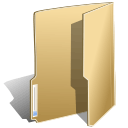
OpenXML (13)
class Program { static void Main(string[] args) { string DEMOPATH = @"..\..\Documents\Myppt14.pptx"; // Retrieve the number of slides, excluding the hidden slides. Console.WriteLine(RetrieveNumberOfSlides(DEMOPATH, false)); // Retrieve the number of slides, including the hidden slides. Console.WriteLine(RetrieveNumberOfSlides(DEMOPATH)); Console.ReadKey(); } public static int RetrieveNumberOfSlides(string fileName, bool includeHidden = true) { int slidesCount = 0; using (PresentationDocument doc = PresentationDocument.Open(fileName, false)) { // Get the presentation part of the document. PresentationPart presentationPart = doc.PresentationPart; if (presentationPart != null) { if (includeHidden) { slidesCount = presentationPart.SlideParts.Count(); } else { // Each slide can include a Show property, which if hidden // will contain the value "0". The Show property may not // exist, and most likely will not, for non-hidden slides. var slides = presentationPart.SlideParts.Where( (s) => (s.Slide != null) && ((s.Slide.Show == null) || (s.Slide.Show.HasValue && s.Slide.Show.Value))); slidesCount = slides.Count(); } } } return slidesCount; } }
Published in
OpenXML
class Program { static void Main(string[] args) { MoveSlide(@"..\..\Documents\Myppt13.pptx", 0, 1); } // Counting the slides in the presentation. public static int CountSlides(string presentationFile) { // Open the presentation as read-only. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, false)) { // Pass the presentation to the next CountSlides method // and return the slide count. return CountSlides(presentationDocument); } } // Count the slides in the presentation. public static int CountSlides(PresentationDocument presentationDocument) { // Check for a null document object. if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } int slidesCount = 0; // Get the presentation part of document. PresentationPart presentationPart = presentationDocument.PresentationPart; // Get the slide count from the SlideParts. if (presentationPart != null) { slidesCount = presentationPart.SlideParts.Count(); } // Return the slide count to the previous method. return slidesCount; } // Move a slide to a different position in the slide order in the presentation. public static void MoveSlide(string presentationFile, int from, int to) { using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, true)) { MoveSlide(presentationDocument, from, to); } } // Move a slide to a different position in the slide order in the presentation. public static void MoveSlide(PresentationDocument presentationDocument, int from, int to) { if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } // Call the CountSlides method to get the number of slides in the presentation. int slidesCount = CountSlides(presentationDocument); // Verify that both from and to positions are within range and different from one another. if (from < 0 || from >= slidesCount) { throw new ArgumentOutOfRangeException("from"); } if (to < 0 || from >= slidesCount || to == from) { throw new ArgumentOutOfRangeException("to"); } // Get the presentation part from the presentation document. PresentationPart presentationPart = presentationDocument.PresentationPart; // The slide count is not zero, so the presentation must contain slides. Presentation presentation = presentationPart.Presentation; SlideIdList slideIdList = presentation.SlideIdList; // Get the slide ID of the source slide. SlideId sourceSlide = slideIdList.ChildElements[from] as SlideId; SlideId targetSlide = null; // Identify the position of the target slide after which to move the source slide. if (to == 0) { targetSlide = null; } if (from < to) { targetSlide = slideIdList.ChildElements[to] as SlideId; } else { targetSlide = slideIdList.ChildElements[to - 1] as SlideId; } // Remove the source slide from its current position. sourceSlide.Remove(); // Insert the source slide at its new position after the target slide. slideIdList.InsertAfter(sourceSlide, targetSlide); // Save the modified presentation. presentation.Save(); } }
Published in
OpenXML
class Program { static void Main(string[] args) { string sourceFile = @"..\..\Documents\Myppt12.pptx"; string targetFile = @"..\..\Documents\Myppt11.pptx"; MoveParagraphToPresentation(sourceFile, targetFile); } // Moves a paragraph range in a TextBody shape in the source document // to another TextBody shape in the target document. public static void MoveParagraphToPresentation(string sourceFile, string targetFile) { // Open the source file as read/write. using (PresentationDocument sourceDoc = PresentationDocument.Open(sourceFile, true)) { // Open the target file as read/write. using (PresentationDocument targetDoc = PresentationDocument.Open(targetFile, true)) { // Get the first slide in the source presentation. SlidePart slide1 = GetFirstSlide(sourceDoc); // Get the first TextBody shape in it. TextBody textBody1 = slide1.Slide.Descendants().First(); // Get the first paragraph in the TextBody shape. // Note: "Drawing" is the alias of namespace DocumentFormat.OpenXml.Drawing Drawing.Paragraph p1 = textBody1.Elements().First(); // Get the first slide in the target presentation. SlidePart slide2 = GetFirstSlide(targetDoc); // Get the first TextBody shape in it. TextBody textBody2 = slide2.Slide.Descendants().First(); // Clone the source paragraph and insert the cloned. paragraph into the target TextBody shape. // Passing "true" creates a deep clone, which creates a copy of the // Paragraph object and everything directly or indirectly referenced by that object. textBody2.Append(p1.CloneNode(true)); // Remove the source paragraph from the source file. textBody1.RemoveChild(p1); // Replace the removed paragraph with a placeholder. textBody1.AppendChild(new Drawing.Paragraph()); // Save the slide in the source file. slide1.Slide.Save(); // Save the slide in the target file. slide2.Slide.Save(); } } } // Get the slide part of the first slide in the presentation document. public static SlidePart GetFirstSlide(PresentationDocument presentationDocument) { // Get relationship ID of the first slide PresentationPart part = presentationDocument.PresentationPart; SlideId slideId = part.Presentation.SlideIdList.GetFirstChild(); string relId = slideId.RelationshipId; // Get the slide part by the relationship ID. SlidePart slidePart = (SlidePart)part.GetPartById(relId); return slidePart; } }
Published in
OpenXML
class Program { static void Main(string[] args) { InsertNewSlide(@"..\..\Documents\Myppt2.pptx", 1, "My new slide"); } // Insert a slide into the specified presentation. public static void InsertNewSlide(string presentationFile, int position, string slideTitle) { // Open the source document as read/write. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, true)) { // Pass the source document and the position and title of the slide to be inserted to the next method. InsertNewSlide(presentationDocument, position, slideTitle); } } // Insert the specified slide into the presentation at the specified position. public static void InsertNewSlide(PresentationDocument presentationDocument, int position, string slideTitle) { if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } if (slideTitle == null) { throw new ArgumentNullException("slideTitle"); } PresentationPart presentationPart = presentationDocument.PresentationPart; // Verify that the presentation is not empty. if (presentationPart == null) { throw new InvalidOperationException("The presentation document is empty."); } // Declare and instantiate a new slide. Slide slide = new Slide(new CommonSlideData(new ShapeTree())); uint drawingObjectId = 1; // Construct the slide content. // Specify the non-visual properties of the new slide. NonVisualGroupShapeProperties nonVisualProperties = slide.CommonSlideData.ShapeTree.AppendChild(new NonVisualGroupShapeProperties()); nonVisualProperties.NonVisualDrawingProperties = new NonVisualDrawingProperties() { Id = 1, Name = "" }; nonVisualProperties.NonVisualGroupShapeDrawingProperties = new NonVisualGroupShapeDrawingProperties(); nonVisualProperties.ApplicationNonVisualDrawingProperties = new ApplicationNonVisualDrawingProperties(); // Specify the group shape properties of the new slide. slide.CommonSlideData.ShapeTree.AppendChild(new GroupShapeProperties()); // Declare and instantiate the title shape of the new slide. Shape titleShape = slide.CommonSlideData.ShapeTree.AppendChild(new Shape()); drawingObjectId++; // Specify the required shape properties for the title shape. titleShape.NonVisualShapeProperties = new NonVisualShapeProperties (new NonVisualDrawingProperties() { Id = drawingObjectId, Name = "Title" }, new NonVisualShapeDrawingProperties(new Drawing.ShapeLocks() { NoGrouping = true }), new ApplicationNonVisualDrawingProperties(new PlaceholderShape() { Type = PlaceholderValues.Title })); titleShape.ShapeProperties = new ShapeProperties(); // Specify the text of the title shape. titleShape.TextBody = new TextBody(new Drawing.BodyProperties(), new Drawing.ListStyle(), new Drawing.Paragraph(new Drawing.Run(new Drawing.Text() { Text = slideTitle }))); // Declare and instantiate the body shape of the new slide. Shape bodyShape = slide.CommonSlideData.ShapeTree.AppendChild(new Shape()); drawingObjectId++; // Specify the required shape properties for the body shape. bodyShape.NonVisualShapeProperties = new NonVisualShapeProperties(new NonVisualDrawingProperties() { Id = drawingObjectId, Name = "Content Placeholder" }, new NonVisualShapeDrawingProperties(new Drawing.ShapeLocks() { NoGrouping = true }), new ApplicationNonVisualDrawingProperties(new PlaceholderShape() { Index = 1 })); bodyShape.ShapeProperties = new ShapeProperties(); // Specify the text of the body shape. bodyShape.TextBody = new TextBody(new Drawing.BodyProperties(), new Drawing.ListStyle(), new Drawing.Paragraph()); // Create the slide part for the new slide. SlidePart slidePart = presentationPart.AddNewPart(); // Save the new slide part. slide.Save(slidePart); // Modify the slide ID list in the presentation part. // The slide ID list should not be null. SlideIdList slideIdList = presentationPart.Presentation.SlideIdList; // Find the highest slide ID in the current list. uint maxSlideId = 1; SlideId prevSlideId = null; foreach (SlideId slideId in slideIdList.ChildElements) { if (slideId.Id > maxSlideId) { maxSlideId = slideId.Id; } position--; if (position == 0) { prevSlideId = slideId; } } maxSlideId++; // Get the ID of the previous slide. SlidePart lastSlidePart; if (prevSlideId != null) { lastSlidePart = (SlidePart)presentationPart.GetPartById(prevSlideId.RelationshipId); } else { lastSlidePart = (SlidePart)presentationPart.GetPartById(((SlideId)(slideIdList.ChildElements[0])).RelationshipId); } // Use the same slide layout as that of the previous slide. if (null != lastSlidePart.SlideLayoutPart) { slidePart.AddPart(lastSlidePart.SlideLayoutPart); } // Insert the new slide into the slide list after the previous slide. SlideId newSlideId = slideIdList.InsertAfter(new SlideId(), prevSlideId); newSlideId.Id = maxSlideId; newSlideId.RelationshipId = presentationPart.GetIdOfPart(slidePart); // Save the modified presentation. presentationPart.Presentation.Save(); } }
Published in
OpenXML
class Program { static void Main(string[] args) { foreach (string s in GetSlideTitles(@"..\..\Documents\Myppt10.pptx")) Console.WriteLine(s); } // Get a list of the titles of all the slides in the presentation. public static IList GetSlideTitles(string presentationFile) { // Open the presentation as read-only. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, false)) { return GetSlideTitles(presentationDocument); } } // Get a list of the titles of all the slides in the presentation. public static IList GetSlideTitles(PresentationDocument presentationDocument) { if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } // Get a PresentationPart object from the PresentationDocument object. PresentationPart presentationPart = presentationDocument.PresentationPart; if (presentationPart != null && presentationPart.Presentation != null) { // Get a Presentation object from the PresentationPart object. Presentation presentation = presentationPart.Presentation; if (presentation.SlideIdList != null) { List titlesList = new List(); // Get the title of each slide in the slide order. foreach (var slideId in presentation.SlideIdList.Elements()) { SlidePart slidePart = presentationPart.GetPartById(slideId.RelationshipId) as SlidePart; // Get the slide title. string title = GetSlideTitle(slidePart); // An empty title can also be added. titlesList.Add(title); } return titlesList; } } return null; } // Get the title string of the slide. public static string GetSlideTitle(SlidePart slidePart) { if (slidePart == null) { throw new ArgumentNullException("presentationDocument"); } // Declare a paragraph separator. string paragraphSeparator = null; if (slidePart.Slide != null) { // Find all the title shapes. var shapes = from shape in slidePart.Slide.Descendants() where IsTitleShape(shape) select shape; StringBuilder paragraphText = new StringBuilder(); foreach (var shape in shapes) { // Get the text in each paragraph in this shape. foreach (var paragraph in shape.TextBody.Descendants()) { // Add a line break. paragraphText.Append(paragraphSeparator); foreach (var text in paragraph.Descendants()) { paragraphText.Append(text.Text); } paragraphSeparator = "\n"; } } return paragraphText.ToString(); } return string.Empty; } // Determines whether the shape is a title shape. private static bool IsTitleShape(Shape shape) { var placeholderShape = shape.NonVisualShapeProperties.ApplicationNonVisualDrawingProperties.GetFirstChild(); if (placeholderShape != null && placeholderShape.Type != null && placeholderShape.Type.HasValue) { switch ((PlaceholderValues)placeholderShape.Type) { // Any title shape. case PlaceholderValues.Title: // A centered title. case PlaceholderValues.CenteredTitle: return true; default: return false; } } return false; } }
Published in
OpenXML
class Program { static void Main(string[] args) { Console.Write("Please enter a presentation file name without extension: "); string fileName = Console.ReadLine(); string file = @"..\..\Documents\" + fileName + ".pptx"; int numberOfSlides = CountSlides(file); System.Console.WriteLine("Number of slides = {0}", numberOfSlides); string slideText; for (int i = 0; i < numberOfSlides; i++) { GetSlideIdAndText(out slideText, file, i); System.Console.WriteLine("Slide #{0} contains: {1}", i + 1, slideText); } System.Console.ReadKey(); } public static int CountSlides(string presentationFile) { // Open the presentation as read-only. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, false)) { // Pass the presentation to the next CountSlides method // and return the slide count. return CountSlides(presentationDocument); } } // Count the slides in the presentation. public static int CountSlides(PresentationDocument presentationDocument) { // Check for a null document object. if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } int slidesCount = 0; // Get the presentation part of document. PresentationPart presentationPart = presentationDocument.PresentationPart; // Get the slide count from the SlideParts. if (presentationPart != null) { slidesCount = presentationPart.SlideParts.Count(); } // Return the slide count to the previous method. return slidesCount; } public static void GetSlideIdAndText(out string sldText, string docName, int index) { using (PresentationDocument ppt = PresentationDocument.Open(docName, false)) { // Get the relationship ID of the first slide. PresentationPart part = ppt.PresentationPart; OpenXmlElementList slideIds = part.Presentation.SlideIdList.ChildElements; string relId = (slideIds[index] as SlideId).RelationshipId; // Get the slide part from the relationship ID. SlidePart slide = (SlidePart)part.GetPartById(relId); // Build a StringBuilder object. StringBuilder paragraphText = new StringBuilder(); // Get the inner text of the slide: IEnumerable texts = slide.Slide.Descendants(); foreach (A.Text text in texts) { paragraphText.Append(text.Text); } sldText = paragraphText.ToString(); } } }
Published in
OpenXML
class Program { static void Main(string[] args) { foreach (string s in GetAllTextInSlide(@"..\..\Documents\Myppt8.pptx", 1)) Console.WriteLine(s); } // Get all the text in a slide. public static string[] GetAllTextInSlide(string presentationFile, int slideIndex) { // Open the presentation as read-only. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, false)) { // Pass the presentation and the slide index // to the next GetAllTextInSlide method, and // then return the array of strings it returns. return GetAllTextInSlide(presentationDocument, slideIndex); } } public static string[] GetAllTextInSlide(PresentationDocument presentationDocument, int slideIndex) { // Verify that the presentation document exists. if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } // Verify that the slide index is not out of range. if (slideIndex < 0) { throw new ArgumentOutOfRangeException("slideIndex"); } // Get the presentation part of the presentation document. PresentationPart presentationPart = presentationDocument.PresentationPart; // Verify that the presentation part and presentation exist. if (presentationPart != null && presentationPart.Presentation != null) { // Get the Presentation object from the presentation part. Presentation presentation = presentationPart.Presentation; // Verify that the slide ID list exists. if (presentation.SlideIdList != null) { // Get the collection of slide IDs from the slide ID list. DocumentFormat.OpenXml.OpenXmlElementList slideIds = presentation.SlideIdList.ChildElements; // If the slide ID is in range... if (slideIndex < slideIds.Count) { // Get the relationship ID of the slide. string slidePartRelationshipId = (slideIds[slideIndex] as SlideId).RelationshipId; // Get the specified slide part from the relationship ID. SlidePart slidePart = (SlidePart)presentationPart.GetPartById(slidePartRelationshipId); // Pass the slide part to the next method, and // then return the array of strings that method // returns to the previous method. return GetAllTextInSlide(slidePart); } } } // Else, return null. return null; } public static string[] GetAllTextInSlide(SlidePart slidePart) { // Verify that the slide part exists. if (slidePart == null) { throw new ArgumentNullException("slidePart"); } // Create a new linked list of strings. LinkedList texts = new LinkedList(); // If the slide exists... if (slidePart.Slide != null) { // Iterate through all the paragraphs in the slide. foreach (DocumentFormat.OpenXml.Drawing.Paragraph paragraph in slidePart.Slide.Descendants()) { // Create a new string builder. StringBuilder paragraphText = new StringBuilder(); // Iterate through the lines of the paragraph. foreach (DocumentFormat.OpenXml.Drawing.Text text in paragraph.Descendants()) { // Append each line to the previous lines. paragraphText.Append(text.Text); } if (paragraphText.Length > 0) { // Add each paragraph to the linked list. texts.AddLast(paragraphText.ToString()); } } } if (texts.Count > 0) { // Return an array of strings. return texts.ToArray(); } else { return null; } } }
Published in
OpenXML
class Program { static void Main(string[] args) { string fileName = @"..\..\Documents\Myppt7.pptx"; foreach (string s in GetAllExternalHyperlinksInPresentation(fileName)) Console.WriteLine(s); } // Returns all the external hyperlinks in the slides of a presentation. public static IEnumerable GetAllExternalHyperlinksInPresentation(string fileName) { // Declare a list of strings. List ret = new List(); // Open the presentation file as read-only. using (PresentationDocument document = PresentationDocument.Open(fileName, false)) { // Iterate through all the slide parts in the presentation part. foreach (SlidePart slidePart in document.PresentationPart.SlideParts) { IEnumerable links = slidePart.Slide.Descendants(); // Iterate through all the links in the slide part. foreach (Drawing.HyperlinkType link in links) { // Iterate through all the external relationships in the slide part. foreach (HyperlinkRelationship relation in slidePart.HyperlinkRelationships) { // If the relationship ID matches the link ID… if (relation.Id.Equals(link.Id)) { // Add the URI of the external relationship to the list of strings. ret.Add(relation.Uri.AbsoluteUri); } } } } } // Return the list of strings. return ret; } }
Published in
OpenXML
class Program { static void Main(string[] args) { string fileName = @"..\..\Documents\myppt6.pptx"; string author = "MZ"; DeleteCommentsByAuthorInPresentation(fileName, author); } // Remove all the comments in the slides by a certain author. public static void DeleteCommentsByAuthorInPresentation(string fileName, string author) { if (String.IsNullOrEmpty(fileName) || String.IsNullOrEmpty(author)) throw new ArgumentNullException("File name or author name is NULL!"); using (PresentationDocument doc = PresentationDocument.Open(fileName, true)) { // Get the specifed comment author. IEnumerable commentAuthors = doc.PresentationPart.CommentAuthorsPart.CommentAuthorList.Elements() .Where(e => e.Name.Value.Equals(author)); // Iterate through all the matching authors. foreach (CommentAuthor commentAuthor in commentAuthors) { UInt32Value authorId = commentAuthor.Id; // Iterate through all the slides and get the slide parts. foreach (SlidePart slide in doc.PresentationPart.SlideParts) { SlideCommentsPart slideCommentsPart = slide.SlideCommentsPart; // Get the list of comments. if (slideCommentsPart != null && slide.SlideCommentsPart.CommentList != null) { IEnumerable commentList = slideCommentsPart.CommentList.Elements().Where(e => e.AuthorId == authorId.Value); List comments = new List(); comments = commentList.ToList(); foreach (Comment comm in comments) { // Delete all the comments by the specified author. slideCommentsPart.CommentList.RemoveChild(comm); } // If the commentPart has no existing comment. if (slideCommentsPart.CommentList.ChildElements.Count == 0) // Delete this part. slide.DeletePart(slideCommentsPart); } } // Delete the comment author from the comment authors part. doc.PresentationPart.CommentAuthorsPart.CommentAuthorList.RemoveChild(commentAuthor); } } } }
Published in
OpenXML
class Program { static void Main(string[] args) { DeleteSlide(@"..\..\Documents\Myppt5.pptx", 2); Console.WriteLine("Number of slides = {0}",CountSlides(@"..\..\Documents\Myppt5.pptx")); } // Get the presentation object and pass it to the next CountSlides method. public static int CountSlides(string presentationFile) { // Open the presentation as read-only. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, false)) { // Pass the presentation to the next CountSlide method // and return the slide count. return CountSlides(presentationDocument); } } // Count the slides in the presentation. public static int CountSlides(PresentationDocument presentationDocument) { // Check for a null document object. if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } int slidesCount = 0; // Get the presentation part of document. PresentationPart presentationPart = presentationDocument.PresentationPart; // Get the slide count from the SlideParts. if (presentationPart != null) { slidesCount = presentationPart.SlideParts.Count(); } // Return the slide count to the previous method. return slidesCount; } // // Get the presentation object and pass it to the next DeleteSlide method. public static void DeleteSlide(string presentationFile, int slideIndex) { // Open the source document as read/write. using (PresentationDocument presentationDocument = PresentationDocument.Open(presentationFile, true)) { // Pass the source document and the index of the slide to be deleted to the next DeleteSlide method. DeleteSlide(presentationDocument, slideIndex); } } // Delete the specified slide from the presentation. public static void DeleteSlide(PresentationDocument presentationDocument, int slideIndex) { if (presentationDocument == null) { throw new ArgumentNullException("presentationDocument"); } // Use the CountSlides sample to get the number of slides in the presentation. int slidesCount = CountSlides(presentationDocument); if (slideIndex < 0 || slideIndex >= slidesCount) { throw new ArgumentOutOfRangeException("slideIndex"); } // Get the presentation part from the presentation document. PresentationPart presentationPart = presentationDocument.PresentationPart; // Get the presentation from the presentation part. Presentation presentation = presentationPart.Presentation; // Get the list of slide IDs in the presentation. SlideIdList slideIdList = presentation.SlideIdList; // Get the slide ID of the specified slide SlideId slideId = slideIdList.ChildElements[slideIndex] as SlideId; // Get the relationship ID of the slide. string slideRelId = slideId.RelationshipId; // Remove the slide from the slide list. slideIdList.RemoveChild(slideId); // // Remove references to the slide from all custom shows. if (presentation.CustomShowList != null) { // Iterate through the list of custom shows. foreach (var customShow in presentation.CustomShowList.Elements()) { if (customShow.SlideList != null) { // Declare a link list of slide list entries. LinkedList slideListEntries = new LinkedList(); foreach (SlideListEntry slideListEntry in customShow.SlideList.Elements()) { // Find the slide reference to remove from the custom show. if (slideListEntry.Id != null && slideListEntry.Id == slideRelId) { slideListEntries.AddLast(slideListEntry); } } // Remove all references to the slide from the custom show. foreach (SlideListEntry slideListEntry in slideListEntries) { customShow.SlideList.RemoveChild(slideListEntry); } } } } // Save the modified presentation. presentation.Save(); // Get the slide part for the specified slide. SlidePart slidePart = presentationPart.GetPartById(slideRelId) as SlidePart; // Remove the slide part. presentationPart.DeletePart(slidePart); } }
Published in
OpenXML
