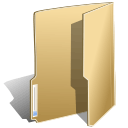
.NET (1270)
Children categories
Copy Header/Footer between Word Documents in C#, VB.NET
2015-11-11 06:02:54 Written by support iceblueWhen you create several Word documents that are closely related, you may want the header or footer of one document to be used as the header or footer of other documents. For example, you're creating internal documents with your company logo or name or other material been placed in header, you only have to create the header once and copy the header to other places.
In this article, I'll introduce you a simple and efficient solution to copy the entire header (including text and graphic) from one Word document and insert it to another.
Source Document:
Detail Steps:
Step 1: Create a new instance of Document class and load the source file.
Document doc1 = new Document(); doc1.LoadFromFile("test1.docx");
Step 2: Get the header section from the source document.
HeaderFooter header = doc1.Sections[0].HeadersFooters.Header;
Step 3: Initialize a new instance of Document and load another file that you want to insert header.
Document doc2 = new Document("test2.docx");
Step 4: Call DocuentObject.Clone() method to copy each object in the header of source file, then call DocumentObjectCollection.Add() method to insert copied object into the header of destination file.
foreach (Section section in doc2.Sections) { foreach (DocumentObject obj in header.ChildObjects) { section.HeadersFooters.Header.ChildObjects.Add(obj.Clone()); } }
Step 5: Save the changes and launch the file.
doc2.SaveToFile("test2.docx", FileFormat.Docx2013); System.Diagnostics.Process.Start("test2.docx");
Destination Document:
Full Code:
Document doc1 = new Document(); doc1.LoadFromFile("test1.docx"); HeaderFooter header = doc1.Sections[0].HeadersFooters.Header; Document doc2 = new Document("test2.docx"); foreach (Section section in doc2.Sections) { foreach (DocumentObject obj in header.ChildObjects) { section.HeadersFooters.Header.ChildObjects.Add(obj.Clone()); } } doc2.SaveToFile("test2.docx", FileFormat.Docx2013); System.Diagnostics.Process.Start("test2.docx");
Dim doc1 As New Document() doc1.LoadFromFile("test1.docx") Dim header As HeaderFooter = doc1.Sections(0).HeadersFooters.Header Dim doc2 As New Document("test2.docx") For Each section As Section In doc2.Sections For Each obj As DocumentObject In header.ChildObjects section.HeadersFooters.Header.ChildObjects.Add(obj.Clone()) Next Next doc2.SaveToFile("test2.docx", FileFormat.Docx2013) System.Diagnostics.Process.Start("test2.docx")
Sometimes, we need to copy the data with formatting from one cell range (a row or a column) to another. It is an extremely easy work in MS Excel, because we can select the source range and then use Copy and Paste function to insert the same data in destination cells.
In fact, Spire.XLS has provided two methods Worksheet.Copy(CellRange sourceRange, CellRange destRange, bool copyStyle) and Worksheet.Copy(CellRange sourceRange, Worksheet worksheet, int destRow, int destColumn, bool copyStyle) that allow programmers to copy rows and columns within or between workbooks. This article will present how to duplicate a row within a workbook using Spire.XLS.
Test File:
Code Snippet:
Step 1: Create a new instance of Workbook class and load the sample file.
Workbook book = new Workbook(); book.LoadFromFile("sample.xlsx", ExcelVersion.Version2010);
Step 2: Get the first worksheet.
Worksheet sheet = book.Worksheets[0];
Step 3: Call Worksheet.Copy(CellRange sourceRange, CellRange destRange, bool copyStyle) method to copy data from source range (A1:G1) to destination range (A4:G4) and maintain the formatting.
sheet.Copy(sheet.Range["A1:G1"], sheet.Range["A4:G4"],true);
Step 4: Save and launch the file.
book.SaveToFile("result.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("result.xlsx");
Output:
Full Code:
using Spire.Xls; namespace DuplicateRowExcel { class Program { static void Main(string[] args) { Workbook book = new Workbook(); book.LoadFromFile("sample.xlsx", ExcelVersion.Version2010); Worksheet sheet = book.Worksheets[0]; sheet.Copy(sheet.Range["A1:G1"], sheet.Range["A4:G4"], true); book.SaveToFile("result.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("result.xlsx"); } } }
Imports Spire.Xls Namespace DuplicateRowExcel Class Program Private Shared Sub Main(args As String()) Dim book As New Workbook() book.LoadFromFile("sample.xlsx", ExcelVersion.Version2010) Dim sheet As Worksheet = book.Worksheets(0) sheet.Copy(sheet.Range("A1:G1"), sheet.Range("A4:G4"), True) book.SaveToFile("result.xlsx", ExcelVersion.Version2010) System.Diagnostics.Process.Start("result.xlsx") End Sub End Class End Namespace
With the help of Spire.Presentation for .NET, we can easily save PowerPoint slides as image in C# and VB.NET. Sometimes, we need to use the resulted images for other purpose and the image size becomes very important. To ensure the image is easy and beautiful to use, we need to set the image with specified size beforehand. This example will demonstrate how to save a particular presentation slide as image with specified size by using Spire.Presentation for your .NET applications.
Note: Before Start, please download the latest version of Spire.Presentation and add Spire.Presentation.dll in the bin folder as the reference of Visual Studio.
Step 1: Create a presentation document and load the document from file.
Presentation presentation = new Presentation(); presentation.LoadFromFile("sample.pptx");
Step 2: Save the first slide to Image and set the image size to 600*400.
Image img = presentation.Slides[0].SaveAsImage(600, 400);
Step 3: Save image to file.
img.Save("result.png",System.Drawing.Imaging.ImageFormat.Png);
Effective screenshot of the resulted image with specified size:
Full codes:
using Spire.Presentation; using System.Drawing; namespace SavePowerPointSlideasImage { class Program { static void Main(string[] args) { Presentation presentation = new Presentation(); presentation.LoadFromFile("sample.pptx"); Image img = presentation.Slides[0].SaveAsImage(600, 400); img.Save("result.png", System.Drawing.Imaging.ImageFormat.Png); } }
How to Set the Background Color of Legend in an Excel Chart
2015-10-27 08:50:44 Written by support iceblueThere are many kinds of areas in a chart, such as chart area, plot area, legend area. Spire.XLS offers properties to set the performance of each area easily in C# and VB.NET. We have already shown you how to set the background color and image for chart area and plot area in C#. This article will show you how to set the background color for chart legend in C# with the help of Spire.XLS 7.8.43 or above.
Firstly, please check the original screenshot of excel chart with the automatic setting for chart legend.
Code Snippet of how to set the background color of legend in a Chart:
Step 1: Create a new workbook and load from file.
Workbook workbook = new Workbook(); workbook.LoadFromFile("sample.xlsx");
Step 2: Get the first worksheet from workbook and then get the first chart from the worksheet.
Worksheet ws = workbook.Worksheets[0]; Chart chart = ws.Charts[0];
Step 3: Change the background color of the legend in a chart and specify a Solid Fill of SkyBlue.
XlsChartFrameFormat x = chart.Legend.FrameFormat as XlsChartFrameFormat; x.Fill.FillType = ShapeFillType.SolidColor; x.ForeGroundColor = Color.SkyBlue;
Step 4: Save the document to file.
workbook.SaveToFile("result.xlsx",ExcelVersion.Version2010);
Effective screenshot after fill the background color for Excel chart legend:
Full codes:
using Spire.Xls; using Spire.Xls.Core.Spreadsheet.Charts; using System.Drawing; namespace SetBackgroundColor { class Program { static void Main(string[] args) { Workbook workbook = new Workbook(); workbook.LoadFromFile("sample.xlsx"); Worksheet ws = workbook.Worksheets[0]; Chart chart = ws.Charts[0]; XlsChartFrameFormat x = chart.Legend.FrameFormat as XlsChartFrameFormat; x.Fill.FillType = ShapeFillType.SolidColor; x.ForeGroundColor = Color.SkyBlue; workbook.SaveToFile("result.xlsx", ExcelVersion.Version2010); } } }
The format of data marker in a line, scatter and radar chart can be changed and customized, which makes it more attractive and distinguishable. We could set markers' built-in type, size, background color, foreground color and transparency in Excel. This article is going to introduce how to achieve those features in C# using Spire.XLS.
Note: before start, please download the latest version of Spire.XLS and add the .dll in the bin folder as the reference of Visual Studio.
Step 1: Create a workbook with sheet and add some sample data.
Workbook workbook = new Workbook(); workbook.CreateEmptySheets(1); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Demo"; sheet.Range["A1"].Value = "Tom"; sheet.Range["A2"].NumberValue = 1.5; sheet.Range["A3"].NumberValue = 2.1; sheet.Range["A4"].NumberValue = 3.6; sheet.Range["A5"].NumberValue = 5.2; sheet.Range["A6"].NumberValue = 7.3; sheet.Range["A7"].NumberValue = 3.1; sheet.Range["B1"].Value = "Kitty"; sheet.Range["B2"].NumberValue = 2.5; sheet.Range["B3"].NumberValue = 4.2; sheet.Range["B4"].NumberValue = 1.3; sheet.Range["B5"].NumberValue = 3.2; sheet.Range["B6"].NumberValue = 6.2; sheet.Range["B7"].NumberValue = 4.7;
Step 2: Create a Scatter-Markers chart based on the sample data.
Chart chart = sheet.Charts.Add(ExcelChartType.ScatterMarkers); chart.DataRange = sheet.Range["A1:B7"]; chart.PlotArea.Visible=false; chart.SeriesDataFromRange = false; chart.TopRow = 5; chart.BottomRow = 22; chart.LeftColumn = 4; chart.RightColumn = 11; chart.ChartTitle = "Chart with Markers"; chart.ChartTitleArea.IsBold = true; chart.ChartTitleArea.Size = 10;
Step 3: Format the markers in the chart by setting the background color, foreground color, type, size and transparency.
Spire.Xls.Charts.ChartSerie cs1 = chart.Series[0]; cs1.DataFormat.MarkerBackgroundColor = Color.RoyalBlue; cs1.DataFormat.MarkerForegroundColor = Color.WhiteSmoke; cs1.DataFormat.MarkerSize = 7; cs1.DataFormat.MarkerStyle = ChartMarkerType.PlusSign; cs1.DataFormat.MarkerTransparencyValue = 0.8; Spire.Xls.Charts.ChartSerie cs2 = chart.Series[1]; cs2.DataFormat.MarkerBackgroundColor = Color.Pink; cs2.DataFormat.MarkerSize = 9; cs2.DataFormat.MarkerStyle = ChartMarkerType.Diamond; cs2.DataFormat.MarkerTransparencyValue = 0.9;
Step 4: Save the document and launch to see effects.
workbook.SaveToFile("S3.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("S3.xlsx");
Effects:
Full Codes:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Xls; using System.Drawing; namespace ConsoleApplication2 { class Program { static void Main(string[] args) { Workbook workbook = new Workbook(); workbook.CreateEmptySheets(1); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Demo"; sheet.Range["A1"].Value = "Tom"; sheet.Range["A2"].NumberValue = 1.5; sheet.Range["A3"].NumberValue = 2.1; sheet.Range["A4"].NumberValue = 3.6; sheet.Range["A5"].NumberValue = 5.2; sheet.Range["A6"].NumberValue = 7.3; sheet.Range["A7"].NumberValue = 3.1; sheet.Range["B1"].Value = "Kitty"; sheet.Range["B2"].NumberValue = 2.5; sheet.Range["B3"].NumberValue = 4.2; sheet.Range["B4"].NumberValue = 1.3; sheet.Range["B5"].NumberValue = 3.2; sheet.Range["B6"].NumberValue = 6.2; sheet.Range["B7"].NumberValue = 4.7; Chart chart = sheet.Charts.Add(ExcelChartType.ScatterMarkers); chart.DataRange = sheet.Range["A1:B7"]; chart.PlotArea.Visible=false; chart.SeriesDataFromRange = false; chart.TopRow = 5; chart.BottomRow = 22; chart.LeftColumn = 4; chart.RightColumn = 11; chart.ChartTitle = "Chart with Markers"; chart.ChartTitleArea.IsBold = true; chart.ChartTitleArea.Size = 10; Spire.Xls.Charts.ChartSerie cs1 = chart.Series[0]; cs1.DataFormat.MarkerBackgroundColor = Color.RoyalBlue; cs1.DataFormat.MarkerForegroundColor = Color.WhiteSmoke; cs1.DataFormat.MarkerSize = 7; cs1.DataFormat.MarkerStyle = ChartMarkerType.PlusSign; cs1.DataFormat.MarkerTransparencyValue = 0.8; Spire.Xls.Charts.ChartSerie cs2 = chart.Series[1]; cs2.DataFormat.MarkerBackgroundColor = Color.Pink; cs2.DataFormat.MarkerSize = 9; cs2.DataFormat.MarkerStyle = ChartMarkerType.Diamond; cs2.DataFormat.MarkerTransparencyValue = 0.9; workbook.SaveToFile("S3.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("S3.xlsx"); } } }
In some cases, you might need to rotate PDF pages. For example, when you receive a PDF document that contains disoriented pages, you may wish to rotate the pages so you can read the document easier. In this article, you will learn how to rotate pages in PDF in C# and VB.NET using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.PDF
Rotate a Specific Page in PDF using C# and VB.NET
Rotation is based on 90-degree increments. You can rotate a PDF page by 0/90/180/270 degrees. The following are the steps to rotate a PDF page:
- Create an instance of PdfDocument class.
- Load a PDF document using PdfDocument.LoadFromFile() method.
- Get the desired page by its index (zero-based) through PdfDocument.Pages[pageIndex] property.
- Get the original rotation angle of the page through PdfPageBase.Rotation property.
- Increase the original rotation angle by desired degrees.
- Apply the new rotation angle to the page through PdfPageBase.Rotation property.
- Save the result document using PdfDocument.SaveToFile() method.
- C#
- VB.NET
using Spire.Pdf; namespace RotatePdfPage { class Program { static void Main(string[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.LoadFromFile("Sample.pdf"); //Get the first page PdfPageBase page = pdf.Pages[0]; //Get the original rotation angle of the page int rotation = (int)page.Rotation; //Rotate the page 180 degrees clockwise based on the original rotation angle rotation += (int)PdfPageRotateAngle.RotateAngle180; page.Rotation = (PdfPageRotateAngle)rotation; //Save the result document pdf.SaveToFile("Rotate.pdf"); } } }
Rotate All Pages in PDF using C# and VB.NET
The following are the steps to rotate all pages in a PDF document:
- Create an instance of PdfDocument class.
- Load a PDF document using PdfDocument.LoadFromFile() method.
- Loop through each page in the document.
- Get the original rotation angle of the page through PdfPageBase.Rotation property.
- Increase the original rotation angle by desired degrees.
- Apply the new rotation angle to the page through PdfPageBase.Rotation property.
- Save the result document using PdfDocument.SaveToFile() method.
- C#
- VB.NET
using Spire.Pdf; namespace RotateAllPdfPages { class Program { static void Main(string[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.LoadFromFile("Sample.pdf"); foreach (PdfPageBase page in pdf.Pages) { //Get the original rotation angle of the page int rotation = (int)page.Rotation; //Rotate the page 180 degrees clockwise based on the original rotation angle rotation += (int)PdfPageRotateAngle.RotateAngle180; page.Rotation = (PdfPageRotateAngle)rotation; } //Save the result document pdf.SaveToFile("RotateAll.pdf"); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
In certain cases, you may need to adjust the page size of a Word document to ensure that it fits your specific needs or preferences. For instance, if you are creating a document that will be printed on a small paper size, such as a brochure or flyer, you may want to decrease the page size of the document to avoid any cropping or scaling issues during printing. In this article, we will explain how to adjust the page size of a Word document in C# and VB.NET using Spire.Doc for .NET.
- Adjust the Page Size of a Word Document to a Standard Page Size in C# and VB.NET
- Adjust the Page Size of a Word Document to a Custom Page Size in C# and VB.NET
Install Spire.Doc for .NET
To begin with, you need to add the DLL files included in the Spire.Doc for.NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.Doc
Adjust the Page Size of a Word Document to a Standard Page Size in C# and VB.NET
With Spire.Doc for .NET, you can easily adjust the page sizes of Word documents to a variety of standard page sizes, such as A3, A4, A5, A6, B4, B5, B6, letter, legal, and tabloid. The following steps explain how to change the page size of a Word document to a standard page size using Spire.Doc for .NET:
- Initialize an instance of the Document class.
- Load a Word document using the Document.LoadFromFile() method.
- Iterate through the sections in the document.
- Adjust the page size of each section to a standard page size by setting the value of the Section.PageSetup.PageSize property to a constant value of the PageSize enum.
- Save the result document using the Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using Spire.Doc.Documents; namespace ChangePageSizeToStandardSize { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document doc = new Document(); //Load a Word document doc.LoadFromFile("Input.docx"); //Iterate through the sections in the document foreach (Section section in doc.Sections) { //Change the page size of each section to A4 section.PageSetup.PageSize = PageSize.A4; } //Save the result document doc.SaveToFile("StandardSize.docx", FileFormat.Docx2016); } } }
Adjust the Page Size of a Word Document to a Custom Page Size in C# and VB.NET
If you plan to print your document on paper with dimensions that don't match any standard paper size, you can change the page size of your document to a custom page size that matches the exact dimensions of the paper. The following steps explain how to change the page size of a Word document to a custom page size using Spire.Doc for .NET:
- Initialize an instance of the Document class.
- Load a Word document using the Document.LoadFromFile() method.
- Initialize an instance of the SizeF structure from specified dimensions.
- Iterate through the sections in the document.
- Adjust the page size of each section to the specified dimensions by assigning the SizeF instance to the Section.PageSetup.PageSize property.
- Save the result document using the Document.SaveToFile() method.
- C#
- VB.NET
using Spire.Doc; using System.Drawing; namespace ChangePageSizeToCustomSize { internal class Program { static void Main(string[] args) { //Initialize an instance of the Document class Document doc = new Document(); //Load a Word document doc.LoadFromFile("Input.docx"); //Initialize an instance of the SizeF structure from specified dimensions SizeF customSize = new SizeF(600, 800); //Iterate through the sections in the document foreach (Section section in doc.Sections) { //Change the page size of each section to the specified dimensions section.PageSetup.PageSize = customSize; } //Save the result document doc.SaveToFile("CustomSize.docx", FileFormat.Docx2016); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
A gauge chart (or speedometer chart) is a combination of doughnut chart and pie chart. It only displays a single value which is used to indicate how far you are from reaching a goal. In this article, you'll learn how to create a gauge chart in C# via Spire.XLS.
Here is the gauge chart that I'm going to create.
Code Snippet:
Step 1: Create a new workbook and add some sample data into the first sheet.
Workbook book = new Workbook(); Worksheet sheet = book.Worksheets[0]; sheet.Range["A1"].Value = "Value"; sheet.Range["A2"].Value = "30"; sheet.Range["A3"].Value = "60"; sheet.Range["A4"].Value = "90"; sheet.Range["A5"].Value = "180"; sheet.Range["C2"].Value = "value"; sheet.Range["C3"].Value = "pointer"; sheet.Range["C4"].Value = "End"; sheet.Range["D2"].Value = "10"; sheet.Range["D3"].Value = "1"; sheet.Range["D4"].Value = "189";
Step 2: Create a doughnut chart based on the data from A1 to A5. Set the chart position.
Chart chart = sheet.Charts.Add(ExcelChartType.Doughnut); chart.DataRange = sheet.Range["A1:A5"]; chart.SeriesDataFromRange = false; chart.HasLegend = true; chart.LeftColumn = 2; chart.TopRow = 7; chart.RightColumn = 9; chart.BottomRow = 25;
Step 3: Set format of Value series. Following code makes the graphic looks like a semi-circle.
var cs1 = (ChartSerie)chart.Series["Value"]; cs1.Format.Options.DoughnutHoleSize = 60; cs1.DataFormat.Options.FirstSliceAngle = 270; cs1.DataPoints[0].DataFormat.Fill.ForeColor = Color.Yellow; cs1.DataPoints[1].DataFormat.Fill.ForeColor = Color.PaleVioletRed; cs1.DataPoints[2].DataFormat.Fill.ForeColor = Color.DarkViolet; cs1.DataPoints[3].DataFormat.Fill.Visible = false;
Step 4: Add a new series to the doughnut chart, set chart type as Pie, and set the data range for the series. Format the each data point in the series to make sure only the pointer category is visible in the graphic.
var cs2 = (ChartSerie)chart.Series.Add("Pointer", ExcelChartType.Pie); cs2.Values = sheet.Range["D2:D4"]; cs2.UsePrimaryAxis = false; cs2.DataPoints[0].DataLabels.HasValue= true; cs2.DataFormat.Options.FirstSliceAngle = 270; cs2.DataPoints[0].DataFormat.Fill.Visible = false; cs2.DataPoints[1].DataFormat.Fill.FillType = ShapeFillType.SolidColor; cs2.DataPoints[1].DataFormat.Fill.ForeColor = Color.Black; cs2.DataPoints[2].DataFormat.Fill.Visible = false;
Step 5: Save and launch to view the effect.
book.SaveToFile("AddGaugeChart.xlsx", FileFormat.Version2010); System.Diagnostics.Process.Start("AddGaugeChart.xlsx");
Full Code:
using Spire.Xls; using Spire.Xls.Charts; using System.Drawing; namespace CreateGauge { class Program { static void Main(string[] args) { Workbook book = new Workbook(); Worksheet sheet = book.Worksheets[0]; sheet.Range["A1"].Value = "Value"; sheet.Range["A2"].Value = "30"; sheet.Range["A3"].Value = "60"; sheet.Range["A4"].Value = "90"; sheet.Range["A5"].Value = "180"; sheet.Range["C2"].Value = "value"; sheet.Range["C3"].Value = "pointer"; sheet.Range["C4"].Value = "End"; sheet.Range["D2"].Value = "10"; sheet.Range["D3"].Value = "1"; sheet.Range["D4"].Value = "189"; Chart chart = sheet.Charts.Add(ExcelChartType.Doughnut); chart.DataRange = sheet.Range["A1:A5"]; chart.SeriesDataFromRange = false; chart.HasLegend = true; chart.LeftColumn = 2; chart.TopRow = 7; chart.RightColumn = 9; chart.BottomRow = 25; var cs1 = (ChartSerie)chart.Series["Value"]; cs1.Format.Options.DoughnutHoleSize = 60; cs1.DataFormat.Options.FirstSliceAngle = 270; cs1.DataPoints[0].DataFormat.Fill.ForeColor = Color.Yellow; cs1.DataPoints[1].DataFormat.Fill.ForeColor = Color.PaleVioletRed; cs1.DataPoints[2].DataFormat.Fill.ForeColor = Color.DarkViolet; cs1.DataPoints[3].DataFormat.Fill.Visible = false; var cs2 = (ChartSerie)chart.Series.Add("Pointer", ExcelChartType.Pie); cs2.Values = sheet.Range["D2:D4"]; cs2.UsePrimaryAxis = false; cs2.DataPoints[0].DataLabels.HasValue = true; cs2.DataFormat.Options.FirstSliceAngle = 270; cs2.DataPoints[0].DataFormat.Fill.Visible = false; cs2.DataPoints[1].DataFormat.Fill.FillType = ShapeFillType.SolidColor; cs2.DataPoints[1].DataFormat.Fill.ForeColor = Color.Black; cs2.DataPoints[2].DataFormat.Fill.Visible = false; book.SaveToFile("AddGaugeChart.xlsx", FileFormat.Version2010); System.Diagnostics.Process.Start("AddGaugeChart.xlsx"); } } }
In our tutorials, there are articles introducing the method to insert, remove, position text box and extract text from text box. This article is going to give the documentation of how to set the internal margin for textbox with the position and line style settings included using Spire.Doc.
Note: before start, please download the latest version of Spire.Doc and add the .dll in the bin folder as the reference of Visual Studio.
Step 1: Create a Word document and add a section.
Document document = new Document(); Section sec = document.AddSection();
Step 2: Add a text box and append sample text.
TextBox TB = document.Sections[0].AddParagraph().AppendTextBox(310, 90); Paragraph para = TB.Body.AddParagraph(); TextRange TR = para.AppendText("Using Spire.Doc, developers will find a simple and effective method to endow their applications with rich MS Word features. "); TR.CharacterFormat.FontName = "Cambria "; TR.CharacterFormat.FontSize = 13;
Step 3: Set exact position for the text box.
TB.Format.HorizontalOrigin = HorizontalOrigin.Page; TB.Format.HorizontalPosition = 80; TB.Format.VerticalOrigin = VerticalOrigin.Page; TB.Format.VerticalPosition = 100;
Step 4: Set line style for the text box.
TB.Format.LineStyle = TextBoxLineStyle.Double; TB.Format.LineColor = Color.CornflowerBlue; TB.Format.LineDashing = LineDashing.DashDotDot; TB.Format.LineWidth = 5;
Step 5: Set internal margin for the text box:
TB.Format.InternalMargin.Top = 15; TB.Format.InternalMargin.Bottom = 10; TB.Format.InternalMargin.Left = 12; TB.Format.InternalMargin.Right = 10;
Step 6: Save the document and launch to see effects.
document.SaveToFile("result.docx",FileFormat.docx2013); System.Diagnostics.Process.Start("result.docx");
Effects:
Full Codes:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Doc; using Spire.Doc.Documents; using Spire.Doc.Fields; using System.Drawing; namespace Demo { class Program { static void Main(string[] args) { Document document = new Document(); Section sec = document.AddSection(); TextBox TB = document.Sections[0].AddParagraph().AppendTextBox(310, 90); Paragraph para = TB.Body.AddParagraph(); TextRange TR = para.AppendText("Using Spire.Doc, developers will find a simple and effective method to endow their applications with rich MS Word features. "); TR.CharacterFormat.FontName = "Cambria "; TR.CharacterFormat.FontSize = 13; TB.Format.HorizontalOrigin = HorizontalOrigin.Page; TB.Format.HorizontalPosition = 80; TB.Format.VerticalOrigin = VerticalOrigin.Page; TB.Format.VerticalPosition = 100; TB.Format.LineStyle = TextBoxLineStyle.Double; TB.Format.LineColor = Color.CornflowerBlue; TB.Format.LineDashing = LineDashing.DashDotDot; TB.Format.LineWidth = 5; TB.Format.InternalMargin.Top = 15; TB.Format.InternalMargin.Bottom = 10; TB.Format.InternalMargin.Left = 12; TB.Format.InternalMargin.Right = 10; document.SaveToFile("result.docx",FileFormat.docx2013); System.Diagnostics.Process.Start("result.docx"); } } }
By default, Excel sets the axis properties automatically for charts. These properties include axis options like maximum & minimum value, major & minor unit, major & minor tick mark type, axis labels position, axis across value and whether values in reverse order. Sometimes we need to set those properties manually to beautify and perfect the charts. This article is going to introduce the method to customize axis setting for Excel chart in C# using Spire.XLS.
Note: before start, please download the latest version of Spire.XLS and add the .dll in the bin folder as the reference of Visual Studio.
Step 1: Create a workbook and add a sheet filled with some sample data.
Workbook workbook = new Workbook(); workbook.CreateEmptySheets(1); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Demo"; sheet.Range["A1"].Value = "Month"; sheet.Range["A2"].Value = "Jan"; sheet.Range["A3"].Value = "Feb"; sheet.Range["A4"].Value = "Mar"; sheet.Range["A5"].Value = "Apr"; sheet.Range["A6"].Value = "May"; sheet.Range["A7"].Value = "Jun"; sheet.Range["A8"].Value = "Jul"; sheet.Range["A9"].Value = "Aug"; sheet.Range["B1"].Value = "Planned"; sheet.Range["B2"].NumberValue = 38; sheet.Range["B3"].NumberValue = 47; sheet.Range["B4"].NumberValue = 39; sheet.Range["B5"].NumberValue = 36; sheet.Range["B6"].NumberValue = 27; sheet.Range["B7"].NumberValue = 25; sheet.Range["B8"].NumberValue = 36; sheet.Range["B9"].NumberValue = 48;
Step 2: Create a column clustered chart based on the sample data.
Chart chart = sheet.Charts.Add(ExcelChartType.ColumnClustered); chart.DataRange = sheet.Range["B1:B9"]; chart.SeriesDataFromRange = false; chart.PlotArea.Visible = false; chart.TopRow = 6; chart.BottomRow = 25; chart.LeftColumn = 2; chart.RightColumn = 9; chart.ChartTitle = "Chart with Customized Axis"; chart.ChartTitleArea.IsBold = true; chart.ChartTitleArea.Size = 12; Spire.Xls.Charts.ChartSerie cs1 = chart.Series[0]; cs1.CategoryLabels = sheet.Range["A2:A9"];
Step 3: Set the customized axis properties for the chart.
chart.PrimaryValueAxis.MajorUnit = 8; chart.PrimaryValueAxis.MinorUnit = 2; chart.PrimaryValueAxis.MaxValue = 50; chart.PrimaryValueAxis.MinValue = 0; chart.PrimaryValueAxis.IsReverseOrder = false; chart.PrimaryValueAxis.MajorTickMark = TickMarkType.TickMarkOutside; chart.PrimaryValueAxis.MinorTickMark = TickMarkType.TickMarkInside; chart.PrimaryValueAxis.TickLabelPosition = TickLabelPositionType.TickLabelPositionNextToAxis; chart.PrimaryValueAxis.CrossesAt = 0;
Step 4: Save the document and launch to see effects.
workbook.SaveToFile("Result.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("Result.xlsx");
Effects:
Full codes:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Xls; using System.Drawing; namespace ConsoleApplication2 { class Program { static void Main(string[] args) { Workbook workbook = new Workbook(); workbook.CreateEmptySheets(1); Worksheet sheet = workbook.Worksheets[0]; sheet.Name = "Demo"; sheet.Range["A1"].Value = "Month"; sheet.Range["A2"].Value = "Jan"; sheet.Range["A3"].Value = "Feb"; sheet.Range["A4"].Value = "Mar"; sheet.Range["A5"].Value = "Apr"; sheet.Range["A6"].Value = "May"; sheet.Range["A7"].Value = "Jun"; sheet.Range["A8"].Value = "Jul"; sheet.Range["A9"].Value = "Aug"; sheet.Range["B1"].Value = "Planned"; sheet.Range["B2"].NumberValue = 38; sheet.Range["B3"].NumberValue = 47; sheet.Range["B4"].NumberValue = 39; sheet.Range["B5"].NumberValue = 36; sheet.Range["B6"].NumberValue = 27; sheet.Range["B7"].NumberValue = 25; sheet.Range["B8"].NumberValue = 36; sheet.Range["B9"].NumberValue = 48; Chart chart = sheet.Charts.Add(ExcelChartType.ColumnClustered); chart.DataRange = sheet.Range["B1:B9"]; chart.SeriesDataFromRange = false; chart.PlotArea.Visible = false; chart.TopRow = 6; chart.BottomRow = 25; chart.LeftColumn = 2; chart.RightColumn = 9; chart.ChartTitle = "Chart with Customized Axis"; chart.ChartTitleArea.IsBold = true; chart.ChartTitleArea.Size = 12; Spire.Xls.Charts.ChartSerie cs1 = chart.Series[0]; cs1.CategoryLabels = sheet.Range["A2:A9"]; chart.PrimaryValueAxis.MajorUnit = 8; chart.PrimaryValueAxis.MinorUnit = 2; chart.PrimaryValueAxis.MaxValue = 50; chart.PrimaryValueAxis.MinValue = 0; chart.PrimaryValueAxis.IsReverseOrder = false; chart.PrimaryValueAxis.MajorTickMark = TickMarkType.TickMarkOutside; chart.PrimaryValueAxis.MinorTickMark = TickMarkType.TickMarkInside; chart.PrimaryValueAxis.TickLabelPosition = TickLabelPositionType.TickLabelPositionNextToAxis; chart.PrimaryValueAxis.CrossesAt = 0; workbook.SaveToFile("Result.xlsx", ExcelVersion.Version2010); System.Diagnostics.Process.Start("Result.xlsx"); } } }
