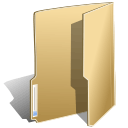
Spire.Barcode for Python (1)
Children categories
QR codes are a type of two-dimensional barcode that can store a variety of information, including URLs, contact details, and even payment information. QR codes have become increasingly popular, allowing for quick and convenient access to digital content, making them a useful tool in our modern, technology-driven world.
In this article, you will learn how to create and scan QR codes in Python using Spire.Barcode for Python.
Get a Free Trial License
The trial version of Spire.Barcode for Python does not support scanning QR code images without a valid license being applied. Additionally, it displays an evaluation message on any QR code images that are generated.
To remove these limitations, you can get a 30-day trial license for free.
Create a QR Code in Python
Spire.Barcode for Python offers the BarcodeSettings class, which enables you to configure the settings for generating a barcode. These settings encompass the barcode type, the data to be encoded, the color, the margins, and the horizontal and vertical resolution.
After you have set up the desired settings, you can create a BarcodeGenerator instance using those configurations. Subsequently, you can invoke the GenerateImage() method of the generator to produce the barcode image.
The following are the steps to create a QR code in Python.
- Create a BarcodeSettings object.
- Set the barcode type to QR code using BarcodeSettings.Type property.
- Set the data of the 2D barcode using BarcodeSettings.Data2D property.
- Set other attributes of the barcode using the properties under the BarcodeSettings object.
- Create a BarCodeGenerator object based on the settings.
- Create a QR code image using BarCodeGenerator.GenerateImage() method.
- Python
from spire.barcode import * # Write all bytes to a file def WriteAllBytes(fname: str, data): with open(fname, "wb") as fp: fp.write(data) fp.close() # Apply license key License.SetLicenseKey("license key") # Create a BarcodeSettings object barcodeSettings = BarcodeSettings() # Set the type of barcode to QR code barcodeSettings.Type = BarCodeType.QRCode # Set the data for the 2D barcode barcodeSettings.Data2D = "Hello, World" # Set margins barcodeSettings.LeftMargin = 0.2 barcodeSettings.RightMargin = 0.2 barcodeSettings.TopMargin = 0.2 barcodeSettings.BottomMargin = 0.2 # Set the horizontal resolution barcodeSettings.DpiX = 500 # Set the vertical resolution barcodeSettings.DpiY = 500 # Set error correction level barcodeSettings.QRCodeECL = QRCodeECL.M # Do not display text on barcode barcodeSettings.ShowText = False # Add a logo at the center of the QR code barcodeSettings.SetQRCodeLogoImage("C:\\Users\\Administrator\\Desktop\\logo.png") # Create an instance of BarCodeGenerator with the specified settings barCodeGenerator = BarCodeGenerator(barcodeSettings) # Generate the image for the barcode image = barCodeGenerator.GenerateImage() # Write the PNG image to disk WriteAllBytes("output/QRCode.png", image)
Scan a QR Code Image in Python
Spire.Barcode provides the BarcodeScanner class, which is responsible for barcode image recognition. This class offers several methods to extract data from barcodes, including:
- ScanOneFile(): Scans a single barcode image file and returns the extracted data.
- ScanFile(): Scans all barcodes present in a specified image file and returns the extracted data.
- ScanStream(): Scans barcodes from a stream of image data and returns the extracted information.
The following code demonstrates how to scan a QR code image using it.
- Python
from spire.barcode import * # Apply license key License.SetLicenseKey("license key") # Scan an image file that contains one barcode result = BarcodeScanner.ScanOneFile("C:\\Users\\Administrator\\Desktop\\QRCode.png") # Scan an image file that contains multiple barcodes # results = BarcodeScanner.ScanFile("C:\\Users\\Administrator\\Desktop\\Image.png") # Print the result print(result)
