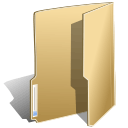
Page Background (1)
Adding background colors or pictures to your Word documents is a powerful way to enhance their visual appeal and captivate your audience. Whether you're creating a professional report, a creative flyer, or a personal invitation, incorporating a well-chosen background color or image can transform an ordinary document into a visually captivating piece. In this article, we will demonstrate how to add a background color or picture to a Word document in Python using Spire.Doc for Python.
- Add a Background Color to Word in Python
- Add a Gradient Background to Word in Python
- Add a Background Picture to Word in Python
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Add a Background Color to Word in Python
You can set a background color for a Word document by changing its background type to "Color" and then selecting a color as the background. The detailed steps are as follows.
- Create a Document object.
- Load a Word document using Document.LoadFromFile() method.
- Get the background of the document using Document.Background property.
- Set the background type as Color using Background.Type property.
- Set a color as the background using Background.Color property.
- Save the resulting document using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load a Word document document.LoadFromFile("Sample.docx") # Get the document's background background = document.Background # Set the background type as Color background.Type = BackgroundType.Color # Set the background color background.Color = Color.get_AliceBlue() #save the resulting document document.SaveToFile("AddBackgroundColor.docx", FileFormat.Docx2016) document.Close()
Add a Gradient Background to Word in Python
A gradient background refers to a background style that transitions smoothly between two or more colors. To add a gradient background, you need to change the background type as "Gradient", specify the gradient colors and then set the gradient shading variant and style. The detailed steps are as follows.
- Create a Document object.
- Load a Word document using Document.LoadFromFile() method.
- Get the background of the document using Document.Background property.
- Set the background type as Gradient using Background.Type property.
- Set two gradient colors using Background.Gradient.Color1 and Background.Gradient.Color2 properties.
- Set gradient shading variant and style using Background.Gradient.ShadingVariant and Background.Gradient.ShadingStyle properties.
- Save the resulting document using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load a Word document document.LoadFromFile("Sample.docx") # Get the document's background background = document.Background # Set the background type as Gradient background.Type = BackgroundType.Gradient # Set two gradient colors background.Gradient.Color1 = Color.get_White() background.Gradient.Color2 = Color.get_LightBlue() # Set gradient shading variant and style background.Gradient.ShadingVariant = GradientShadingVariant.ShadingDown background.Gradient.ShadingStyle = GradientShadingStyle.Horizontal #Save the resulting document document.SaveToFile("AddGradientBackground.docx", FileFormat.Docx2016) document.Close()
Add a Background Picture to Word in Python
To add a background picture to a Word document, you need to change the background type as "Picture", and then set a picture as the background. The detailed steps are as follows.
- Create a Document object.
- Load a Word document using Document.LoadFromFile() method.
- Get the background of the document using Document.Background property.
- Set the background type as Picture using Background.Type property.
- Set a picture as the background using Background.SetPicture() method.
- Save the resulting document using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load a Word document document.LoadFromFile("Sample.docx") # Get the document's background background = document.Background # Set the background type as Picture background.Type = BackgroundType.Picture # Set the background picture background.SetPicture("background.jpg") #save the resulting document document.SaveToFile("AddBackgroundPicture.docx", FileFormat.Docx2016) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
