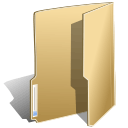
Conversion (22)
Converting images to PDF is beneficial for many reasons. For one reason, it allows you to convert images into a format that is more readable and easier to share. For another reason, it dramatically reduces the size of the file while preserving the quality of images. In this article, you will learn how to convert images to PDF in Java using Spire.PDF for Java.
There is no straightforward method provided by Spire.PDF to convert images to PDF. You could, however, create a new PDF document and draw images at the specified locations. Depending on whether the page size of the generated PDF matches the image, this topic can be divided into two subtopics.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Additionally, the imgscalr library is used in the first code example to resize images. It is not necessary to install it if you do not need to adjust the image’s size.
Add an Image to PDF at a Specified Location
The following are the steps to add an image to PDF at a specified location using Spire.PDF for Java.
- Create a PdfDocument object.
- Set the page margins using PdfDocument.getPageSettings().setMargins() method.
- Add a page using PdfDocument.getPages().add() method
- Load an image using ImageIO.read() method, and get the image width and height.
- If the image width is larger than the page (the content area) width, resize the image to make it to fit to the page width using the imgscalr library.
- Create a PdfImage object based on the scaled image or the original image.
- Draw the PdfImage object on the first page at (0, 0) using PdfPageBase.getCanvas().drawImage() method.
- Save the document to a PDF file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.graphics.PdfImage; import org.imgscalr.Scalr; import javax.imageio.ImageIO; import java.awt.image.BufferedImage; import java.io.FileInputStream; import java.io.IOException; public class AddImageToPdf { public static void main(String[] args) throws IOException { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Set the margins doc.getPageSettings().setMargins(20); //Add a page PdfPageBase page = doc.getPages().add(); //Load an image BufferedImage image = ImageIO.read(new FileInputStream("C:\\Users\\Administrator\\Desktop\\announcement.jpg")); //Get the image width and height int width = image.getWidth(); int height = image.getHeight(); //Declare a PdfImage variable PdfImage pdfImage; //If the image width is larger than page width if (width > page.getCanvas().getClientSize().getWidth()) { //Resize the image to make it to fit to the page width int widthFitRate = width / (int)page.getCanvas().getClientSize().getWidth(); int targetWidth = width / widthFitRate; int targetHeight = height / widthFitRate; BufferedImage scaledImage = Scalr.resize(image,Scalr.Method.QUALITY,targetWidth,targetHeight); //Load the scaled image to the PdfImage object pdfImage = PdfImage.fromImage(scaledImage); } else { //Load the original image to the PdfImage object pdfImage = PdfImage.fromImage(image); } //Draw image at (0, 0) page.getCanvas().drawImage(pdfImage, 0, 0, pdfImage.getWidth(), pdfImage.getHeight()); //Save to file doc.saveToFile("output/AddImage.pdf"); } }
Convert an Image to PDF with the Same Width and Height
The following are the steps to convert an image to a PDF with the same page size as the image using Spire.PDF for Java.
- Create a PdfDocument object.
- Set the page margins to zero using PdfDocument.getPageSettings().setMargins() method.
- Load an image using ImageIO.read() method, and get the image width and height.
- Add a page to PDF based on the size of the image using PdfDocument.getPages().add() method.
- Create a PdfImage object based on the image.
- Draw the PdfImage object on the first page from the coordinate (0, 0) using PdfPageBase.getCanvas().drawImage() method.
- Save the document to a PDF file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.graphics.PdfImage; import javax.imageio.ImageIO; import java.awt.*; import java.awt.image.BufferedImage; import java.io.FileInputStream; import java.io.IOException; public class ConvertImageToPdfWithSameSize { public static void main(String[] args) throws IOException { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Set the margins to 0 doc.getPageSettings().setMargins(0); //Load an image BufferedImage image = ImageIO.read(new FileInputStream("C:\\Users\\Administrator\\Desktop\\announcement.jpg")); //Get the image width and height int width = image.getWidth(); int height = image.getHeight(); //Add a page of the same size as the image PdfPageBase page = doc.getPages().add(new Dimension(width, height)); //Create a PdfImage object based on the image PdfImage pdfImage = PdfImage.fromImage(image); //Draw image at (0, 0) of the page page.getCanvas().drawImage(pdfImage, 0, 0, pdfImage.getWidth(), pdfImage.getHeight()); //Save to file doc.saveToFile("output/ConvertPdfWithSameSize.pdf"); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
In the article of convert PDF to SVG by Spire.PDF, each page on the PDF file has been saved as a single SVG file. For example, if the PDF contains 10 pages, we will get 10 SVG files separately. From version 2.7.6, Spire.PDF for Java supports to convert a multipage PDF to one single SVG file in Java.
import com.spire.pdf.*; public class PDFtoSVG { public static void main(String[] args) throws Exception { String inputPath = "Sample.pdf"; PdfDocument document = new PdfDocument(); document.loadFromFile(inputPath); document.getConvertOptions().setOutputToOneSvg(true); document.saveToFile("output.svg", FileFormat.SVG); document.close(); } }
Effective screenshot of the resulted one SVG file:
PDF (Portable Document Format) and XPS (XML Paper Specification) are two commonly used document formats for sharing and printing documents. While PDF is widely known and supported, XPS is a Microsoft-developed format that has gained popularity due to its superior graphics rendering capabilities. In this article, we will demonstrate how to use Spire.PDF for Java to convert PDF to XPS and XPS to PDF in high quality.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert PDF to XPS in Java
Spire.PDF for Java has a powerful conversion feature, which can convert PDF to XPS in just three steps. The detailed steps are as follows:
- Create a PdfDocument instance.
- Load a PDF sample document using PdfDocument.loadFromFile() method.
- Save the document as XPS using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class PDFtoXPS { public static void main(String[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load the PDF file pdf.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf.pdf"); //Save to XPS pdf.saveToFile("ToXPS.xps", FileFormat.XPS); pdf.close(); } }
Convert XPS to PDF in Java
The PdfDocument.saveToFile() method provided by Spire.PDF for Java enables the conversion of a XPS file into a PDF document. The following are steps to convert XPS to PDF.
- Create a PdfDocument instance.
- Load a XPS file document using PdfDocument.loadFromFile() method.
- Save the document as PDF using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class XPStoPDF { public static void main(String[] args) { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a XPS file pdf.loadFromXPS("C:\\Users\\Administrator\\Desktop\\sample.xps"); //Save to PDF pdf.saveToFile("toPDF.pdf", FileFormat.PDF); pdf.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
SVG, short for scalable vector graphics, is a vector image format based on XML for two-dimensional graphics. Vector image files, like SVG and PDF files, are very similar. They can display text, images, and other elements in the same appearance and keep the definition no matter how you zoom them. And because of their similarity, PDF files can be converted to SVG files almost losslessly. This article shows an easy method to convert PDF files to SVG files using Spire.PDF for Java.
- Convert Each Page of a PDF File to an SVG File
- Convert All the Pages of a PDF File to a Single SVG File
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert Each Page of a PDF File to an SVG File
The detailed steps are as follows:
- Create an object of PdfDocument class.
- Load a PDF document from disk using PdfDocument.loadFromFile() method.
- Convert the document to SVG file and save it using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class PDFToSVG { public static void main(String[] args) { //Create an object of Document class PdfDocument pdf = new PdfDocument(); //Load a PDF document from disk pdf.loadFromFile("D:/Samples/Sample.pdf"); //Convert the document to SVG and Save it pdf.saveToFile("D:/javaOutput/PDFToSVG.svg", FileFormat.SVG); } }
Convert All the Pages of a PDF File to a Single SVG File
The detailed steps are as follows:
- Create an object of PdfDocument class.
- Load a PDF document from disk using PdfDocument.loadFromFile() method.
- Change the conversion settings to convert the PDF file to a single SVG file using PdfDocument.getConvertOptions().setOutputToOneSvg() method.
- Convert the document to SVG file and save it using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; public class PDFToSVG { public static void main(String[] args) { //Create an object of Document class PdfDocument pdf = new PdfDocument(); //Load a PDF document from disk pdf.loadFromFile("D:/Samples/Sample.pdf"); //Change the conversion settings to convert the PDF file to a single SVG file pdf.getConvertOptions().setOutputToOneSvg(true); //Convert the document to SVG and Save it pdf.saveToFile("D:/javaOutput/PDFToSVG.svg", FileFormat.SVG); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF file format makes the presentation of documents consistent across devices. However, when you need to put PDF documents on web pages, it's better to convert them to HTML files. In this way, all the content of your document can be displayed in the browser directly, with no need for downloading files. And the loading of large PDF documents takes a long time, while HTML files can be rendered in the browser very quickly. In addition, compared to PDF files, it is much easier for search engines to crawl HTML web pages to get information, which will give your website more exposure. This article will show how to convert PDF documents into HTML files in Java using Spire.PDF for Java.
- Convert a PDF document to an HTML file in Java
- Convert a PDF document to an HTML file with SVG Embedded
- Convert a PDF document to HTML Stream in Java
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert a PDF document to an HTML file in Java
The conversion from a PDF document to an HTML file can be directly done by loading a PDF document and saving it as an HTML file using PdfDocument.saveToFile(String filename, FileFormat.HTML) method provided by Spire.PDF for Java. The detailed steps are as follows.
- Create an object of PdfDocument.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Save the PDF file as an HTML file using PdfDocument.saveToFle() method.
- Java
Java import com.spire.pdf.*; public class convertPDFToHTML { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument pdf = new PdfDocument(); //Load a PDF file pdf.loadFromFile("C:/Guide to a Foreign Past.pdf"); //Save the PDF file as an HTML file pdf.saveToFile("PDFToHTML.html",FileFormat.HTML); pdf.close(); } }
Convert a PDF document to an HTML file with SVG Embedded
Spire.PDF for Java also provides the PdfDocument.getConvertOptions().setPdfToHtmlOptions(true) method to enable embedding SVG while converting. The detailed steps for converting a PDF file to an HTML file with SVG embedded are as follows.
- Create an object of PdfDocument.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Enable embedding SVG using PdfDocument.getConvertOptions().setPdfToHtmlOptions(true) method.
- Save the PDF file as an HTML file using PdfDocument.saveToFle() method.
- Java
import com.spire.pdf.*; public class convertPDFToHTMLEmbeddingSVG { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument doc = new PdfDocument(); //Load a PDF file doc.loadFromFile("C:/Guide to a Foreign Past.pdf"); //Set embedding SVG doc.getConvertOptions().setPdfToHtmlOptions(true); //Save the PDF file as an HTML file doc.saveToFile("PDFToHTMLEmbeddingSVG.html", FileFormat.HTML); doc.close(); } }
Convert a PDF document to HTML Stream in Java
Spire.PDF for Java also supports converting PDF documents to HTML stream. The detailed steps are as follows.
- Create an object of PdfDocument.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Save the PDF file as HTML stream using PdfDocument.saveToStream() method.
- Java
import com.spire.pdf.*; import java.io.*; public class convertPDFToHTMLStream { public static void main(String[] args) throws FileNotFoundException { //Create an object of PdfDocument PdfDocument pdf = new PdfDocument(); //Load a PDF file pdf.loadFromFile("C:/Guide to a Foreign Past.pdf"); //Save the PDF file as HTML stream File outFile = new File("PDFToHTMLStream.html"); OutputStream outputStream = new FileOutputStream(outFile); pdf.saveToStream(outputStream, FileFormat.HTML); pdf.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Nowadays, it is not difficult to convert PDF documents into Word files using a software. However, if you want to maintain the layout and even the font formatting while converting, it is not something that every software can accomplish. Spire.PDF for Java does it well and offers you the following two modes when converting PDF to Word in Java.
Fixed Layout mode has fast conversion speed and is conducive to maintaining the original appearance of PDF files to the greatest extent. However, the editability of the resulting document will be limited since each line of text in PDF will be presented in a separate frame in the generated Word document.
Flowable Structure is a full recognition mode. The converted content will not be presented in frames, and the structure of the resulting document is flowable. The generated Word document is easy to re-edit but may look different from the original PDF file.
Install Spire.PDF for Java
First, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert PDF to Doc/Docx with Fixed Layout
The following are the steps to convert PDF to Doc or Docx with fixed layout.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Convert the PDF document to a Doc or Docx format file using PdfDocument.saveToFile(String fileName, FileFormat fileFormat) method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; public class ConvertPdfToWordWithFixedLayout { public static void main(String[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load a sample PDF document doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf"); //Convert PDF to Doc and save it to a specified path doc.saveToFile("output/ToDoc.doc", FileFormat.DOC); //Convert PDF to Docx and save it to a specified path doc.saveToFile("output/ToDocx.docx", FileFormat.DOCX); doc.close(); } }
Convert PDF to Doc/Docx with Flowable Structure
The following are the steps to convert PDF to Doc or Docx with flowable structure.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Set the conversion mode as flow using PdfDocument. getConvertOptions().setConvertToWordUsingFlow() method.
- Convert the PDF document to a Doc or Docx format file using PdfDocument.saveToFile(String fileName, FileFormat fileFormat) method.
- Java
import com.spire.pdf.FileFormat; import com.spire.pdf.PdfDocument; public class ConvertPdfToWordWithFlowableStructure { public static void main(String[] args) { //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load a sample PDF document doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\sample.pdf"); //Convert PDF to Word with flowable structure doc.getConvertOptions().setConvertToWordUsingFlow(true); //Convert PDF to Doc doc.saveToFile("output/ToDoc.doc", FileFormat.DOC); //Convert PDF to Docx doc.saveToFile("output/ToDocx.docx", FileFormat.DOCX); doc.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF/A is a kind of PDF format designed for archiving and long-term preservation of electronic documents. Unlike paper documents that are easily damaged or smeared, PDF/A format ensures that documents can be reproduced in exactly the same way even after long-term storage. This article will demonstrate how to convert PDF to PDF/A-1A, 2A, 3A, 1B, 2B and 3B compliant PDF using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.PDF.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert PDF to PDF/A
The detailed steps are as follows:
- Create a PdfStandardsConverter instance, and pass in a sample PDF file as a parameter.
- Convert the sample file to PdfA1A conformance level using PdfStandardsConverter.toPdfA1A() method.
- Convert the sample file to PdfA1B conformance level using PdfStandardsConverter. toPdfA1B() method.
- Convert the sample file to PdfA2A conformance level using PdfStandardsConverter. toPdfA2A() method.
- Convert the sample file to PdfA2B conformance level using PdfStandardsConverter. toPdfA2B() method.
- Convert the sample file to PdfA3A conformance level using PdfStandardsConverter. toPdfA3A() method.
- Convert the sample file to PdfA3B conformance level using PdfStandardsConverter. toPdfA3B() method.
- Java
import com.spire.pdf.conversion.PdfStandardsConverter; public class ConvertPdfToPdfA { public static void main(String[] args) { //Create a PdfStandardsConverter instance, and pass in a sample file as a parameter PdfStandardsConverter converter = new PdfStandardsConverter("sample.pdf"); //Convert to PdfA1A converter.toPdfA1A("output/ToPdfA1A.pdf"); //Convert to PdfA1B converter.toPdfA1B("output/ToPdfA1B.pdf"); //Convert to PdfA2A converter.toPdfA2A( "output/ToPdfA2A.pdf"); //Convert to PdfA2B converter.toPdfA2B("output/ToPdfA2B.pdf"); //Convert to PdfA3A converter.toPdfA3A("output/ToPdfA3A.pdf"); //Convert to PdfA3B converter.toPdfA3B("output/ToPdfA3B.pdf"); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Although PDF documents are widely supported across different devices and platforms, images may be more suitable for certain tasks since they can be easily added to videos or other documents, especially when you only wish to display one PDF page. This article will demonstrate how to programmatically convert PDF to images from the following two aspects using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Convert a Whole PDF Document to Multiple Images
The following are steps to convert a whole PDF document to multiple images.
- Create a PdfDocument instance.
- Load a PDF sample document using PdfDocument.loadFromFile() method.
- Loop through all pages of the document and set the image Dpi when converting them to images using PdfDocument.saveAsImage(int pageIndex, PdfImageType type, int dpiX, int dpiY) method.
- Save images to a specific folder as .png files.
- Java
import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import com.spire.pdf.PdfDocument; import com.spire.pdf.graphics.PdfImageType; import javax.imageio.ImageIO; public class WholePDFToImages { public static void main(String[] args) throws IOException { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF sample document pdf.loadFromFile("sample.pdf"); //Loop through every page for (int i = 0; i < pdf.getPages().getCount(); i++) { //Convert all pages to images and set the image Dpi BufferedImage image = pdf.saveAsImage(i, PdfImageType.Bitmap,500,500); //Save images to a specific folder as a .png files File file = new File("C:\\Users\\Administrator\\Desktop\\PDFToImages" + "/" + String.format(("ToImage-img-%d.png"), i)); ImageIO.write(image, "PNG", file); } pdf.close(); } }
Convert a Particular PDF Page to an Image
The following steps show you how to convert a particular PDF page to an image.
- Create a PdfDocument instance.
- Load a PDF sample document using PdfDocument.loadFromFile() method.
- Convert a specific page to an image and set the image Dpi using PdfDocument.saveAsImage(int pageIndex, PdfImageType type, int dpiX, int dpiY) method.
- Save the image to another file as a .png format.
- Java
import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import com.spire.pdf.PdfDocument; import com.spire.pdf.graphics.PdfImageType; import javax.imageio.ImageIO; public class ParticularPDFToImage { public static void main(String[] args) throws IOException { //Create a PdfDocument instance PdfDocument pdf = new PdfDocument(); //Load a PDF sample document pdf.loadFromFile("sample.pdf"); //Convert the first page to an image and set the image Dpi BufferedImage image= pdf.saveAsImage(0, PdfImageType.Bitmap,500,500); //Save the image to another file as a .png format ImageIO.write(image, "PNG", new File("output/ToPNG.png")); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
