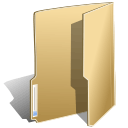
Page Setup (9)
Page borders can be a useful design element in Microsoft Word documents. They can help to frame the content and provide a polished, professional. Page borders draw the reader's eye to the main content area and create a sense of structure and cohesion. Conversely, you may want to remove page borders if they are not needed or if they distract from the content.
In this article, you will learn how to add, adjust, and remove page borders in a Word document using Java and Spire.Doc for Java.
- Add Page Borders to a Word Document
- Adjust Page Borders in a Word Document
- Remove Page Borders from a Word Document
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Add Page Borders to a Word Document in Java
Spire.Doc for Java includes the Borders class, which enables developers to manage the page borders in a Word document. This class provides a collection of methods that allow you to control various aspects of the page border, such as the border type, color, and line width.
To add borders to all pages in a Word document using Java, the general steps are as follows:
- Create a Document object.
- Load a Word file from the given file path.
- Iterate through the sections in the document.
- Get a specific section.
- Get the PageSetup object of the section.
- Apply borders to all page by passing PageBordersApplyType.All_Pages as the parameter of PageSetup.setPageBordersApplyType() method.
- Get the Borders object using PageSetup.getBorders() method.
- Set the border type, color, line width and other attributes using the methods under the Borders object.
- Save the updated document to a different Word file.
- Java
import com.spire.doc.*; import com.spire.doc.documents.BorderStyle; import java.awt.*; public class AddPageBorder { public static void main(String[] args) { // Create a Document object Document doc = new Document(); // Load a Word file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.docx"); // Iterate through the sections in the document for (int i = 0; i < doc.getSections().getCount(); i++) { // Get a specific section Section section = doc.getSections().get(i); // Get page setup object PageSetup pageSetup = section.getPageSetup(); // Apply page border to all pages pageSetup.setPageBordersApplyType(PageBordersApplyType.All_Pages); // Set the border type pageSetup.getBorders().setBorderType(BorderStyle.Dash_Large_Gap); // Set the border width pageSetup.getBorders().setLineWidth(2); // Set the border color pageSetup.getBorders().setColor(Color.RED); // Set the spacing between borders and text within them pageSetup.getBorders().getTop().setSpace(30); pageSetup.getBorders().getBottom().setSpace(30); pageSetup.getBorders().getLeft().setSpace(30); pageSetup.getBorders().getRight().setSpace(30); } // Save the updated document to a different file doc.saveToFile("AddPageBorder.docx", FileFormat.Docx); // Dispose resources doc.dispose(); } }
Adjust Page Borders in a Word Document in Java
The page borders of an existing Word document can be obtained using the PageSetup.getBorders() method. You can change the appearance of the page borders using the setBorderType(), setColor(), and setLineWidth() methods.
The steps to adjust page borders in a Word document using Java are as follows.
- Create a Document object.
- Load a Word file from the given file path.
- Iterate through the sections in the document.
- Get a specific section.
- Get the PageSetup object of the section.
- Get the Borders object using PageSetup.getBorders() method.
- Set the border type, color, line width and other attributes using the methods under the Borders object.
- Save the updated document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.PageSetup; import com.spire.doc.Section; import com.spire.doc.documents.BorderStyle; import java.awt.*; public class AdjustPageBorders { public static void main(String[] args) { // Create a Document object Document doc = new Document(); // Load a Word file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Borders.docx"); // Iterate through the sections in the document for (int i = 0; i < doc.getSections().getCount(); i++) { // Get a specific section Section section = doc.getSections().get(i); // Get page setup of the section PageSetup pageSetup = section.getPageSetup(); // Change the border type section.getPageSetup().getBorders().setBorderType(BorderStyle.Double); // Change the border color section.getPageSetup().getBorders().setColor(Color.DARK_GRAY); // Change the border width section.getPageSetup().getBorders().setLineWidth(3); } // Save the updated document to a different file doc.saveToFile("AdjustBorder.docx", FileFormat.Docx); // Dispose resources doc.dispose(); } }
Remove Page Borders from a Word Document in Java
To move page borders from a Word document, pass the BorderStyle.None as the parameter of the Borders.setBorderType() method. By setting the border type as none, you are instructing the document to remove any existing page borders, resulting in a clean, border-free document layout.
The steps to remove page borders from a Word document using Java are as follows:
- Create a Document object.
- Load a Word file from the given file path.
- Iterate through the sections in the document.
- Get a specific section.
- Get the PageSetup object of the section.
- Get the Borders object using PageSetup.getBorders() method.
- Set the border type as BorderStyle.None using Borders.setBorderType() method.
- Save the updated document to a different Word file.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.PageSetup; import com.spire.doc.Section; import com.spire.doc.documents.BorderStyle; public class RemovePageBorders { public static void main(String[] args) { // Create a Document object Document doc = new Document(); // Load a Word file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Borders.docx"); // Iterate through the sections in the document for (int i = 0; i < doc.getSections().getCount(); i++) { // Get a specific section Section section = doc.getSections().get(i); // Get page setup object PageSetup pageSetup = section.getPageSetup(); // Set the border type to none pageSetup.getBorders().setBorderType(BorderStyle.None); } // Save the updated document to a different file doc.saveToFile("RemovePageBorders.docx", FileFormat.Docx); // Dispose resources doc.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Adding gutters on Word document pages can assist users in better page layout and design, especially when preparing documents for printing or creating books that require gutter. Gutter can indicate the gutter position on the page, helping users to design with appropriate blank areas to avoid text or images being cut off. By setting gutter, users can have better control over the appearance and layout of the document, ensuring that the final output meets the expected gutter requirements, enhancing the professionalism and readability of the document. This article shows how to add gutters on Word document pages by programming using Spire.Doc for Java.
- Add a Gutter at the Top of a Word Document Page in Java
- Add a Gutter at the Left of a Word Document Page in Java
Install Spire.Doc for Java
First, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Add a Gutter at the Top of a Word Document Page in Java
In a Word document, you can set section.getPageSetup().isTopGutter(true) to place the gutter at the top of the page. By default, the gutter area is displayed as blank without any content. This example also includes steps on how to add content, such as the dash symbol, to the gutter area to customize content around the gutter. Here are the detailed steps:
- Create a Document object.
- Load a document using the Document.loadFromFile() method.
- Iterate through all sections of the document using a for loop and Document.getSections().
- Set Section.getPageSetup().isTopGutter(true) to display the gutter at the top of the page.
- Use Section.getPageSetup().setGutter() to set the width of the gutter.
- Call the custom addTopGutterContent() method to add content to the gutter area.
- Save the document using the Document.saveToFile() method.
- Java
import com.spire.doc.*; import com.spire.doc.documents.*; import com.spire.doc.fields.*; import com.spire.doc.formatting.CharacterFormat; import java.awt.*; public class AddTopGutter { public static void main(String[] args) { // Create a document object Document document = new Document(); // Load the document document.loadFromFile("Sample1.docx"); // Iterate through all sections of the document for (int i = 0; i < document.getSections().getCount(); i++) { // Get the current section Section section = document.getSections().get(i); // Set whether to add a gutter at the top of the page to true section.getPageSetup().isTopGutter(true); // Set the width of the gutter to 100f section.getPageSetup().setGutter(100f); // Call the method to add content to the top gutter addTopGutterContent(section); } // Save the modified document to a file document.saveToFile("AddGutterOnTop.docx", FileFormat.Docx_2016); // Release document resources document.dispose(); } // Method to add content to the top gutter static void addTopGutterContent(Section section) { // Get the header of the section HeaderFooter header = section.getHeadersFooters().getHeader(); // Set the width of the text box to the width of the page float width = (float) section.getPageSetup().getPageSize().getWidth(); // Set the height of the text box to 40 float height = 40; // Add a text box to the header TextBox textBox = header.addParagraph().appendTextBox(width, height); // Set the text box without borders textBox.getFormat().setNoLine(true); // Set the vertical origin of the text box to the top margin area textBox.setVerticalOrigin(VerticalOrigin.Top_Margin_Area); // Set the vertical position of the text box textBox.setVerticalPosition(140); // Set the horizontal alignment of the text box to left textBox.setHorizontalAlignment(ShapeHorizontalAlignment.Left); // Set the horizontal origin of the text box to the left margin area textBox.setHorizontalOrigin(HorizontalOrigin.Left_Margin_Area); // Set the text anchor to the bottom textBox.getFormat().setTextAnchor(ShapeVerticalAlignment.Bottom); // Set the text wrapping style to in front of text textBox.getFormat().setTextWrappingStyle(TextWrappingStyle.In_Front_Of_Text); // Set the text wrapping type to both sides textBox.getFormat().setTextWrappingType(TextWrappingType.Both); // Create a paragraph object Paragraph paragraph = new Paragraph(section.getDocument()); // Set the paragraph alignment to center paragraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Center); // Create a font object Font font = new Font("SimSun", Font.PLAIN, 8); Graphics graphics = new java.awt.image.BufferedImage(1, 1, java.awt.image.BufferedImage.TYPE_INT_ARGB).getGraphics(); graphics.setFont(font); FontMetrics fontMetrics = graphics.getFontMetrics(); String text1 = " - "; int textWidth1 = fontMetrics.stringWidth(text1); int count = (int) (textBox.getWidth() / textWidth1); StringBuilder stringBuilder = new StringBuilder(); for (int i = 1; i < count; i++) { stringBuilder.append(text1); } // Create a character format object CharacterFormat characterFormat = new CharacterFormat(section.getDocument()); characterFormat.setFontName(font.getFontName()); characterFormat.setFontSize(font.getSize()); TextRange textRange = paragraph.appendText(stringBuilder.toString()); textRange.applyCharacterFormat(characterFormat); // Add the paragraph to the text box textBox.getChildObjects().add(paragraph); } }
Add a Gutter at the Left of a Word Document Page in Java
To set the gutter on the left side of the page, the key is to set Section.getPageSetup().isTopGutter(false). Here are the detailed steps:
- Create a Document object.
- Load a document using the Document.loadFromFile() method.
- Iterate through all sections of the document using a for loop and Document.getSections().
- Set Section.getPageSetup().isTopGutter(false) to display the gutter on the left side of the page.
- Use Section.getPageSetup().setGutter() to set the width of the gutter.
- Call the custom addLeftGutterContent() method to add content to the gutter area.
- Save the document using the Document.saveToFile() method.
- Java
import com.spire.doc.*; import com.spire.doc.documents.*; import com.spire.doc.fields.*; import com.spire.doc.formatting.CharacterFormat; import java.awt.*; public class AddLeftGutter { public static void main(String[] args) { // Create a document object Document document = new Document(); // Load the document document.loadFromFile("Sample1.docx"); // Iterate through all sections of the document for (int i = 0; i < document.getSections().getCount(); i++) { // Get the current section Section section = document.getSections().get(i); // Set whether to add a gutter at the top of the page to false, it will be added to the left side of the page section.getPageSetup().isTopGutter(false); // Set the width of the gutter to 100f section.getPageSetup().setGutter(100f); // Call the method to add content to the left gutter AddLeftGutterContent (section); } // Save the modified document to a file document.saveToFile("AddGutterOnLeft.docx", FileFormat.Docx_2016); // Release document resources document.dispose(); } // Method to add content to the left gutter static void AddLeftGutterContent(Section section) { // Get the header of the section HeaderFooter header = section.getHeadersFooters().getHeader(); // Set the width of the text box to 40 float width = 40; // Get the page height float height = (float) section.getPageSetup().getPageSize().getHeight(); // Add a text box to the header TextBox textBox = header.addParagraph().appendTextBox(width, height); // Set the text box without borders textBox.getFormat().setNoLine(true); // Set the text direction in the text box from right to left textBox.getFormat().setLayoutFlowAlt(TextDirection.Right_To_Left); // Set the horizontal starting position of the text box textBox.setHorizontalOrigin(HorizontalOrigin.Left_Margin_Area); // Set the horizontal position of the text box textBox.setHorizontalPosition(140); // Set the vertical alignment of the text box to the top textBox.setVerticalAlignment(ShapeVerticalAlignment.Top); // Set the vertical origin of the text box to the top margin area textBox.setVerticalOrigin(VerticalOrigin.Top_Margin_Area); // Set the text anchor to the top textBox.getFormat().setTextAnchor(ShapeVerticalAlignment.Top); // Set the text wrapping style to in front of text textBox.getFormat().setTextWrappingStyle(TextWrappingStyle.In_Front_Of_Text); // Set the text wrapping type to both sides textBox.getFormat().setTextWrappingType(TextWrappingType.Both); // Create a paragraph object Paragraph paragraph = new Paragraph(section.getDocument()); // Set the paragraph alignment to center paragraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Center); // Create a font object, SimSun, size 8 Font font = new Font("SimSun", Font.PLAIN, 8); Graphics graphics = new java.awt.image.BufferedImage(1, 1, java.awt.image.BufferedImage.TYPE_INT_ARGB).getGraphics(); graphics.setFont(font); FontMetrics fontMetrics = graphics.getFontMetrics(); String text1 = " - "; int textWidth1 = fontMetrics.stringWidth(text1); int count = (int) (textBox.getHeight() / textWidth1); StringBuilder stringBuilder = new StringBuilder(); for (int i = 1; i < count ; i++) { stringBuilder.append(text1); } // Create a character format object CharacterFormat characterFormat = new CharacterFormat(section.getDocument()); characterFormat.setFontName(font.getFontName()); characterFormat.setFontSize(font.getSize()); TextRange textRange = paragraph.appendText(stringBuilder.toString()); textRange.applyCharacterFormat(characterFormat); // Add the paragraph to the text box textBox.getChildObjects().add(paragraph); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
By inserting a page break into your Word document, you can end a page at the place you want and begin a new page at once without hitting the enter key repeatedly. In this article, we will demonstrate how to insert page breaks into a Word document in Java using Spire.Doc for Java library.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Insert Page Break after a Specific Paragraph
The following are the steps to insert page break after a specific paragraph:
- Create a Document instance.
- Load a Word document using Document.loadFromFile() method.
- Get the desired section using Document.getSections().get(sectionIndex) method.
- Get the desired paragraph using Section.getParagraphs().get(paragraphIndex) method.
- Add a page break to the paragraph using Paragraph.appendBreak(BreakType.Page_Break) method.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.Section; import com.spire.doc.documents.BreakType; import com.spire.doc.documents.Paragraph; import com.spire.doc.FileFormat; public class InsertPageBreakAfterParagraph { public static void main(String[] args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the first section Section section = document.getSections().get(0); //Get the 2nd paragraph in the section Paragraph paragraph = section.getParagraphs().get(1); //Append a page break to the paragraph paragraph.appendBreak(BreakType.Page_Break); //Save the result document document.saveToFile("InsertPageBreak.docx", FileFormat.Docx_2013); } }
Insert Page Break after a Specific Text
The following are the steps to insert a page break after a specific text:
- Create a Document instance.
- Load a Word document using Document.loadFromFile() method.
- Find a specific text using Document.findString() method.
- Access the text range of the searched text using TextSelection.getAsOneRange() method.
- Get the paragraph where the text range is located using ParagraphBase.getOwnerParagraph() method.
- Get the position index of the text range in the paragraph using Paragraph.getChildObjects().indexOf() method.
- Initialize an instance of Break class to create a page break.
- Insert the page break after the searched text using Paragraph.getChildObjects().insert() method.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Break; import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.documents.BreakType; import com.spire.doc.documents.Paragraph; import com.spire.doc.documents.TextSelection; import com.spire.doc.fields.TextRange; public class InsertPageBreakAfterText { public static void main(String[] args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Search a specific text TextSelection selection = document.findString("celebration", true, true); //Get the text range of the seached text TextRange range = selection.getAsOneRange(); //Get the paragraph where the text range is located Paragraph paragraph = range.getOwnerParagraph(); //Get the position index of the text range in the paragraph int index = paragraph.getChildObjects().indexOf(range); //Create a page break Break pageBreak = new Break(document, BreakType.Page_Break); //Insert the page break after the searched text paragraph.getChildObjects().insert(index + 1, pageBreak); //Save the result document document.saveToFile("InsertPageBreakAfterText.docx", FileFormat.Docx_2013); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Gutter margins are designed to add extra space to the existing margins of a document, which ensures that the text of the document will not be obscured when binding. This is very useful when you need to bind some important official documents, books, or examination papers. This article will introduce how to set gutter margins on the left edges of the pages in a Word document using Spire.Doc for Java.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Set Gutter Margins in Word
Although you can adjust the normal margins to add more space for document binding, setting gutter margins is a more efficient way to achieve the same function. The following are the steps to set gutter margins in a Word document:
- Create a Document instance.
- Load a Word document using Document.loadFromFile() method.
- Get a specific section using Document.getSections().get() method.
- Set gutter margin for that specified section using Section.getPageSetup().setGutter() method.
- Save the document to file using Document.saveToFile() method.
- Java
import com.spire.doc.*; import java.io.IOException; public class addGutter { public static void main(String[] args) throws IOException { //Create a Document instance Document document = new Document(); //Load a sample Word document document.loadFromFile("input.docx"); //Get the first section Section section = document.getSections().get(0); //Set gutter margin section.getPageSetup().setGutter(100f); //Save the file document.saveToFile("addGutter_output.docx", FileFormat.Docx); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Java set the character spacing and paragraph spacing on Word document
2021-05-12 06:31:17 Written by support iceblueThis article will show you how to use Spire.Doc for Java to set the character spacing and paragraph spacing on Word.
import com.spire.doc.*; import com.spire.doc.documents.Paragraph; import com.spire.doc.fields.TextRange; import java.awt.*; import java.io.*; public class setSpacing { public static void main(String[] args)throws IOException { //Load the sample document Document document= new Document("Sample1.docx"); //Add a new paragraph and append the text Paragraph para = new Paragraph(document); TextRange textRange1 = para.appendText("Newly added paragraph and set the paragraph spacing and character spacing"); textRange1.getCharacterFormat().setTextColor(Color.blue); textRange1.getCharacterFormat().setFontSize(14); //Set the spacing before and after paragraph para.getFormat().setBeforeAutoSpacing(false); para.getFormat().setBeforeSpacing(10); para.getFormat().setAfterAutoSpacing(false); para.getFormat().setAfterSpacing(10); //Set the character spacing for (DocumentObject object :(Iterable<DocumentObject>)para.getChildObjects()) { TextRange textRange= (TextRange) object; textRange.getCharacterFormat().setCharacterSpacing(3f); } //Insert the paragraph document.getSections().get(0).getParagraphs().insert(2, para); //Save the document to file document.saveToFile("Result.docx", FileFormat.Docx); } }
Output:
Normally, the default page size of a Word document is “Letter” (8.5 x 11 inches), and the default page orientation is “Portrait”. In most cases, the general page setup can meet the needs of most users, but sometimes you may also need to adjust the page size and orientation to design a different document such as an application form, certificate, or brochure. In this article, you will learn how to programmatically change the page size and page orientation in a Word document using Spire.Doc for Java.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Change Page Size and Page Orientation in Word
The detailed steps are as follows:
- Create a Document instance.
- Load a sample Word document using Document.loadFromFile() method.
- Get the first section using Document.getSections().get() method.
- Change the default page size using Section.getPageSetup().setPageSize() method.
- Change the default page orientation using Section.getPageSetup().setOrientation() method.
- Save the document to file using Document.saveToFile() method.
- Java
import com.spire.doc.*; import com.spire.doc.documents.*; public class WordPageSetup { public static void main(String[] args) throws Exception { //Create a Document instance Document doc= new Document(); //Load a sample Word document doc.loadFromFile("sample.docx"); //Get the first section Section section = doc.getSections().get(0); //Change the page size to A3 section.getPageSetup().setPageSize(PageSize.A3); //Change the page orientation to Landscape section.getPageSetup().setOrientation(PageOrientation.Landscape); //Save the document to file doc.saveToFile("Result.docx",FileFormat.Docx_2013); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Page numbers in Word documents are marked on each page to indicate the order and the number of pages. They can facilitate document creators to manage the document content and help users quickly find specific content in the document, thus improving reading speed and reading experience. This article is going to show how to use Spire.Doc for Java to add page numbers to Word documents programmatically.
- Add Page Numbers to a Word Document in Java
- Restart Page Numbering for Each Section in a Word Document in Java
- Add Page Numbers to a Specific Section in a Word Document in Java
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Add Page Numbers to a Word Document in Java
Page numbers in Word are displayed using specific types of fields. For example, the Page field displays the page number of the current page, the NumPages field displays the total number of pages in a document, and the SectionPages field displays the total number of pages in a section.
Spire.Doc for Java provides the Paragraph.appendField(String fieldName, FieldType fieldType) method to add various types of fields to a Word document, including the Page field (FieldType.Field_Page), the NumPages field (FieldType.Field_Num_Pages), and the SectionPages field (FieldType.Field_Section_Pages).
The following steps explain how to add a Page field and a NumPages field to the footer of a Word document to display the current page number and the total number of pages in the document using Spire.Doc for Java:
- Create an object of Document class.
- Load a Word document using Document.loadFromFile() method.
- Get the first section using Document.getSections().get() method.
- Get the footer of the first section using Section.getHeadersFooters().getFooter() method.
- Add a paragraph to the footer, and then add a Page field and a NumPages field to the paragraph using Paragraph.appendField(String fieldName, FieldType fieldType) method.
- Save the document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FieldType; import com.spire.doc.HeaderFooter; import com.spire.doc.Section; import com.spire.doc.documents.HorizontalAlignment; import com.spire.doc.documents.Paragraph; public class addPageNumbersWholeDocument { public static void main(String[] args) { //Create an object of Document class Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the first section Section section = document.getSections().get(0); //Get the footer of the section HeaderFooter footer = section.getHeadersFooters().getFooter(); //Add Page and NumPages fields to the footer and set the format Paragraph footerParagraph = footer.addParagraph(); footerParagraph.appendField("page number", FieldType.Field_Page); footerParagraph.appendText(" / "); footerParagraph.appendField("page count", FieldType.Field_Num_Pages); footerParagraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Center); footerParagraph.getStyle().getCharacterFormat().setFontSize(16); //Save the document document.saveToFile("PageNumberWholeDocument.docx"); document.dispose(); } }
Restart Page Numbering for Each Section in a Word Document in Java
Restarting page numbering allows you to start the page numbers at a particular number in each section, rather than continuing from the page numbers of the previous section.
To restart page numbering for each section of a Word document, you need to loop through all sections in the document and add a Page field and a SectionPages field to each section. Then use the Section.getPageSetup().setRestartPageNumbering(true) method to enable restarting page numbering and the Section.getPageSetup().setPageStartingNumber(int value) method to set the starting page number for each section. The detailed steps are as follows:
- Create an object of Document class.
- Load a Word document using Document.loadFromFile() method.
- Loop through the sections in the document.
- Call the Paragraph.appendField(String fieldName, FieldType fieldType) method to add a Page field and a SectionPages field to each section.
- Call the Section.getPageSetup().setRestartPageNumbering(true) method to enable restarting page numbering and the Section.getPageSetup().setPageStartingNumber(int value) method to set the starting page number for each section.
- Save the document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FieldType; import com.spire.doc.HeaderFooter; import com.spire.doc.documents.HorizontalAlignment; import com.spire.doc.documents.Paragraph; public class addPageNumbersEachSection { public static void main(String[] args) { //Create an object of Document class Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the count of sections in the document int s = document.getSections().getCount(); //Loop through the sections in the document for (int i = 0; i < s; i++) { //Add Page and SectionPages fields to each section HeaderFooter footer = document.getSections().get(i).getHeadersFooters().getFooter(); Paragraph footerParagraph = footer.addParagraph(); footerParagraph.appendField("page number", FieldType.Field_Page); footerParagraph.appendText(" / "); footerParagraph.appendField("section page count", FieldType.Field_Section_Pages); footerParagraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Center); footerParagraph.getStyle().getCharacterFormat().setFontSize(16); //Restart page numbering for each section if (i == s-1) break; else { document.getSections().get(i + 1).getPageSetup().setRestartPageNumbering(true); document.getSections().get(i + 1).getPageSetup().setPageStartingNumber(1); } } //Save the document document.saveToFile("PageNumbersSections.docx"); document.dispose(); } }
Add Page Numbers to a Specific Section in a Word Document in Java
By default, when you insert page numbers into the footer of a section, the subsequent sections will automatically link to the previous section to continue displaying the page numbers. If you want to add page numbers to only a specific section, you will need to unlink the subsequent sections from the previous section, and then delete the content of the footers in the subsequent sections. The detailed steps are as follows:
- Create an object of Document class.
- Load a Word document using Document.loadFromFile() method.
- Get the second section of the document using Document.getSections().get() method.
- Call the Section.getPageSetup().setRestartPageNumbering(true) method to enable restarting page numbering and the Section.getPageSetup().setPageStartingNumber(int value) method to set the starting page number for the section.
- Call the Paragraph.appendField(String fieldName, FieldType fieldType) method to add a Page field and a SectionPages field to the section.
- Unlink the subsequent section from the second section using the Section.getHeadersFooters().getFooter().setLinkToPrevious(false) method.
- Delete the content of the footers in the subsequent sections.
- Save the document using Doucment.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FieldType; import com.spire.doc.HeaderFooter; import com.spire.doc.Section; import com.spire.doc.documents.HorizontalAlignment; import com.spire.doc.documents.Paragraph; public class addPageNumbersToSpecificSection { public static void main(String[] args) { //Create an object of Document Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the second section Section section = document.getSections().get(1); //Get the footer of the second section HeaderFooter footer = section.getHeadersFooters().getFooter(); //Set the start page as the first page of the second section and the starting number as 1 section.getPageSetup().setRestartPageNumbering(true); section.getPageSetup().setPageStartingNumber(1); //Add page numbers to the footer and set the format Paragraph footerParagraph = footer.addParagraph(); footerParagraph.appendField("Page number", FieldType.Field_Page); footerParagraph.appendText(" / "); footerParagraph.appendField("Number of pages", FieldType.Field_Section_Pages); footerParagraph.getFormat().setHorizontalAlignment(HorizontalAlignment.Center); footerParagraph.getStyle().getCharacterFormat().setFontSize(12); //Unlink the subsequent section from the second section document.getSections().get(2).getHeadersFooters().getFooter().setLinkToPrevious(false); //Delete the content of the footers in the subsequent sections for (int i = 2; i < document.getSections().getCount(); i++) { document.getSections().get(i).getHeadersFooters().getFooter().getChildObjects().clear(); document.getSections().get(i).getHeadersFooters().getFooter().addParagraph(); } //Save the document document.saveToFile("PageNumberOneSection.docx"); document.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
We can format Word document in a multi-column newsletter layout by adding columns. This article demonstrates how to add multiple columns to a Word document and specify the column width and the spacing between columns using Spire.Doc for Java.
import com.spire.doc.*; import com.spire.doc.documents.*; public class CreateMutiColumnWordDocument { public static void main(String[] args){ //create a Document object Document document = new Document(); //add a section Section section = document.addSection(); //add 3 columns to the section section.addColumn(100, 20); section.addColumn(100, 20); section.addColumn(100, 20); //add a paragraph to the section Paragraph paragraph = section.addParagraph(); //add a paragraph to the section paragraph = section.addParagraph(); String text = "Spire.Doc for Java is a professional Java Word API that enables Java applications " +"to create, convert, manipulate and print Word documents without using Microsoft Office."; //add text to the paragraph paragraph.appendText(text); //add column break to the paragraph paragraph.appendBreak(BreakType.Column_Break); //add a paragraph to the section paragraph = section.addParagraph(); //add text to the paragraph paragraph.appendText(text); //add column break to the paragraph paragraph.appendBreak(BreakType.Column_Break); //add a paragraph to the section paragraph = section.addParagraph(); //add text to the paragraph paragraph.appendText(text); //add line between columns section.getPageSetup().setColumnsLineBetween(true); //save the resultant document document.saveToFile("Muti-Column Document.docx", FileFormat.Docx_2013); } }
Output:
Page margins are the blank spaces between the body content and the page edges. In Microsoft Word, the default margins of each page are set as 1 inch, but sometimes you may need to resize the margins to accordance with your requirements. In this article, you will learn how to set page margins for Word documents in Java using Spire.Doc for Java.
Install Spire.Doc for Java
First of all, you're required to add the Spire.Doc.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc</artifactId> <version>12.9.0</version> </dependency> </dependencies>
Set Page Margins in Word in Java
The following are the steps to set page margins in a Word document:
- Initialize an instance of Document class.
- Load a Word document using Document.loadFromFile() method.
- Get the desired section through Document.getSections().get(sectionIndex) method.
- Set the top, bottom, left and right margins for the pages in the section through Section.getPageSetup().getMargins().setTop(), Section. getPageSetup().getMargins().setBottom(), Section. getPageSetup().getMargins().setLeft(), Section.getPageSetup().getMargins().setRight() methods.
- Save the result document using Document.saveToFile() method.
- Java
import com.spire.doc.Document; import com.spire.doc.FileFormat; import com.spire.doc.Section; public class SetPageMargins { public static void main(String []args){ //Create a Document instance Document document = new Document(); //Load a Word document document.loadFromFile("Sample.docx"); //Get the first section Section section = document.getSections().get(0); //Set top, bottom, left and right page margins for the section section.getPageSetup().getMargins().setTop(17.9f); section.getPageSetup().getMargins().setBottom(17.9f); section.getPageSetup().getMargins().setLeft(17.9f); section.getPageSetup().getMargins().setRight(17.9f); //Save the result document document.saveToFile("SetMargins.docx", FileFormat.Docx_2013); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
