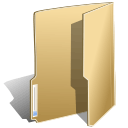
Security (5)
C#/VB.NET: Add or Remove Digital Signatures in PowerPoint
2023-02-02 07:28:00 Written by support iceblueA digital signature is a modern alternative to signing documents manually on paper with pen. It uses an advanced mathematical technique to check the authenticity and integrity of digital documents, which guarantees that the contents in a digital document comes from the signer and has not been altered since then. Sometimes PowerPoint documents that contain confidential information may require a signature. In this article, you will learn how to programmatically add or remove digital signatures in PowerPoint using Spire.Presentation for .NET.
- Add a Digital Signature to PowerPoint in C# and VB.NET
- Remove All Digital Signatures from PowerPoint in C# and VB.NET
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Add a Digital Signature to PowerPoint in C# and VB.NET
To add a digital signature, you'll need to have a valid signature certificate first. Then you can digitally sign a PowerPoint document with the certificate using Presentation.AddDigitalSignature (X509Certificate2 certificate, string comments, DateTime signTime) method. The detailed steps are as follows.
- Create a Presentation instance.
- Load a sample PowerPoint document using Presentation.LoadFromFile() method.
- Initializes an instance of X509Certificate2 class with the certificate file name and password.
- Add a digital signature to the PowerPoint document using Presentation.AddDigitalSignature (X509Certificate2 certificate, string comments, DateTime signTime) method.
- Save result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; using System; using System.Security.Cryptography.X509Certificates; namespace AddDigitalSignature { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile("input.pptx"); //Load the certificate X509Certificate2 x509 = new X509Certificate2("gary.pfx", "e-iceblue"); //Add a digital signature ppt.AddDigitalSignature(x509, "e-iceblue", DateTime.Now); //Save the result document ppt.SaveToFile("AddDigitalSignature.pptx", FileFormat.Pptx2013); } } }
Remove All Digital Signatures from PowerPoint in C# and VB.NET
At some point you may need to remove the digital signatures from a PowerPoint document. Spire.Presentation for .NET provides the Presentation.RemoveAllDigitalSignatures() method to remove all digital signatures at once. The detailed steps are as follows:
- Create a Presentation instance.
- Load a sample PowerPoint document using Presentation.LoadFromFile() method.
- Determine if the document contains digital signatures using Presentation.IsDigitallySigned property.
- Remove all digital signatures from the document using Presentation.RemoveAllDigitalSignatures() method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace RemoveDigitalSignature { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile("AddDigitalSignature.pptx"); //Detect if the document is digitally signed if (ppt.IsDigitallySigned == true) { //Remove all digital signatures ppt.RemoveAllDigitalSignatures(); } //Save the result document ppt.SaveToFile("RemoveDigitalSignature.pptx", FileFormat.Pptx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Modify Password of Encrypted PowerPoint Document in C#, VB.NET
2016-07-01 03:10:31 Written by support iceblueSometimes we may need to re-encrypt the document whose password has been or is at the risk of being leaked out. This article will show you how to load an encrypted PowerPoint document and modify its password using Spire.Presentation.
Code Snippet:
Step 1: Create an object of Presentation class.
Presentation presentation = new Presentation();
Step 2: Load an encrypted PowerPoint document into the Presentation object.
presentation.LoadFromFile("Encrypted.pptx",FileFormat.Pptx2010, "oldPassword");
Step 3: Remove the encryption.
presentation.RemoveEncryption();
Step 4: Protect the document by setting a new password.
presentation.Protect("newPassword");
Step 5: Save the file.
presentation.SaveToFile("result.pptx", FileFormat.Pptx2010);
Full Code:
using Spire.Presentation; namespace ModifyPassword { class Program { static void Main(string[] args) { Presentation presentation = new Presentation(); presentation.LoadFromFile("Encrypted.pptx", FileFormat.Pptx2010, "oldPassword"); presentation.RemoveEncryption(); presentation.Protect("newPassword"); presentation.SaveToFile("result.pptx", FileFormat.Pptx2010); } } }
Imports Spire.Presentation Namespace ModifyPassword Class Program Private Shared Sub Main(args As String()) Dim presentation As New Presentation() presentation.LoadFromFile("Encrypted.pptx", FileFormat.Pptx2010, "oldPassword") presentation.RemoveEncryption() presentation.Protect("newPassword") presentation.SaveToFile("result.pptx", FileFormat.Pptx2010) End Sub End Class End Namespace
Remove Encryption on Password-Protected PowerPoint File
2014-07-29 05:58:32 Written by support iceblueEverytime we try to open a password-protected PowerPoint file, we will then be prompted to enter the password. This can be really a bothering thing itself. But things get worse if we forget the password. To avoid this situation from being occurred, we can choose to remove the encryption if the PowerPoint file is not necessarily protected. In this article, I’ll introduce you how to remove encryption on password-protected PowerPoint file using Spire.Presentation.
In the classes of Spire.Presentation, you can invoke Presentation.LoadFromFile(string file, string password) method to load the file that you want to remove protection, then you're entitled to remove encryption by calling Presentation.RemoveEncryption() method. More details:
Step 1: Create Presentation instance and load file.
Presentation presentation = new Presentation(); presentation.LoadFromFile("Presentation1.pptx", "test");
Step 2: Remove encryption.
presentation.RemoveEncryption();
Step 3: Save and launch the file.
presentation.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx");
Effect Screenshot:
Full Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Presentation; namespace RemoveProtect { class Program { static void Main(string[] args) { // create Presentation instance and load file Presentation presentation = new Presentation(); presentation.LoadFromFile("Presentation1.pptx", "test"); //remove encryption presentation.RemoveEncryption(); //save the file presentation.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx"); } } }
Imports System Imports System.Collections.Generic Imports System.Linq Imports System.Text Imports Spire.Presentation Namespace RemoveProtect Class Program Shared Sub Main(ByVal args() As String) ' create Presentation instance and load file Dim presentation As Presentation = New Presentation() presentation.LoadFromFile("Presentation1.pptx", "test") 'remove encryption presentation.RemoveEncryption() 'save the file presentation.SaveToFile("result.pptx", FileFormat.Pptx2010) System.Diagnostics.Process.Start("result.pptx") End Sub End Class End Namespace
How to Use Password to Protect a PowerPoint Document Using C#
2014-05-15 08:05:13 Written by support iceblueSpire.Presentation for .NET is designed to help .NET developers to create, access, copy and edit PowerPoint documents as well as protect PowerPoint documents. By using Spire.Presentation, developers can save PowerPoint files with write protection to allow the presentation to be read in read only mode. This section aims to provide guidance for how to save PowerPoint documents as ReadOnly using Spire.Presentation component.
To begin with, create or open a .NET class application in Visual Studio 2005 or above versions, add Spire.Presentation.dll to your .NET project assemblies. Then, you are able to set a PPT documents to ReadOnly using the sample demo C# code we have offered below.
Step 1: Create a PPT document
Presentation presentation = new Presentation();
Step 2: Load PPT file from disk
presentation.LoadFromFile(@"..\..\..\..\..\..\Data\sample.pptx");
Step 3: Protect the document with a string password
presentation.Protect("test");
Step 4: Save and preview
presentation.SaveToFile("readonly.pptx", FileFormat.Pptx2007); System.Diagnostics.Process.Start("readonly.pptx");
Screen effect:
Full code:
//create PPT document Presentation presentation = new Presentation(); //load PPT file from disk presentation.LoadFromFile(@"..\..\..\..\..\..\Data\sample.pptx"); //protect the document with password "test" presentation.Protect("test"); //save the document presentation.SaveToFile("readonly.ppt", FileFormat.PPT); System.Diagnostics.Process.Start("readonly.ppt");
'create PPT document Dim presentation As New Presentation() 'load PPT file from disk presentation.LoadFromFile("..\..\..\..\..\..\Data\sample.pptx") protect the document with password "test" presentation.Protect("test") 'save the document presentation.SaveToFile("readonly.ppt", FileFormat.PPT) System.Diagnostics.Process.Start("readonly.ppt")
By default, anyone who can access a PowerPoint document can open and edit it. If you want to prevent your PowerPoint document from unauthorized viewing or modification, you can protect it with a password. In addition to adding password protection, you can also choose other ways to protect the document, such as marking it as final to discourage editing. When you want to make the document public, you can unprotect it at any time. In this article, we will demonstrate how to protect or unprotect PowerPoint documents in C# and VB.NET using Spire.Presentation for .NET.
- Protect a PowerPoint Document with a Password
- Mark a PowerPoint Document as Final
- Remove Password Protection from a PowerPoint Document
- Remove Mark as Final Option from a PowerPoint Document
Install Spire.Presentation for .NET
To begin with, you need to add the DLL files included in the Spire.Presentation for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Presentation
Protect a PowerPoint Document with a Password in C# and VB.NET
You can protect a PowerPoint document with a password to ensure that only the people who have the right password can view and edit it.
The following steps demonstrate how to protect a PowerPoint document with a password:
- Initialize an instance of Presentation class.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Encrypt the document with a password using Presentation.Encrypt() method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace ProtectPPTWithPassword { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation presentation = new Presentation(); //Load a PowerPoint document presentation.LoadFromFile(@"Sample.pptx"); //Encrypt the document with a password presentation.Encrypt("your password"); //Save the result document presentation.SaveToFile("Encrypted.pptx", FileFormat.Pptx2013); } } }
Mark a PowerPoint Document as Final in C# and VB.NET
You can mark a PowerPoint document as final to inform readers that the document is final and no further editing is expected.
The following steps demonstrate how to mark a PowerPoint document as final:
- Initialize an instance of Presentation class.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Mark the document as final through Presentation.DocumentProperty[] property.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace MarkPPTAsFinal { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile(@"Sample.pptx"); //Mark the document as final ppt.DocumentProperty["_MarkAsFinal"] = true; //Save the result document ppt.SaveToFile("MarkAsFinal.pptx", FileFormat.Pptx2013); } } }
Remove Password Protection from a PowerPoint Document in C# and VB.NET
You can remove password protection from a PowerPoint document by loading the document with the correct password, then removing the password protection from it.
The following steps demonstrate how to remove password protection from a PowerPoint document:
- Initialize an instance of Presentation class.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Mark the document as final through Presentation.RemoveEncryption() method.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace RemovePasswordProtectionFromPPT { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation presentation = new Presentation(); //Load a password-protected PowerPoint document with the right password presentation.LoadFromFile(@"Encrypted.pptx", "your password"); //Remove password protection from the document presentation.RemoveEncryption(); //Save the result document presentation.SaveToFile("RemoveProtection.pptx", FileFormat.Pptx2013); } } }
Remove Mark as Final Option from a PowerPoint Document in C# and VB.NET
The mark as final feature makes a PowerPoint document read-only to prevent further changes, if you decide to make changes to the document later, you can remove the mark as final option from it.
The following steps demonstrate how to remove mark as final option from a PowerPoint document:
- Initialize an instance of Presentation class.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Mark the document as final through Presentation.DocumentProperty[] property.
- Save the result document using Presentation.SaveToFile() method.
- C#
- VB.NET
using Spire.Presentation; namespace RemoveMarkAsFinalFromPPT { class Program { static void Main(string[] args) { //Create a Presentation instance Presentation ppt = new Presentation(); //Load a PowerPoint document ppt.LoadFromFile(@"MarkAsFinal.pptx"); //Remove mark as final option from the document ppt.DocumentProperty["_MarkAsFinal"] = false; //Save the result document ppt.SaveToFile("RemoveMarkAsFinal.pptx", FileFormat.Pptx2013); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
