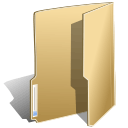
Conversion (15)
XML (Extensible Markup Language) is widely used for its structured format and readability on different platforms and systems. Its self-descriptive tags enable you to process data more easily. Meanwhile, Word XML focuses specifically on storing and exchanging Microsoft Word documents. It allows Word documents to transfer without loss. They both show flexibility under various scenarios that Word documents cannot achieve.
On the page, you will learn how to convert Word to XML and Word XML formats using Python with Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows.
Convert Word to XML in Python with Spire.Doc for Python
This part will explain how to convert Word documents to XML in Python with step-by-step instructions and a code example. Spire.Doc for Python provides the Document.SaveToFile() method to make it easy to save Word as XML. Check out the steps below and start processing your Word documents without effort!
Steps to Convert Word to XML:
- Create a new Document object.
- Load the Word document that you wish to be operated using Document.LoadFromFile() method.
- Covert it to XML by calling Document.SaveToFile() method.
Here's the code example:
- Python
from spire.doc import * from spire.doc.common import * # Create a Word document object document = Document() # Load the file from the disk document.LoadFromFile("sample.docx") # Save the document to an XML file document.SaveToFile("WordtoXML.xml", FileFormat.Xml) document.Close()
Convert Word to Word XML in Python
To convert Word to Word XML, you can utilize the Document.SaveToFile() method provided by Spire.Doc for Python. It not only helps to convert Word documents to Word XML but also to many other formats, such as PDF, XPS, HTML, RTF, etc.
Steps to Convert Word to Word XML:
- Create a new Document object.
- Load the Word document by Document.LoadFromFile() method.
- Convert it to Word XML using Document.SaveToFile() method.
Here's the code example for you:
- Python
from spire.doc import * from spire.doc.common import * # Create a Word document object document = Document() # Load the file from the disk document.LoadFromFile("sample.docx") # For Word 2003 document.SaveToFile("WordtoWordML.wordml", FileFormat.WordML) # For Word 2007-2013 document.SaveToFile("WordtoWordXML.wordxml", FileFormat.WordXml) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Markdown has become a popular choice for writing structured text due to its simplicity and readability, making it widely used for documentation, README files, and note-taking. However, sometimes there arises a need to present this content in a more universal and polished format, such as PDF, which is compatible across various devices and platforms without formatting inconsistencies. Converting Markdown files to PDF documents not only enhances portability but also adds a professional touch, enabling easier distribution for reports, manuals, or sharing content with non-technical audiences who may not be familiar with Markdown syntax.
This article will demonstrate how to convert Markdown files to PDF documents using Spire.Doc for Python to automate the conversion process.
- Convert Markdown Files to PDF Documents with Python
- Convert Markdown to PDF and Customize Page Settings
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to: How to Install Spire.Doc for Python on Windows
Convert Markdown Files to PDF Documents with Python
With Spire.Doc for Python, developers can load Markdown files using Document.LoadFromFile(string: fileName, FileFormat.Markdown) method, and then save the files to PDF documents using Document.SaveToFile(string: fileName, FileFormat.PDF) method. Besides, developers can also convert Markdown files to HTML, XPS, and SVG formats by specifying enumeration items of the FileFormat enumeration class.
The detailed steps for converting a Markdown file to a PDF document are as follows:
- Create an instance of Document class.
- Load a Markdown file using Document.LoadFromFile(string: fileName, FileFormat.Markdown) method.
- Convert the Markdown file to a PDF document and save it using Document.SaveToFile(string: fileName, FileFormat.PDF) method.
- Python
from spire.doc import * from spire.doc.common import * # Create an object of Document class doc = Document() # Load a Markdown file doc.LoadFromFile("Sample.md", FileFormat.Markdown) # Save the file to a PDF document doc.SaveToFile("output/MarkdownToPDF.pdf", FileFormat.PDF) doc.Dispose()
Convert Markdown to PDF and Customize Page Settings
Spire.Doc for Python supports performing basic page setup before converting Markdown files to formats like PDF, allowing for control over the appearance of the converted document.
The detailed steps to convert a Markdown file to a PDF document and customize the page settings are as follows:
- Create an instance of Document class.
- Load a Markdown file using Document.LoadFromFile(string: fileName, FileFormat.Markdown) method.
- Get the default section using Document.Sections.get_Item() method.
- Get the page settings through Section.PageSetup property and set the page size, orientation, and margins through properties under PageSetup class.
- Convert the Markdown file to a PDF document and save it using Document.SaveToFile(string: fileName, FileFormat.PDF) method.
- Python
from spire.doc import * from spire.doc.common import * # Create an instance of Document class doc = Document() # Load a Word document doc.LoadFromFile("Sample.md", FileFormat.Markdown) # Get the default section section = doc.Sections.get_Item(0) # Get the page settings pageSetup = section.PageSetup # Customize the page settings pageSetup.PageSize = PageSize.A4() pageSetup.Orientation = PageOrientation.Landscape pageSetup.Margins.All = 50 # Save the Markdown document to a PDF file doc.SaveToFile("output/MarkdownToPDFPageSetup.pdf", FileFormat.PDF) doc.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Markdown is a lightweight markup language that is becoming increasingly popular for writing content on the web. It offers a simple and human-readable syntax for formatting text, adding links, images, lists, and more. Many websites and content management systems support Markdown, as it can be easily converted to HTML. On the other hand, Microsoft Word is a widely used word-processing software that utilizes its own proprietary file format. While Word offers robust formatting options, its files are not always compatible with other platforms or content management systems.
In certain scenarios, it is useful to convert between Word and Markdown file formats. It allows you to take advantage of Word's advanced editing tools while also being able to publish your content in a web-friendly Markdown format. In this article, we will demonstrate how to convert Markdown to Word DOC or DOCX and convert Word DOC or DOCX to Markdown in Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert Markdown to Word in Python
You can load a Markdown file using the Document.LoadFromFile(fileName, FileFormat.Markdown) method and then convert it to Word DOC or DOCX format using the Document.SaveToFile(fileName, FileFormat.Doc) or Document.SaveToFile(fileName, FileFormat.Docx) method. The detailed steps are as follows.
- Create an object of the Document class.
- Load a Markdown file using the Document.LoadFromFile(fileName, FileFormat.Markdown) method.
- Save the Markdown file to a Word DOC or DOCX file using Document.SaveToFile(fileName, FileFormat.Doc) or Document.SaveToFile(fileName, FileFormat.Docx) method.
- Python
from spire.doc import * from spire.doc.common import * # Create an object of the Document class document = Document() # Load a Markdown file document.LoadFromFile("input.md") # Save the Markdown file to a Word DOCX file document.SaveToFile("MdToDocx.docx", FileFormat.Docx) # Save the Markdown file to a Word DOC file document.SaveToFile("MdToDoc.doc", FileFormat.Doc) document.Close()
Convert Word to Markdown in Python
You are also able to convert a Word DOC or DOCX file to Markdown format using the Document.SaveToFile(fileName, FileFormat.Markdown) method. The detailed steps are as follows.
- Create an object of the Document class.
- Load a Word DOC or DOCX file using the Document.LoadFromFile(fileName) method.
- Save the Word DOC or DOCX file to a Markdown file using Document.SaveToFile(fileName, FileFormat.Markdown) method.
- Python
from spire.doc import * from spire.doc.common import * # Create an object of the Document class document = Document() # Load a Word DOCX file document.LoadFromFile("input.docx") # Or load a Word DOC file #document.LoadFromFile("input.doc") # Save the Word file to a Markdown file document.SaveToFile("WordToMarkdown.md", FileFormat.Markdown) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Converting a document from Word to TIFF can be useful when you need to share the content as an image file, such as for electronic forms, presentations, or publishing. The TIFF format preserves the visual layout and appearance of the document. Conversely, converting a TIFF image to a Word document can be helpful when you want to present information in the Word format.
This article demonstrates how to convert Word to TIFF and TIFF to Word (non-editable) using Python and the Spire.Doc for Python library.
Install the Required Libraries
This situation relies on the combination of Spire.Doc for Python and Pillow (PIL). Spire.Doc is used to read, create and convert Word documents, while the PIL library is used for handling TIFF files and accessing their frames.
The libraries can be easily installed on your device through the following pip commands.
pip install Spire.Doc pip install pillow
Convert Word to TIFF in Python
To convert a Word document into a TIFF image, the initial step is to use the Spire.Doc library to load the Word document and transform the individual pages into image data streams. Then, you can leverage the functionality provided by the PIL to merge these separate image streams into a unified TIFF image.
The following are the steps to convert Word to TIFF using Python.
- Create a Document object.
- Load a Word document from a specified file path.
- Iterate through the pages in the document.
- Convert each page into an image stream using Document.SaveImageToSteams() method.
- Convert the image stream into a PIL image.
- Combine these PIL images into a single TIFF image.
- Python
from spire.doc import * from spire.doc.common import * from PIL import Image from io import BytesIO # Create a Document object doc = Document() # Load a Word document doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.docx") # Create an empty list to store PIL Images images = [] # Iterate through pages in the document for i in range(doc.GetPageCount()): # Convert a specific page to image stream with doc.SaveImageToStreams(i, ImageType.Bitmap) as imageData: # Open a specific image stream as a PIL image img = Image.open(BytesIO(imageData.ToArray())) # Append the PIL image to list images.append(img) # Save the PIL Images as a multi-page TIFF file images[0].save("Output/ToTIFF.tiff", save_all=True, append_images=images[1:]) # Dispose resources doc.Dispose()
Convert TIFF to Word in Python
By utilizing PIL library, you can load a TIFF file and break it down into separate PNG images for each frame. You can then utilize the Spire.Doc library to incorporate these separate PNG files as distinct pages within a Microsoft Word document.
To convert a TIFF image to a Word document using Python, follow these steps.
- Create a Document object.
- Add a section to it and set the page margins to zero.
- Load a TIFF image.
- Iterate though the frames in the TIFF image.
- Get a specific frame, and save it as a PNG file.
- Add a paragraph to the section.
- Append the image file to the paragraph.
- Set the page size to be the same as the image size.
- Save the document to a Word file.
- Python
from spire.doc import * from spire.doc.common import * from PIL import Image import io # Create a Document object doc = Document() # Add a section section = doc.AddSection() # Set margins to 0 section.PageSetup.Margins.All = 0.0 # Load a TIFF image tiff_image = Image.open("C:\\Users\\Administrator\\Desktop\\TIFF.tiff") # Iterate through the frames in it for i in range(tiff_image.n_frames): # Go to the current frame tiff_image.seek(i) # Extract the image of the current frame frame_image = tiff_image.copy() # Save the image to a PNG file frame_image.save(f"temp/output_frame_{i}.png") # Add a paragraph paragraph = section.AddParagraph() # Append image to the paragraph image = paragraph.AppendPicture(f"temp/output_frame_{i}.png") # Get image width and height width = image.Width height = image.Height # Set the page size to be the same as the image size section.PageSetup.PageSize = SizeF(width, height) # Save the document to a Word file doc.SaveToFile("Output/ToWord.docx",FileFormat.Docx2019) # Dispose resources doc.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Converting Word documents to XPS, PostScript, and OFD documents is of significant importance. Firstly, this conversion makes it easier to share and display documents across different platforms and applications, as these formats typically have broader compatibility.
Secondly, converting to these formats can preserve the document's formatting, layout, and content, ensuring consistent display across different systems.
Additionally, XPS and OFD formats support high-quality printing, helping to maintain the visual appearance and print quality of the document. The PostScript format is commonly used for printing and graphic processing, converting to PostScript can ensure that the document maintains high quality when printed.
In this article, you will learn how to convert Word to XPS, PostScript, or OFD with Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert Word to XPS in Python
The Document.SaveToFile(filename:str, FileFormat.XPS) method provided by Spire.Doc for Python can convert a Word document to XPS format. The detailed steps are as follows:
- Create an object of the Document class.
- Use the Document.LoadFromFile() method to load the Word document.
- Use the Document.SaveToFile(filename:str, FileFormat.XPS) method to convert the Word document to an XPS document.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object doc = Document() # Load a Word document doc.LoadFromFile("Sample.docx") # Save the loaded document as an XPS document doc.SaveToFile("Result.xps", FileFormat.XPS) # Close the document object and release the resources occupied by the document object doc.Close() doc.Dispose()
Convert Word to PostScript in Python
With Document.SaveToFile(filename:str, FileFormat.PostScript) method in Spire.Doc for Python, you can convert a Word document to PostScript format. The detailed steps are as follows:
- Create an object of the Document class.
- Use the Document.LoadFromFile() method to load the Word document.
- Use the Document.SaveToFile(filename:str, FileFormat.PostScript) method to convert the Word document to a PostScript document.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object doc = Document() # Load a Word document doc.LoadFromFile("Sample.docx") # # Save the loaded document as a PostScript document doc.SaveToFile("Result.ps", FileFormat.PostScript) # Close the document object and release the resources occupied by the document object doc.Close() doc.Dispose()
Convert Word to OFD in Python
By utilizing the Document.SaveToFile() method in the Spire.Doc for Python library and specifying the file format as FileFormat.OFD, you can save a Word document as an OFD file format. The detailed steps are as follows:
- Create an object of the Document class.
- Use the Document.LoadFromFile() method to load the Word document.
- Use the Document.SaveToFile(filename:str, FileFormat.OFD) method to convert the Word document to an OFD document.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object doc = Document() # Load a Word document doc.LoadFromFile("Sample.docx") # Save the loaded document as an OFD document doc.SaveToFile("Result.ofd", FileFormat.OFD) # Close the document object and release the resources occupied by the document object doc.Close() doc.Dispose()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
The conversion from HTML to image allows you to capture the appearance and layout of the HTML content as a static image file. It can be useful for various purposes, such as generating website previews, creating screenshots, archiving web pages, or integrating HTML content into applications that primarily deal with images. In this article, you will learn how to convert an HTML file or an HTML string to an image in Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert an HTML File to an Image in Python
When an HTML file is loaded into the Document object using the Document.LoadFromFile() method, its contents are automatically rendered as the contents of a Word page. Then, a specific page can be saved as an image stream using the Document.SaveImageToStreams() method.
The following are the steps to convert an HTML file to an image with Python.
- Create a Document object.
- Load a HTML file using Document.LoadFromFile() method.
- Convert a specific page to an image stream using Document.SaveImageToStreams() method.
- Save the image stream as a PNG file using BufferedWriter.write() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load an HTML file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.html", FileFormat.Html, XHTMLValidationType.none) # Save the first page as an image stream imageStream = document.SaveImageToStreams(0, ImageType.Bitmap) # Convert the image stream as a PNG file with open("output/HtmlToImage.png",'wb') as imageFile: imageFile.write(imageStream.ToArray()) document.Close()
Convert an HTML String to an Image in Python
To render uncomplicated HTML strings (typically text and its formatting) as a Word page, you can utilize the Paragraph.AppendHTML() method. Afterwards, you can convert it to an image stream using the Document.SaveImageToStreams() method.
The following are the steps to convert an HTML string to an image in Python.
- Create a Document object.
- Add a section using Document.AddSection() method.
- Add a paragraph using Section.AddParagraph() method.
- Specify the HTML string, and add the it to the paragraph using Paragraph.AppendHTML() method.
- Convert a specific page to an image stream using Document.SaveImageToStreams() method.
- Save the image stream as a PNG file using BufferedWriter.write() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Add a section to the document sec = document.AddSection() # Add a paragraph to the section paragraph = sec.AddParagraph() # Specify the HTML string htmlString = """ <html> <head> <title>HTML to Word Example</title> <style> body { font-family: Arial, sans-serif; } h1 { color: #FF5733; font-size: 24px; margin-bottom: 20px; } p { color: #333333; font-size: 16px; margin-bottom: 10px; } ul { list-style-type: disc; margin-left: 20px; margin-bottom: 15px; } li { font-size: 14px; margin-bottom: 5px; } table { border-collapse: collapse; width: 100%; margin-bottom: 20px; } th, td { border: 1px solid #CCCCCC; padding: 8px; text-align: left; } th { background-color: #F2F2F2; font-weight: bold; } td { color: #0000FF; } </style> </head> <body> <h1>This is a Heading</h1> <p>This is a paragraph.</p> <p>Here's an unordered list:</p> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <p>And here's a table:</p> <table> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> </tr> <tr> <td>John Smith</td> <td>35</td> <td>Male</td> </tr> <tr> <td>Jenny Garcia</td> <td>27</td> <td>Female</td> </tr> </table> </body> </html> """ # Append the HTML string to the paragraph paragraph.AppendHTML(htmlString) # Save the first page as an image stream imageStream = document.SaveImageToStreams(0, ImageType.Bitmap) # Convert the image stream as a PNG file with open("output/HtmlToImage2.png",'wb') as imageFile: imageFile.write(imageStream.ToArray()) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
HTML is the standard markup language for creating web pages, while PDF is a widely accepted format for sharing and preserving documents with consistent formatting across different platforms. By converting HTML to PDF, you can create printable documents, share web content offline, or generate reports with ease. In this article, you will learn how to convert a HTML file or a HTML string to PDF in Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert an HTML File to PDF in Python
The Document.LoadFromFile method supports loading not only Doc or Docx files, but also HTML files. You can load an HTML file using this method and save it as a PDF file using the Document.SaveToFile() method. The following are the steps to convert an HTML file to PDF in Python.
- Create a Document object.
- Load an HTML file using Document.LoadFromFile() method.
- Convert it to PDF using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load an HTML file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Sample.html", FileFormat.Html, XHTMLValidationType.none) # Save the HTML file to a pdf file document.SaveToFile("output/ToPdf.pdf", FileFormat.PDF) document.Close()
Convert an HTML String to PDF in Python
To render uncomplicated HTML strings (usually text and its formatting) on Word pages, you can use the Paragraph.AppendHTML() method. The Word document can be then saved as a PDF file using the Document.SaveToFile() method. The following are the steps to convert an HTML string to PDF in Python.
- Create a Document object.
- Add a section using Document.AddSection() method.
- Add a paragraph using Section.AddParagraph() method.
- Specify the HTML string.
- Add the HTML string to the paragraph using Paragraph.AppendHTML() method.
- Save the document as a PDF file using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Add a section to the document sec = document.AddSection() # Add a paragraph to the section paragraph = sec.AddParagraph() # Specify the HTML string htmlString = """ <html> <head> <title>HTML to Word Example</title> <style> body { font-family: Arial, sans-serif; } h1 { color: #FF5733; font-size: 24px; margin-bottom: 20px; } p { color: #333333; font-size: 16px; margin-bottom: 10px; } ul { list-style-type: disc; margin-left: 20px; margin-bottom: 15px; } li { font-size: 14px; margin-bottom: 5px; } table { border-collapse: collapse; width: 100%; margin-bottom: 20px; } th, td { border: 1px solid #CCCCCC; padding: 8px; text-align: left; } th { background-color: #F2F2F2; font-weight: bold; } td { color: #0000FF; } </style> </head> <body> <h1>This is a Heading</h1> <p>This is a paragraph.</p> <p>Here's an unordered list:</p> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <p>And here's a table:</p> <table> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> </tr> <tr> <td>John Smith</td> <td>35</td> <td>Male</td> </tr> <tr> <td>Jenny Garcia</td> <td>27</td> <td>Female</td> </tr> </table> </body> </html> """ # Append the HTML string to the paragraph paragraph.AppendHTML(htmlString) # Save the document as a pdf file document.SaveToFile("output/HtmlStringToPdf.pdf", FileFormat.PDF) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
EPUB, short for Electronic Publication, is a widely used standard format for eBooks. It is an open and free format based on web standards, enabling compatibility with various devices and software applications. EPUB files are designed to provide a consistent reading experience across different platforms, including e-readers, tablets, smartphones, and computers. By converting your Word document to EPUB, you can ensure that your content is accessible and enjoyable to a broader audience, regardless of the devices and software they use. In this article, we will demonstrate how to convert Word documents to EPUB format in Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert Word to EPUB in Python
The Document.SaveToFile(fileName:str, fileFormat:FileFormat) method provided by Spire.Doc for Python supports converting a Word document to EPUB format. The detailed steps are as follows.
- Create an object of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Save the Word document to EPUB format using Document.SaveToFile(fileName:str, fileFormat:FileFormat) method.
- Python
from spire.doc import * from spire.doc.common import * # Specify the input Word document and output EPUB file paths inputFile = "Sample.docx" outputFile = "ToEpub.epub" # Create an object of the Document class doc = Document() # Load a Word document doc.LoadFromFile(inputFile) # Save the Word document to EPUB format doc.SaveToFile(outputFile, FileFormat.EPub) # Close the Document object doc.Close()
Convert Word to EPUB with a Cover Image in Python
Spire.Doc for Python enables you to convert a Word document to EPUB format and set a cover image for the resulting EPUB file by using the Document.SaveToEpub(fileName:str, coverImage:DocPicture) method. The detailed steps are as follows.
- Create an object of the Document class.
- Load a Word document using Document.LoadFromFile() method.
- Create an object of the DocPicture class, and then load an image using DocPicture.LoadImage() method.
- Save the Word document as an EPUB file and set the loaded image as the cover image of the EPUB file using Document.SaveToEpub(fileName:str, coverImage:DocPicture) method.
- Python
from spire.doc import * from spire.doc.common import * # Specify the input Word document and output EPUB file paths inputFile = "Sample.docx" outputFile = "ToEpubWithCoverImage.epub" # Specify the file path for the cover image imgFile = "Cover.png" # Create a Document object doc = Document() # Load the Word document doc.LoadFromFile(inputFile) # Create a DocPicture object picture = DocPicture(doc) # Load the cover image file picture.LoadImage(imgFile) # Save the Word document as an EPUB file and set the cover image doc.SaveToEpub(outputFile, picture) # Close the Document object doc.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
RTF (Rich Text Format) is a versatile file format that can be opened and viewed by various word processing software. It supports a wide range of text formatting options, such as font style, size, color, tables, images, and more. When working with RTF files, you may sometimes need to convert them to PDF files for better sharing and printing, or to HTML format for publishing on the web. In this article, you will learn how to convert RTF to PDF or HTML with Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip commands.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert RTF to PDF in Python
To convert an RTF file to PDF, simply load a file with .rtf extension and then save it as a PDF file using Document.SaveToFile(fileName, FileFormat.PDF) method. The following are the detailed steps.
- Create a Document object.
- Load an RTF file using Document.LoadFromFile() method.
- Save the RTF file as a PDF file using Document.SaveToFile(fileName, FileFormat.PDF) method.
- Python
from spire.doc import * from spire.doc.common import * inputFile = "input.rtf" outputFile = "RtfToPDF.pdf" # Create a Document object doc = Document() # Load an RTF file from disk doc.LoadFromFile(inputFile) # Save the RTF file as a PDF file doc.SaveToFile(outputFile, FileFormat.PDF) doc.Close()
Convert RTF to HTML in Python
Spire.Doc for Python also allows you to use the Document.SaveToFile(fileName, FileFormat.Html) method to convert the loaded RTF file to HTML format. The following are the detailed steps.
- Create a Document object.
- Load an RTF file using Document.LoadFromFile() method.
- Save the RTF file in HTML format using Document.SaveToFile(fileName, FileFormat.Html) method.
- Python
from spire.doc import * from spire.doc.common import * inputFile = "input.rtf" outputFile = "RtfToHtml.html" # Create a Document object doc = Document() # Load an RTF file from disk doc.LoadFromFile(inputFile) # Save the RTF file in HTML format doc.SaveToFile(outputFile, FileFormat.Html) doc.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
RTF is a flexible file format that preserves formatting and basic styling while offering compatibility with various word processing software. Converting Word to RTF enables users to retain document structure, fonts, hyperlinks, and other essential elements without the need for specialized software. Similarly, converting RTF back to Word format provides the flexibility to edit and enhance documents using the powerful features of Microsoft Word. In this article, you will learn how to convert Word to RTF and vice versa in Python using Spire.Doc for Python.
Install Spire.Doc for Python
This scenario requires Spire.Doc for Python and plum-dispatch v1.7.4. They can be easily installed in your Windows through the following pip command.
pip install Spire.Doc
If you are unsure how to install, please refer to this tutorial: How to Install Spire.Doc for Python on Windows
Convert Word to RTF in Python
With Spire.Doc for Python, you can load a Word file using the Document.LoadFromFile() method and convert it to a different format, such as RTF, using the Document.SaveToFile() method; Conversely, you can load an RTF file in the same way and save it as a Word file.
The following are the steps to convert Word to RTF using Spire.Doc for Python.
- Create a Document object.
- Load a Word file using Document.LoadFromFile() method.
- Convert it to an RTF file using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load a Word file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.docx") # Convert to a RTF file document.SaveToFile("output/ToRtf.rtf", FileFormat.Rtf) document.Close()
Convert RTF to Word in Python
The code for converting RTF to Word is quite simply, too. Follow the steps below.
- Create a Document object.
- Load an RTF file using Document.LoadFromFile() method.
- Convert it to a Word file using Document.SaveToFile() method.
- Python
from spire.doc import * from spire.doc.common import * # Create a Document object document = Document() # Load a Rtf file document.LoadFromFile("C:\\Users\\Administrator\\Desktop\\input.rtf") # Convert to a Word file document.SaveToFile("output/ToWord.docx", FileFormat.Docx2019) document.Close()
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
