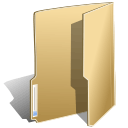
Conversion (11)
Charts and shapes in Excel are vital tools for clear and effective data presentation. Sometimes, it's beneficial to convert these visual elements into images. Perhaps you need to include a specific chart in a report or presentation outside of Excel. Or maybe you want to use an Excel-created infographic on your company's website. Regardless of the use case, knowing how to export these visuals as standalone image files can be invaluable. In this guide, we will explore how to convert charts and shapes in Excel to images in Python using Spire.XLS for Python.
- Convert a Specific Chart in an Excel Worksheet to Image in Python
- Convert All Charts in an Excel Worksheet to Images in Python
- Convert a Chart Sheet in Excel to Image in Python
- Convert Shapes in Excel to Images in Python
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert a Specific Chart in an Excel Worksheet to Image in Python
Spire.XLS for Python offers the Workbook.SaveChartAsImage(worksheet: Worksheet, chartIndex: int) method, allowing you to convert a specific chart within a worksheet into an image stream. This image stream can then be saved as an image file in various formats, including PNG, JPG, BMP, and more. The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an Excel file using the Workbook.LoadFromFile() method.
- Get a specific worksheet in the file using the Workbook.Worksheets[] property.
- Save a specific chart in the worksheet to an image stream using the Workbook.SaveChartAsImage(worksheet: Worksheet, chartIndex: int) method.
- Save the image stream to an image file using the Stream.Save() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Charts.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] # Save the first chart in the worksheet to an image stream image_stream = workbook.SaveChartAsImage(sheet, 0) # Save the image stream to a PNG image file image_stream.Save("Output/chart.png") workbook.Dispose()
Convert All Charts in an Excel Worksheet to Images in Python
To convert all charts in an Excel worksheet to images, you can use the Workbook.SaveChartAsImage(worksheet: Worksheet) method. The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an Excel file using the Workbook.LoadFromFile() method.
- Get a specific worksheet in the file using the Workbook.Worksheets[] property.
- Save all charts in the worksheet to a list of image streams using the Workbook.SaveChartAsImage(worksheet: Worksheet) method.
- Iterate through the image streams in the list and save them to separate image files.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Charts.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] image_streams = [] # Save the charts in the worksheet to a list of image streams image_streams = workbook.SaveChartAsImage(sheet) # Save the image streams to PNG image files for i, image_stream in enumerate(image_streams): image_stream.Save(f"Output/chart-{i}.png") workbook.Dispose()
Convert a Chart Sheet in Excel to Image in Python
In Microsoft Excel, a chart sheet is a special type of sheet that is dedicated to displaying a single chart or graph. You can convert a chart sheet in an Excel workbook to an image using the Workbook.SaveChartAsImage(chartSheet: ChartSheet) method. The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an Excel file using the Workbook.LoadFromFile() method.
- Get a specific chart sheet in the file using the Workbook.Chartsheets[] property.
- Save the chart sheet to an image stream using the Workbook.SaveChartAsImage(chartSheet: ChartSheet) method.
- Save the image stream to an image file.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("ChartSheet.xlsx") # Get the first chart sheet chart_sheet = workbook.Chartsheets[0] # Save the chart sheet to an image stream image_stream = workbook.SaveChartAsImage(chart_sheet) # Save the image stream to a PNG image file image_stream.Save("Output/chartSheet.png") workbook.Dispose()
Convert Shapes in Excel to Images in Python
In addition to converting charts or chart sheets to images, you can also convert shapes in an Excel worksheet to images by using the XlsShape.SaveToImage() method. The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an Excel file using the Workbook.LoadFromFile() method.
- Get a specific worksheet in the file using the Workbook.Worksheets[] property.
- Iterate through all the shapes in the worksheet.
- Typecast the shape to an XlsShape object.
- Save the shape to an image stream using the XlsShape.SaveToImage() method.
- Save the image stream to an image file.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Shapes.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] # Iterate through all the shapes in the worksheet for i, shape in enumerate(sheet.PrstGeomShapes): xls_shape = XlsShape(shape) # Save the shape to an image stream image_stream = shape.SaveToImage() # Save the image stream to a PNG image file image_stream.Save(f"Output/shape_{i}.png") workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Python: Convert Excel to ODS, XPS, PostScript and PDF/A-1b
2024-07-30 00:49:07 Written by support iceblueConverting XLS files to various formats is necessary for data management and presentation. ODS, XPS, PostScript, and PDF/A-1b offer unique advantages and are suitable for different scenarios.
ODS is widely used for compatibility with many office suites. XPS preserves document fidelity and is ideal for sharing and archiving. PostScript is a versatile page description language often used for printing and graphic design. PDF/A-1b ensures long-term archiving by complying with strict preservation standards.
This guide will illustrate how to convert Excel to ODS, XPS, PostScript, and PDF/A-1b with Python using Spire.XLS for Python, leveraging their specific strengths to meet diverse needs.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install it, please refer to this tutorial: How to Install Spire.XLS for Python on Windows.
Convert Excel to ODS, XPS, and PostScript with Python
To convert Excel to ODS, XPS, and PostScript documents, you can utilize Workbook.SaveToFile() method. It supports converting CSV to Excel and PDF, Excel to PDF and XLSX, etc. By using this method provided by Spire.XLS for Python, you can seamlessly transform your documents into these formats while maintaining accuracy without data loss. Read the following steps to learn more:
Steps to convert Excel to ODS, XPS, and PostScript:
- Create a new Workbook object.
- Import the file to be converted from the disk using Workbook.LoadFromFile() method.
- Convert it to ODS, XPS, or PostScript with Workbook.SaveToFile() method.
Here is the code example for reference:
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load the file from the disk workbook.LoadFromFile("sample.xlsx") # Save the document to an ODS file workbook.SaveToFile("to_ods.ods", FileFormat.ODS) # Save the document as an XPS file workbook.SaveToFile("to_xps.xps", FileFormat.XPS) # Save the document as a PostScript file workbook.SaveToFile("to_postscript.ps", FileFormat.PostScript) workbook.Dispose()
Note: Images 1, 2, and 3 show the results of converting Excel files to ODS, XPS, and PostScript formats, respectively.
How to Convert Excel Documents to PDF/A-1b Format
If you need to convert Excel to PDF/A-1b Format with Python, call Workbook.SaveToFile will help you. The steps to transform Excel documents to PDF/A-1b are similar to those above, except the former involves an additional step. This tutorial will guide you through the process with detailed steps and a code example.
Steps to convert Excel to PDF/A-1b
- Instantiate a new Workbook object.
- Read the Excel document from the disk using Workbook.LoadFromFile() method.
- Set the PDF conformance level to PDF/A-1b.
- Save the generated document as PDF with Workbook.SaveToFile() method.
Here is the code example for you:
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Open the file from the disk workbook.LoadFromFile("sample.xlsx") # Set the PDF conformance to PDF/A-1b workbook.ConverterSetting.PdfConformanceLevel = PdfConformanceLevel.Pdf_A1B # Convert the Excel document to PDF/A-1b workbook.SaveToFile("to_pdfa1b", FileFormat.PDF) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Excel has been a widely used tool for data organization and analysis for many years. Over time, Microsoft has introduced different file formats for storing Excel data, the most common being the older XLS format and the more modern XLSX format.
The XLS format, introduced in the late 1990s, had certain limitations, such as a file size limit of 65,536 rows and 256 columns, and a maximum of 65,000 unique styles. The XLSX format, introduced in 2007, addressed these limitations by allowing for larger file sizes, more rows and columns, and expanded style capabilities. While XLSX is now the standard format, there are still many existing XLS files that need to be accessed and used, which makes the ability to convert between these formats an essential skill. In this article, we will explain how to convert Excel XLS to XLSX and vice versa in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert XLSX to XLS in Python
To convert an XLSX file to XLS format, you can use the Workbook.SaveToFile(fileName, ExcelVersion.Version97to2003) method. The ExcelVersion.Version97to2003 parameter specifies that the workbook should be saved in the Excel 97-2003 (XLS) format. The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an XLSX file using the Workbook.LoadFromFile() method.
- Save the XLSX file to XLS format using the Workbook.SaveToFile(fileName, ExcelVersion.Version97to2003) method.
- Python
from spire.xls import * from spire.xls.common import * # Specify the input and output file paths inputFile = "Sample1.xlsx" outputFile = "XlsxToXls.xls" # Create a Workbook object workbook = Workbook() # Load the XLSX file workbook.LoadFromFile(inputFile) # Save the XLSX file to XLS format workbook.SaveToFile(outputFile, ExcelVersion.Version97to2003) workbook.Dispose()
Convert XLS to XLSX in Python
To convert an XLS file to XLSX format, you need to specify the target Excel version to a version higher than 97-2003, such as 2007 (ExcelVersion.Version2007), 2010 (ExcelVersion.Version2010), 2013 (ExcelVersion.Version2013), or 2016 (ExcelVersion.Version2016). The detailed steps are as follows.
- Create an object of the Workbook class.
- Load an XLS file using the Workbook.LoadFromFile() method.
- Save the XLS file to an Excel 2016 (XLSX) file using the Workbook.SaveToFile(fileName, ExcelVersion.Version2016) method.
- Python
from spire.xls import * from spire.xls.common import * # Specify the input and output file paths inputFile = "Sample2.xls" outputFile = "XlsToXlsx.xlsx" # Create a Workbook object workbook = Workbook() # Load the XLS file workbook.LoadFromFile(inputFile) # Save the XLS file to XLSX format workbook.SaveToFile(outputFile, ExcelVersion.Version2016) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Text files have a distinct advantage over Excel spreadsheets in terms of simplicity as they don't contain complex formatting, macros or formulas. This streamlined nature not only enhances portability, but also reduces the possibility of file corruption. Consequently, converting Excel files to text files can greatly facilitates data parsing and ensures compatibility with various applications. In this article, you will learn how to convert Excel to TXT text file in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert Excel to TXT in Python
Spire.XLS for Python offers the Worksheet.SaveToFile(fileName: str, separator: str, encoding: Encoding) method to convert a specified worksheet to a TXT text file. The three parameters represent:
- fileName: Specifies the path and the name of the output text file.
- separator: Specifies the separator for the output text file. Common separators include commas (,), tabs, semicolons (;), etc.
- encoding: Specifies the encoding format of the file, e.g. UTF-8, Unicode, ASCII, etc. You need to use the correct encoding format to ensure that the text is represented and interpreted correctly.
The following are the detailed steps to convert Excel to text files in Python.
- Create a Workbook instance.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get a specified worksheet by its index using Workbook.Worksheets[sheetIndex] property.
- Convert the Excel worksheet to a TXT file using Worksheet.SaveToFile() method.
- Python
import os import sys curPath = os.path.abspath(os.path.dirname(__file__)) rootPath = os.path.split(curPath)[0] sys.path.append(rootPath) from spire.xls import * from spire.xls.common import * inputFile = "Inventories.xlsx" outputFile = "ExceltoTxt.txt" # Create a Workbook instance workbook = Workbook() # Load an Excel document from disk workbook.LoadFromFile(inputFile) # Get the first worksheet sheet = workbook.Worksheets[0] # Save the worksheet as a txt file sheet.SaveToFile(outputFile, " ", Encoding.get_UTF8()) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
SVG (Scalable Vector Graphics) is a flexible file format widely used on the web. Unlike traditional image formats, SVG files are not based on pixels. Instead, they use mathematical equations to define shapes, lines, and colors. This unique characteristic allows SVG files to be scaled up or down without any loss of quality, making them an excellent choice for creating interactive and visually appealing graphics. By converting Excel files to SVG, you can seamlessly embed the resulting SVG files into web pages, ensuring smooth integration and display of your Excel data on the web. In this article, we will demonstrate how to convert Excel to SVG format in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert a Worksheet in Excel to SVG in Python
Spire.XLS for Python provides the Worksheet.ToSVGStream() method to convert an Excel worksheet to SVG. The detailed steps are as follows:
- Create an object of the Workbook class.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet by its index through Workbook.Worksheets[] property.
- Create an object of the Stream class.
- Save the worksheet to an SVG using Worksheet.ToSVGStream() method.
- Python
from spire.xls.common import * from spire.xls import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Sample1.xlsx") # Get the first worksheet worksheet = workbook.Worksheets[0] # Save the worksheet to an SVG stream = Stream("WorksheetToSVG.svg") worksheet.ToSVGStream(stream, 0, 0, 0, 0) stream.Flush() stream.Close() workbook.Dispose()
Convert a Chart Sheet in Excel to SVG in Python
A chart sheet in Excel is a separate sheet within an Excel workbook that is dedicated to displaying a chart. Spire.XLS for Python allows you to convert a chart sheet to SVG by using the ChartSheet.ToSVGStream() method. The detailed steps are as follows:
- Create an object of the Workbook class.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific chart sheet using Workbook.GetChartSheetByName() method.
- Create an object of the Stream class.
- Save the chart sheet to an SVG using ChartSheet.ToSVGStream() method.
- Python
from spire.xls.common import * from spire.xls import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Sample2.xlsx") # Get a specific chart sheet chartSheet = workbook.GetChartSheetByName("Chart1") # Save the chart sheet to an SVG stream = Stream("ChartSheetToSVG.svg") chartSheet.ToSVGStream(stream) stream.Flush() stream.Close() workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Python: Convert Excel to Open XML or Open XML to Excel
2023-12-27 01:21:07 Written by support iceblueIn the context of Excel, Open XML refers to the underlying file format used by Excel to store spreadsheet data, formatting, formulas, and other related information. It provides a powerful and flexible basis for working with Excel files programmatically.
By converting Excel to Open XML, developers gain greater control and automation when working with spreadsheet-related tasks. In turn, you can also generate Excel files from Open XML to take advantage of Excel's built-in capabilities to perform advanced data operations. In this article, you will learn how to convert Excel to Open XML or Open XML to Excel in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert Excel to Open XML in Python
Spire.XLS for Python offers the Workbook.SaveAsXml() method to save an Excel file in Open XML format. The following are the detailed steps.
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Save the Excel file in Open XML format using Workbook.SaveAsXml() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("sample.xlsx") # Save the Excel file in Open XML file format workbook.SaveAsXml("ExcelToXML.xml") workbook.Dispose()
Convert Open XML to Excel in Python
To convert an Open XML file to Excel, you need to load the Open XML file through the Workbook.LoadFromXml() method, and then call the Workbook.SaveToFile() method to save it as an Excel file. The following are the detailed steps.
- Create a Workbook object.
- Load an Open XML file using Workbook.LoadFromXml() method.
- Save the Open XML file to Excel using Workbook.SaveToFile() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Open XML file workbook.LoadFromXml("ExcelToXML.xml") # Save the Open XML file to Excel XLSX format workbook.SaveToFile("XMLToExcel.xlsx", ExcelVersion.Version2016) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
If you need to display or interact with the contents of an Excel spreadsheet on a web page, converting Excel to HTML is a good choice. This conversion allows users to view and manipulate the table data directly on the web page without having to download the Excel file, providing a more convenient way to share and display the data. When needed, you can also convert the HTML file back to Excel format for better data editing. In this article, we will show you how to convert Excel to HTML and HTML to Excel in Python by using Spire.XLS for Python.
- Convert Excel to HTML in Python
- Convert Excel to HTML with Images Embedded in Python
- Convert HTML to Excel in Python
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert Excel to HTML in Python
Spire.XLS for Python supports converting a specific Excel worksheet to HTML using Worksheet.SaveToHtml() method. Detailed steps are listed below.
- Create a Workbook instance.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet using Workbook.Worksheets[] property.
- Save the worksheet as an HTML file using Worksheet.SaveToHtml() method.
- Python
from spire.xls import * from spire.xls.common import * inputFile = "C:/Users/Administrator/Desktop/Sample_1.xlsx" outputFile = "C:/Users/Administrator/Desktop/ToHtml.html" # Create a Workbook instance workbook = Workbook() # Load a sample Excel file workbook.LoadFromFile(inputFile) # Get the first sheet of this file sheet = workbook.Worksheets[0] # Save the worksheet to HTML sheet.SaveToHtml(outputFile) workbook.Dispose()
Convert Excel to HTML with Images Embedded in Python
If the Excel file you want to convert contains images, you can embed the images into the HTML file by setting the ImageEmbedded property to "True". Detailed steps are listed below.
- Create a Workbook instance.
- Load a sample Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet using Workbook.Worksheets[] property.
- Create an HTMLOptions instance.
- Set the ImageEmbedded as “True” to embed images to HTML.
- Save the worksheet as an HTML file using Worksheet.SaveToHtml() method.
- Python
from spire.xls import * from spire.xls.common import * inputFile = "C:/Users/Administrator/Desktop/Sample_2.xlsx" outputFile = "C:/Users/Administrator/Desktop/ToHtmlwithImages.html" # Create a Workbook instance workbook = Workbook() # Load a sample Excel file workbook.LoadFromFile(inputFile) # Get the first sheet of this file sheet = workbook.Worksheets[0] # Create an HTMLOptions instance options = HTMLOptions() # Embed images to HTML options.ImageEmbedded = True # Save the worksheet to HTML sheet.SaveToHtml(outputFile, options) workbook.Dispose()
Convert HTML to Excel in Python
You are also allowed to convert an HTML back to an Excel file by calling the Workbook.SaveToFile() method provided by Spire.XLS for Python. Detailed steps are listed below.
- Create a Workbook instance.
- Load an HTML file from disk using Workbook.LoadFromFile() method.
- Save the HTML file to an Excel file by using Workbook.SaveToFile() method.
- Python
from spire.xls import * from spire.xls.common import * inputFile = "C:/Users/Administrator/Desktop/Sample.html" outputFile = "C:/Users/Administrator/Desktop/ToExcel.xlsx" # Create a Workbook instance workbook = Workbook() # Load an HTML file from disk workbook.LoadFromHtml(inputFile) # Save the HTML file to an Excel file workbook.SaveToFile(outputFile, ExcelVersion.Version2013) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
A CSV (Comma-Separated Values) file is a plain text file used to store tabular data. Although CSV files are widely supported by spreadsheet programs, there may still be times when you need to convert them to PDF files to ensure broader accessibility and also enable security features. This article will demonstrate how to convert CSV to PDF in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert CSV to PDF in Python
The Workbook.SaveToFile() method provided by Spire.XLS for Python allows to save a CSV file as a PDF file. The following are the detailed steps.
- Create a Workbook object.
- Load a CSV file using Workbook.LoadFromFile() method.
- Set the Workbook.ConverterSetting.SheetFitToPage property as true to ensure the worksheet is rendered to one PDF page.
- Get the first worksheet in the Workbook using Workbook.Worksheets[] property.
- Loop through the columns in the worksheet and auto-fit the width of each column using Worksheet.AutoFitColumn() method.
- Convert the CSV file to PDF using Workbook.SaveToFile() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load a CSV file workbook.LoadFromFile("sample.csv", ",", 1, 1) # Set the SheetFitToPage property as true workbook.ConverterSetting.SheetFitToPage = True # Get the first worksheet sheet = workbook.Worksheets[0] # Autofit columns in the worksheet i = 1 while i < sheet.Columns.Length: sheet.AutoFitColumn(i) i += 1 # Save the CSV file to PDF workbook.SaveToFile("CSVToPDF.pdf", FileFormat.PDF) workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Converting Excel spreadsheets to image formats can be extremely valuable and versatile in a wide range of situations. Whether you need to share data with others who don’t have Excel installed on their devices, present information in a document or presentation, or publish content online, converting Excel to image format offers a convenient solution. In this article, we will introduce how to programmatically convert Excel to images in Python using Spire.XLS for Python.
- Convert an Excel Worksheet to an Image in Python
- Convert an Excel Worksheet to an Image without White Margins in Python
- Convert a Specific Cell Range to an Image in Python
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert an Excel Worksheet to an Image in Python
You can easily convert a whole Excel worksheet to an image by using the Worksheet.SaveToImage() method provided by Spire.XLS for Python. The detailed steps are as follows:
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet by its index using Workbook.Worksheets[int index] property.
- Convert the worksheet to an image using Worksheet.ToImage() method.
- Save the image to a PNG file (you can also save the image as other image formats such as JPG and BMP).
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Sample.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] # Save the worksheet to an image image = sheet.ToImage(sheet.FirstRow, sheet.FirstColumn, sheet.LastRow, sheet.LastColumn) # Save the image to a PNG file image.Save("SheetToImage.png") workbook.Dispose()
Convert an Excel Worksheet to an Image without White Margins in Python
When converting an Excel worksheet to an image, you may find the resulting image has unwanted white margins surrounding the cells. If you want to convert the worksheet to an image without any extraneous margins, you can remove the page margins set in the original worksheet. The detailed steps are as follows:
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet by its index using Workbook.Worksheets[int index] property.
- Remove all margins from the worksheet by setting its left, right, top, and bottom margin values to zero.
- Convert the worksheet to an image using Worksheet.ToImage() method.
- Save the image to a PNG file.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Sample.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] # Set all margins of the worksheet to zero sheet.PageSetup.LeftMargin = 0 sheet.PageSetup.BottomMargin = 0 sheet.PageSetup.TopMargin = 0 sheet.PageSetup.RightMargin = 0 # Convert the worksheet to an image image = sheet.ToImage(sheet.FirstRow, sheet.FirstColumn, sheet.LastRow, sheet.LastColumn) # Save the image to a PNG file image.Save("SheetToImageWithoutMargins.png") workbook.Dispose()
Convert a Specific Cell Range to an Image in Python
In addition to converting a whole worksheet to an image, Spire.XLS for Python also supports converting a specific cell range of a worksheet to an image. The detailed steps are as follows:
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet by its index using Workbook.Worksheets[int index] property.
- Convert a specific cell range of the worksheet to an image using Worksheet.ToImage() method and pass the index of the start row, start column, end row, and end column of the cell range to the method as parameters.
- Save the image to a PNG file.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel file workbook.LoadFromFile("Sample.xlsx") # Get the first worksheet sheet = workbook.Worksheets[0] # Convert a specific cell range of the worksheet to an image image = sheet.ToImage(5, 2, 17, 5) # Save the image to a PNG file image.Save("CellRangeToImage.png") workbook.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Excel spreadsheets are widely used for organizing and analyzing data, while CSV files offer a simpler and more universal format for data exchange. The process of converting between these formats allows for greater flexibility in working with data across different platforms and applications. In this article, you will learn how to convert Excel to CSV and vice versa in Python using Spire.XLS for Python.
Install Spire.XLS for Python
This scenario requires Spire.XLS for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.XLS
If you are unsure how to install, please refer to this tutorial: How to Install Spire.XLS for Python on Windows
Convert Excel to CSV in Python
Spire.XLS for Python offers the Worksheet.SaveToFile() method, allowing users to export a certain worksheet within a workbook as an individual CSV file. The detailed steps to convert an Excel worksheet to CSV are as follows.
- Create a Workbook object.
- Load an Excel file using Workbook.LoadFromFile() method.
- Get a specific worksheet through Workbook.Worksheets[index] property.
- Convert the worksheet to a CSV file using Worksheet.SaveToFile() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load an Excel document workbook.LoadFromFile("C:\\Users\\Administrator\\Desktop\\sample.xlsx") # Get the first sheet sheet = workbook.Worksheets[0] # Convert it to CSV file sheet.SaveToFile("output/ToCSV.csv", ",", Encoding.get_UTF8()) workbook.Dispose()
Convert CSV to Excel in Python
The Workbook.LoadFromFile() method provided by Spire.XLS for Python can not only load Excel but also CSV files. After a CSV file is loaded, it can be saved as an Excel document using Workbook.SaveToFile() method. The following are the detailed steps to convert CSV to Excel using Spire.XLS for Python.
- Create a Workbook object.
- Load a CSV file using Workbook.LoadFromFile() method.
- Convert the workbook to an Excel file using Workbook.SaveToFile() method.
- Python
from spire.xls import * from spire.xls.common import * # Create a Workbook object workbook = Workbook() # Load a CSV file workbook.LoadFromFile("C:\\Users\\Administrator\\Desktop\\CSV.csv", ",", 1, 1) # Get the first worksheet sheet = workbook.Worksheets[0] # Display numbers as text sheet.AllocatedRange.IgnoreErrorOptions = IgnoreErrorType.NumberAsText # Autofit column width sheet.AllocatedRange.AutoFitColumns() # Save to an Excel file workbook.SaveToFile("output/ToExcel.xlsx", ExcelVersion.Version2013)
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
More...
