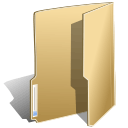
.NET (1273)
Children categories
In the earlier tutorial, we have given a brief introduction on how to insert Textbox in Word and this article will demonstrate how to position the text vertically in a text box using Spire.Doc for .NET.
using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; using System; namespace WordTextbox { class Program { static void Main(string[] args) { // Instantiate document object Document document = new Document(); //Add a section Section section = document.AddSection(); //Set the margin section.PageSetup.Margins.Left = 90; section.PageSetup.Margins.Right = 90; Paragraph paragraph = section.AddParagraph(); //Add texbox 1 TextBox textBox1 = paragraph.AppendTextBox(section.PageSetup.Margins.Left - 20, section.PageSetup.PageSize.Height + 20); //Fix the position of textbox textBox1.Format.HorizontalOrigin = HorizontalOrigin.Page; textBox1.Format.HorizontalPosition = 0; textBox1.Format.VerticalPosition = -10f; textBox1.Format.VerticalOrigin = VerticalOrigin.Page; //Set the text vertically textBox1.Format.TextAnchor = ShapeVerticalAlignment.Center; textBox1.Format.LayoutFlowAlt = TextDirection.LeftToRight; //Add text and set the font Paragraph textboxPara1 = textBox1.Body.AddParagraph(); TextRange txtrg = textboxPara1.AppendText("Name_______Number_________Class__________"); txtrg.CharacterFormat.FontName = "Arial"; txtrg.CharacterFormat.FontSize = 10; txtrg.CharacterFormat.TextColor = System.Drawing.Color.Black; textboxPara1.Format.HorizontalAlignment = HorizontalAlignment.Center; //Save the document document.SaveToFile("Result.docx"); } } }
Namespace WordTextbox Class Program Private Shared Sub Main(ByVal args() As String) Dim document As Document = New Document Dim section As Section = document.AddSection section.PageSetup.Margins.Left = 90 section.PageSetup.Margins.Right = 90 Dim paragraph As Paragraph = section.AddParagraph Dim textBox1 As TextBox = paragraph.AppendTextBox((section.PageSetup.Margins.Left - 20), (section.PageSetup.PageSize.Height + 20)) textBox1.Format.HorizontalOrigin = HorizontalOrigin.Page textBox1.Format.HorizontalPosition = 0 textBox1.Format.VerticalPosition = -10! textBox1.Format.VerticalOrigin = VerticalOrigin.Page textBox1.Format.TextAnchor = ShapeVerticalAlignment.Center textBox1.Format.LayoutFlowAlt = TextDirection.LeftToRight Dim textboxPara1 As Paragraph = textBox1.Body.AddParagraph Dim txtrg As TextRange = textboxPara1.AppendText("Name_______Number_________Class__________") txtrg.CharacterFormat.FontName= "Arial" txtrg.CharacterFormat.FontSize = 10 txtrg.CharacterFormat.TextColor = System.Drawing.Color.Black textboxPara1.Format.HorizontalAlignment = HorizontalAlignment.Center document.SaveToFile("Result.docx") End Sub End Class End Namespace
Output
How to use Spire.OCR for .NET in .NET Core Applications
2021-06-17 05:49:10 Written by support iceblueThis article demonstrates the steps to use Spire.OCR for .NET in .NET Core applications.
Step 1: Create a .NET Core project in Visual Studio.
Step 2: Add reference to Spire.OCR for .NET DLLs in your project.
You can add reference to Spire.OCR for .NET DLLs through one of the following two ways:
1. Install Spire.OCR for .NET through NuGet using NuGet Package Manager (recommended):
- In Solution Explorer, right-click the project or "Dependencies" and select "Manage NuGet Packages".
- Click "Browse" tab and search Spire.OCR.
- Install Spire.OCR.
2. Manually add reference to Spire.OCR for .NET DLLs.
- Download Spire.OCR for .NET package from the following link, unzip it, you will get the DLLs from the "netstandard2.0" folder.
- Right-click the project or "Dependencies" – select "Add Reference" – click "Browse" – select all DLLs under "netstandard2.0" folder – click "Add".
- Install the other two packages: SkiaSharp and System.Text.Encoding.CodePages in your project via the NuGet Package Manager.
Right-click the project or "Dependencies" – select "Manage NuGet Packages" – click "Browse" – type the package name – select the package from the search results – click "Install".
Step 3: Copy dependency DLLs to running directory of your project.
If you install Spire.OCR from NuGet and your project's target framework is .NET Core 3.0 or above, please build the project, then copy the 6 DLLs from bin\Debug\netcoreapp3.0\runtimes\win-x64\native folder to the running directory such as bin\Debug\netcoreapp3.0 or C:\Windows\System32 .
If your project's target framework is below .NET Core 3.0 or you download Spire.OCR from our website, please copy the 6 DLLs from Spire.OCR\Spire.OCR_Dependency\x64 folder to the running directory such as bin\Debug\netcoreapp2.1 or C:\Windows\System32.
Step 4: Now you have successfully included Spire.OCR in your project. You can refer the following code example to scan images using Spire.OCR.
using Spire.OCR; using System.IO; namespace SpireOCR { class Program { static void Main(string[] args) { OcrScanner scanner = new OcrScanner(); scanner.Scan("image.png"); File.WriteAllText("output.txt", scanner.Text.ToString()); } } }
Imports Spire.OCR Imports System.IO Namespace SpireOCR Class Program Private Shared Sub Main(ByVal args As String()) Dim scanner As OcrScanner = New OcrScanner() scanner.Scan("image.png") File.WriteAllText("output.txt", scanner.Text.ToString()) End Sub End Class End Namespace
It is possible to perform Word to PDF conversion in Azure apps such as Azure Web apps and Azure Functions apps using Spire.Doc for .NET. In this article, you can see the code example to achieve this function with Spire.Doc for .NET.
The input Word document:
Step 1: Install Spire.Doc NuGet Package as a reference to your project from NuGet.org.
Step 2: Add the following code to convert Word to PDF.
//Create a Document instance Document document = new Document(false);
//Load the Word document document.LoadFromFile(@"sample.docx"); //Create a ToPdfParameterList instance ToPdfParameterList ps = new ToPdfParameterList { UsePSCoversion = true }; //Save Word document to PDF using PS conversion document.SaveToFile("ToPdf.pdf", ps);
Private Sub SurroundingSub() Dim document As Document = New Document(false)
document.LoadFromFile("sample.docx") Dim ps As ToPdfParameterList = New ToPdfParameterList With { .UsePSCoversion = True } document.SaveToFile("ToPdf.pdf", ps) End Sub
The Output PDF document:
When merging datasets from different sources or copying data from other worksheets, duplicate rows may appear if the data are not properly matched. These duplicate rows may distort data analysis and calculations, leading to incorrect results. Therefore, removing duplicate rows is a frequently needed task, and this article demonstrates how to accomplish this task programmatically using Spire.XLS for .NET.
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.XLS
Remove Duplicate Rows in Excel in C# and VB.NET
Removing duplicate rows manually is a very repetitive and time-consuming task. With Spire.XLS for .NET, you can identify and remove all duplicate rows at once. The following are the detailed steps.
- Create a Workbook instance.
- Load a sample Excel document using Workbook.LoadFromFile() method.
- Get a specified worksheet by its index using Workbook.Worksheets[sheetIndex] property.
- Specify the cell range where duplicate records need to be deleted using Worksheet.Range property.
- Get the rows that contain duplicate content in the specified cell range.
- Loop through all duplicated rows and delete them using Worksheet.DeleteRow() method.
- Save the result document using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; using System.Linq; namespace RemoveDuplicateRows { class Program { static void Main(string[] args) { //Create a Workbook instance Workbook workbook = new Workbook(); //Load a sample Excel document workbook.LoadFromFile("Test.xlsx"); //Get the first worksheet Worksheet sheet = workbook.Worksheets[0]; //Specify the cell range where duplicate records need to be deleted var range = sheet.Range["A1:A" + sheet.LastRow]; //Get the duplicate row numbers var duplicatedRows = range.Rows .GroupBy(x => x.Columns[0].DisplayedText) .Where(x => x.Count() > 1) .SelectMany(x => x.Skip(1)) .Select(x => x.Columns[0].Row) .ToList(); //Remove the duplicate rows for (int i = 0; i < duplicatedRows.Count; i++) { sheet.DeleteRow(duplicatedRows[i] - i); } //Save the result document workbook.SaveToFile("RemoveDuplicateRows.xlsx"); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
C#/VB.NET: Add or Remove Digital Signatures in PowerPoint
2023-02-02 07:28:00 Written by support iceblueA digital signature is a modern alternative to signing documents manually on paper with pen. It uses an advanced mathematical technique to check the authenticity and integrity of digital documents, which guarantees that the contents in a digital document comes from the signer and has not been altered since then. Sometimes PowerPoint documents that contain confidential information may require a signature. In this article, you will learn how to programmatically add or remove digital signatures in PowerPoint using Spire.Presentation for .NET.
- Add a Digital Signature to PowerPoint in C# and VB.NET
- Remove All Digital Signatures from PowerPoint in C# and VB.NET
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Add a Digital Signature to PowerPoint in C# and VB.NET
To add a digital signature, you'll need to have a valid signature certificate first. Then you can digitally sign a PowerPoint document with the certificate using Presentation.AddDigitalSignature (X509Certificate2 certificate, string comments, DateTime signTime) method. The detailed steps are as follows.
- Create a Presentation instance.
- Load a sample PowerPoint document using Presentation.LoadFromFile() method.
- Initializes an instance of X509Certificate2 class with the certificate file name and password.
- Add a digital signature to the PowerPoint document using Presentation.AddDigitalSignature (X509Certificate2 certificate, string comments, DateTime signTime) method.
- Save result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; using System; using System.Security.Cryptography.X509Certificates; namespace AddDigitalSignature { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile("input.pptx"); //Load the certificate X509Certificate2 x509 = new X509Certificate2("gary.pfx", "e-iceblue"); //Add a digital signature ppt.AddDigitalSignature(x509, "e-iceblue", DateTime.Now); //Save the result document ppt.SaveToFile("AddDigitalSignature.pptx", FileFormat.Pptx2013); } } }
Remove All Digital Signatures from PowerPoint in C# and VB.NET
At some point you may need to remove the digital signatures from a PowerPoint document. Spire.Presentation for .NET provides the Presentation.RemoveAllDigitalSignatures() method to remove all digital signatures at once. The detailed steps are as follows:
- Create a Presentation instance.
- Load a sample PowerPoint document using Presentation.LoadFromFile() method.
- Determine if the document contains digital signatures using Presentation.IsDigitallySigned property.
- Remove all digital signatures from the document using Presentation.RemoveAllDigitalSignatures() method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace RemoveDigitalSignature { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile("AddDigitalSignature.pptx"); //Detect if the document is digitally signed if (ppt.IsDigitallySigned == true) { //Remove all digital signatures ppt.RemoveAllDigitalSignatures(); } //Save the result document ppt.SaveToFile("RemoveDigitalSignature.pptx", FileFormat.Pptx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
This article shows you how to download a PDF document from an URL using Spire.PDF with C# and VB.NET.
using System.IO; using System.Net; using Spire.Pdf; namespace DownloadPdfFromUrl { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Create a WebClient object WebClient webClient = new WebClient(); //Download data from URL and save as memory stream using (MemoryStream ms = new MemoryStream(webClient.DownloadData("https://www.e-iceblue.com/article/toDownload.pdf"))) { //Load the stream doc.LoadFromStream(ms); } //Save to PDF file doc.SaveToFile("result.pdf", FileFormat.PDF); } } }
Imports System.IO Imports System.Net Imports Spire.Pdf Namespace DownloadPdfFromUrl Class Program Shared Sub Main(ByVal args() As String) 'Create a PdfDocument object Dim doc As PdfDocument = New PdfDocument() 'Create a WebClient object Dim webClient As WebClient = New WebClient() 'Download data from URL and save as memory stream Imports(MemoryStream ms = New MemoryStream(webClient.DownloadData("https:'www.e-iceblue.com/article/toDownload.pdf"))) { 'Load the stream doc.LoadFromStream(ms) } 'Save to PDF file doc.SaveToFile("result.pdf", FileFormat.PDF) End Sub End Class End Namespace
This article shows you how to create a hyperlink to a bookmark within the same Word document by using Spire.Doc with C# and VB.NET.
using Spire.Doc; using Spire.Doc.Documents; namespace LinkToBookmark { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Add two sections Section section1 = doc.AddSection(); Section section2 = doc.AddSection(); //Insert a paragraph in section 2 and add a bookmark named "myBookmark" to it Paragraph bookmarkParagrapg = section2.AddParagraph(); bookmarkParagrapg.AppendText("Here is a bookmark"); BookmarkStart start = bookmarkParagrapg.AppendBookmarkStart("myBookmark"); bookmarkParagrapg.Items.Insert(0, start); bookmarkParagrapg.AppendBookmarkEnd("myBookmark"); //Link to the bookmark Paragraph paragraph = section1.AddParagraph(); paragraph.AppendText("Link to a bookmark: "); paragraph.AppendHyperlink("myBookmark", "Jump to a location in this document", HyperlinkType.Bookmark); //Save to file doc.SaveToFile("LinkToBookmark.docx", FileFormat.Docx2013); } } }
Imports Spire.Doc Imports Spire.Doc.Documents Namespace LinkToBookmark Class Program Shared Sub Main(ByVal args() As String) 'Create a Document object Document doc = New Document() 'Add two sections Dim section1 As Section = doc.AddSection() Dim section2 As Section = doc.AddSection() 'Insert a paragraph in section 2 and add a bookmark named "myBookmark" to it Dim bookmarkParagrapg As Paragraph = section2.AddParagraph() bookmarkParagrapg.AppendText("Here is a bookmark") Dim start As BookmarkStart = bookmarkParagrapg.AppendBookmarkStart("myBookmark") bookmarkParagrapg.Items.Insert(0, start) bookmarkParagrapg.AppendBookmarkEnd("myBookmark") 'Link to the bookmark Dim paragraph As Paragraph = section1.AddParagraph() paragraph.AppendText("Link to a bookmark: ") paragraph.AppendHyperlink("myBookmark", "Jump to a location in this document", HyperlinkType.Bookmark) 'Save to file doc.SaveToFile("LinkToBookmark.docx", FileFormat.Docx2013) End Sub End Class End Namespace
Spire.Presentation supports to insert text watermark and image watermark to PowerPoint document. This article will show you how to use Spire.Presentation to add multiple watermarks to the presentation slides in C#/VB.NET.
using Spire.Presentation; using Spire.Presentation.Drawing; using System; using System.Drawing; using System.Windows.Forms; namespace WatermarkDemo { class Program { static void Main(string[] args) { //Create a PPT document and load file Presentation presentation = new Presentation(); presentation.LoadFromFile("Sample.pptx"); //Get the size of the watermark string Font font = new Font("Arial", 20); String watermarkText = "E-iceblue"; SizeF size = TextRenderer.MeasureText("E-iceblue", font); float x = 30; float y = 80; for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { //Define a rectangle range RectangleF rect = new RectangleF(x, y, size.Width, size.Height); //Add a rectangle shape with a defined range IAutoShape shape = presentation.Slides[0].Shapes.AppendShape(Spire.Presentation.ShapeType.Rectangle, rect); //Set the style of the shape shape.Fill.FillType = FillFormatType.None; shape.ShapeStyle.LineColor.Color = Color.White; shape.Rotation = -45; shape.Locking.SelectionProtection = true; shape.Line.FillType = FillFormatType.None; //Add text to the shape shape.TextFrame.Text = watermarkText; TextRange textRange = shape.TextFrame.TextRange; //Set the style of the text range textRange.Fill.FillType = FillFormatType.Solid; textRange.Fill.SolidColor.Color = Color.FromArgb(120, Color.HotPink); textRange.EastAsianFont = new TextFont(font.Name); textRange.FontHeight = font.Size; x += (100 + size.Width); } x = 30; y += (100 + size.Height); } //Save the document presentation.SaveToFile("Watermark_result.pptx", FileFormat.Pptx2010); } } }
Imports Spire.Presentation Imports Spire.Presentation.Drawing Imports System Imports System.Drawing Imports System.Windows.Forms Namespace WatermarkDemo Class Program Private Shared Sub Main(ByVal args() As String) 'Create a PPT document and load file Dim presentation As Presentation = New Presentation presentation.LoadFromFile("Sample.pptx") 'Get the size of the watermark string Dim font As Font = New Font("Arial", 20) Dim watermarkText As String = "E-iceblue" Dim size As SizeF = TextRenderer.MeasureText("E-iceblue", font) Dim x As Single = 30 Dim y As Single = 80 Dim i As Integer = 0 Do While (i < 3) Dim j As Integer = 0 Do While (j < 3) 'Define a rectangle range Dim rect As RectangleF = New RectangleF(x, y, size.Width, size.Height) 'Add a rectangle shape with a defined range Dim shape As IAutoShape = presentation.Slides(0).Shapes.AppendShape(Spire.Presentation.ShapeType.Rectangle, rect) 'Set the style of the shape shape.Fill.FillType = FillFormatType.None shape.ShapeStyle.LineColor.Color = Color.White shape.Rotation = -45 shape.Locking.SelectionProtection = true shape.Line.FillType = FillFormatType.None 'Add text to the shape shape.TextFrame.Text = watermarkText Dim textRange As TextRange = shape.TextFrame.TextRange 'Set the style of the text range textRange.Fill.FillType = FillFormatType.Solid textRange.Fill.SolidColor.Color = Color.FromArgb(120, Color.HotPink) textRange.EastAsianFont = New TextFont(font.Name) textRange.FontHeight = font.Size x = (x + (100 + size.Width)) j = (j + 1) Loop x = 30 y = (y + (100 + size.Height)) i = (i + 1) Loop 'Save the document presentation.SaveToFile("Watermark_result.pptx", FileFormat.Pptx2010) End Sub End Class End Namespace
Output:
Detect if the PDF file is password protected in C#/VB.NET
2021-02-07 03:47:35 Written by support iceblueThis article demonstrates how to detect whether a PDF file is encrypted or not by using Spire.PDF for .NET. Spire.PDF offers a method named IsPasswordProtected(string fileName) which returns a boolean value. If the value is true, means the PDF is encrypted with password, otherwise it's not.
using Spire.Pdf; using System; namespace PdfDemo { class Program { static void Main(string[] args) { string fileName = "Sample.pdf"; bool value = PdfDocument.IsPasswordProtected(fileName); Console.WriteLine(value); Console.ReadKey(); } } }
Imports Spire.Pdf Imports System Namespace PdfDemo Class Program Private Shared Sub Main(ByVal args() As String) Dim fileName As String = "Sample.pdf" Dim value As Boolean = PdfDocument.IsPasswordProtected(fileName) Console.WriteLine(value) Console.ReadKey End Sub End Class End Namespace
After running the project, we get the Output that shows the PDF file is password protected:
This article demonstrates how to add line numbers before chunks of text in a PDF page by using Spire.PDF for .NET.
Below is a screenshot of the input document.
using Spire.Pdf; using Spire.Pdf.General.Find; using Spire.Pdf.Graphics; using System.Drawing; namespace AddLineNumber { class Program { static void Main(string[] args) { //Create a PdfDocument of instance PdfDocument doc = new PdfDocument(); //Load a PDF document doc.LoadFromFile(@"G:\360MoveData\Users\Administrator\Desktop\sample.pdf"); //Get the first page PdfPageBase page = doc.Pages[0]; //Find the spcific text in the fisrt line PdfTextFind topLine = page.FindText("C# (pronounced See Sharp)", TextFindParameter.None).Finds[0]; //Get the line height float lineHeight = topLine.Bounds.Height; //Get the Y coordinate of the selected text float y = topLine.Bounds.Y; //Find the spcific text in the second line PdfTextFind secondLine = page.FindText("language. C#", TextFindParameter.None).Finds[0]; //Calculate the line spacing float lineSpacing = secondLine.Bounds.Top - topLine.Bounds.Bottom; //Find the specific text in the last line PdfTextFind bottomLine = page.FindText("allocation of objects", TextFindParameter.None).Finds[0]; //Get the height of the chunks float height = bottomLine.Bounds.Bottom; //Create a font PdfFont font = new PdfFont(PdfFontFamily.TimesRoman, 11f); int i = 1; while (y < height) { //Draw line number before a specific line of text page.Canvas.DrawString(i.ToString(), font, PdfBrushes.Black, new PointF(15, y)); y += lineHeight + lineSpacing; i++; } //Save the document doc.SaveToFile("result.pdf"); } } }
Imports Spire.Pdf Imports Spire.Pdf.General.Find Imports Spire.Pdf.Graphics Imports System.Drawing Namespace AddLineNumber Class Program Shared Sub Main(ByVal args() As String) 'Create a PdfDocument of instance Dim doc As PdfDocument = New PdfDocument() 'Load a PDF document doc.LoadFromFile("G:\360MoveData\Users\Administrator\Desktop\sample.pdf") 'Get the first page Dim page As PdfPageBase = doc.Pages(0) 'Find the spcific text in the fisrt line Dim topLine As PdfTextFind = page.FindText("C# (pronounced See Sharp)",TextFindParameter.None).Finds(0) 'Get the line height Dim lineHeight As single = topLine.Bounds.Height 'Get the Y coordinate of the selected text Dim y As single = topLine.Bounds.Y 'Find the spcific text in the second line Dim secondLine As PdfTextFind = page.FindText("language. C#",TextFindParameter.None).Finds(0) 'Calculate the line spacing Dim lineSpacing As single = secondLine.Bounds.Top - topLine.Bounds.Bottom 'Find the specific text in the last line Dim bottomLine As PdfTextFind = page.FindText("allocation of objects",TextFindParameter.None).Finds(0) 'Get the height of the chunks Dim height As single = bottomLine.Bounds.Bottom 'Create a font Dim font As PdfFont = New PdfFont(PdfFontFamily.TimesRoman,11f) Dim i As Integer = 1 While y < height 'Draw line number before a specific line of text page.Canvas.DrawString(i.ToString(),font,PdfBrushes.Black,New PointF(15,y)) y += lineHeight + lineSpacing i = i + 1 End While 'Save the document doc.SaveToFile("result.pdf") End Sub End Class End Namespace
Output
