ReplaceType property of the object.
- Create a PdfTextReplacer object, and apply the replace options using Options property of it.
- Replace all occurrences of the target text in the page with new text using PdfTextReplacer.ReplaceAllText() method.
- Save the document to a different PDF file.
- C#
using Spire.Pdf; using Spire.Pdf.Texts; namespace ReplaceTextInPage { class Program { static void Main(string[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Create a PdfTextReplaceOptions object PdfTextReplaceOptions textReplaceOptions = new PdfTextReplaceOptions(); // Specify the options for text replacement textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.IgnoreCase; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.WholeWord; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.AutofitWidth; // Get a specific page PdfPageBase page = doc.Pages[0]; // Create a PdfTextReplacer object based on the page PdfTextReplacer textReplacer = new PdfTextReplacer(page); // Set the replace options textReplacer.Options = textReplaceOptions; // Replace all occurrences of target text with new text textReplacer.ReplaceAllText(".NET Framework", "New Content"); // Save the document to a different PDF file doc.SaveToFile("ReplaceTextInPage.pdf"); // Dispose resources doc.Dispose(); } } }
Replace Text in an Entire PDF Document in C#
In order to replace all occurrences of target text in the entire document with new text, you need to iterate through pages in the document and replace text on each page using the PdfTextReplacer.ReplaceAllText() method.
The following are the steps to replace text in an entire PDF document using C#.
- Create a PdfDocument object.
- Load a PDF file for a specified path.
- Create a PdfTextReplaceOptions object, and specify the replace options using ReplaceType property of the object.
- Iterate through the pages in the document.
- Create a PdfTextReplacer object based on a specified page, and apply the replace options using Options property of it.
- Replace all occurrences of the target text in the page with new text using PdfTextReplacer.ReplaceAllText() method.
- Save the document to a different PDF file.
- C#
using Spire.Pdf; using Spire.Pdf.Texts; namespace ReplaceInEntireDocument { class Program { static void Main(string[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Create a PdfTextReplaceOptions object PdfTextReplaceOptions textReplaceOptions = new PdfTextReplaceOptions(); // Specify the options for text replacement textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.IgnoreCase; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.WholeWord; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.AutofitWidth; for (int i = 0; i < doc.Pages.Count; i++) { // Get a specific page PdfPageBase page = doc.Pages[i]; // Create a PdfTextReplacer object based on the page PdfTextReplacer textReplacer = new PdfTextReplacer(page); // Set the replace options textReplacer.Options = textReplaceOptions; // Replace all occurrences of target text with new text textReplacer.ReplaceAllText(".NET Framework", "New Content"); } // Save the document to a different PDF file doc.SaveToFile("ReplaceTextInDocument.pdf"); // Dispose resources doc.Dispose(); } } }
Replace the First Occurrence of the Target Text in C#
Instead of replacing all text on a page, you can only replace the first occurrence of the target text by utilizing the ReplaceText() method of the PdfTextReplacer class.
The following are the steps to replace the first occurrence of the target text using C#.
- Create a PdfDocument object.
- Load a PDF file for a specified path.
- Get a specific page from the document.
- Create a PdfTextReplaceOptions object, and specify the replace options using ReplaceType property of the object.
- Create a PdfTextReplacer object, and apply the replace options using Options property of it.
- Replace the first occurrence of the target text in the page with new text using PdfTextReplacer.ReplaceText() method.
- Save the document to a different PDF file.
- C#
using Spire.Pdf; using Spire.Pdf.Texts; namespace ReplaceFirstOccurance { class Program { static void Main(string[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Create a PdfTextReplaceOptions object PdfTextReplaceOptions textReplaceOptions = new PdfTextReplaceOptions(); // Specify the options for text replacement textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.IgnoreCase; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.WholeWord; textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.AutofitWidth; // Get a specific page PdfPageBase page = doc.Pages[1]; // Create a PdfTextReplacer object based on the page PdfTextReplacer textReplacer = new PdfTextReplacer(page); // Set the replace options textReplacer.Options = textReplaceOptions; // Replace the first occurrence of target text with new text textReplacer.ReplaceText(".NET Framework", "New Content"); // Save the document to a different PDF file doc.SaveToFile("ReplaceFirstOccurance.pdf"); // Dispose resources doc.Dispose(); } } }
Replace Text Based on a Regular Expression in C#
Regular expressions are powerful and versatile patterns used for matching and manipulating text. With Spire.PDF, you utilize regular expressions to search for specific text patterns in a PDF and replace them with new strings.
The steps to replace text in PDF based on a regular expression are as follows.
- Create a PdfDocument object.
- Load a PDF file for a specified path.
- Get a specific page from the document.
- Create a PdfTextReplaceOptions object.
- Specify the replace type as Regex using PdfTextReplaceOptions.ReplaceType property.
- Create a PdfTextReplacer object, and apply the replace options using Options property of it.
- Find and replace the text that matches a specified regular expression using PdfTextReplacer.ReplaceAllText() method.
- Save the document to a different PDF file.
- C#
using Spire.Pdf; using Spire.Pdf.Texts; namespace ReplaceUsingRegularExpression { class Program { static void Main(string[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Create a PdfTextReplaceOptions object PdfTextReplaceOptions textReplaceOptions = new PdfTextReplaceOptions(); // Set the replace type as Regex textReplaceOptions.ReplaceType = PdfTextReplaceOptions.ReplaceActionType.Regex; // Get a specific page PdfPageBase page = doc.Pages[1]; // Create a PdfTextReplacer object based on the page PdfTextReplacer textReplacer = new PdfTextReplacer(page); // Set the replace options textReplacer.Options = textReplaceOptions; // Specify the regular expression string regularExpression = @"\bC\w*?R\b"; // Replace all occurrences that match the regular expression with new text textReplacer.ReplaceAllText(regularExpression, "NEW"); // Save the document to a different PDF file doc.SaveToFile("ReplaceWithRegularExpression.pdf"); // Dispose resources doc.Dispose(); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
This article demonstrates how to add tooltip to the text on an existing PDF document in C#. Spire.PDF for .NET supports to create tooltips by adding invisible button over the searched text from the PDF file.
Step 1: Load the sample document file.
PdfDocument doc = new PdfDocument(); doc.LoadFromFile("Sample.pdf");
Step 2: Searched the text “Add tooltip to PDF” from the first page of the sample document and get the position of it.
PdfPageBase page = doc.Pages[0]; PdfTextFind[] result = page.FindText("Add tooltip to PDF").Finds; RectangleF rec = result[0].Bounds;
Step 3: Create invisible button on text position
PdfButtonField field1 = new PdfButtonField(page, "field1"); field1.Bounds = rec;
Step 4: Set the content and format for the tooltip field.
field1.ToolTip = "E-iceblue Co. Ltd., a vendor of .NET, Java and WPF development components"; field1.BorderWidth = 0; field1.BackColor = Color.Transparent; field1.ForeColor = Color.Transparent; field1.LayoutMode = PdfButtonLayoutMode.IconOnly; field1.IconLayout.IsFitBounds = true;
Step 5: Save the document to file.
doc.SaveToFile("Addtooltip.pdf", FileFormat.PDF);
Effective screenshot after adding the tooltip to PDF:
using Spire.Pdf; using Spire.Pdf.Fields; using Spire.Pdf.General.Find; using System.Drawing; namespace TooltipPDF { class Program { static void Main(string[] args) { PdfDocument doc = new PdfDocument(); doc.LoadFromFile("Sample.pdf"); PdfPageBase page = doc.Pages[0]; PdfTextFind[] result = page.FindText("Add tooltip to PDF").Finds; RectangleF rec = result[0].Bounds; PdfButtonField field1 = new PdfButtonField(page, "field1"); field1.Bounds = rec; field1.ToolTip = "E-iceblue Co. Ltd., a vendor of .NET, Java and WPF development components"; field1.BorderWidth = 0; field1.BackColor = Color.Transparent; field1.ForeColor = Color.Transparent; field1.LayoutMode = PdfButtonLayoutMode.IconOnly; field1.IconLayout.IsFitBounds = true; doc.SaveToFile("Addtooltip.pdf", FileFormat.PDF); } } }
