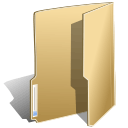
.NET (1270)
Children categories
The transition of Slide can make presentation of PPT files more attractive. Spire.Presentation for .NET allows developers to manage or customize the slide transition of the slides. Developers can not only apply different slide transitions on the slides, but also customize the behavior of these transition.
To create simple slide transition and set speed and sound mode for transition, follow the steps below:
Step 1: Download Spire.Presentation and add references in VS.
Step 2: Create an instance of Presentation and add a new presentation file.
Presentation ppt = new Presentation(); ppt.LoadFromFile(fp.TestPath + "first_quarter_business_upd.ppt");
Step 3: There are 23 transition types can be set by Spire.Presentation, such as Circle, Cover, Plus, Push, etc. These transitions can be found in TransitionType enum.
ppt.Slides[0].SlideShowTransition.Type = TransitionType.Push;
Step 4: We just applied a simple transition on the slide. Now, to make that even better and controlled, we can set the speed of the transition to create a more customized effect. Spire.Presentation offers Fast, Medium, None, Slow 4 options.
ppt.Slides[0].SlideShowTransition.Speed = TransitionSpeed.Slow;
Step 5: Set the transition sound mode such as None, StartSound, StopPreviousSound.
ppt.Slides[0].SlideShowTransition.SoundMode = TransitionSoundMode.StartSound;
Step 6: Finally write the modified presentation as a PPTX file.
ppt.SaveToFile("setTransitons.pptx", FileFormat.Pptx2010);
The PdfBorders class in Spire.PDF mainly contains three properties - DashStyle, Color and Width. By setting the value of these properties, you're able to change the appearance of grid border. In this article, I'll take color as an example to explain how to design gird border with Spire.PDF in C#.
As is shown in the following screenshot, Spire.PDF enables programmers to add color to PDF grid border as well as making the border as invisible.
Code Snippets:
Step 1: Create a new PDF document.
PdfDocument document = new PdfDocument(); PdfPageBase page=document.Pages.Add();
Step 2: Create a string array, create a 4 rows x 3 columns grid according to the length of string array. Set column width and row height.
String[] data = { "VendorName;Address;City", "Cacor Corporation;161 Southfield Rd;Southfield", "Underwater;50 N 3rd Street;Indianapolis", "J.W. Luscher Mfg.;65 Addams Street;Berkely" }; PdfGrid grid = new PdfGrid(); for (int r = 0; r < data.Length; r++) { PdfGridRow row = grid.Rows.Add(); } grid.Columns.Add(3); float width = page.Canvas.ClientSize.Width - (grid.Columns.Count + 1); grid.Columns[0].Width = width*0.15f; grid.Columns[1].Width = width * 0.15f; grid.Columns[2].Width = width * 0.15f; float height=page.Canvas.ClientSize.Height-(grid.Rows.Count+1); grid.Rows[0].Height = 12.5f; grid.Rows[1].Height = 12.5f; grid.Rows[2].Height = 12.5f; grid.Rows[3].Height = 12.5f;
Step 3: Insert data into grid.
for (int r = 0; r < data.Length; r++) { String[] rowData = data[r].Split(';'); for (int c = 0; c < rowData.Length; c++) { grid.Rows[r].Cells[c].Value = rowData[c]; } }
Step 4: Initialize a new instance of PdfBorders and set color property as LightBlue or Transparent. Apply border style to PDF grid.
PdfBorders border = new PdfBorders(); border.All = new PdfPen(Color.LightBlue); foreach (PdfGridRow pgr in grid.Rows) { foreach (PdfGridCell pgc in pgr.Cells) { pgc.Style.Borders = border; } }
Step 5: Draw the grid on PDF and save the file.
PdfLayoutResult result = grid.Draw(page, new PointF(10, 30)); document.SaveToFile("result.pdf"); System.Diagnostics.Process.Start("result.pdf");
Entire Code:
using Spire.Pdf; using Spire.Pdf.Graphics; using Spire.Pdf.Grid; using System; using System.Drawing; namespace ChangeColorofGridBorder { class Program { static void Main(string[] args) { PdfDocument document = new PdfDocument(); PdfPageBase page = document.Pages.Add(); String[] data = { "VendorName;Address;City", "Cacor Corporation;161 Southfield Rd;Southfield", "Underwater;50 N 3rd Street;Indianapolis", "J.W. Luscher Mfg.;65 Addams Street;Berkely" }; PdfGrid grid = new PdfGrid(); for (int r = 0; r < data.Length; r++) { PdfGridRow row = grid.Rows.Add(); } grid.Columns.Add(3); float width = page.Canvas.ClientSize.Width - (grid.Columns.Count + 1); grid.Columns[0].Width = width * 0.15f; grid.Columns[1].Width = width * 0.15f; grid.Columns[2].Width = width * 0.15f; float height = page.Canvas.ClientSize.Height - (grid.Rows.Count + 1); grid.Rows[0].Height = 12.5f; grid.Rows[1].Height = 12.5f; grid.Rows[2].Height = 12.5f; grid.Rows[3].Height = 12.5f; //insert data to grid for (int r = 0; r < data.Length; r++) { String[] rowData = data[r].Split(';'); for (int c = 0; c < rowData.Length; c++) { grid.Rows[r].Cells[c].Value = rowData[c]; } } grid.Rows[0].Style.Font = new PdfTrueTypeFont(new Font("Arial", 8f, FontStyle.Bold), true); //Set borders color to LightBule PdfBorders border = new PdfBorders(); border.All = new PdfPen(Color.LightBlue); foreach (PdfGridRow pgr in grid.Rows) { foreach (PdfGridCell pgc in pgr.Cells) { pgc.Style.Borders = border; } } PdfLayoutResult result = grid.Draw(page, new PointF(10, 30)); document.SaveToFile("result.pdf"); System.Diagnostics.Process.Start("result.pdf"); } } }
How to add a row to an existing table in PowerPoint documents
2015-02-05 01:43:12 Written by support iceblueSpire.Presentation is a powerful and standalone .NET component which designed for developers to operate the PowerPoint documents in C# and VB.NET. Spire.Presentation enable developers to insert a new table, remove rows or columns in an existing table, and remove the whole table from the presentation slides. This article we will show you how to add a row to an existing table in presentation slide by using C# code.
Step 1: Create Presentation instance and load file.
Presentation ppt = new Presentation(); ppt.LoadFromFile("table.pptx");
Step 2: Get the table within the PowerPoint document.
ITable table = ppt.Slides[0].Shapes[4] as ITable;
Step 3: Add a new row into the PowerPoint table and set the data for the cells in the new row.
//Get the first row TableRow row = table.TableRows[0]; //Clone the row and add it to the end of table table.TableRows.Append(row); int rowCount = table.TableRows.Count; //Get the last row TableRow lastRow = table.TableRows[rowCount - 1]; //Set new data of the first cell of last row lastRow[0].TextFrame.Text = " The first cell"; //Set new data of the second cell of last row lastRow[1].TextFrame.Text = " The second cell";
Step 4: Save the document and preview it.
ppt.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx");
Effective screenshot:
Full codes:
using Spire.Presentation; namespace AddRow { class Program { static void Main(string[] args) { Presentation ppt = new Presentation(); ppt.LoadFromFile("table.pptx"); ITable table = ppt.Slides[0].Shapes[4] as ITable; TableRow row = table.TableRows[0]; table.TableRows.Append(row); int rowCount = table.TableRows.Count; TableRow lastRow = table.TableRows[rowCount - 1]; lastRow[0].TextFrame.Text = "The first cell"; lastRow[1].TextFrame.Text = "The second cell"; ppt.SaveToFile("result.pptx", FileFormat.Pptx2010); System.Diagnostics.Process.Start("result.pptx"); } } }
How to add image watermark in PowerPoint document in C#
2015-01-30 07:54:04 Written by support iceblueSpire.Presentation offer developers an easy way to add the watermarks to the presentation slides. There are two kinds of watermarks in PowerPoint documents: text watermark and image watermark. We'll learn how to add image watermark in PowerPoint document via Spire.Presentation.
The goal of the article is to make an image as a background img watermark as following screenshot.
Here comes to the steps of how to add image watermarks in C#:
Step 1: Create a presentation document and load the document from the file
Presentation ppt = new Presentation(); ppt.LoadFromFile(fileName);
Step 2: Get the image you want to add as image watermark.
IImageData image = ppt.Images.Append(Image.FromFile("Header.png"));
Step 3: Set the properties of SlideBackground, and then fill the image as watermark.
ppt.Slides[0].SlideBackground.Type = Spire.Presentation.Drawing.BackgroundType.Custom; ppt.Slides[0].SlideBackground.Fill.FillType = FillFormatType.Picture; ppt.Slides[0].SlideBackground.Fill.PictureFill.FillType=PictureFillType.Stretch; ppt.Slides[0].SlideBackground.Fill.PictureFill.Picture.EmbedImage = image;
step4: Save the document to a new file.
ppt.SaveToFile(resultFileName, Spire.Presentation.FileFormat.PPT);
Full codes:
using Spire.Presentation; using Spire.Presentation.Drawing; using System.Drawing; namespace AddimageWatermark { class Program { static void Main(string[] args) { Presentation ppt = new Presentation(); ppt.LoadFromFile(fileName); IImageData image = ppt.Images.Append(Image.FromFile("Header.png")); ppt.Slides[0].SlideBackground.Type = Spire.Presentation.Drawing.BackgroundType.Custom; ppt.Slides[0].SlideBackground.Fill.FillType = FillFormatType.Picture; ppt.Slides[0].SlideBackground.Fill.PictureFill.FillType = PictureFillType.Stretch; ppt.Slides[0].SlideBackground.Fill.PictureFill.Picture.EmbedImage = image; if (fileExtensions == ".ppt") { ppt.SaveToFile(resultFileName, Spire.Presentation.FileFormat.PPT); } else { ppt.SaveToFile(resultFileName, Spire.Presentation.FileFormat.Pptx2007); } Viewer(resultFileName); } } }
How to extract the attachments from PDF document via PDFViewer
2015-01-29 07:58:39 Written by support iceblueSometimes we need to make use of the attachments added to the PDF files. There are 2 kinds of attachment in pdf, one is common attachment that added to the file directly, the other is attachment annotations which is add to the particular place as an annotation like the following picture, when you click correlative icon, that attachment will be opened.
In this article, we will learn how to extract these two kinds of attachment in C# via Spire.PdfViewer.
Here are the steps:
Step 1: Download PdfViewer, add PdfViewer Control to VS Toolbox (How to add control).
Step 2: Create a Windows Forms application, design Form as below.
- Two buttons respectively for common attachment and attachment annotation, and set name for them.
- One open file button and one close attachment button.
- A PdfDocmentViewer in the middle and one ViewList on the bottom.
Step 3: Get attachments
1) Get common attachment via methord GetAttachments(), then traverse attachment array and get common attachment property.
PdfDocumentAttachment[] attchments = this.pdfDocumentViewer1.GetAttachments(); if (attchments != null && attchments.Length > 0) { for (int i = 0; i < attchments.Length; i++) { PdfDocumentAttachment attachment = attchments[i]; string fileName = attachment.FileName; string mimeType = attachment.MimeType; string desc = attachment.Description; DateTime createDate = attachment.CreationTime; DateTime modifyDate = attachment.ModifyTime; Object data = attachment.Data; ListViewItem item = new ListViewItem(); item.Text = Path.GetFileName(fileName); item.SubItems.Add(mimeType); item.SubItems.Add(desc); item.SubItems.Add(createDate.ToShortDateString()); item.SubItems.Add(modifyDate.ToShortDateString()); item.Tag = attachment; this.listView1.Items.Add(item); } }
Results:
2) Extract attachment annotation:
Get attachment annotations via methord GetAttachmentAnnotaions(). Then traverse annotation array and save each annotation property as an individual item in listview.
PdfDocumentAttachmentAnnotation[] annotations = this.pdfDocumentViewer1.GetAttachmentAnnotaions(); if (annotations != null && annotations.Length > 0) { for (int i = 0; i < annotations.Length; i++) { PdfDocumentAttachmentAnnotation annotation = annotations[i]; ListViewItem item = new ListViewItem(annotation.FileName); item.SubItems.Add(annotation.Text); item.SubItems.Add(annotation.PageIndex.ToString()); item.SubItems.Add(annotation.Location.ToString()); item.Tag = annotation; this.listView1.Items.Add(item); } }
Results:
3) ListView
Here if we click the file name information of annotation attachment in the listView, PdfDocumentViewer will go to specified attachment annotation.
if (this.m_isAttachmentAnnotation) { PdfDocumentAttachmentAnnotation annotation = (PdfDocumentAttachmentAnnotation)this.listView1.SelectedItems[0].Tag; this.pdfDocumentViewer1.GotoAttachmentAnnotation(annotation); }
Double click it, the attachment can be saved to local. Get data of annotation, and write into file.
PdfDocumentAttachmentAnnotation annotation = (PdfDocumentAttachmentAnnotation)item.Tag; byte[] data = annotation.Data; writer.Write(data);
About saving common attachment:
PdfDocumentAttachment annotation = (PdfDocumentAttachment)item.Tag; byte[] data = annotation.Data; writer.Write(data);
Spire.PDFViewer is a powerful PDF Viewer component performed on .NET and WPF. It enables developers to load PDF document from stream, file and byte array. Also it supports rotation, page layout setup and thumbnail control. Worth a shot. Click to know more
How to Fill Chart Elements with Pictures in C#, VB.NET
2015-01-28 03:29:45 Written by support iceblueA chart filled with an image of company logo or propaganda is more impressive than a plain chart; adding a proper image into a chart as background will dramatically draw attention from your readers. As is similar to MS Excel, Spire.XLS enables users to insert pictures to specific chart elements such as chart area, bar area and plot area. In this article, I'll introduce how to enhance your chart by inserting image in chart area and plot area in C#, VB.NET.
Test File:
Code Snippet for Inserting Background Image:
Step 1: Create a new workbook and load the test file
Workbook workbook = new Workbook(); workbook.LoadFromFile("test.xlsx");
Step 2: Get the first worksheet from workbook, get the first chart from worksheet.
Worksheet ws = workbook.Worksheets[0]; Chart chart = ws.Charts[0];
Step 3:
A) Insert chart area with a custom picture and set the transparency of plot area as 0.9. If you don't make plot area transparent, it will cover up the background image filled in chart area. Anyway, it all depends on your own needs.
chart.ChartArea.Fill.CustomPicture(Image.FromFile("05.jpg"), "None"); chart.PlotArea.Fill.Transparency = 0.9;
B) Insert plot area with a custom picture
chart.PlotArea.Fill.CustomPicture(Image.FromFile("01.jpg"), "None");
Step 4: Save the file
workbook.SaveToFile("Sample.xlsx",ExcelVersion.Version2010);
Result:
A) Fill chart area with image
B) Fill plot area with image
Full Code:
using Spire.Xls; using System.Drawing; namespace FillChartElement { class Program { static void Main(string[] args) { Workbook workbook = new Workbook(); workbook.LoadFromFile("test.xlsx"); Worksheet ws = workbook.Worksheets[0]; Chart chart = ws.Charts[0]; // A. Fill chart area with image chart.ChartArea.Fill.CustomPicture(Image.FromFile("05.jpg"), "None"); chart.PlotArea.Fill.Transparency = 0.9; //// B.Fill plot area with image //chart.PlotArea.Fill.CustomPicture(Image.FromFile("05.jpg"), "None"); workbook.SaveToFile("Sample.xlsx", ExcelVersion.Version2010); } } }
Imports Spire.Xls Imports System.Drawing Namespace FillChartElement Class Program Private Shared Sub Main(args As String()) Dim workbook As New Workbook() workbook.LoadFromFile("test.xlsx") Dim ws As Worksheet = workbook.Worksheets(0) Dim chart As Chart = ws.Charts(0) ' A. Fill chart area with image chart.ChartArea.Fill.CustomPicture(Image.FromFile("05.jpg"), "None") chart.PlotArea.Fill.Transparency = 0.9 '''/ B.Fill plot area with image 'chart.PlotArea.Fill.CustomPicture(Image.FromFile("05.jpg"), "None"); workbook.SaveToFile("Sample.xlsx", ExcelVersion.Version2010) End Sub End Class End Namespace
There are two kinds of watermarks in PowerPoint documents we usually used in presentation slides: text watermark and image watermark. By using Spire.Presentation, developers can easily add the watermarks to the presentation slides. This section will show you how to add text watermark in PowerPoint document in C#.
Firstly, please check the effective screenshot of the text watermark in PowerPoint file added by Spire.Presentation.
Here comes to the steps of how to add text watermark in C#:
Step 1: Create a presentation document and load the document from the file
Presentation presentation = new Presentation(); presentation.LoadFromFile("sample.pptx");
Step 2: Get the size of watermark string
Font stringFont = new Font("Arial", 45); Size size = TextRenderer.MeasureText("E-iceblue", stringFont);
Step 3: Add a rectangle shape with the defined rectangle range
RectangleF rect = new RectangleF((presentation.SlideSize.Size.Width - size.Width) / 2, (presentation.SlideSize.Size.Height - size.Height) / 2, size.Width, size.Height); IAutoShape shape = presentation.Slides[0].Shapes.AppendShape(Spire.Presentation.ShapeType.Rectangle, rect);
Step 4: Set the style for the shape
shape.Fill.FillType = FillFormatType.None; shape.ShapeStyle.LineColor.Color = Color.White; shape.Rotation = -45; shape.Locking.SelectionProtection = true; shape.Line.FillType = FillFormatType.None;
Step 5: Add text to the shape and set the style of the text range
shape.TextFrame.Text = "E-iceblue"; TextRange textRange = shape.TextFrame.TextRange; //set the style of the text range textRange.Fill.FillType = Spire.Presentation.Drawing.FillFormatType.Solid; textRange.Fill.SolidColor.Color = Color.FromArgb(120, Color.Black); textRange.FontHeight = 45;
Step 6: Save the document to file
presentation.SaveToFile("result.pptx",FileFormat.Pptx2007);
Full codes:
using Spire.Presentation; using Spire.Presentation.Drawing; using System.Drawing; namespace AddTextWatermark { class Program { static void Main(string[] args) { Presentation presentation = new Presentation(); presentation.LoadFromFile("sample.pptx"); //Get the size of watermark string Font stringFont = new Font("Arial", 45); Size size = TextRenderer.MeasureText("E-iceblue", stringFont); //Define a rectangle range RectangleF rect = new RectangleF((presentation.SlideSize.Size.Width - size.Width) / 2, (presentation.SlideSize.Size.Height - size.Height) / 2, size.Width, size.Height); //Add a rectangle shape with a defined range IAutoShape shape = presentation.Slides[0].Shapes.AppendShape(Spire.Presentation.ShapeType.Rectangle, rect); //Set the style of shape shape.Fill.FillType = FillFormatType.None; shape.ShapeStyle.LineColor.Color = Color.White; shape.Rotation = -45; shape.Locking.SelectionProtection = true; shape.Line.FillType = FillFormatType.None; //add text to shape shape.TextFrame.Text = "E-iceblue"; TextRange textRange = shape.TextFrame.TextRange; //set the style of the text range textRange.Fill.FillType = Spire.Presentation.Drawing.FillFormatType.Solid; textRange.Fill.SolidColor.Color = Color.FromArgb(120, Color.Black); textRange.FontHeight = 45; presentation.SaveToFile("result.pptx", FileFormat.Pptx2007); } } }
How to set word bullet style by appending the HTML code in C#
2015-01-21 07:02:29 Written by support iceblueWith the help of Spire.Doc for .NET, developers can easily set bullet style for the existing word documents via invoke p.ListFormat.ApplyBulletStyle() method to format. This article will show you how to convert HTML list into word and set the bullet style for the word list in C#.
Firstly, please view the effective screenshot for the result word document:
Here comes to the steps:
Step 1: Create a new document and add a section to the document.
Document document = new Document(); Section section=document.AddSection();
Step 2: Add word list to the paragraph by appending HTML.
Paragraph paragraph = section.AddParagraph(); paragraph.AppendHTML("<ol><li>Version 1</li><li>Version 2</li><li>Version 3</li></ol>");
Step 3: Set the bullet style for the paragraph.
foreach (Paragraph p in section.Paragraphs) { p.ApplyStyle(BuiltinStyle.Heading2); p.ListFormat.CurrentListLevel.NumberPosition = 20; p.ListFormat.CurrentListLevel.TextPosition = 30; }
Step 4: Save the document to file
document.SaveToFile("result.docx",FileFormat.Docx);
Full codes:
using Spire.Doc; using Spire.Doc.Documents; namespace SetWordBullet { class Program { static void Main(string[] args) { Document document = new Document(); Section section = document.AddSection(); Paragraph paragraph = section.AddParagraph(); paragraph.AppendHTML(""); foreach (Paragraph p in section.Paragraphs) { p.ApplyStyle(BuiltinStyle.Heading2); p.ListFormat.CurrentListLevel.NumberPosition = 20; p.ListFormat.CurrentListLevel.TextPosition = 30; } document.SaveToFile("result.docx", FileFormat.Docx); } } }
- Version 1
- Version 2
- Version 3
In some cases, you have several items that you want them displayed on multiple lines within a PDF grid cell. However if you don't enter a line break at a specific point in a cell, these items will appear as a whole sentence. In the article, you can learn how to insert line breaks in PDF grid cell via Spire.PDF in C#, VB.NET.
Here come the detailed steps:
Step 1: Initialize a new instance of PdfDocument and add a new page to PDF document.
PdfDocument doc = new PdfDocument(); PdfPageBase page = doc.Pages.Add();
Step 2: Create a PDF gird with one row and three columns.
PdfGrid grid = new PdfGrid(); grid.Style.CellPadding = new PdfPaddings(1, 1, 1, 1); PdfGridRow row = grid.Rows.Add(); grid.Columns.Add(3); grid.Columns[0].Width = 80; grid.Columns[1].Width = 80; grid.Columns[2].Width = 80;
Step 3: Initialize a new instance of PdfGridCellTextAndStyleList class and PdfGridCellTextAndStyle class. Set parameters of the variable textAndStyle such as text, font and brush. Add textAndStlye into PdfGridCellTextAndStyleList.
PdfGridCellTextAndStyleList lst = new PdfGridCellTextAndStyleList(); PdfGridCellTextAndStyle textAndStyle = new PdfGridCellTextAndStyle(); textAndStyle.Text = "Line 1"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Airal", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle);
Step 4: Repeat step 3 to add three other lines. Here you should insert '\n' to where you want this line break appears.
textAndStyle = new PdfGridCellTextAndStyle(); textAndStyle.Text = "\nLine 2"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); textAndStyle = new PdfGridCellTextAndStyle(); textAndStyle.Text = "\nLine 3"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); textAndStyle = new PdfGridCellTextAndStyle(); textAndStyle.Text = "\nLine 4"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial",8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); row.Cells[0].Value = lst;
Step 5: Draw the gird on PDF page and save the file.
grid.Draw(page, new PointF(10, 20)); String outputFile = "..\\..\\Sample.pdf"; doc.SaveToFile(outputFile, FileFormat.PDF); System.Diagnostics.Process.Start(outputFile);
Result:
Full Code:
using Spire.Pdf; using Spire.Pdf.Graphics; using Spire.Pdf.Grid; using System; using System.Drawing; namespace LineBreak { class Program { static void Main(string[] args) { PdfDocument doc = new PdfDocument(); PdfPageBase page = doc.Pages.Add(); PdfGrid grid = new PdfGrid(); grid.Style.CellPadding = new PdfPaddings(1, 1, 1, 1); PdfGridRow row = grid.Rows.Add(); grid.Columns.Add(3); grid.Columns[0].Width = 80; grid.Columns[1].Width = 80; grid.Columns[2].Width = 80; PdfGridCellContentList lst = new PdfGridCellContentList(); PdfGridCellContent textAndStyle = new PdfGridCellContent(); textAndStyle.Text = "Line 1"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Airal", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); textAndStyle = new PdfGridCellContent(); textAndStyle.Text = "\nLine 2"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); textAndStyle = new PdfGridCellContent(); textAndStyle.Text = "\nLine 3"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); textAndStyle = new PdfGridCellContent(); textAndStyle.Text = "\nLine 4"; textAndStyle.Font = new PdfTrueTypeFont(new System.Drawing.Font("Arial", 8f, FontStyle.Regular), true); textAndStyle.Brush = PdfBrushes.Black; lst.List.Add(textAndStyle); row.Cells[0].Value = lst; grid.Draw(page, new PointF(10, 20)); String outputFile = "..\\..\\Sample.pdf"; doc.SaveToFile(outputFile, FileFormat.PDF); System.Diagnostics.Process.Start(outputFile); } } }
Imports Spire.Pdf Imports Spire.Pdf.Graphics Imports Spire.Pdf.Grid Imports System.Drawing Namespace LineBreak Class Program Private Shared Sub Main(args As String()) Dim doc As New PdfDocument() Dim page As PdfPageBase = doc.Pages.Add() Dim grid As New PdfGrid() grid.Style.CellPadding = New PdfPaddings(1, 1, 1, 1) Dim row As PdfGridRow = grid.Rows.Add() grid.Columns.Add(3) grid.Columns(0).Width = 80 grid.Columns(1).Width = 80 grid.Columns(2).Width = 80 Dim lst As New PdfGridCellContentList() Dim textAndStyle As New PdfGridCellContent() textAndStyle.Text = "Line 1" textAndStyle.Font = New PdfTrueTypeFont(New System.Drawing.Font("Airal", 8F, FontStyle.Regular), True) textAndStyle.Brush = PdfBrushes.Black lst.List.Add(textAndStyle) textAndStyle = New PdfGridCellContent() textAndStyle.Text = vbLf & "Line 2" textAndStyle.Font = New PdfTrueTypeFont(New System.Drawing.Font("Arial", 8F, FontStyle.Regular), True) textAndStyle.Brush = PdfBrushes.Black lst.List.Add(textAndStyle) textAndStyle = New PdfGridCellContent() textAndStyle.Text = vbLf & "Line 3" textAndStyle.Font = New PdfTrueTypeFont(New System.Drawing.Font("Arial", 8F, FontStyle.Regular), True) textAndStyle.Brush = PdfBrushes.Black lst.List.Add(textAndStyle) textAndStyle = New PdfGridCellContent() textAndStyle.Text = vbLf & "Line 4" textAndStyle.Font = New PdfTrueTypeFont(New System.Drawing.Font("Arial", 8F, FontStyle.Regular), True) textAndStyle.Brush = PdfBrushes.Black lst.List.Add(textAndStyle) row.Cells(0).Value = lst grid.Draw(page, New PointF(10, 20)) Dim outputFile As [String] = "..\..\Sample.pdf" doc.SaveToFile(outputFile, FileFormat.PDF) System.Diagnostics.Process.Start(outputFile) End Sub End Class End Namespace
How to create vertical table at one side of the word document
2015-01-13 08:46:41 Written by support iceblueSpire.Doc can help developers to create word table with data and format cells easily and it also supports to add text watermark into the word documents. This article will show you how to create a vertical table at one side of the word document, which looks like the vertical watermark in the word document.
Firstly, please check the effective screenshot of the vertical table at the right of the word document added by Spire.Doc:
Here comes to the steps of how to create vertical table in C#.
Step 1: Create a new document and add a section to the document.
Document document = new Document(); Section section=document.AddSection();
Step 2: Add a table with rows and columns and set the text for the table.
Table table = section.AddTable(); table.ResetCells(1, 1); TableCell cell = table.Rows[0].Cells[0]; table.Rows[0].Height = 150; cell.AddParagraph().AppendText("Draft copy in vertical style");
Step 3: Set the TextDirection for the table to RightToLeftRotated.
cell.CellFormat.TextDirection = TextDirection.RightToLeftRotated;
Step 4: Set the table format.
table.TableFormat.WrapTextAround = true; table.TableFormat.Positioning.VertRelationTo = VerticalRelation.Page; table.TableFormat.Positioning.HorizRelationTo = HorizontalRelation.Page; table.TableFormat.Positioning.HorizPosition = section.PageSetup.PageSize.Width- table.Width; table.TableFormat.Positioning.VertPosition = 200;
Step 5: Save the document to file.
document.SaveToFile("result.docx",FileFormat.docx2013);
Full codes in C#:
using Spire.Doc; using Spire.Doc.Documents; namespace CreateVerticalTable { class Program { static void Main(string[] args) { Document document = new Document(); Section section=document.AddSection(); Table table = section.AddTable(); table.ResetCells(1, 1); TableCell cell = table.Rows[0].Cells[0]; table.Rows[0].Height = 150; cell.AddParagraph().AppendText("Draft copy in vertical style"); cell.CellFormat.TextDirection = TextDirection.RightToLeftRotated; table.TableFormat.WrapTextAround = true; table.TableFormat.Positioning.VertRelationTo = VerticalRelation.Page; table.TableFormat.Positioning.HorizRelationTo = HorizontalRelation.Page; table.TableFormat.Positioning.HorizPosition = section.PageSetup.PageSize.Width- table.Width; table.TableFormat.Positioning.VertPosition = 200; document.SaveToFile("result.docx",FileFormat.docx2013); } } }
