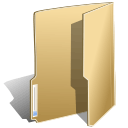
.NET (1273)
Children categories
Compared with Word document format, pictures are more convenient to share and preview across platforms, because they do not require MS Word to be installed on machines. Moreover, converting Word to images can preserve the original appearance of the document, which is useful when further modifications are not desired. In this article, you will learn how to convert Word documents to images in C# and VB.NET using Spire.Doc for .NET.
- Convert Word to JPG in C#, VB.NET
- Convert Word to SVG in C#, VB.NET
- Convert Word to PNG with Customized Resolution in C#, VB.NET
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Convert Word to JPG in C#, VB.NET
Spire.Doc for .NET offers the Document.SaveToImages() method to convert a whole Word document into individual Bitmap or Metafile images. Then, a Bitmap or Metafile image can be saved as a BMP, EMF, JPEG, PNG, GIF, or WMF format file. The following are the steps to convert a Word document to JPG images using this library.
- Create a Document object.
- Load a Word document using Document.LoadFromFile() method.
- Convert the document to Bitmap images using Document.SaveToImages() method.
- Loop through the image collection to get the specific one and save it as a JPG file.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; using System; using System.Drawing; using System.Drawing.Imaging; namespace ConvertWordToJPG { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Template.docx"); //Convert the whole document into individual images Image[] images = doc.SaveToImages(ImageType.Bitmap); //Loop through the image collection for (int i = 0; i < images.Length; i++) { //Save the image to a JPEG format file string outputfile = String.Format("Image-{0}.jpg", i); images[i].Save("C:\\Users\\Administrator\\Desktop\\Images\\" + outputfile, ImageFormat.Jpeg); } } } }
Convert Word to SVG in C#, VB.NET
Using Spire.Doc for .NET, you can save a Word document as a queue of byte arrays. Each byte array can then be written as a SVG file. The detailed steps to convert Word to SVG are as follows.
- Create a Document object.
- Load a Word file using Document.LoadFromFile() method.
- Save the document as a queue of byte arrays using Document.SaveToSVG() method.
- Loop through the items in the queue to get a specific byte array.
- Write the byte array to a SVG file.
- C#
- VB.NET
using Spire.Doc; using System; using System.Collections.Generic; using System.IO; namespace CovnertWordToSVG { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Template.docx"); //Save the document as a queue of byte arrays Queue<byte[]> svgBytes = doc.SaveToSVG(); //Loop through the items in the queue for (int i = 0; i < svgBytes.Count; i++) { //Convert the queue to an array byte[][] bytes = svgBytes.ToArray(); //Specify the output file name string outputfile = String.Format("Image-{0}.svg", i); //Write the byte[] in a SVG format file FileStream fs = new FileStream("C:\\Users\\Administrator\\Desktop\\Images\\" + outputfile, FileMode.Create); fs.Write(bytes[i], 0, bytes[i].Length); fs.Close(); } } } }
Convert Word to PNG with Customized Resolution in C#, VB.NET
An image with higher resolution is generally more clear. You can customize the image resolution while converting Word to PNG by following the following steps.
- Create a Document object.
- Load a Word file using Document.LoadFromFile() method.
- Convert the document to Bitmap images using Document.SaveToImages() method.
- Loop through the image collection to get the specific one.
- Call the custom method ResetResolution() to reset the image resolution.
- Save the image as a PNG file.
- C#
- VB.NET
using Spire.Doc; using System; using System.Drawing; using System.Drawing.Imaging; using Spire.Doc.Documents; namespace ConvertWordToPng { class Program { static void Main(string[] args) { //Create a Document object Document doc = new Document(); //Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Template.docx"); //Convert the whole document into individual images Image[] images = doc.SaveToImages(ImageType.Metafile); //Loop through the image collection for (int i = 0; i < images.Length; i++) { //Reset the resolution of a specific image Image newimage = ResetResolution(images[i] as Metafile, 150); //Save the image to a PNG format file string outputfile = String.Format("Image-{0}.png", i); newimage.Save("C:\\Users\\Administrator\\Desktop\\Images\\" + outputfile, ImageFormat.Png); } } //Set the image resolution by the ResetResolution() method public static Image ResetResolution(Metafile mf, float resolution) { int width = (int)(mf.Width * resolution / mf.HorizontalResolution); int height = (int)(mf.Height * resolution / mf.VerticalResolution); Bitmap bmp = new Bitmap(width, height); bmp.SetResolution(resolution, resolution); using (Graphics g = Graphics.FromImage(bmp)) { g.DrawImage(mf, Point.Empty); } return bmp; } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
The emphasis mark is used in Word documents to emphasize words and make them more noticeable. It is usually a dot or a circle placed above or under the emphasized words. However, manually selecting words and applying emphasis marks on them takes a lot of work. Fortunately, Spire.Doc for .NET provides a much easier way to apply emphasis marks by codes. This article will show you how to apply emphasis marks to text in Word documents using Spire.Doc for .NET.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Apply Emphasis Mark to Specified Text
The detailed steps are as follows:
- Create a Document instance.
- Load the Word document from disk using Document.LoadFromFile() method.
- Find the text you need to emphasize using Document.FindAllString() method.
- Apply emphasis mark to the found text through CharacterFormat.EmphasisMark property.
- Save the document to another Word file using Document.SaveToFile() method.
- C#
- VB.NET
using System; using Spire.Doc; using Spire.Doc.Documents; namespace applyemphasismark { class Program { static void Main(string[] args) { //Create a Document instance Document document = new Document(); //Load the Word document from disk document.LoadFromFile(@"D:\testp\test.docx"); //Find text you want to emphasize TextSelection[] textSelections = document.FindAllString("Spire.Doc for .NET", false, true); //Apply emphasis mark to the found text foreach (TextSelection selection in textSelections) { selection.GetAsOneRange().CharacterFormat.EmphasisMark = Emphasis.Dot; } //Save the document to another Word file string output = "ApllyEmphasisMark.docx"; document.SaveToFile(output, FileFormat.Docx); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PowerPoint is a presentation document that is typically used for product introductions, performance reports, teaching, and other purposes. Since the design of PowerPoint is a visual behavior and needs constant fine-tuning, it is not recommended to create PowerPoint from scratch programmatically. But if you do have the requirement to create PowerPoint documents in C# or VB.NET, you can try this solution provided by Spire.Presentation for .NET.
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Create a Simple PowerPoint Document
Spire.Presentation for .NET offers the Presentation class and the ISlide interface to represent a PowerPoint document and a slide respectively. It is quite straightforward and simple for developers to use the properties and methods under them to create or manipulate PowerPoint files. The following are the steps to generate a simple PowerPoint document using it.
- Create a Presentation object, and set the slide size type to screen 16x9 through the Presentation.SlideSize.Type property.
- Get the first slide through the Presentation.Slides[] property.
- Set the background image of the slide using ISlide.SlideBackground property.
- Add a rectangle to the slide using ISlide.Shapes.AppendShape() method, positioning the shape at the center of the slide using IAutoShape.SetShapeAlignment() method.
- Set the fill color, line style, font color, and text of the shape through other properties under the IAutoShape object.
- Save the presentation to a .pptx file using Presentation.SaveToFile() method.
- C#
- VB.NET
using System.Drawing; using Spire.Presentation; using Spire.Presentation.Drawing; namespace CreatePowerPoint { class Program { static void Main(string[] args) { //Create a Presentation object Presentation presentation = new Presentation(); //Set the slide size type to screen 16x9 presentation.SlideSize.Type = SlideSizeType.Screen16x9; //Get the first slide ISlide slide = presentation.Slides[0]; //Set the background image string imgPath = @"C:\Users\Administrator\Desktop\bgImage.jpg"; IImageData imageData = presentation.Images.Append(Image.FromFile(imgPath)); slide.SlideBackground.Type = Spire.Presentation.Drawing.BackgroundType.Custom; slide.SlideBackground.Fill.FillType = Spire.Presentation.Drawing.FillFormatType.Picture; slide.SlideBackground.Fill.PictureFill.FillType = PictureFillType.Stretch; slide.SlideBackground.Fill.PictureFill.Picture.EmbedImage = imageData; //Insert a rectangle shape Rectangle rect = new Rectangle(100, 100, 500, 80); IAutoShape shape = slide.Shapes.AppendShape(ShapeType.Rectangle, rect); //Position the shape at the center of the slide shape.SetShapeAlignment(ShapeAlignment.AlignCenter); shape.SetShapeAlignment(ShapeAlignment.DistributeVertically); //Set the fill color, line style and font color of the shape shape.Fill.FillType = FillFormatType.Solid; shape.Fill.SolidColor.Color = Color.BlueViolet; shape.ShapeStyle.LineStyleIndex = 0;//no line shape.ShapeStyle.FontColor.Color = Color.White; //Set the text of the shape shape.TextFrame.Text = "This article shows you how to create a simple PowerPoint document using Spire.Presentation for Java."; //Save to file presentation.SaveToFile("CreatePowerPoint.pptx", FileFormat.Pptx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF linearization, also known as "Fast Web View", is a way of optimizing PDF files. Ordinarily, users can view a multipage PDF file online only when their web browsers have downloaded all pages from the server. However, if the PDF file is linearized, the browsers can display the first page very quickly even if the full download has not been completed. This article will demonstrate how to convert a PDF to linearized in C# and VB.NET using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.PDF
Convert PDF to Linearized
The following are the steps to convert a PDF file to linearized:
- Load a PDF file using PdfToLinearizedPdfConverter class.
- Convert the file to linearized using PdfToLinearizedPdfConverter.ToLinearizedPdf() method.
- C#
- VB.NET
using Spire.Pdf.Conversion; namespace ConvertPdfToLinearized { class Program { static void Main(string[] args) { //Load a PDF file PdfToLinearizedPdfConverter converter = new PdfToLinearizedPdfConverter("Sample.pdf"); //Convert the file to a linearized PDF converter.ToLinearizedPdf("Linearized.pdf"); } } }
Imports Spire.Pdf.Conversion Namespace ConvertPdfToLinearized Friend Class Program Private Shared Sub Main(ByVal args As String()) 'Load a PDF file Dim converter As PdfToLinearizedPdfConverter = New PdfToLinearizedPdfConverter("Sample.pdf") 'Convert the file to a linearized PDF converter.ToLinearizedPdf("Linearized.pdf") End Sub End Class End Namespace
Open the result file in Adobe Acrobat and take a look at the document properties, you can see the value of “Fast Web View” is Yes which means the file is linearized.
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
C#/VB.NET: Convert PDF to Grayscale (Black and White)
2021-11-12 06:43:17 Written by support iceblueConverting a PDF with color images to grayscale can help you reduce the file size and print the PDF in a more affordable mode without consuming colored ink. In this article, you will learn how to achieve the conversion programmatically in C# and VB.NET using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.PDF
Convert PDF to Grayscale
The following are the steps to convert a color PDF to grayscale:
- Load a PDF file using PdfGrayConverter class.
- Convert the PDF to grayscale using PdfGrayConverter.ToGrayPdf() method.
- C#
- VB.NET
using Spire.Pdf.Conversion; namespace ConvertPdfToGrayscale { class Program { static void Main(string[] args) { //Create a PdfGrayConverter instance and load a PDF file PdfGrayConverter converter = new PdfGrayConverter(@"Sample.pdf"); //Convert the PDF to grayscale converter.ToGrayPdf("Grayscale.pdf"); converter.Dispose(); } } }
Imports Spire.Pdf.Conversion Namespace ConvertPdfToGrayscale Friend Class Program Private Shared Sub Main(ByVal args As String()) 'Create a PdfGrayConverter instance and load a PDF file Dim converter As PdfGrayConverter = New PdfGrayConverter("Sample.pdf") 'Convert the PDF to grayscale converter.ToGrayPdf("Grayscale.pdf") converter.Dispose() End Sub End Class End Namespace
The input PDF:
The output PDF:
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
C#/VB.NET: Distribute Table Rows and Columns in PowerPoint
2021-11-09 08:46:03 Written by support iceblueWhen creating a new table in PowerPoint, the rows and columns are evenly distributed by default. As you insert data into the table cells, the row heights and column widths will be automatically adjusted to fit with the contents. To make the table nicely organized, you may want to re-distribute the rows and columns. This article demonstrates how to accomplish this task in C# and VB.NET using Spire.Presentation for .NET.
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Distribute Table Rows and Columns
The following are the steps to distribute table rows and columns evenly in PowerPoint.
- Create a Presentation object, and load the sample PowerPoint document using Presentation.LoadFromFile() method.
- Get the first slide through Presentation.Slides[0] property.
- Loop through the shapes in the first slide, and determine if a certain shape is a table. If yes, convert the shape to an ITable object.
- Distribute the table rows and columns using ITable.DistributeRows() method and ITable.DistributeColumns() method, respectively.
- Save the changes to another file using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace DistributeRowsAndColumns { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation presentation = new Presentation(); //Load the PowerPoint document presentation.LoadFromFile(@"C:\Users\Administrator\Desktop\Table.pptx"); //Get the first slide ISlide slide = presentation.Slides[0]; //Loop through the shapes for (int i = 0; i < slide.Shapes.Count; i++) { //Determine if a shape is table if (slide.Shapes[i] is ITable) { //Get the table in the slide ITable table = (ITable)slide.Shapes[i]; //Distribute table rows table.DistributeRows(0, table.TableRows.Count-1); //Distribute table columns table.DistributeColumns(0, table.ColumnsList.Count-1); } } //Save the result to file presentation.SaveToFile("DistributeRowsAndColumns.pptx", FileFormat.Pptx2013); } } }
Imports Spire.Presentation Namespace DistributeRowsAndColumns Class Program Shared Sub Main(ByVal args() As String) 'Create a Presentation instance Dim presentation As Presentation = New Presentation() 'Load the PowerPoint document presentation.LoadFromFile("C:\Users\Administrator\Desktop\Table.pptx") 'Get the first slide Dim slide As ISlide = presentation.Slides(0) 'Loop through the shapes Dim i As Integer For i = 0 To slide.Shapes.Count- 1 Step i + 1 'Determine if a shape is table If TypeOf slide.Shapes(i) Is ITable Then 'Get the table in the slide Dim table As ITable = CType(slide.Shapes(i), ITable) 'Distribute table rows table.DistributeRows(0, table.TableRows.Count-1) 'Distribute table columns table.DistributeColumns(0, table.ColumnsList.Count-1) End If Next 'Save the result to file presentation.SaveToFile("DistributeRowsAndColumns.pptx", FileFormat.Pptx2013) End Sub End Class End Namespace
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Sometimes after you have finished an Excel workbook, you may need to replace some of the existing pictures with better ones for the purpose of making the workbook more appealing and persuasive. In this tutorial, you will learn how to replace a picture in Excel using Spire.XLS for .NET.
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for .NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.XLS
Replace a Picture in Excel
The following are the detailed steps to replace a picture with another one using Spire.XLS for .NET.
- Create a Workbook object.
- Load a sample file using Workbook.LoadFromFile() method.
- Use Workbook.Worksheets[0] to get the first worksheet.
- Use Worksheet.Pictures[0] property to get the first picture from the first worksheet
- Use Image.FromFile() method to load an image from the specified file, and set it as a new value of ExcelPicture.Picture property. To do so, the original picture will be replaced with the new one.
- Save the document using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; using Spire.Xls.Collections; using System.Drawing; namespace ReplacePictureinExcel { class Program { static void Main(string[] args) { //Create a Workbook instance Workbook workbook = new Workbook(); //Load the Excel file workbook.LoadFromFile (“Input.xls”); //Get the first sheet Worksheet sheet = workbook.Worksheets[0]; //Get Excel picture collection PicturesCollection pictureCollection = sheet.Pictures; //Get the first picture from the collection ExcelPicture excelPicture = pictureCollection[0]; // Creates an Image from the specified file. excelPicture.Picture = Image.FromFile (image); //Save the document workbook.SaveToFile("ReplaceImage.xlsx", ExcelVersion.Version2013); } } }
Imports Spire.Xls Imports Spire.Xls.Collections Imports System.Drawing Namespace ReplacePictureinExcel Class Program Private Shared Sub Main(args As String()) 'Create a Workbook instance Dim workbook As New Workbook() 'Load the Excel file workbook.LoadFromFile(Input.xls) 'Get the first sheet Dim sheet As Worksheet = workbook.Worksheets(0) 'Get Excel picture collection Dim pictureCollection As PicturesCollection = sheet.Pictures 'Get the first picture from the collection Dim excelPicture As ExcelPicture = pictureCollection(0) ' Creates an Image from the specified file. excelPicture.Picture = Image.FromFile(image) 'Save the document workbook.SaveToFile("ReplaceImage.xlsx", ExcelVersion.Version2013) End Sub End Class End Namespace
The original file:
The generated file:
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
C#/VB.NET: Copy Cell Ranges Between Different Workbooks
2021-10-26 01:23:39 Written by support iceblueWhen you're dealing Excel documents, it is a common task that you may need to copy data from a main workbook and paste into a separate workbook. You can copy either a selected cell range or an entire worksheet between different workbooks. This article demonstrates how to copy a selected cell range from one workbook to another by using Spire.XLS for .NET.
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for.NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.XLS
Copy a Cell Range Between Different Workbooks
Spire.XLS offers the Worksheet.Copy() method to copy data from a source range to a destination range. The destination range can be a cell range inside the same workbook or from a different workbook. The following are the steps to copy a cell range from a workbook to another.
- Create a Workbook object to load the source Excel document.
- Get the source worksheet and the source cell range using Workbook.Worksheets property and Worksheet.Range property respectively.
- Create another Workbook object to load the destination Excel document.
- Get the destination worksheet and cell range.
- Copy the data from the source range to the destination range using Worksheet.Copy(CellRange source, CellRange destRange).
- Copy the column widths from the source range to the destination range, so that the data can display properly in the destination workbook.
- Save the destination workbook to an Excel file using Workbook.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; namespace CopyCellRange { class Program { static void Main(string[] args) { //Create a Workbook object Workbook sourceBook = new Workbook(); //Load the source workbook sourceBook.LoadFromFile(@"C:\Users\Administrator\Desktop\source.xlsx"); //Get the source worksheet Worksheet sourceSheet = sourceBook.Worksheets[0]; //Get the source cell range CellRange sourceRange = sourceSheet.Range["A1:E4"]; //Create another Workbook objecy Workbook destBook = new Workbook(); //Load the destination workbook destBook.LoadFromFile(@"C:\Users\Administrator\Desktop\destination.xlsx"); //Get the destination worksheet Worksheet destSheet = destBook.Worksheets[0]; //Get the destination cell range CellRange destRange = destSheet.Range["B2:F5"]; //Copy data from the source range to the destination range sourceSheet.Copy(sourceRange, destRange); //Loop through the columns in the source range for (int i = 0; i < sourceRange.Columns.Length; i++) { //Copy the column widths also from the source range to destination range destRange.Columns[i].ColumnWidth = sourceRange.Columns[i].ColumnWidth; } //Save the destination workbook to an Excel file destBook.SaveToFile("CopyRange.xlsx"); } } }
Imports Spire.Xls Namespace CopyCellRange Class Program Shared Sub Main(ByVal args() As String) 'Create a Workbook object Dim sourceBook As Workbook = New Workbook() 'Load the source workbook sourceBook.LoadFromFile("C:\Users\Administrator\Desktop\source.xlsx") 'Get the source worksheet Dim sourceSheet As Worksheet = sourceBook.Worksheets(0) 'Get the source cell range Dim sourceRange As CellRange = sourceSheet.Range("A1:E4") 'Create another Workbook objecy Dim destBook As Workbook = New Workbook() 'Load the destination workbook destBook.LoadFromFile("C:\Users\Administrator\Desktop\destination.xlsx") 'Get the destination worksheet Dim destSheet As Worksheet = destBook.Worksheets(0) 'Get the destination cell range Dim destRange As CellRange = destSheet.Range("B2:F5") 'Copy data from the source range to the destination range sourceSheet.Copy(sourceRange, destRange) 'Loop through the columns in the source range Dim i As Integer For i = 0 To sourceRange.Columns.Length- 1 Step i + 1 'Copy the column widths also from the source range to destination range destRange.Columns(i).ColumnWidth = sourceRange.Columns(i).ColumnWidth Next 'Save the destination workbook to an Excel file destBook.SaveToFile("CopyRange.xlsx") End Sub End Class End Namespace
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF is one of the most popular document formats for sharing and writing data. You may encounter the situation where you need to extract data from PDF documents, especially the data in tables. For example, there is useful information stored in the tables of your PDF invoices and you want to extract the data for further analysis or calculation. This article demonstrates how to extract data out of PDF tables and save it in a TXT file by using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.PDF
Extract Data from PDF Tables
The following are the main steps to extract tables from a PDF document.
- Create an instance of PdfDocument class.
- Load the sample PDF document using PdfDocument.LoadFromFile() method.
- Extract tables from a specific page using PdfTableExtractor.ExtractTable(int pageIndex) method.
- Get text of a certain table cell using PdfTable.GetText(int rowIndex, int columnIndex) method.
- Save the extracted data in a .txt file.
- C#
- VB.NET
using System.IO; using System.Text; using Spire.Pdf; using Spire.Pdf.Utilities; namespace ExtractPdfTable { class Program { static void Main(string[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load the sample PDF file doc.LoadFromFile(@"C:\Users\Administrator\Desktop\table.pdf"); //Create a StringBuilder object StringBuilder builder = new StringBuilder(); //Initialize an instance of PdfTableExtractor class PdfTableExtractor extractor = new PdfTableExtractor(doc); //Declare a PdfTable array PdfTable[] tableList = null; //Loop through the pages for (int pageIndex = 0; pageIndex < doc.Pages.Count; pageIndex++) { //Extract tables from a specific page tableList = extractor.ExtractTable(pageIndex); //Determine if the table list is null if (tableList != null && tableList.Length > 0) { //Loop through the table in the list foreach (PdfTable table in tableList) { //Get row number and column number of a certain table int row = table.GetRowCount(); int column = table.GetColumnCount(); //Loop though the row and colunm for (int i = 0; i < row; i++) { for (int j = 0; j < column; j++) { //Get text from the specific cell string text = table.GetText(i, j); //Add text to the string builder builder.Append(text + " "); } builder.Append("\r\n"); } } } } //Write to a .txt file File.WriteAllText("Table.txt", builder.ToString()); } } }
Imports System.IO Imports System.Text Imports Spire.Pdf Imports Spire.Pdf.Utilities Namespace ExtractPdfTable Class Program Shared Sub Main(ByVal args() As String) 'Create a PdfDocument object Dim doc As PdfDocument = New PdfDocument() 'Load the sample PDF file doc.LoadFromFile("C:\Users\Administrator\Desktop\table.pdf") 'Create a StringBuilder object Dim builder As StringBuilder = New StringBuilder() 'Initialize an instance of PdfTableExtractor class Dim extractor As PdfTableExtractor = New PdfTableExtractor(doc) 'Declare a PdfTable array Dim tableList() As PdfTable = Nothing 'Loop through the pages Dim pageIndex As Integer For pageIndex = 0 To doc.Pages.Count- 1 Step pageIndex + 1 'Extract tables from a specific page tableList = extractor.ExtractTable(pageIndex) 'Determine if the table list is null If tableList <> Nothing And tableList.Length > 0 Then 'Loop through the table in the list Dim table As PdfTable For Each table In tableList 'Get row number and column number of a certain table Dim row As Integer = table.GetRowCount() Dim column As Integer = table.GetColumnCount() 'Loop though the row and colunm Dim i As Integer For i = 0 To row- 1 Step i + 1 Dim j As Integer For j = 0 To column- 1 Step j + 1 'Get text from the specific cell Dim text As String = table.GetText(i,j) 'Add text to the string builder builder.Append(text + " ") Next builder.Append("\r\n") Next Next End If Next 'Write to a .txt file File.WriteAllText("Table.txt", builder.ToString()) End Sub End Class End Namespace
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Sometimes you may want to print Word documents in accordance with your own preferences, for instance, print your files on custom paper sizes to make them more personalized. In this article, you will learn how to achieve this function using Spire.Doc for .NET.
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for .NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
- Package Manager
PM> Install-Package Spire.Doc
Print Word on a Custom Paper Size
The table below shows a list of core classes, methods and properties utilized in this scenario.
Name | Description |
Document Class | Represents a document model for Word. |
PaperSize Class | Specifies the size of a piece of paper. |
PrintDocument Class | Defines a reusable object that sends output to a printer, when printing from a Windows Forms application. |
PrintDocument.DefaultPageSettings Property | Gets or sets page settings that are used as defaults for all pages to be printed. |
Document.PrintDocument Property | Gets the PrintDocument object. |
DefaultPageSettings.PaperSize Property | Sets the custom paper size. |
Document.LoadFromFile() Method | Loads the sample document. |
PrintDocument.Print() Method | Prints the document. |
The following are the steps to print Word on a custom paper size.
- Instantiate a Document object
- Load the sample document using Document.LoadFromFile() method.
- Get the PrintDocument object using Document.PrintDocument property.
- Set the custom paper size using DefaultPageSettings.PaperSize Property.
- Print the document using PrintDocument.Print() method.
- C#
- VB.NET
using Spire.Doc; using System.Drawing.Printing; namespace PrintWord { class Program { static void Main(string[] args) { //Instantiate a Document object. Document doc = new Document(); //Load the document doc.LoadFromFile(@"Sample.docx"); //Get the PrintDocument object PrintDocument printDoc = doc.PrintDocument; //Customize the paper size printDoc.DefaultPageSettings.PaperSize = new PaperSize("custom", 900, 800); //Print the document printDoc.Print(); } } }
Imports Spire.Doc Imports System.Drawing.Printing Namespace PrintWord Class Program Private Shared Sub Main(args As String()) 'Instantiate a Document object. Dim doc As New Document() 'Load the document doc.LoadFromFile("Sample.docx") 'Get the PrintDocument object Dim printDoc As PrintDocument = doc.PrintDocument 'Customize the paper size printDoc.DefaultPageSettings.PaperSize = New PaperSize("custom", 900, 800) 'Print the document printDoc.Print() End Sub End Class End Namespace
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
