Monday, 05 March 2012 07:29
Export PDF Document to images
The sample demonstrates how to export PDF pages as images by PdfDocumentViewer Component.
using System; using System.Drawing; using System.IO; using System.Windows.Forms; using Spire.PdfViewer.Forms; namespace Spire.PdfView.Demos.Export { class Program { private static PdfDocumentViewer viewer = null; [STAThread] static void Main(string[] args) { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); viewer = new PdfDocumentViewer(); viewer.LoadFromFile("PDFViewer.pdf"); //form and child components Form mainForm = new Form(); mainForm.Text = "Spire.PdfView Demo - Export"; mainForm.Size = new System.Drawing.Size(800, 600); mainForm.StartPosition = FormStartPosition.CenterScreen; TableLayoutPanel table = new TableLayoutPanel(); table.ColumnCount = 3; table.ColumnStyles.Add(new ColumnStyle(SizeType.Percent, 50)); table.ColumnStyles.Add(new ColumnStyle(SizeType.Absolute, 20)); table.ColumnStyles.Add(new ColumnStyle(SizeType.Percent, 50)); table.RowCount = 2; table.RowStyles.Add(new RowStyle(SizeType.Percent, 100)); table.RowStyles.Add(new RowStyle(SizeType.Absolute, 30)); table.Controls.Add(viewer, 0, 0); table.SetColumnSpan(viewer, 3); viewer.Dock = DockStyle.Fill; //Export current page to one image Button button = new Button(); button.Text = "Export to one image"; button.Size = new Size(180, 24); button.TextAlign = ContentAlignment.MiddleCenter; table.Controls.Add(button, 0, 1); button.Dock = DockStyle.Right; button.Click += ExportToOneImage; //Export current pdf document to multiple images button = new Button(); button.Text = "Export to multiple images"; button.Size = new Size(180, 24); button.TextAlign = ContentAlignment.MiddleCenter; table.Controls.Add(button, 2, 1); button.Dock = DockStyle.Left; button.Click += ExportToMultipleImages; mainForm.Controls.Add(table); table.Dock = DockStyle.Fill; Application.Run(mainForm); } private static void ExportToOneImage(object sender, EventArgs e) { if (viewer.PageCount > 0) { SaveFileDialog dialog = new SaveFileDialog(); dialog.Filter = "PNG Format(*.png)|*.png"; if (dialog.ShowDialog() == DialogResult.OK) { int currentPage = viewer.CurrentPageNumber; Bitmap image = viewer.SaveAsImage(currentPage - 1); image.Save(dialog.FileName); } } } private static void ExportToMultipleImages(object sender, EventArgs e) { if (viewer.PageCount > 0) { FolderBrowserDialog dialog = new FolderBrowserDialog(); if (dialog.ShowDialog() == DialogResult.OK) { int currentPage = viewer.CurrentPageNumber; Bitmap[] images = viewer.SaveAsImage(0, currentPage - 1); for (int i = 0; i < images.Length; i++) { String fileName = Path.Combine(dialog.SelectedPath, String.Format("PDFViewer-{0}.png", i)); images[i].Save(fileName); } } } } } }
Imports System.Drawing Imports System.IO Imports System.Windows.Forms Imports Spire.PdfViewer.Forms Namespace Spire.PdfView.Demos.Export Friend NotInheritable Class Program Private Shared viewer As PdfDocumentViewer = Nothing _ Shared Sub Main() Application.EnableVisualStyles() Application.SetCompatibleTextRenderingDefault(False) viewer = New PdfDocumentViewer() viewer.LoadFromFile("PDFViewer.pdf") 'form and child components Dim mainForm As New Form() mainForm.Text = "Spire.PdfView Demo - Export" mainForm.Size = New System.Drawing.Size(800, 600) mainForm.StartPosition = FormStartPosition.CenterScreen Dim table As New TableLayoutPanel() table.ColumnCount = 3 table.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 50)) table.ColumnStyles.Add(New ColumnStyle(SizeType.Absolute, 20)) table.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 50)) table.RowCount = 2 table.RowStyles.Add(New RowStyle(SizeType.Percent, 100)) table.RowStyles.Add(New RowStyle(SizeType.Absolute, 30)) table.Controls.Add(viewer, 0, 0) table.SetColumnSpan(viewer, 3) viewer.Dock = DockStyle.Fill 'Export current page to one image Dim button As New Button() button.Text = "Export to one image" button.Size = New Size(180, 24) button.TextAlign = ContentAlignment.MiddleCenter table.Controls.Add(button, 0, 1) button.Dock = DockStyle.Right AddHandler button.Click, AddressOf ExportToOneImage 'Export current pdf document to multiple images button = New Button() button.Text = "Export to multiple images" button.Size = New Size(180, 24) button.TextAlign = ContentAlignment.MiddleCenter table.Controls.Add(button, 2, 1) button.Dock = DockStyle.Left AddHandler button.Click, AddressOf ExportToMultipleImages mainForm.Controls.Add(table) table.Dock = DockStyle.Fill Application.Run(mainForm) End Sub Private Shared Sub ExportToOneImage(ByVal sender As Object, ByVal e As EventArgs) If viewer.PageCount > 0 Then Dim dialog As New SaveFileDialog() dialog.Filter = "PNG Format(*.png)|*.png" If dialog.ShowDialog() = DialogResult.OK Then Dim currentPage As Integer = viewer.CurrentPageNumber Dim image As Bitmap = viewer.SaveAsImage(currentPage - 1) image.Save(dialog.FileName) End If End If End Sub Private Shared Sub ExportToMultipleImages(ByVal sender As Object, ByVal e As EventArgs) If viewer.PageCount > 0 Then Dim dialog As New FolderBrowserDialog() If dialog.ShowDialog() = DialogResult.OK Then Dim currentPage As Integer = viewer.CurrentPageNumber Dim images As Bitmap() = viewer.SaveAsImage(0, currentPage - 1) For i As Integer = 0 To images.Length - 1 Dim fileName As [String] = Path.Combine(dialog.SelectedPath, [String].Format("PDFViewer-{0}.png", i)) images(i).Save(fileName) Next End If End If End Sub End Class End Namespace
Published in
PDF Document Viewer
Wednesday, 06 April 2011 02:05
PDF DataSource to Table in C#, VB.NET
The sample demonstrates how to export data from database into a table in PDF document and set table format.
using System; using System.Data; using System.Data.OleDb; using System.Drawing; using Spire.Pdf; using Spire.Pdf.Graphics; using Spire.Pdf.Tables; namespace DataSource { class Program { static void Main(string[] args) { //Create a pdf document. PdfDocument doc = new PdfDocument(); //margin PdfUnitConvertor unitCvtr = new PdfUnitConvertor(); PdfMargins margin = new PdfMargins(); margin.Top = unitCvtr.ConvertUnits(2.54f, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point); margin.Bottom = margin.Top; margin.Left = unitCvtr.ConvertUnits(3.17f, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point); margin.Right = margin.Left; // Create one page PdfPageBase page = doc.Pages.Add(PdfPageSize.A4, margin); float y = 10; //title PdfBrush brush1 = PdfBrushes.Black; PdfTrueTypeFont font1 = new PdfTrueTypeFont(new Font("Arial", 16f, FontStyle.Bold)); PdfStringFormat format1 = new PdfStringFormat(PdfTextAlignment.Center); page.Canvas.DrawString("Country List", font1, brush1, page.Canvas.ClientSize.Width / 2, y, format1); y = y + font1.MeasureString("Country List", format1).Height; y = y + 5; //create data table PdfTable table = new PdfTable(); table.Style.CellPadding = 2; table.Style.BorderPen = new PdfPen(brush1, 0.75f); table.Style.DefaultStyle.BackgroundBrush = PdfBrushes.SkyBlue; table.Style.DefaultStyle.Font = new PdfTrueTypeFont(new Font("Arial", 10f)); table.Style.AlternateStyle = new PdfCellStyle(); table.Style.AlternateStyle.BackgroundBrush = PdfBrushes.LightYellow; table.Style.AlternateStyle.Font = new PdfTrueTypeFont(new Font("Arial", 10f)); table.Style.HeaderSource = PdfHeaderSource.ColumnCaptions; table.Style.HeaderStyle.BackgroundBrush = PdfBrushes.CadetBlue; table.Style.HeaderStyle.Font = new PdfTrueTypeFont(new Font("Arial", 11f, FontStyle.Bold)); table.Style.HeaderStyle.StringFormat = new PdfStringFormat(PdfTextAlignment.Center); table.Style.ShowHeader = true; using (OleDbConnection conn = new OleDbConnection()) { conn.ConnectionString = @"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=demo.mdb"; OleDbCommand command = new OleDbCommand(); command.CommandText = " select Name,Capital,Continent,Area,Population from country "; command.Connection = conn; using (OleDbDataAdapter dataAdapter = new OleDbDataAdapter(command)) { DataTable dataTable = new DataTable(); dataAdapter.Fill(dataTable); table.DataSourceType = PdfTableDataSourceType.TableDirect; table.DataSource = dataTable; } } float width = page.Canvas.ClientSize.Width - (table.Columns.Count + 1) * table.Style.BorderPen.Width; table.Columns[0].Width = width * 0.24f * width; table.Columns[0].StringFormat = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle); table.Columns[1].Width = width * 0.21f * width; table.Columns[1].StringFormat = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle); table.Columns[2].Width = width * 0.24f * width; table.Columns[2].StringFormat = new PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle); table.Columns[3].Width = width * 0.13f * width; table.Columns[3].StringFormat = new PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle); table.Columns[4].Width = width * 0.18f * width; table.Columns[4].StringFormat = new PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle); PdfLayoutResult result = table.Draw(page, new PointF(0, y)); y = y + result.Bounds.Height + 5; PdfBrush brush2 = PdfBrushes.Gray; PdfTrueTypeFont font2 = new PdfTrueTypeFont(new Font("Arial", 9f)); page.Canvas.DrawString(String.Format("* {0} countries in the list.", table.Rows.Count), font2, brush2, 5, y); //Save pdf file. doc.SaveToFile("DataSource.pdf"); doc.Close(); //Launching the Pdf file. System.Diagnostics.Process.Start("DataSource.pdf"); } } }
Imports System.Data Imports System.Data.OleDb Imports System.Drawing Imports Spire.Pdf Imports Spire.Pdf.Graphics Imports Spire.Pdf.Tables Namespace DataSource Friend Class Program Shared Sub Main(ByVal args() As String) 'Create a pdf document. Dim doc As New PdfDocument() 'margin Dim unitCvtr As New PdfUnitConvertor() Dim margin As New PdfMargins() margin.Top = unitCvtr.ConvertUnits(2.54F, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point) margin.Bottom = margin.Top margin.Left = unitCvtr.ConvertUnits(3.17F, PdfGraphicsUnit.Centimeter, PdfGraphicsUnit.Point) margin.Right = margin.Left ' Create one page Dim page As PdfPageBase = doc.Pages.Add(PdfPageSize.A4, margin) Dim y As Single = 10 'title Dim brush1 As PdfBrush = PdfBrushes.Black Dim font1 As New PdfTrueTypeFont(New Font("Arial", 16.0F, FontStyle.Bold)) Dim format1 As New PdfStringFormat(PdfTextAlignment.Center) page.Canvas.DrawString("Country List", font1, brush1, page.Canvas.ClientSize.Width \ 2, y, format1) y = y + font1.MeasureString("Country List", format1).Height y = y + 5 'create data table Dim table As New PdfTable() table.Style.CellPadding = 2 table.Style.BorderPen = New PdfPen(brush1, 0.75F) table.Style.DefaultStyle.BackgroundBrush = PdfBrushes.SkyBlue table.Style.DefaultStyle.Font = New PdfTrueTypeFont(New Font("Arial", 10.0F)) table.Style.AlternateStyle = New PdfCellStyle() table.Style.AlternateStyle.BackgroundBrush = PdfBrushes.LightYellow table.Style.AlternateStyle.Font = New PdfTrueTypeFont(New Font("Arial", 10.0F)) table.Style.HeaderSource = PdfHeaderSource.ColumnCaptions table.Style.HeaderStyle.BackgroundBrush = PdfBrushes.CadetBlue table.Style.HeaderStyle.Font = New PdfTrueTypeFont(New Font("Arial", 11.0F, FontStyle.Bold)) table.Style.HeaderStyle.StringFormat = New PdfStringFormat(PdfTextAlignment.Center) table.Style.ShowHeader = True Using conn As New OleDbConnection() conn.ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=demo.mdb" Dim command As New OleDbCommand() command.CommandText = " select Name,Capital,Continent,Area,Population from country " command.Connection = conn Using dataAdapter As New OleDbDataAdapter(command) Dim dataTable As New DataTable() dataAdapter.Fill(dataTable) table.DataSourceType = PdfTableDataSourceType.TableDirect table.DataSource = dataTable End Using End Using Dim width As Single = page.Canvas.ClientSize.Width - (table.Columns.Count + 1) * table.Style.BorderPen.Width table.Columns(0).Width = width * 0.24F * width table.Columns(0).StringFormat = New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle) table.Columns(1).Width = width * 0.21F * width table.Columns(1).StringFormat = New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle) table.Columns(2).Width = width * 0.24F * width table.Columns(2).StringFormat = New PdfStringFormat(PdfTextAlignment.Left, PdfVerticalAlignment.Middle) table.Columns(3).Width = width * 0.13F * width table.Columns(3).StringFormat = New PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle) table.Columns(4).Width = width * 0.18F * width table.Columns(4).StringFormat = New PdfStringFormat(PdfTextAlignment.Right, PdfVerticalAlignment.Middle) Dim result As PdfLayoutResult = table.Draw(page, New PointF(0, y)) y = y + result.Bounds.Height + 5 Dim brush2 As PdfBrush = PdfBrushes.Gray Dim font2 As New PdfTrueTypeFont(New Font("Arial", 9.0F)) page.Canvas.DrawString(String.Format("* {0} countries in the list.", table.Rows.Count), font2, brush2, 5, y) 'Save pdf file. doc.SaveToFile("DataSource.pdf") doc.Close() 'Launching the Pdf file. Process.Start("DataSource.pdf") End Sub End Class End Namespace
Published in
Table
Saturday, 03 July 2010 01:00
EXCEL Data Export in C#, VB.NET
The sample demonstrates how to export the data from spreadsheet to datatable.
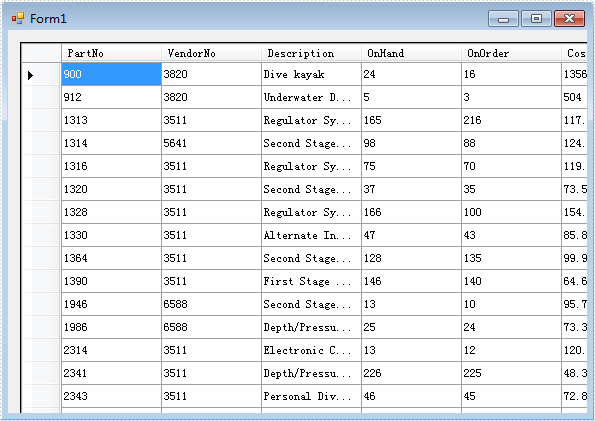
using System; using System.Data; using System.Windows.Forms; using Spire.Xls; namespace ExcelSheetToDataTable { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { Workbook workbook = new Workbook(); workbook.LoadFromFile(@"..\..\parts.xls",ExcelVersion.Version97to2003); //Initialize worksheet Worksheet sheet = workbook.Worksheets[0]; DataTable dataTable = sheet.ExportDataTable(); this.dataGridView1.DataSource = dataTable; } } }
Imports System.Data Imports System.Windows.Forms Imports Spire.Xls Namespace ExcelSheetToDataTable Public Partial Class Form1 Inherits Form Public Sub New() InitializeComponent() End Sub Private Sub button1_Click(sender As Object, e As EventArgs) Dim workbook As New Workbook() workbook.LoadFromFile("..\..\parts.xls", ExcelVersion.Version97to2003) 'Initialize worksheet Dim sheet As Worksheet = workbook.Worksheets(0) Dim dataTable As DataTable = sheet.ExportDataTable() Me.dataGridView1.DataSource = dataTable End Sub End Class End Namespace
Published in
DataTable