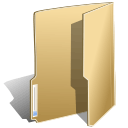
Annotation (5)
Java: Add or Remove Watermark Annotations in PDF Documents
2024-07-02 00:56:02 Written by support iceblueAdding and removing watermarks in PDF documents play a crucial role in document management, copyright protection, and information security. A watermark can serve as a visual marker, such as a company logo, copyright notice, or the word "Confidential", indicating the source, status, or ownership of the document.
Spire.PDF provides a method for adding watermarks by embedding watermark annotations within the PDF document. This approach affords the flexibility to remove the watermark post-insertion, offering users greater control and options. This article will introduce how to add or remove watermark annotations in PDF documents using Spire.PDF for Java in Java projects.
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Add Watermark Annotations to PDF in Java
Spire.PDF provides a method to add watermark annotations to PDF pages using the PdfWatermarkAnnotation object. Notably, watermarks added by this method can be easily removed later. Here are the key steps involved:
- Initialize an instance of the PdfDocument class.
- Load a PDF document using the PdfDocument.loadFromFile() method.
- Create a PdfTrueTypeFont font object to draw the watermark text.
- Create a Rectangle2D type object to define the boundary of the page.
- Use a for loop to iterate over all PdfPageBase objects in the PDF document.
- Create a PdfTemplate object for drawing the watermark, setting its size to match the current page.
- Call a custom insertWatermark() method to draw the watermark content onto the PdfTemplate object.
- Create a PdfWatermarkAnnotation object and define the position where the watermark should be placed.
- Create a PdfAppearance object to configure the visual effects of the watermark.
- Use the PdfAppearance.setNormal(PdfTemplate) method to associate the PdfTemplate object with the PdfAppearance object.
- Use the PdfWatermarkAnnotation.setAppearance(PdfAppearance) method to associate the PdfAppearance object with the PdfWatermarkAnnotation object.
- Add the watermark annotation to the page by calling PdfPageBase.getAnnotationsWidget().add(PdfWatermarkAnnotation).
- Save the changes to a file using the PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; import com.spire.pdf.annotations.*; import com.spire.pdf.annotations.appearance.*; import com.spire.pdf.graphics.*; import java.awt.*; import java.awt.geom.*; public class AddWatermarkInPDF { public static void main(String[] args) { // Create a PdfDocument object PdfDocument pdfDocument = new PdfDocument(); // Load the PDF document from a file pdfDocument.loadFromFile("Sample1.pdf"); // Set the font style Font font = new Font("Arial", Font.PLAIN, 22); // Create a PdfTrueTypeFont object for subsequent text rendering PdfTrueTypeFont trueTypeFont = new PdfTrueTypeFont(font); // Get the page object PdfPageBase page; // Define the watermark text String watermarkAnnotationText = "ID_0"; // Create a size object Dimension2D dimension2D = new Dimension(); // Create a rectangle object Rectangle2D loRect = new Rectangle2D.Float(); // Iterate through each page in the PDF for (int i = 0; i < pdfDocument.getPages().getCount(); i++) { // Get the current page page = pdfDocument.getPages().get(i); // Set the size object to the size of the current page dimension2D.setSize(page.getClientSize().getWidth(), page.getClientSize().getHeight()); // Set the rectangle object frame, which is the entire page range loRect.setFrame(new Point2D.Float(0, 0), dimension2D); // Create a PdfTemplate object to draw the watermark PdfTemplate template = new PdfTemplate(page.getClientSize().getWidth(), page.getClientSize().getHeight()); // Insert the watermark insertWatermark(template, trueTypeFont, "Non Editable"); // Create a PdfWatermarkAnnotation object to define the watermark position PdfWatermarkAnnotation watermarkAnnotation = new PdfWatermarkAnnotation(loRect); // Create a PdfAppearance object to set the watermark appearance PdfAppearance appearance = new PdfAppearance(watermarkAnnotation); // Set the normal state template of the watermark appearance.setNormal(template); // Set the appearance to the watermark object watermarkAnnotation.setAppearance(appearance); // Set the watermark text watermarkAnnotation.setText(watermarkAnnotationText); // Set the watermark print matrix to control the watermark's position and size watermarkAnnotation.getFixedPrint().setMatrix(new float[]{1, 0, 0, 1, 0, 0}); // Set the horizontal offset watermarkAnnotation.getFixedPrint().setHorizontalTranslation(0.5f); // Set the vertical offset watermarkAnnotation.getFixedPrint().setVerticalTranslation(0.5f); // Add the watermark to the page's annotation widget page.getAnnotationsWidget().add(watermarkAnnotation); } // Save the PDF document to a file pdfDocument.saveToFile("AddWatermark.pdf"); // Close and release the PDF document resources pdfDocument.dispose(); } static void insertWatermark(PdfTemplate template, PdfTrueTypeFont font, String watermark) { // Create a Dimension2D object to set the size of the watermark Dimension2D dimension2D = new Dimension(); // Set the size of the watermark to half the width and one third the height of the template dimension2D.setSize(template.getWidth() / 2, template.getHeight() / 3); // Create a PdfTilingBrush object for repeating pattern fill of the watermark PdfTilingBrush brush = new PdfTilingBrush(dimension2D); // Set the transparency of the watermark to 0.3 brush.getGraphics().setTransparency(0.3F); // Start a group of graphic state saves brush.getGraphics().save(); // Translate the graphics context so its center aligns with the center of the watermark tile brush.getGraphics().translateTransform((float) brush.getSize().getWidth() / 2, (float) brush.getSize().getHeight() / 2); // Rotate the graphics context to tilt the watermark at 45 degrees brush.getGraphics().rotateTransform(-45); // Draw the watermark text in the graphics context using the specified font, color, and centered alignment brush.getGraphics().drawString(watermark, font, PdfBrushes.getGray(), 0, 0, new PdfStringFormat(PdfTextAlignment.Center)); // End the group of graphic state saves and restore brush.getGraphics().restore(); // Reset the watermark transparency to 1, i.e., completely opaque brush.getGraphics().setTransparency(1); // Create a Rectangle2D object to define the area for filling the watermark Rectangle2D loRect = new Rectangle2D.Float(); // Set the fill area for the watermark to cover the entire size of the template loRect.setFrame(new Point2D.Float(0, 0), template.getSize()); // Draw the watermark on the template using the watermark tile template.getGraphics().drawRectangle(brush, loRect); } }
Remove Watermark Annotations from PDF in Java
Spire.PDF can remove watermark annotations added to PDF pages via the PdfWatermarkAnnotation object. Here are the detailed steps:
- Initialize an instance of the PdfDocument class.
- Load a PDF document using the PdfDocument.loadFromFile() method.
- Iterate over every PdfPageBase object in the PDF document using a for loop.
- Retrieve all annotations on the current page using the PdfPageBase.getAnnotationsWidget() method.
- Again, use a for loop to iterate over every annotation object on the current page, filtering out annotations of type PdfWatermarkAnnotationWidget.
- Determine the target watermark annotation by invoking the PdfWatermarkAnnotationWidget.getText() method and perform the deletion operation.
- Save the changes to a file using the PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.*; import com.spire.pdf.annotations.*; public class RemoveWatermarkFromPDF { public static void main(String[] args) { // Create a PdfDocument object PdfDocument pdfDocument = new PdfDocument(); // Load the PDF document from a file pdfDocument.loadFromFile("Sample2.pdf"); // Define a string ID to match and remove a specific watermark String id = "ID_0"; // Iterate through every page in the PDF document for (int i = 0; i < pdfDocument.getPages().getCount(); i++) { // Get all annotations on the current page PdfAnnotationCollection annotationWidget = pdfDocument.getPages().get(i).getAnnotationsWidget(); // Iterate through all annotations on the current page for (int j = 0; j < annotationWidget.getCount(); j++) { // Check if the current annotation is a watermark annotation if (annotationWidget.get(j) instanceof PdfWatermarkAnnotationWidget) { // If the watermark text equals the ID, remove the watermark if (annotationWidget.get(j).getText().equals(id)) { annotationWidget.remove(annotationWidget.get(j)); } } } } // Save the modified PDF document to a new file pdfDocument.saveToFile("RemoveWatermark.pdf"); // Dispose of the PdfDocument object resources pdfDocument.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF Annotations are additional objects added to a PDF document. Sometimes you may need to extract these additional data from the PDF file so as to learn about the annotation details without opening the document. In this article, we will describe how to get the annotations from PDF in Java using Spire.PDF for Java.
Install Spire.PDF for Java
First of all, you need to add the Spire.PDF.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Get Annotations from a PDF File
Spire.PDF for Java offers PdfPageBase.getAnnotationsWidget() method to get the annotation collection of the specified page of the document.
The following are the steps to get all the annotations from the first page of PDF file:
- Create an object of PdfDocument class.
- Load a sample PDF document using PdfDocument.loadFromFile() method.
- Create a StringBuilder object.
- Get the annotation collection of the first page of the document by using PdfPageBase.getAnnotationsWidget() method.
- Loop through the pop-up annotations, after extract data from each annotation using PdfAnnotation.getText()method, then append the data to the StringBuilder instance using StringBuilder.append() method.
- Write the extracted data to a txt document using Writer.write() method.
- Java
import com.spire.pdf.*; import com.spire.pdf.annotations.*; import java.io.FileWriter; public class Test { public static void main(String[] args) throws Exception { //Create an object of PdfDocument class. PdfDocument pdf = new PdfDocument(); //Load the sample PDF document pdf.loadFromFile("Annotations.pdf"); //Get the annotation collection of the first page of the document. PdfAnnotationCollection annotations = pdf.getPages().get(0).getAnnotationsWidget(); //Create a StringBuilder object StringBuilder content = new StringBuilder(); //Traverse all the annotations for (int i = 0; i < annotations.getCount(); i++) { //If it is the pop-up annotations, continue if (annotations.get(i) instanceof PdfPopupAnnotationWidget) continue; //Get the annotations’ author content.append("Annotation Author: " + annotations.get(i).getAuthor()+"\n"); //Get the annotations’ text content.append("Annotation Text: " + annotations.get(i).getText()+"\n"); //Get the annotations’ modified date String modifiedDate = annotations.get(i).getModifiedDate().toString(); content.append("Annotation ModifiedDate: " + modifiedDate+"\n"); //Get the annotations’ name content.append("Annotation Name: " + annotations.get(i).getName()+"\n"); //Get the annotations’ location content.append ("Annotation Location: " + annotations.get(i).getLocation()+"\n"); } //Write to a .txt file FileWriter fw = new FileWriter("GetAnnotations.txt"); fw.write(content.toString()); fw.flush(); fw.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
PDF annotations are notes or markers added to documents, which are great for making comments, giving explanations, giving feedback, etc. Co-creators of documents often communicate with annotations. However, when the issues associated with the annotations have been dealt with or the document has been finalized, it is necessary to remove the annotations to make the document more concise and professional. This article shows how to delete PDF annotations programmatically using Spire.PDF for Java.
- Remove the Specified Annotation
- Remove All Annotations from a Page
- Remove All Annotations from a PDF Document
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Remove the Specified Annotation
Annotations are page-level document elements. Therefore, deleting an annotation requires getting the page where the annotation is located first, and then you can use the PdfPageBase.getAnnotationsWidget().removeAt() method to delete the annotation. The detailed steps are as follows.
- Create a PdfDocument instance.
- Load a PDF document using PdfDocument.loadFromFile() method.
- Get the first page using PdfDocument.getPages().get() method.
- Remove the first annotation from this page using PdfPageBase.getAnnotationsWidget().removeAt() method.
- Save the document using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; public class RemoveAnnotation { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.loadFromFile("C:/Annotations.pdf"); //Get the first page PdfPageBase page = pdf.getPages().get(0); //Remove the first annotation page.getAnnotationsWidget().removeAt(0); //Save the document pdf.saveToFile("RemoveOneAnnotation.pdf"); } }
Remove All Annotations from a Page
Spire.PDF for Java also provides PdfPageBase.getAnnotationsWidget().clear() method to remove all annotations in the specified page. The detailed steps are as follows.
- Create a PdfDocument instance.
- Load a PDF document using PdfDocument.loadFromFile() method.
- Get the first page using PdfDocument.getPages().get() method.
- Remove all annotations from the page using PdfPageBase.getAnnotationsWidget().clear() method.
- Save the document using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; public class RemoveAllAnnotationPage { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.loadFromFile("C:/Annotations.pdf"); //Get the first page PdfPageBase page = pdf.getPages().get(0); //Remove all annotations in the page page.getAnnotationsWidget().clear(); //Save the document pdf.saveToFile("RemoveAnnotationsPage.pdf"); } }
Remove All Annotations from a PDF Document
To remove all annotations from a PDF document, we need to loop through all pages in the document and delete all annotations from each page. The detailed steps are as follows.
- Create a PdfDocument instance.
- Load a PDF document using PdfDocument.loadFromFile() method.
- Loop through all pages to delete annotations.
- Delete annotations in each page using PdfPageBase.getAnnotationsWidget().clear() method.
- Save the document using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; public class RemoveAllAnnotations { public static void main(String[] args) { //Create an object of PdfDocument PdfDocument pdf = new PdfDocument(); //Load a PDF document pdf.loadFromFile("C:/Users/Sirion/Desktop/Annotations.pdf"); //Loop through the pages in the document for (PdfPageBase page : (Iterable) pdf.getPages()) { //Remove annotations in each page page.getAnnotationsWidget().clear(); } //Save the document pdf.saveToFile("RemoveAllAnnotations.pdf"); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
Adding annotations to PDFs enhances document collaboration and comprehension. Whether highlighting key points, adding comments, or drawing shapes, annotations help convey thoughts and ideas clearly. By utilizing annotations, users can streamline feedback processes and ensure that important information stands out.
In this article, you will learn how to add a variety of annotations to a PDF document using Spire.PDF for Java.
- Annotation Types Supported by Spire.PDF
- Add a Text Markup Annotation to PDF
- Add a Free Text Annotation to PDF
- Add a Popup Annotation to PDF
- Add a Shape Annotation to PDF
- Add a Web Link Annotation to PDF
- Add a File Link Annotation to PDF
- Add a Document Link Annotation to PDF
Install Spire.PDF for Java
First of all, you're required to add the Spire.Pdf.jar file as a dependency in your Java program. The JAR file can be downloaded from this link. If you use Maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Annotations Types Supported by Spire.PDF
PDF documents provide extensive support for various annotation types, which empower users to enhance their documents with comments, markups, interactive features, and additional supplementary content. The table below outlines some of the most commonly used annotation types supported by Spire.PDF.
Annotation type | Definition | Represented by |
Text markup annotation | An annotation that is used to highlight or markup a specific chunk of text of a document. | PdfTextMarkupAnnotation class |
Free text annotation | An annotation that allows the user to add freeform text directly onto the PDF page. | PdfFreeTextAnnotation class |
Popup annotation | An annotation that appears as a pop-up window when clicked on, providing additional information or context related to the content of the document. | PdfPopupAnnotation class |
Stamp annotation | An annotation that adds a pre-defined stamp or image onto the document, often used for indicating approval, review status, or other relevant information. | PdfRubberStampAnnotation class |
Shape annotation | An annotation that allows users to draw shapes or lines on the document, which can be used for highlighting specific areas or adding visual cues. | PdfLineAnnotation class PdfPolygonAnnotation class |
Web link annotation | An annotation that creates a clickable link to a webpage, allowing users to easily access additional resources or related information online. | PdfUriAnnotation class |
File link annotation | An annotation that creates a clickable link to a file, allowing users to open external documents or files related to the content of the PDF. | PdfFileLinkAnnotation class |
Document link annotation | An annotation that creates a clickable link to another section or page within the same document. | PdfDocumentLinkAnnotation class |
Add a Text Markup Annotation to PDF in Java
A text markup annotation is designed to highlight specific portions of text in a PDF document. To locate the text for markup, you can utilize the PdfTextFinder class, which offers methods that simplify the process of finding the desired text on a page.
After identifying the text, you can create a PdfTextMarkupAnnotation object based on the text's bounding information. You can then set various attributes for the annotation, such as the annotation text and the markup color.
The steps to add a text markup annotation to PDF in Java are as follows:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create an object of the PdfTextMarkupAnnotation class based on the text’s bounds.
- Set the annotation text and markup color using the methods under the PdfTextMarkupAnnotation object.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfTextMarkupAnnotation; import com.spire.pdf.graphics.PdfRGBColor; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.*; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddMarkupAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("These safeguards vary based on the sensitivity of \n" + "the information that we collect and store."); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Specify annotation text String text = "This is a markup annotation."; // Iterate through the text bounds for (int i = 0; i < textFragment.getBounds().length; i++) { // Get a specific bound Rectangle2D rect = textFragment.getBounds()[i]; // Create a text markup annotation PdfTextMarkupAnnotation annotation = new PdfTextMarkupAnnotation(rect); // Set the annotation text annotation.setText(text); // Set the markup color annotation.setTextMarkupColor(new PdfRGBColor(Color.green)); // Add the annotation to the collection of the annotations page.getAnnotations().add(annotation); } // Save result to file doc.saveToFile("output/MarkupAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a Free Text Annotation to PDF in Java
To add a free text annotation in the appropriate location, such as at the end of a sentence, you can continue to use the PdfTextFinder class. This class assists in locating the desired text within a document, along with its coordinates.
Once you have the coordinate information, you can create an instance of PdfFreeTextAnnotation. After that, you can set the annotation text and customize its appearance.
The steps to add a free text annotation to PDF in Java are as follows:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfFreeTextAnnotation object, specifying its location and size.
- Set the annotation text and customize its appearance using the methods under the PdfFreeTextAnnotation object.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfAnnotationBorder; import com.spire.pdf.annotations.PdfFreeTextAnnotation; import com.spire.pdf.annotations.PdfTextMarkupAnnotation; import com.spire.pdf.graphics.PdfFont; import com.spire.pdf.graphics.PdfFontFamily; import com.spire.pdf.graphics.PdfFontStyle; import com.spire.pdf.graphics.PdfRGBColor; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.*; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddFreeTextAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Specify the x and y coordinates to add annotation double x = rect.getMaxX() + 3; double y = rect.getMaxY(); // Create a free text annotation Rectangle2D rectangle = new Rectangle2D.Double(x, y, 170, 40); PdfFreeTextAnnotation textAnnotation = new PdfFreeTextAnnotation(rectangle); // Set the content of the annotation textAnnotation.setText("Here is a free text annotation\radded by Spire.PDF for Java."); // Set other properties of annotation PdfFont font = new PdfFont(PdfFontFamily.Times_Roman, 12f, PdfFontStyle.Bold); PdfAnnotationBorder border = new PdfAnnotationBorder(1f); textAnnotation.setFont(font); textAnnotation.setBorder(border); textAnnotation.setBorderColor(new PdfRGBColor(Color.blue)); textAnnotation.setColor(new PdfRGBColor(Color.green)); textAnnotation.setOpacity(1.0f); // Add the annotation to the collection of the annotations page.getAnnotations().add(textAnnotation); // Save result to file doc.saveToFile("output/FreeTextAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a Popup Annotation to PDF in Java
Still, you can begin by using the PdfTextFinder class to identify the optimal position for adding annotations. Next, create a PdfPopupAnnotation object and customize its attributes, such as the text, color, and icon.
The steps to add a popup annotation to PDF in Java are as follows:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfPopupAnnotation object, specifying its location and size.
- Set the annotation text, color and icon using the methods under the PdfPopupAnnotation object.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfPopupAnnotation; import com.spire.pdf.annotations.PdfPopupIcon; import com.spire.pdf.graphics.PdfRGBColor; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.*; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddPopupAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Specify the x and y coordinates to add annotation double x = rect.getMaxX(); double y = rect.getMaxY(); // Create a free text annotation Rectangle2D rectangle = new Rectangle2D.Double(x, y, 30, 30); PdfPopupAnnotation popupAnnotation = new PdfPopupAnnotation(rectangle); // Set the content of the annotation popupAnnotation.setText("Here is a popup annotation\radded by Spire.PDF for Java."); // Set the icon and color of the annotation popupAnnotation.setIcon(PdfPopupIcon.Comment); popupAnnotation.setColor(new PdfRGBColor(Color.red)); // Add the annotation to the collection of the annotations page.getAnnotations().add(popupAnnotation); // Save result to file doc.saveToFile("output/PopupAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a Shape Annotation to PDF in Java
A shape annotation typically refers to a graphical element that represents a specific geometric shape, such as a rectangle, ellipse, polygon, or line. When adding a shape annotation to a PDF, multiple coordinate points are usually required to define the shape's location and size.
You can use the PdfTextFinder class to obtain the coordinates of the specified text. Based on these coordinates, you can calculate the necessary points for the shape annotation. After adding the shape annotation, you can then set the text for it.
The following are the steps to add a polygon annotation to PDF using Java:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfPolygonAnnotation object, specifying its location.
- Set the annotation text using the PdfPolygonAnnotation.setText() method.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.*; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.geom.Point2D; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddShapeAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Get the coordinates to add annotation double left = rect.getMinX(); double top = rect.getMinY(); double right = rect.getMaxX(); double bottom = rect.getMaxY(); // Create a shape annotation PdfPolygonAnnotation polygonAnnotation = new PdfPolygonAnnotation(page, new Point2D[]{new Point2D.Double(left, top), new Point2D.Double(right, top), new Point2D.Double(right + 5, bottom), new Point2D.Double(left - 5, bottom), new Point2D.Double(left, top)}); // Set the annotation border PdfAnnotationBorder border = new PdfAnnotationBorder(1f); polygonAnnotation.setBorder(border); polygonAnnotation.setBorderEffect(PdfBorderEffect.None); // Set the annotation text polygonAnnotation.setText("Here is a shape annotation\radded by Spire.PDF for Java."); // Add the annotation to the collection of the annotations page.getAnnotations().add(polygonAnnotation); // Save result to file doc.saveToFile("output/ShapeAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a Web Link Annotation to PDF in Java
A web link annotation is typically added to specific text within a PDF. You can use the PdfTextFinder class to locate the desired text and retrieve its bounding information on the page. Afterward, you can create a PdfUriAnnotation object in that area and specify an external web link.
The following are the steps to add a web link annotation to PDF using Java:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfWebLinkAnnotation object based on the text's bound, specifying a web link for the annotation.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfUriAnnotation; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddWebLinkAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Create an Url annotation PdfUriAnnotation urlAnnotation = new PdfUriAnnotation(rect, "https:\\\\www.e-iceblue.com\\"); // Add the annotation to the collection of the annotations page.getAnnotations().add(urlAnnotation); // Save result to file doc.saveToFile("output/WebLinkAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a File Link Annotation to PDF in Java
A file link annotation is also typically created based on a specific piece of text. You can use the PdfTextFinder class to locate the specified text and obtain its bounding information on the page. Then, you can create a PdfFileLinkAnnotation object using that information and specify a link that redirects to an external file.
The steps to add a file link annotation to PDF using Java are as follows:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfFileLinkAnnotation object based on the text's bound, specifying a file link for the annotation.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfFileLinkAnnotation; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddFileLinkAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Create a file link annotation PdfFileLinkAnnotation fileLinkAnnotation = new PdfFileLinkAnnotation(rect, "C:\\Users\\Administrator\\Desktop\\Report.docx"); // Add the annotation to the collection of the annotations page.getAnnotations().add(fileLinkAnnotation); // Save result to file doc.saveToFile("output/FileLinkAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Add a Document Link Annotation to PDF in Java
To locate a piece of text on a page for adding a document link annotation, you can utilize the PdfTextFinder class. After finding the text, create a PdfDocumentLinkAnnotation object based on its bounding area. Finally, you'll need to set a destination for the annotation, typically pointing to a different page.
The steps to add a document link annotation to PDF using Java are as follows:
- Create a PdfDocument object.
- Load a PDF file from a given file path using the PdfDocument.loadFromFile() method.
- Get a page from the document using the PdfDocument.getPages().get() method.
- Create a PdfTextFinder object to find a specific piece of text within the page.
- Get the bounding information of the desired text.
- Create a PdfDocumentLinkAnnotation object based on the text's bound.
- Set the destination for the annotation using the PdfDocumentLinkAnnotation.setDestination() method.
- Add the annotation to the page using the PdfPageBase.getAnnotations().add() method.
- Save the modified document to a different PDF file.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfDocumentLinkAnnotation; import com.spire.pdf.general.PdfDestination; import com.spire.pdf.texts.PdfTextFinder; import com.spire.pdf.texts.PdfTextFragment; import com.spire.pdf.texts.TextFindParameter; import java.awt.geom.Rectangle2D; import java.util.EnumSet; import java.util.List; public class AddDocumentLinkAnnotation { public static void main(String[] args) { // Create a PdfDocument object PdfDocument doc = new PdfDocument(); // Load a PDF file doc.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get a specific page PdfPageBase page = doc.getPages().get(0); // Create a PdfTextFinder object based on the page PdfTextFinder finder = new PdfTextFinder(page); // Set the find options EnumSet<TextFindParameter> parameterSet = EnumSet.of(TextFindParameter.WholeWord, TextFindParameter.IgnoreCase); finder.getOptions().setTextFindParameter(parameterSet); // Find the instances of the specified text List<PdfTextFragment> fragments = finder.find("collect and store"); // Get the first instance PdfTextFragment textFragment = fragments.get(0); // Get the text bound Rectangle2D rect = textFragment.getBounds()[0]; // Create a document link annotation PdfDocumentLinkAnnotation documentLinkAnnotation = new PdfDocumentLinkAnnotation(rect); // Set the destination of the annotation documentLinkAnnotation.setDestination(new PdfDestination(doc.getPages().get(1))); // Add the annotation to the collection of the annotations page.getAnnotations().add(documentLinkAnnotation); // Save result to file doc.saveToFile("output/DocumentLinkAnnotation.pdf"); // Dispose resources doc.dispose(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
When it comes to PDF documents, stamps offer an indispensable feature for adding contextual information and visual elements. These stamps can range from simple notes to complex graphics, enabling users to annotate, emphasize, or personalize their PDF content. With the ability to place stamps strategically, businesses can streamline workflows, indicate approvals, or provide concise feedback.
In this article, you will learn how to programmatically add dynamic stamps and image stamps to a PDF document using Spire.PDF for Java.
Install Spire.PDF for Java
To start, make sure to add the Spire.Pdf.jar file as a dependency in your Java program. You can download Spire.PDF for Java from our website and manually import the JAR file into your application. If you are using Maven, simply add the following code to your project's pom.xml file to include the JAR file effortlessly.
<repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>https://repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>10.10.7</version> </dependency> </dependencies>
Prior Knowledge
In PDF, a stamp refers to an annotation or graphical element that can be added to a document to provide additional information. Spire.PDF for Java introduces the PdfRubberStampAnnotation class, which serves as a representation of a rubber stamp. To create the visual representation of a rubber stamp, the PdfTemplate class is employed. This class acts as a canvas allowing you to draw various types of information, including text, images, shapes, and date/time elements.
Add a Dynamic Stamp to PDF in Java
A dynamic stamp refers to a customizable annotation that can be added to a PDF document to indicate a specific status, approval, or other information. Unlike static stamps, dynamic stamps contain variables or fields that can be dynamically updated, such as the current date, time, username, or any other custom data.
The following are the steps to add a dynamic stamp to PDF using Spire.PDF for Java.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Create a PdfTemplate object with desired size.
- Draw strings (including dynamic information such as date and time) on the template using PdfTemplate.getGraphics().drawString() method.
- Create a PdfRubberStampAnnotation object, and set the template as its appearance.
- Add the stamp to a specific PDF page using PdfPageBase.getAnnotationsWidget().add() method.
- Save the document to a different PDF file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfRubberStampAnnotation; import com.spire.pdf.annotations.appearance.PdfAppearance; import com.spire.pdf.graphics.*; import java.awt.*; import java.awt.geom.Point2D; import java.awt.geom.Rectangle2D; import java.text.SimpleDateFormat; public class AddDynamicStampToPdf { public static void main(String[] args) { // Create a PdfDocument object PdfDocument document = new PdfDocument(); // Load a PDF file document.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get the last page PdfPageBase page = document.getPages().get(document.getPages().getCount() - 1); // Create a PdfTemplate object PdfTemplate template = new PdfTemplate(195, 50); // Create two fonts PdfTrueTypeFont font1 = new PdfTrueTypeFont(new Font("Elephant", Font.ITALIC, 15), true); PdfTrueTypeFont font2 = new PdfTrueTypeFont(new Font("Arial", Font.ITALIC, 10), true); // Create a solid brush and a gradient brush PdfSolidBrush solidBrush = new PdfSolidBrush(new PdfRGBColor(Color.black)); Rectangle2D rect1 = new Rectangle2D.Float(); rect1.setFrame(new Point2D.Float(0, 0), template.getSize()); PdfLinearGradientBrush linearGradientBrush = new PdfLinearGradientBrush(rect1, new PdfRGBColor(Color.white), new PdfRGBColor(Color.orange), PdfLinearGradientMode.Horizontal); // Create rounded rectangle path int CornerRadius = 10; PdfPath path = new PdfPath(); path.addArc(template.getBounds().getX(), template.getBounds().getY(), CornerRadius, CornerRadius, 180, 90); path.addArc(template.getBounds().getX() + template.getWidth() - CornerRadius, template.getBounds().getY(), CornerRadius, CornerRadius, 270, 90); path.addArc(template.getBounds().getX() + template.getWidth() - CornerRadius, template.getBounds().getY() + template.getHeight() - CornerRadius, CornerRadius, CornerRadius, 0, 90); path.addArc(template.getBounds().getX(), template.getBounds().getY() + template.getHeight() - CornerRadius, CornerRadius, CornerRadius, 90, 90); path.addLine(template.getBounds().getX(), template.getBounds().getY() + template.getHeight() - CornerRadius, template.getBounds().getX(), template.getBounds().getY() + CornerRadius / 2); // Draw path on the template template.getGraphics().drawPath(linearGradientBrush, path); template.getGraphics().drawPath(PdfPens.getRed(), path); // Draw dynamic text on the template String s1 = "APPROVED\n"; String s2 = "By Manager at " + dateToString(new java.util.Date(), "yyyy-MM-dd HH:mm:ss"); template.getGraphics().drawString(s1, font1, solidBrush, new Point2D.Float(5, 5)); template.getGraphics().drawString(s2, font2, solidBrush, new Point2D.Float(2, 28)); // Create a rubber stamp, specifying its size and location Rectangle2D rect2 = new Rectangle2D.Float(); rect2.setFrame(new Point2D.Float(50, (float) (page.getActualSize().getHeight() - 300)), template.getSize()); PdfRubberStampAnnotation stamp = new PdfRubberStampAnnotation(rect2); // Create a PdfAppearance object and apply the template as its normal state PdfAppearance appearance = new PdfAppearance(stamp); appearance.setNormal(template); // Apply the appearance to stamp stamp.setAppearance(appearance); // Add the stamp annotation to annotation collection page.getAnnotationsWidget().add(stamp); // Save the file document.saveToFile("output/DynamicStamp.pdf"); document.close(); } // Convert date to string public static String dateToString(java.util.Date date, String dateFormat) { SimpleDateFormat format = new SimpleDateFormat(dateFormat); return format.format(date); } }
Add an Image Stamp to PDF in Java
An image stamp in PDF refers to a graphical element that is added to a document as an annotation or overlay. It involves placing an image onto a specific location within a PDF page.
The steps to add an image stamp to PDF using Spire.PDF for Java are as follows.
- Create a PdfDocument object.
- Load a PDF file using PdfDocument.loadFromFile() method.
- Load an image that you want to stamp on PDF using PdfImage.fromFile() method.
- Create a PdfTemplate object based on the size of the image.
- Draw the image on the template using PdfTemplate.getGraphics().drawImage() method.
- Create a PdfRubberStampAnnotation object, and set the template as its appearance.
- Add the stamp to a specific PDF page using PdfPageBase.getAnnotationsWidget().add() method.
- Save the document to a different PDF file using PdfDocument.saveToFile() method.
- Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import com.spire.pdf.annotations.PdfRubberStampAnnotation; import com.spire.pdf.annotations.appearance.PdfAppearance; import com.spire.pdf.graphics.PdfImage; import com.spire.pdf.graphics.PdfTemplate; import java.awt.geom.Rectangle2D; public class AddImageStampToPdf { public static void main(String[] args) { // Create a PdfDocument object PdfDocument document = new PdfDocument(); // Load a PDF document document.loadFromFile("C:\\Users\\Administrator\\Desktop\\Input.pdf"); // Get the last page PdfPageBase page = document.getPages().get(document.getPages().getCount() - 1); // Load an image file PdfImage image = PdfImage.fromFile("C:\\Users\\Administrator\\Desktop\\stamp-image.png"); // Get the width and height of the image int width = image.getWidth(); int height = image.getHeight(); // Create a PdfTemplate object based on the size of the image PdfTemplate template = new PdfTemplate(width, height); // Draw image on the template template.getGraphics().drawImage(image, 0, 0, width, height); // Create a rubber stamp annotation, specifying its location and position Rectangle2D rect = new Rectangle2D.Float((float) (page.getActualSize().getWidth() - width - 50), (float) (page.getActualSize().getHeight() - 400), width, height); PdfRubberStampAnnotation stamp = new PdfRubberStampAnnotation(rect); // Create a PdfAppearance object PdfAppearance pdfAppearance = new PdfAppearance(stamp); // Set the template as the normal state of the appearance pdfAppearance.setNormal(template); // Apply the appearance to the stamp stamp.setAppearance(pdfAppearance); // Add the stamp annotation to PDF page.getAnnotationsWidget().add(stamp); // Save the file document.saveToFile("output/ImageStamp.pdf"); document.close(); } }
Apply for a Temporary License
If you'd like to remove the evaluation message from the generated documents, or to get rid of the function limitations, please request a 30-day trial license for yourself.
