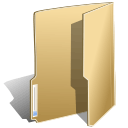
Conversion (4)
Converting between presentation documents is a common task, especially when dealing with different versions of Microsoft PowerPoint. PPS is used to display presentations directly as finalized documents. PPT is an older format compatible with PowerPoint 97-2003. PPTX, the default editable format in the latest version of PowerPoint, offers better data recovery capabilities, smaller file sizes, and enhanced security.
Whether you need to edit PPS documents or ensure compatibility with modern tools and features, converting them to PPTX is essential. This article will demonstrate how to convert PPS and PPT to PPTX documents using Python with Spire.Presentation for Python. Read on to learn more.
Install Spire.Presentation
This scenario requires Spire.Presentation for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.Presentation
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Presentation for Python on Windows.
How to Convert PPS to PPTX with Python
To convert PPS to PPTX format, you can call Document.SaveToFile() method offered by Spire.Presentation for Python. It supports converting various formats to PPT(X), such as PPS and PPT, as well as converting PPT documents to other formats like PDF and images (PNG, JPG, BMP, SVG).
Steps to convert PPS to PPTX:
- Create an object for the Presentation class.
- Import the document to be converted with Document.LoadFromFile() method.
- Convert it to a PPTX document using Document.SaveToFile() method.
Here is the code example for you:
- Python
from spire.presentation.common import * from spire.presentation import * # Create a Presentation document object pre = Presentation() # Load the file from the disk pre.LoadFromFile("input/sample.pps") # Save the document as PPTX pre.SaveToFile("ppstopptx.pptx", FileFormat.Pptx2010) pre.Dispose()
How to Convert PPT to PPTX with Python
Compared with PPT, PPTX has many advantages. Despite being mentioned above, it supports inserting more multimedia content and advanced formats, thereby improving the overall quality and performance of presentations. Spire.Presentation for Python provides Document.SaveToFile() to convert PPT to PPTX without data loss.
Steps to convert PPT to PPTX:
- Instantiate a new Presentation object.
- Load the document from the files with Document.LoadFromFile().
- Save the PPT document as a PPTX document by Document.SaveToFile().
Below is the code example to refer to:
- Python
from spire.presentation.common import * from spire.presentation import * # Create an instance of the Presentation class pre = Presentation() # Load the file to be converted pre.LoadFromFile("input/Sample1.ppt") # Convert the document to PPTX format pre.SaveToFile("ppttopptx.pptx", FileFormat.Pptx2010) pre.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Converting PowerPoint to Html is a way to share your presentations with others online. By converting to Html, you can publish the document content on a web page, making it universally accessible and appealing to diverse audiences. In this article, you will learn how to convert PowerPoint presentations to HTML format in Python using Spire.Presentation for Python.
Install Spire.Presentation for Python
This scenario requires Spire.Presentation for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Presentation
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Presentation for Python on Windows
Convert a PowerPoint Presentation to HTML in Python
The Presentation.SaveToFile() method offered by Spire.Presentation for Python supports converting a PowerPoint presentation to HTML format. The following are the detailed steps:
- Create a Presentation instance.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Save the PowerPoint document to HTML format using Presentation.SaveToFile() method.
- Python
from spire.presentation.common import * from spire.presentation import * inputFile ="Input.pptx" outputFile = "ToHTML.html" # Create a Presentation instance ppt = Presentation() # Load a PowerPoint document ppt.LoadFromFile(inputFile) #Save the document to HTML format ppt.SaveToFile(outputFile, FileFormat.Html) ppt.Dispose()
Convert a Specific PowerPoint Slide to HTML in Python
If you only need to convert a specific presentation slide to HTML, you can use the ISlide.SaveToFile(String, FileFormat) method. The following are the detailed steps.
- Create a Presentation instance.
- Load a PowerPoint document using Presentation.LoadFromFile() method.
- Get a specific slide by its index using Presentation.Slides[] property.
- Save the presentation slide to HTML format using ISlide.SaveToFile() method.
- Python
from spire.presentation.common import * from spire.presentation import * inputFile ="Input.pptx" outputFile = "SlideToHTML.html" # Create a Presentation instance ppt = Presentation() # Load a PowerPoint document ppt.LoadFromFile(inputFile) # Get the second slide slide = ppt.Slides[1] # Save the slide to HTML format slide.SaveToFile(outputFile, FileFormat.Html) ppt.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Python: Convert PowerPoint to Images (PNG, JPG, BMP, SVG)
2023-09-27 01:05:46 Written by support iceblueImages are universally compatible and can be easily shared across various platforms, devices, and applications. By converting PowerPoint slides to images, you can distribute your content effortlessly via email, messaging apps, websites, or social media platforms. This makes your presentation accessible to a wider audience and ensures that it can be viewed by anyone, regardless of the software or device they are using. In this article, we will explain how to convert PowerPoint to images in Python using Spire.Presentation for Python.
- Convert PowerPoint Presentation to JPG, PNG or BMP Images
- Convert PowerPoint Presentation to JPG, PNG or BMP Images with a Specific Size
- Convert PowerPoint Presentation to SVG Images
Install Spire.Presentation for Python
This scenario requires Spire.Presentation for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Presentation
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Presentation for Python on Windows
Convert PowerPoint Presentation to JPG, PNG or BMP Images in Python
Spire.Presentation for Python offers the ISlide.SaveAsImage() method which enables you to convert the slides in a PowerPoint presentation to image files in formats like PNG, JPG or BMP with ease. The detailed steps are as follows:
- Create a Presentation object.
- Load a PowerPoint presentation using Presentation.LoadFromFile() method.
- Loop through the slides in the presentation.
- Save each slide to an image stream using ISlide.SaveAsImage() method.
- Save the image stream to a JPG, PNG or BMP file using Stream.Save() method.
- Python
from spire.presentation.common import * from spire.presentation import * # Create a Presentation object presentation = Presentation() # Load a PowerPoint presentation presentation.LoadFromFile("Sample.pptx") # Loop through the slides in the presentation for i, slide in enumerate(presentation.Slides): # Specify the output file name fileName ="Output/ToImage_ + str(i) + ".png" # Save each slide as a PNG image image = slide.SaveAsImage() image.Save(fileName) image.Dispose() presentation.Dispose()
Convert PowerPoint Presentation to JPG, PNG or BMP Images with a Specific Size in Python
You can convert the slides in a PowerPoint presentation to images with a specific size using ISlide.SaveAsImageByWH() method. The detailed steps are as follows:
- Create a Presentation object.
- Load a PowerPoint presentation using Presentation.LoadFromFile() method.
- Loop through the slides in the presentation.
- Save each slide to an image stream using ISlide.SaveAsImageByWH() method.
- Save the image stream to a JPG, PNG or BMP file using Stream.Save() method.
- Python
from spire.presentation.common import * from spire.presentation import * # Create a Presentation object presentation = Presentation() # Load a PowerPoint presentation presentation.LoadFromFile("Sample.pptx") # Loop through the slides in the presentation for i, slide in enumerate(presentation.Slides): # Specify the output file name fileName ="Output/ToImage_" + str(i) + ".png" # Save each slide to a PNG image with a size of 700 * 400 pixels image = slide.SaveAsImageByWH(700, 400) image.Save(fileName) image.Dispose() presentation.Dispose()
Convert PowerPoint Presentation to SVG Images in Python
To convert the slides in a PowerPoint presentation to SVG images, you can use the ISlide.SaveToSVG() method. The detailed steps are as follows:
- Create a Presentation object.
- Load a PowerPoint presentation using Presentation.LoadFromFile() method.
- Enable the Presentation.IsNoteRetained property to retain notes when converting the presentation to SVG files.
- Loop through the slides in the presentation.
- Save each slide to an SVG stream using ISlide.SaveToSVG() method.
- Save the SVG stream to an SVG file using Stream.Save() method.
- Python
from spire.presentation.common import * from spire.presentation import * # Create a Presentation object presentation = Presentation() # Load a PowerPoint presentation presentation.LoadFromFile("Sample.pptx") # Enable the IsNoteRetained property to retain notes when converting the presentation to SVG files presentation.IsNoteRetained = True # Loop through the slides in the presentation for i, slide in enumerate(presentation.Slides): # Specify the output file name fileName = "SVG/ToSVG_" + str(i) + ".svg" # Save each slide to an SVG image svgStream = slide.SaveToSVG() svgStream.Save(fileName) presentation.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PowerPoint presentations provide visual engaging content delivery with dynamic multimedia elements. However, when it comes to distribution, converting PowerPoint presentations to PDF files offers distinct advantages. PDF documents ensure consistent document appearance across different platforms and devices, while also providing enhanced protection for document content, enabling users to extensively share presentations while ensuring the integrity and security of the content. This article is going to show how to use Spire.Presentation for Python to convert PowerPoint presentations to PDF files in Python programs.
- Convert a PowerPoint Presentation to a PDF Document
- Convert PowerPoint Presentation to PDF and Set the Page Size
- Convert a Presentation Slide to a PDF Document
Install Spire.Presentation
This scenario requires Spire.Presentation for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.Presentation
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Presentation for Python on Windows
Convert a PowerPoint Presentations to a PDF Document
Spire.Presentation for Python provides the Presentation.SaveToFile(fileName: str, fileFormat: FileFormat) that enables the conversion of a presentation file to a PDF document. Below are the detailed steps to perform this operation.
- Create an object of Presentation class.
- Load a presentation file using Presentation.LoadFromFile() method.
- Save the presentation to a PDF document using Presentation.SaveToFile(fileName: str, fileFormat: FileFormat) method.
- Python
from spire.presentation import * from spire.presentation.common import * # Create an object of Presentation class presentation = Presentation() # Load a presentation file presentation.LoadFromFile("Sample.pptx") # Convert the presentation file to PDF and save it presentation.SaveToFile("output/PresentationToPDF.pdf", FileFormat.PDF) presentation.Dispose()
Convert PowerPoint Presentation to PDF and Set the Page Size
During the conversion, users can also use the Presentation.SlideSize.Type property to set the slide size, thereby determining the page size of the resulting PDF document. Here are the detailed steps for this operation.
- Create an object of Presentation class.
- Load a presentation file using Presentation.LoadFromFile() method.
- Set the slide size to A4 using Presentation.SlideSize.Type property.
- Save the presentation to a PDF document using Presentation.SaveToFile(file: str, fileFormat: FileFormat) method.
- Python
from spire.presentation import * from spire.presentation.common import * # Create an object of Presentation class presentation = Presentation() # Load a presentation file presentation.LoadFromFile("Sample.pptx") # Change the side size to A4 presentation.SlideSize.Type = SlideSizeType.A4 # Convert the presentation file to PDF and save it presentation.SaveToFile("output/PresentationToPDFA4.pdf", FileFormat.PDF) presentation.Dispose()
Convert a Presentation Slide to a PDF Document
Spire.Presentation for Python also supports converting a single slide from a presentation file to a PDF document. The detailed steps for this operation are as follows.
- Create an object of Presentation class.
- Load a presentation file using Presentation.LoadFromFile() method.
- Get a slide using Presentation.Slides[] property.
- Save the slide as a PDF document using ISlde.SaveToFile(file: str, fileFormat: FileFormat) method.
- Python
from spire.presentation import * from spire.presentation.common import * # Create an object of Presentation class presentation = Presentation() # Load a presentation file presentation.LoadFromFile("Sample.pptx") # Get a slide slide = presentation.Slides[1] # Save the slide as an PDF file slide.SaveToFile("output/SlideToPDF.pdf", FileFormat.PDF)
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
