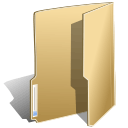
Document Operation (20)
Preserving and displaying documents precisely is a primary function of PDF. However, the viewing preference settings of different devices and users would still affect the display of PDF documents. To solve this problem, PDF provides the viewer preference entry in a document to control the way the PDF document presents on screen. Without it, PDF documents will display according to the current user’s preference setting. This article will show how to set PDF viewer preferences by programming using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Set Viewer Preferences of a PDF Document
Spire.PDF for Java includes several methods under PdfViewerPreferences class, which can decide whether to center the window, display title, fit the window, and hide menu bar as well as tool bar and set page layout, page mode, and scaling mode. The detailed steps of setting viewer preferences are as follows.
- Create an object of PdfDocument class.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Get viewer preferences of the document using PdfDocument.getViewerPreferences() method.
- Set the viewer preferences using the methods under PdfViewerPreferences object.
- Save the file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class setViewerPreference { public static void main(String[] args) { //Create an object of PdfDocument class PdfDocument pdf = new PdfDocument(); //Load a PDF file pdf.loadFromFile("C:/Sample3.pdf"); //Get viewer preferences of the document PdfViewerPreferences preferences = pdf.getViewerPreferences(); //Set viewer preferences preferences.setCenterWindow(true); preferences.setDisplayTitle(false); preferences.setFitWindow(true); preferences.setHideMenubar(true); preferences.setHideToolbar(true); preferences.setPageLayout(PdfPageLayout.Single_Page); //preferences.setPageMode(PdfPageMode.Full_Screen); //preferences.setPrintScaling(PrintScalingMode.App_Default); //Save the file pdf.saveToFile("SetViewerPreference.pdf"); pdf.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF properties, as a part of a PDF document, are not shown on a page. Those properties contain information on documents, including title, author, subject, keywords, creation date, and creator. Some of the property values will not be produced automatically, and we have to set them by ourselves. This article will show you how to set or retrieve PDF properties programmatically using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Set Properties of a PDF Document in Java
The detailed steps of setting PDF properties are as follows.
- Create an object of PdfDocument class.
- Load a PDF document from disk using PdfDocument.loadFromFile() method.
- Set document properties including title, author, subject, keywords, creation date, modification date, creator, and producer using the methods under DocumentInformation object returned by PdfDocument.getDocumentInformation() method.
- Save the document using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; import java.util.Date; public class setPDFProperties { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument pdfDocument = new PdfDocument(); //Load a PDF document from disk pdfDocument.loadFromFile("D:/Samples/Sample.pdf"); //Set the title pdfDocument.getDocumentInformation().setTitle("PDF(Portable Document Format)"); //Set the author pdfDocument.getDocumentInformation().setAuthor("John"); //Set the subject pdfDocument.getDocumentInformation().setSubject("Introduction of PDF"); //Set the keywords pdfDocument.getDocumentInformation().setKeywords("PDF, document format"); //Set the creation time pdfDocument.getDocumentInformation().setCreationDate(new Date()); //Set the creator name pdfDocument.getDocumentInformation().setCreator("John"); //Set the modification time pdfDocument.getDocumentInformation().setModificationDate(new Date()); //Set the producer name pdfDocument.getDocumentInformation().setProducer("Spire.PDF for Java"); //Save the document pdfDocument.saveToFile("output/setPDFProperties.pdf"); } }
Get Properties of a PDF Document in Java
The detailed steps of retrieving PDF properties are as follows.
- Create an object of PdfDocument class.
- Load a PDF document from disk using PdfDocument.loadFromFile() method.
- Create a StringBuilder instance to store the values of document properties.
- Get properties using the methods under DocumentInformation object returned by PdfDocument.getDocumentInformation() method and put them in the StringBuilder.
- Create a new TXT file using File.createNewFile() method.
- Write the StringBuilder to the TXT file using BufferedWriter.write() method.
- Java
import com.spire.pdf.*; import java.io.*; public class getPDFProperties { public static void main(String[] args) throws IOException { //Create an object of PdfDocument class PdfDocument pdf = new PdfDocument(); //Load a PDF document from disk pdf.loadFromFile("D:/Samples/Sample.pdf"); //Create a StringBuilder instance to store the values of document properties StringBuilder stringBuilder = new StringBuilder(); //Retrieve property values and put them in the StringBuilder stringBuilder.append("Title: " + pdf.getDocumentInformation().getTitle() + "\r\n"); stringBuilder.append("Author: " + pdf.getDocumentInformation().getAuthor() + "\r\n"); stringBuilder.append("Subject: " + pdf.getDocumentInformation().getSubject() + "\r\n"); stringBuilder.append("Keywords: " + pdf.getDocumentInformation().getKeywords() + "\r\n"); stringBuilder.append("Creator: " + pdf.getDocumentInformation().getCreator() + "\r\n"); stringBuilder.append("Creation Date: " + pdf.getDocumentInformation().getCreationDate() + "\r\n"); stringBuilder.append("Producer: " + pdf.getDocumentInformation().getProducer() + "\r\n"); //Create a new TXT file File file = new File("D:/output/getPDFProperties.txt"); file.createNewFile(); //Write the StringBuilder to the TXT file FileWriter fileWriter = new FileWriter(file, true); BufferedWriter bufferedWriter = new BufferedWriter(fileWriter); bufferedWriter.write(stringBuilder.toString()); bufferedWriter.flush(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF is a file format developed by Adobe in 1992, and during the years, it has undergone a lot of changes. Sometimes you may find that some devices have strict requirements on the PDF version. In such a case, it's necessary to change the PDF file to a different version for compatibility purpose. This article will show how to programmatically change the PDF version using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Change PDF Version
Spire.PDF for Java supports the PDF versions from 1.0 to 1.7. The following are the detailed steps to change the PDF version.
- Create a PdfDocument object.
- Load a sample PDF file using PdfDocument.loadFromFile() method.
- Change the PDF file to another version using PdfDocument.getFileInfo().setVersion() method.
- Save the document to another file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class ChangePdfVersion { public static void main(String[] args) { //Create a PdfDocument object PdfDocument document = new PdfDocument(); //Load a sample PDF file document.loadFromFile("sample.pdf"); //Change the PDF to version 1.5 document.getFileInfo().setVersion(PdfVersion.Version_1_5); //Save to file document.saveToFile("PdfVersion.pdf", FileFormat.PDF); document.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
By splitting PDF pages into separate files, you get smaller PDF documents that have one or some pages extracted from the original. A split file contains less information and is naturally smaller in size and easier to share over the internet. In this article, you will learn how to split PDF into single-page PDFs and how to split PDF by page ranges in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from ;this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Split a PDF File into Multiple Single-Page PDFs in Java
Spire.PDF for Java offers the split() method to divide a multipage PDF document into multiple single-page files. The following are the detailed steps.
- Create a PdfDcoument object.
- Load a PDF document using PdfDocument.loadFromFile() method.
- Split the document into one-page PDFs using PdfDocument.split(string destFilePattern, int startNumber) method.
- Java
import com.spire.pdf.PdfDocument; public class SplitPdfByEachPage { public static void main(String[] args) { //Specify the input file path String inputFile = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf"; //Specify the output directory String outputDirectory = "C:\\Users\\Administrator\\Desktop\\Output\\"; //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load a PDF file doc.loadFromFile(inputFile); //Split the PDF to one-page PDFs doc.split(outputDirectory + "output-{0}.pdf", 1); } }
Split a PDF File by Page Ranges in Java
No straightforward method is offered for splitting PDF documents by page ranges. To do so, we create two or more new PDF documents and import the selected page or page range from the source document into them. Here are the detailed steps.
- Load the source PDF file while initialing the PdfDocument object.
- Create two additional PdfDocument objects.
- Import the first page from the source file to the first document using PdfDocument.insertPage() method.
- Import the remaining pages from the source file to the second document using PdfDocument.insertPageRange() method.
- Save the two documents as separate PDF files using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; public class SplitPdfByPageRange { public static void main(String[] args) { //Specify the input file path String inputFile = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf"; //Specify the output directory String outputDirectory = "C:\\Users\\Administrator\\Desktop\\Output\\"; //Load the source PDF file while initialing the PdfDocument object PdfDocument sourceDoc = new PdfDocument(inputFile); //Create two additional PdfDocument objects PdfDocument newDoc_1 = new PdfDocument(); PdfDocument newDoc_2 = new PdfDocument(); //Insert the first page of source file to the first document newDoc_1.insertPage(sourceDoc, 0); //Insert the rest pages of source file to the second document newDoc_2.insertPageRange(sourceDoc, 1, sourceDoc.getPages().getCount() - 1); //Save the two documents as PDF files newDoc_1.saveToFile(outputDirectory + "output-1.pdf"); newDoc_2.saveToFile(outputDirectory + "output-2.pdf"); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
If you are having trouble handling a great many PDF files, you might want to merge all of those files into one. Combining multiple files into one PDF lets you store, share and review them more easily. In this article, you will learn how to merge PDF documents in Java by using Spire.PDF for Java.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Merge Multiple PDFs into a Single PDF
Spire.PDF for Java offers the PdfDocument.mergeFiles() method to merge multiple PDF documents into a single document. The detailed steps are as follows.
- Get the paths of the documents to be merged and store them in a String array.
- Call PdfDocument.mergeFiles() method to merge these files. This method also accepts an array of InputStream as the parameter.
- Save the result to a PDF document using PdfDocumentBase.save() method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfDocumentBase; public class MergePdfs { public static void main(String[] args) { //Get the paths of the documents to be merged String[] files = new String[] { "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-1.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-2.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-3.pdf"}; //Merge these documents and return an object of PdfDocumentBase PdfDocumentBase doc = PdfDocument.mergeFiles(files); //Save the result to a PDF file doc.save("output.pdf", FileFormat.PDF); } }
Merge the Selected Pages of Different PDFs into One PDF
Spire.PDF for Java offers the PdfDocument.insertPage() method and the PdfDocument.insertPageRange() method to import a page or a page range from one PDF document to another. The following are the steps to combine the selected pages of different PDF documents into a new PDF document.
- Get the paths of the source documents and store them in a String array.
- Create an array of PdfDocument, and load each source document to a separate PdfDocument object.
- Create another PdfDocument object for generating a new document.
- Insert the selected page or page range of the source documents to the new document using PdfDocument.insertPage() method and PdfDocument.insertPageRange() method.
- Save the new document to a PDF file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; public class MergeSelectedPages { public static void main(String[] args) { //Get the paths of the documents to be merged String[] files = new String[] { "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-1.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-2.pdf", "C:\\Users\\Administrator\\Desktop\\PDFs\\sample-3.pdf"}; //Create an array of PdfDocument PdfDocument[] docs = new PdfDocument[files.length]; //Loop through the documents for (int i = 0; i < files.length; i++) { //Load a specific document docs[i] = new PdfDocument(files[i]); } //Create a PdfDocument object for generating a new PDF document PdfDocument doc = new PdfDocument(); //Insert the selected pages from different documents to the new document doc.insertPage(docs[0], 0); doc.insertPageRange(docs[1], 1,3); doc.insertPage(docs[2], 0); //Save the document to a PDF file doc.saveToFile("output.pdf"); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Generating PDFs through code provides various advantages. It enables integration of dynamic content such as real-time data, database records, and user input. Code-based PDF creation offers greater customization and automation, reducing manual involvement in developing personalized documents. In this article, you will learn how to create a simple PDF document from scratch in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Background Knowledge
A page in Spire.PDF for Java (represented by PdfPageBase class) consists of client area and margins all around. The content area is for users to write various contents, and the margins are usually blank edges.
As shown in the figure below, the origin of the coordinate system on the page is located at the top left corner of the client area, with the x-axis extending horizontally to the right and the y-axis extending vertically down. All elements added to the client area must be based on the specified coordinates.
In addition, the following table lists the important classes and methods, which can help you easily understand the code snippet provided in the following section.
Member | Description |
PdfDocument class | Represents a PDF document model. |
PdfPageBase class | Represents a page in a PDF document. |
PdfSolidBrush class | Represents a brush that fills any object with a solid color. |
PdfTrueTypeFont class | Represents a true type font. |
PdfStringFormat class | Represents text format information, such as alignment, characters spacing and indent. |
PdfTextWidget class | Represents the text area with the ability to span several pages. |
PdfTextLayout class | Represents the text layout information. |
PdfDocument.getPages().add() method | Adds a page to a PDF document. |
PdfPageBase.getCanvas().drawString() method | Draws string on a page at the specified location with specified font and brush objects. |
PdfTextWidget.draw() method | Draws the text widget on a page at the specified location. |
PdfDocument.save() method | Saves the document to a PDF file. |
Create a PDF Document from Scratch in Java
Despite the fact that Spire.PDF for Java enables users to add various elements to PDF documents, this article demonstrates how to create a simple PDF document with only plain text. The following are the detailed steps.
- Create a PdfDocument object.
- Add a page using PdfDocument.getPages().add() method.
- Create brush and font objects.
- Draw string on the page at a specified coordinate using PdfPageBase.getCanvas().drawString() method.
- Create a PdfTextWidget object to hold a chunk of text.
- Draw the text widget on the page at a specified location using PdfTextWidget.draw() method
- Save the document to a PDF file using PdfDocument.save() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.PdfPageSize; import com.spire.pdf.graphics.*; import java.awt.*; import java.awt.geom.Point2D; import java.awt.geom.Rectangle2D; import java.io.*; public class CreatePdfDocument { public static void main(String[] args) throws IOException { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Add a page PdfPageBase page = doc.getPages().add(PdfPageSize.A4, new PdfMargins(35f)); //Specify title text String titleText = "What is MySQL"; //Create solid brushes PdfSolidBrush titleBrush = new PdfSolidBrush(new PdfRGBColor(Color.BLUE)); PdfSolidBrush paraBrush = new PdfSolidBrush(new PdfRGBColor(Color.BLACK)); //Create true type fonts PdfTrueTypeFont titleFont = new PdfTrueTypeFont(new Font("Times New Roman",Font.BOLD,18)); PdfTrueTypeFont paraFont = new PdfTrueTypeFont(new Font("Times New Roman",Font.PLAIN,12)); //Set the text alignment via PdfStringFormat class PdfStringFormat format = new PdfStringFormat(); format.setAlignment(PdfTextAlignment.Center); //Draw title on the page page.getCanvas().drawString(titleText, titleFont, titleBrush, new Point2D.Float((float)page.getClientSize().getWidth()/2, 20),format); //Get paragraph text from a .txt file String paraText = readFileToString("C:\\Users\\Administrator\\Desktop\\content.txt"); //Create a PdfTextWidget object to hold the paragraph content PdfTextWidget widget = new PdfTextWidget(paraText, paraFont, paraBrush); //Create a rectangle where the paragraph content will be placed Rectangle2D.Float rect = new Rectangle2D.Float(0, 50, (float)page.getClientSize().getWidth(),(float)page.getClientSize().getHeight()); //Set the PdfLayoutType to Paginate to make the content paginated automatically PdfTextLayout layout = new PdfTextLayout(); layout.setLayout(PdfLayoutType.Paginate); //Draw paragraph text on the page widget.draw(page, rect, layout); //Save to file doc.saveToFile("output/CreatePdfDocument.pdf"); doc.dispose(); } //Convert a .txt file to String private static String readFileToString(String filepath) throws FileNotFoundException, IOException { StringBuilder sb = new StringBuilder(); String s =""; BufferedReader br = new BufferedReader(new FileReader(filepath)); while( (s = br.readLine()) != null) { sb.append(s + "\n"); } br.close(); String str = sb.toString(); return str; } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
