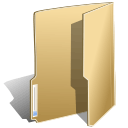
.NET (1270)
Children categories
Excel print options (also called as sheet options) allow users to control how worksheet pages are printed, such as set print paper size, print area, print titles, page order and so on. This article mainly discusses how developers set print options in C# by using Spire.XLS.
Here comes to the details of how developers configure print options in C#:
- Download Spire.XLS for .NET (or Spire.Office for .NET) and install it on your system.
- Add Spire.XLS.dll as reference in the downloaded Bin folder thought the below path: "..\Spire.XLS\Bin\NET4.0\ Spire.XLS.dll".
- You can use the class PageSetup to set the print options.
Set print paper size:
By default, the paper size is A4; you can set the PaperSize property of the worksheet to set the print paper size you desired.
//set print paper size as A3 sheet.PageSetup.PaperSize = PaperSizeType.PaperA3;
Set Print Area:
By default, the print area means all areas of the worksheet that contain data. You can set the PrintArea property of the worksheet to set the print area you want.
//set print area from cell "B2" to cell "F8" sheet.PageSetup.PrintArea = "B2:F8";
Set Print Titles:
Spire.XLS allows you to designate row and column headers to repeat on all pages of a printed worksheet. To do so, use the PageSetup class' PrintTitleColumns and PrintTitleRows properties.
//Set column numbers A & B as title columns sheet.PageSetup.PrintTitleColumns = "$A:$B"; //Set row numbers 1 & 2 as title rows sheet.PageSetup.PrintTitleRows = "$1:$2";
Set Page Order:
The PageSetup class provides the Order property that is used to order multiple pages of your worksheet to be printed. There are two possibilities to order the pages as follows:
//set page order from down then over sheet.PageSetup.Order = OrderType.DownThenOver; //set page order from over then down sheet.PageSetup.Order = OrderType.OverThenDown;
Below picture shows the Microsoft Excel's page print options:
There are many reasons merging PDFs may be necessary. For example, merging PDF files allows you to print a single file rather than queueing several documents for the printer, combining related files simplifies the process of managing and storing many documents by reducing the number of files to search through and organize. In this article, you will learn how to merge multiple PDF documents into one PDF document and how to combine the selected pages from different PDF documents into one PDF in C# and VB.NET by using Spire.PDF for .NET.
Install Spire.PDF for .NET
To begin with, you need to add the DLL files included in the Spire.PDF for.NET package as references in your .NET project. The DLLs files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.PDF
Merge Multiple PDFs into a Single PDF
Spire.PDF for .NET offers the PdfDocument.MergeFiles() method to merge multiple PDF documents into a single document. The detailed steps are as follows.
- Get the paths of the documents to be merged and store them in a string array.
- Call PdfDocument.MergeFiles() method to merge these files.
- Save the result to a PDF document using PdfDocumentBase.Save() method.
- C#
- VB.NET
using System; using Spire.Pdf; namespace MergePDFs { class Program { static void Main(string[] args) { //Get the paths of the documents to be merged String[] files = new String[] { "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-1.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-2.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-3.pdf"}; //Merge these documents and return an object of PdfDocumentBase PdfDocumentBase doc = PdfDocument.MergeFiles(files); //Save the result to a PDF file doc.Save("output.pdf", FileFormat.PDF); } } }
Merge the Selected Pages of Different PDFs into One PDF
Spire.PDF for .NET offers the PdfDocument.InsertPage() method and the PdfDocument.InsertPageRange() method to import a page or a page range from one PDF document to another. The following are the steps to combine the selected pages from different PDF documents into a new PDF document.
- Get the paths of the source documents and store them in a string array.
- Create an array of PdfDocument, and load each source document to a separate PdfDocument object.
- Create another PdfDocument object for generating a new document.
- Insert the selected page or page range of the source documents to the new document using PdfDocument.InsertPage() method and PdfDocument.InsertPageRange() method.
- Save the new document to a PDF file using PdfDocument.SaveToFile() method.
- C#
- VB.NET
using System; using Spire.Pdf; namespace MergeSelectedPages { class Program { static void Main(string[] args) { //Get the paths of the documents to be merged String[] files = new String[] { "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-1.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-2.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-3.pdf"}; //Create an array of PdfDocument PdfDocument[] docs = new PdfDocument[files.Length]; //Loop through the documents for (int i = 0; i < files.Length; i++) { //Load a specific document docs[i] = new PdfDocument(files[i]); } //Create a PdfDocument object for generating a new PDF document PdfDocument doc = new PdfDocument(); //Insert the selected pages from different documents to the new document doc.InsertPage(docs[0], 0); doc.InsertPageRange(docs[1], 1,3); doc.InsertPage(docs[2], 0); //Save the document to a PDF file doc.SaveToFile("output.pdf"); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Compared with Excel files, text files are easier to read and take up less memory as they contain only plain text data without any formatting or complex structure. Therefore, in certain situations where simplicity and efficiency are required, converting Excel files to text files can be beneficial. This article will demonstrate how to programmatically convert Excel to TXT format using Spire.XLS for .NET.
Install Spire.XLS for .NET
To begin with, you need to add the DLL files included in the Spire.XLS for .NET package as references in your .NET project. The DLL files can be either downloaded from this link or installed via NuGet.
PM> Install-Package Spire.XLS
Convert Excel to TXT in C# and VB.NET
Spire.XLS for .NET offers the Worksheet.SaveToFile(string fileName, string separator, Encoding encoding) method to convert a specified worksheet to a txt file. The following are the detailed steps.
- Create a Workbook instance.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get a specified worksheet by its index using Workbook.Worksheets[sheetIndex] property.
- Convert the Excel worksheet to a TXT file using Worksheet.SaveToFile() method.
- C#
- VB.NET
using Spire.Xls; using System.Text; namespace ExcelToTXT { class Program { static void Main(string[] args) { //Create a Workbook instance Workbook workbook = new Workbook(); //Load a sample Excel file workbook.LoadFromFile("sample.xlsx"); //Get the first worksheet Worksheet sheet = workbook.Worksheets[0]; //Save the worksheet as a txt file sheet.SaveToFile("ExceltoTxt.txt", " ", Encoding.UTF8); } } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Convert PDF Page to Image with Specified Resolution
2013-11-15 08:01:20Spire.PDF is an easy-to-use and powerful .NET PDF library. It can do a lot of conversions, and one of them is converting PDF page to image. As to converting PDF page to image, it works conveniently and flexibly. It has 6 overloaded functions named SaveAsImage that can make sure you find one meeting your need.
You can use Spire.PDF to convert any specific page of PDF document to BMP and Metafile image. Check it here.
In this article, we will discuss conversion with specified resolution.
public Image SaveAsImage(int pageIndex, int dpiX, int dpiY)
- pageIndex: specify which page to convert, 0 indicates the first page.
- dpiX: specify the resolution of x coordinate axis in PDF page when converting.
- dpiX: specify the resolution of y coordinate axis in PDF page when converting.
Image image = documemt.SaveAsImage(0, PdfImageType.Bitmap, false, 400, 400)
In the sample code, the size of PDF page is Width = 612.0, Height = 792.0. We set the resolution to 400, 400. And we will get an image with width = 3400, height = 4400.
Here is sample code:
PdfDocument documemt = new PdfDocument(); documemt.LoadFromFile(@"..\..\EnglishText.pdf"); Image image = documemt.SaveAsImage(0, PdfImageType.Bitmap, false, 400, 400); image.Save(@"..\..\result.jpg"); documemt.Close();
Effect Screentshot:
Convert Word document to HTML is popular and widely used by programmers and developers. With the help of Spire.Doc for .NET, a professional word component, without installing MS Word, developers can convert word to html with only two lines of key code in C#. At the same time, Spire.Doc supports convert HTML to word document easily and quickly.
This article still focuses on convert word from/to HTML, while it mainly about the supports of embed image in the word document and HTML. With the improvements of Spire.Doc (starts from Spire.Doc V. 4.9.32), now it supports the new function of ImageEmbedded.
Please download Spire.Doc (version 4.9.32 or above) with .NET framework together and follow the simple steps as below:
Convert Word to HTML in C#:
Step 1: Create the word document.
Document document = new Document();
Step 2: Set the value of imageEmbedded attribute.
doc.HtmlExportOptions.ImageEmbedded=true;
Step 3: Save word document to HTML.
doc.SaveToFile("result.html",FileFormat.Html);
Spire.Doc also supports load the result HTML page and convert it into word document in only three lines of codes as below.
doc.SaveToFile("htmltoword.docx",FileFormat.Docx);
Besides conversion of word from/to HTML, Spire.Doc also supports Convert Word to PDF, Convert Word to Image and Convert Word to XPS in C#.
For the function of converting image to PDF, Spire.PDF can handle it quickly and effectively. This .NET PDF library can not only convert images of commonly used formats to PDF document such as jpg, bmp, png, but also convert gif, tif and ico images to PDF. Just download it here.
To convert multipage image to a PDF file with Spire.PDF, just copy the following code to your application and call method ConvertImagetoPDF and you will get it done.
Step 1: Method to split multipage image
Spire.Pdf has a method called DrawImage to convert image to PDF. But it cannot handle multipage image directly. So before conversion, multipage image need to be split into several one-page images.
Guid guid = image.FrameDimensionsList[0]; FrameDimension dimension = new FrameDimension(guid); int pageCount = image.GetFrameCount(dimension);
This step is to get the total number of frames (pages) in the multipage image.
image.SelectActiveFrame(dimension, i);
And this step is to select one frame of frames within this image object.
image.Save(buffer, format);
Save the selected frame to the buffer.
Step 2: Convert image to PDF
After splitting multipage image, Spire.Pdf can draw these split images directly to PDF using method DrawImage.
PdfImage pdfImg = PdfImage.FromImage(img[i])
Load image file as PdfImage.
page.Canvas.DrawImage(pdfImg, x, 0, width, height);
Draw PdfImage to PDF. The only thing to do is to specify the location of image on PDF. Width and height is the size of area that image will be drawn on. Sometimes we need to scale up or down the size of the original size of image until it fit the PDF page. x and 0 locate the coordinate.
Check the effective screenshots for the original TIF file.
The target PDF file:
Full demo:
using Spire.Pdf; using Spire.Pdf.Graphics; using System; using System.Drawing; using System.Drawing.Imaging; using System.IO; namespace ConvertMultipageImagetoPDF { class Program { static void Main(string[] args) { { ConvertImagetoPDF(@"..\..\Chapter1.tif"); } } public static void ConvertImagetoPDF(String ImageFilename) { using (PdfDocument pdfDoc = new PdfDocument()) { Image image = Image.FromFile(ImageFilename); Image[] img = SplitImages(image, ImageFormat.Png); for (int i = 0; i < img.Length; i++) { PdfImage pdfImg = PdfImage.FromImage(img[i]); PdfPageBase page = pdfDoc.Pages.Add(); float width = pdfImg.Width * 0.3f; float height = pdfImg.Height * 0.3f; float x = (page.Canvas.ClientSize.Width - width) / 2; page.Canvas.DrawImage(pdfImg, x, 0, width, height); } string PdfFilename = "result.pdf"; pdfDoc.SaveToFile(PdfFilename); System.Diagnostics.Process.Start(PdfFilename); } } public static Image[] SplitImages(Image image, ImageFormat format) { Guid guid = image.FrameDimensionsList[0]; FrameDimension dimension = new FrameDimension(guid); int pageCount = image.GetFrameCount(dimension); Image[] frames = new Image[pageCount]; for (int i = 0; i < pageCount; i++) { using (MemoryStream buffer = new MemoryStream()) { image.SelectActiveFrame(dimension, i); image.Save(buffer, format); frames[i] = Image.FromStream(buffer); } } return frames; } } }
The article will introduce an easy way to convert Word to Emf by a powerful and independent Word .NET component called Spire.Doc, without Microsoft Word installed on the machine. It also offers support for converting Word and HTML to frequently-used image formats like Jpeg, Png, Gif, Bmp and Tiff, etc. Just click here to have a try.
Emf is a file extension for Enhanced MetaFile, used as a graphics language for printer drivers by the Windows operating system. In 1993, a newer version with additional commands 32-bit version of Win32/GDI introduced the Enhanced Metafile (Emf). Microsoft also recommends enhanced-format (Emf) functions to be used instead of rarely being used Windows-format (WMF) functions.
Spire.Doc presents almost the easiest solution to convert Word to Emf through the following 5 lines simple code.
using Spire.Doc; using System.Drawing.Imaging; namespace DOCEMF { class Program { static void Main(string[] args) { // create an instance of Spire.Doc.Document Document doc = new Document(); // load the file base on a specified file name doc.LoadFromFile(@"../../Original Word.docx", FileFormat.Docx); //convert the first page of document to image System.Drawing.Image image = doc.SaveToImages(0, Spire.Doc.Documents.ImageType.Metafile); // save the document object to Emf file image.Save(@"../../Convert Word to Image.emf", ImageFormat.Emf); //close the document doc.Close(); } } }
Check the effect screenshot below:
Converting HTML to PDF with C# PDF component is so important that we always try our best to improve our Spire.PDF better and better. We aim to make it is much more convenient for our developers to use. Now besides the previous method of converting HTML to PDF offered by Spire.PDF, we have a new plugin for html conversion to PDF. This section will focus on the new plugin of convert HTML to PDF. With this new plugin, we support to convert the HTML page with rich elements, such as HTTPS, CSS3, HTML5, JavaScript.
You need to download Spire.PDF and install it on your system, add Spire.PDF.dll as reference in the downloaded Bin folder thought the below path '..\Spire.PDF\Bin\NET4.0\Spire.PDF.dll'. And for gain the new plugin, you could get the new plugin from the download file directly: windows-x86.zip windows-x64.zip macosx_x64.zip linux_x64.tar.gz .
On Windows system, you need to unzip the convertor plugin package and copy the folder 'plugins' under the same folder of Spire.Pdf.dll. Before you use QT plugin for converting HTML to PDF, please ensure you have installed Microsoft Visual C++ 2015 Redistributable on your computer.
On Mac and Linux system, you need to copy the zip file under the system and then unzip the convertor plugin package there to use the plugins successfully.
C# HtmlToPdf.zip and VB.NET HtmlToPdfVB.zip, you could download and try it.
Calling the plugins is very simple, please check the below C# code for convert HTML to PDF.
using System.Drawing; using Spire.Pdf.Graphics; using Spire.Pdf.HtmlConverter.Qt; namespace SPIREPDF_HTMLtoPDF { class Program { static void Main(string[] args) { HtmlConverter.Convert("http://www.wikipedia.org/", "HTMLtoPDF.pdf", //enable javascript true, //load timeout 100 * 1000, //page size new SizeF(612, 792), //page margins new PdfMargins(0, 0)); System.Diagnostics.Process.Start("HTMLtoPDF.pdf"); } } }
Imports System.Drawing Imports Spire.Pdf.Graphics Imports Spire.Pdf.HtmlConverter.Qt Module Module1 Sub Main() HtmlConverter.Convert("http://www.wikipedia.org/", "HTMLtoPDF.pdf", True, 100 * 1000, New SizeF(612, 792), New PdfMargins(0, 0)) System.Diagnostics.Process.Start("HTMLtoPDF.pdf") End Sub End Module
Please check the effective screenshot as below:
The following sample will focus on the new plugin of convert HTML string to PDF.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Spire.Pdf; using System.IO; using Spire.Pdf.HtmlConverter; using System.Drawing; namespace HTMLToPDFwithPlugins { class Program { static void Main(string[] args) { string input =@"<strong>This is a test for converting HTML string to PDF </strong> <ul><li>Spire.PDF supports to convert HTML in URL into PDF</li> <li>Spire.PDF supports to convert HTML string into PDF</li> <li>With the new plugin</li></ul>"; string outputFile = "ToPDF.pdf"; Spire.Pdf.HtmlConverter.Qt.HtmlConverter.Convert(input, outputFile, //enable javascript true, //load timeout 10 * 1000, //page size new SizeF(612, 792), //page margins new Spire.Pdf.Graphics.PdfMargins(0), //load from content type LoadHtmlType.SourceCode ); System.Diagnostics.Process.Start(outputFile); } } }
Effective screenshot:
The aim of the article is to introduce the procedure of exporting data into Office OpenXML in only two steps with a .net component. Spire.DataExport is a completely pure .NET component suit for exporting data into MS Excel, MS Word, HTML, Office OpenXML, PDF, MS Access, DBF, RTF, SQL Script, SYLK, DIF, CSV, MS Clipboard format. It has high performance for exporting data from Command, ListView, DataTable components, which help you to save much time and money.
Please download Spire.DataExport for .NET, add Spire.DataExport.dll as reference and set its target framework as .NET 4. Besides, many developers also check and download another C# excel component together - Spire.XLS for .NET.
Step1: Function to fill data in datatable
In this step, Spire.DataExport will help to load Data information from the datatable. After setting up the data source and SQL command, we can even preview and modify data through DataGridVew before exporting.
private void Form1_Load(object sender, EventArgs e) { oleDbConnection1.ConnectionString = txtConnectString.Text; oleDbCommand1.CommandText = txtCommandText.Text; using (OleDbDataAdapter da = new OleDbDataAdapter()) { da.SelectCommand = oleDbCommand1; da.SelectCommand.Connection = oleDbConnection1; DataTable dt = new DataTable(); da.Fill(dt); dataGridView1.DataSource = dt; } }
Check the Screenshot below:
Step2: Export Data to Office OpenXML
The code below shows how to export data from the datatable to Office OpenXML. Spire.DataExport will create a new Office OpenXML and export data into Office OpenXML through DataGridView. It also allows you to rename the generated Office OpenXML in this step.
private void btnExportToXml_Click(object sender, EventArgs e) { Spire.DataExport.XML.XMLExport xmlExport = new Spire.DataExport.XML.XMLExport(); xmlExport.DataSource = Spire.DataExport.Common.ExportSource.DataTable; xmlExport.DataTable = this.dataGridView1.DataSource as DataTable; xmlExport.ActionAfterExport = Spire.DataExport.Common.ActionType.OpenView; xmlExport.FileName = @"..\..\ToXml.xml"; xmlExport.SaveToFile(); }
Check the Screenshot below:
This article will show you a clear introduction of how to Export Data to MS Access in C# via a .NET Data Export component. Spire.DataExport for.NET is designed to help developers to perform data exporting processing tasks. With Spire.DataExport, the whole exporting process is quickly and it only needs two simple steps.
Please download Spire.DataExport for .NET and install it on your system, add Spire.DataExport.dll as reference in the downloaded Bin folder thought the below path: “…\Spire.DataExport\Bin\NET4.0\ Spire.DataExport.dll”.
Step 1: Load Data Information
In this step, Spire.DataExport helps us load data from database. Through DataGridVew, we can even preview and modify data.
private void Form1_Load(object sender, EventArgs e) { oleDbConnection1.ConnectionString = txtConnectString.Text; oleDbCommand1.CommandText = txtCommandText.Text; using (OleDbDataAdapter da = new OleDbDataAdapter()) { da.SelectCommand = oleDbCommand1; da.SelectCommand.Connection = oleDbConnection1; DataTable dt = new DataTable(); da.Fill(dt); dataGridView1.DataSource = dt; } }
Please check the screenshot:
Step 2: Set Export into MS Access
Here we need to set it as Access format. Spire.DataExport will create a new Access and through DataGridView export data into Access file. You can rename the file as you like.
private void btnExportToAccess_Click(object sender, EventArgs e) { Spire.DataExport.Access.AccessExport accessExport = new Spire.DataExport.Access.AccessExport(); accessExport.DataSource = Spire.DataExport.Common.ExportSource.DataTable; accessExport.DataTable = this.dataGridView1.DataSource as DataTable; accessExport.DatabaseName = @"..\..\ToMdb.mdb"; accessExport.TableName = "ExportFromDatatable"; accessExport.SaveToFile(); }
Here comes to the results:
